


PHP cannot upload files successfully, $_FILES['screenshot']['tmp_name'] is empty_PHP Tutorial
PHP cannot upload files successfully, $_FILES['screenshot']['tmp_name'] is empty
Recently, I was studying Chapter 5 of the book "HeadFirst PHP & MySQL", "Using Data Stored in Files", and when I was making a file upload application, an error occurred, that is, the file could not be uploaded successfully. This problem has troubled me for a long time, but fortunately it was finally solved. The reason is that the size of the image file I uploaded exceeds the value specified by the MAX_FILE_SIZE option in the HTML form, 32768Bytes, which is 32KB, so the upload cannot be successful.
I used XAMPP (Apache + MySQL + PHP + Perl) integrated development package and Zend Studio 10.6 is used as the PHP IDE development environment. In addition, I used XDebug for PHP debugging. To configure it in Zend Studio 10.6, I referred to the blog post Zend Studio 10.5 and XDebug Debugging | Zend Debugger Description Drupal Source Code (1)
My PHP code for addscore.php is as follows:
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en" lang="en"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Guitar Wars - Add Your High Score</title> <link rel="stylesheet" type="text/css" href="style.css" /> </head> <body> <h2 id="Guitar-Wars-Add-Your-High-Score">Guitar Wars - Add Your High Score</h2> <?php require_once 'appvars.php'; require_once 'connectvars.php'; if (isset($_POST['submit'])) { // Grab the score data from the POST $name = $_POST['name']; $score = $_POST['score']; $screenshot = $_FILES['screenshot']['name']; // echo "name: $name <br />"; // echo "score: $score <br />"; // echo "screenShot: $screenshot <br />"; if (!empty($name) && !empty($score) && !empty($screenshot)) { // Move the file to the target upload folder $target = GW_UPLOADPATH . $screenshot; echo json_encode($_FILES); if (move_uploaded_file($_FILES['screenshot']['tmp_name'], $target)) { // Connect to the database $dbc = mysqli_connect(DB_HOST, DB_USER, DB_PASSWORD, DB_NAME) or die('Error Connecting to MySQL Database!'); // Write the data to the database $query = "INSERT INTO guitarwars VALUES (0, NOW(), '$name', '$score','$screenshot')"; mysqli_query($dbc, $query) or die('Error querying database;'); // Confirm success with the user echo '<p>Thanks for adding your new high score!</p>'; echo '<p><strong>Name:</strong> ' . $name . '<br />'; echo '<strong>Score:</strong> ' . $score; echo '<img src="/static/imghw/default1.png" data-src="http://www.bkjia.com/uploads/allimg/140725/042Q21b1-0.jpg" class="lazy" . GW_UPLOADPATH . $screenshot . '" alt="Score image" /></p>'; echo '<p><< Back to high scores</p>'; // Clear the score data to clear the form $name = ""; $score = ""; $screenshot = ""; mysqli_close($dbc); } } else { echo '<p class="error">Please enter all of the information to add your high score.</p>'; } } ?> <hr /> </body> </html>
When using Zend Sutdio10.6 to debug the above PHP code, I found that the code block in the line "if (move_uploaded_file($_FILES['screenshot']['tmp_name'], $target)) {" was not executed. Check The value of the super global variable $_FILES['screenshot']['tmp_name'] is empty, and then I print out the value of the $_FILES variable in JSON format before this line of code, as follows:
{"screenshot":{"name":"Penguins.jpg","type":"","tmp_name":"","error":2,"size":0}}
The screenshot is as follows:
Then I checked that $_FILES["screenshot']['error'] was 2. I checked online and found that the $_FILES super global variable is roughly as follows:
Common usages of the $_FILES system function in the PHP programming language are: $_FILES['myFile']['name'] displays the original name of the client file. $_FILES['myFile']['type'] The MIME type of the file, for example "image/gif". $_FILES['myFile']['size'] The size of the uploaded file, in bytes. $_FILES['myFile']['tmp_name'] is the name of the temporary file stored, which is usually the system default. $_FILES['myFile']['error'] This is the error code related to file upload. The following is what the different codes mean: 0; File uploaded successfully. 1; The file size exceeds the size set by the system in php.ini. 2; File size exceeded The value specified by the MAX_FILE_SIZE option. 3; Only part of the file was uploaded. 4; No files were uploaded. 5; The uploaded file size is 0.In addition, check the PHP reference manual for the introduction of the move_uploaded_file function as follows:
move_uploaded_file (PHP 4 >= 4.0.3, PHP 5) move_uploaded_file — 将上传的文件移动到新位置 说明 bool move_uploaded_file ( string $filename , string $destination ) 本函数检查并确保由 filename 指定的文件是合法的上传文件(即通过 PHP 的 HTTP POST 上传机制所上传的)。如果文件合法,则将其移动为由 destination 指定的文件。 这种检查显得格外重要,如果上传的文件有可能会造成对用户或本系统的其他用户显示其内容的话。 参数 filename 上传的文件的文件名。 destination 移动文件到这个位置。 返回值 成功时返回 TRUE。 如果 filename 不是合法的上传文件,不会出现任何操作, move_uploaded_file() 将返回 FALSE。 如果 filename 是合法的上传文件,但出于某些原因无法移动,不会出现任何操作, move_uploaded_file() 将返回 FALSE。此外还会发出一条警告。
Example
Example #1 Uploading multiple files
<?php $uploads_dir = '/uploads'; foreach ($_FILES["pictures"]["error"] as $key => $error) { if ($error == UPLOAD_ERR_OK) { $tmp_name = $_FILES["pictures"]["tmp_name"][$key]; $name = $_FILES["pictures"]["name"][$key]; move_uploaded_file($tmp_name, "$uploads_dir/$name"); } } ?>
...
}The code block was not executed.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


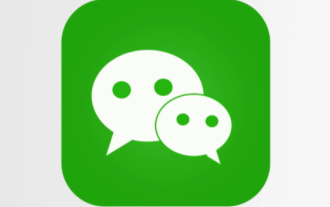
Open WeChat, select Settings in Me, select General and then select Storage Space, select Management in Storage Space, select the conversation in which you want to restore files and select the exclamation mark icon. Tutorial Applicable Model: iPhone13 System: iOS15.3 Version: WeChat 8.0.24 Analysis 1 First open WeChat and click the Settings option on the My page. 2 Then find and click General Options on the settings page. 3Then click Storage Space on the general page. 4 Next, click Manage on the storage space page. 5Finally, select the conversation in which you want to recover files and click the exclamation mark icon on the right. Supplement: WeChat files generally expire in a few days. If the file received by WeChat has not been clicked, the WeChat system will clear it after 72 hours. If the WeChat file has been viewed,
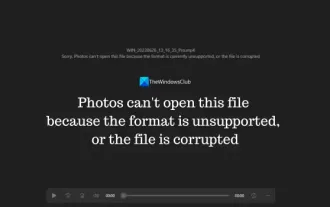
In Windows, the Photos app is a convenient way to view and manage photos and videos. Through this application, users can easily access their multimedia files without installing additional software. However, sometimes users may encounter some problems, such as encountering a "This file cannot be opened because the format is not supported" error message when using the Photos app, or file corruption when trying to open photos or videos. This situation can be confusing and inconvenient for users, requiring some investigation and fixes to resolve the issues. Users see the following error when they try to open photos or videos on the Photos app. Sorry, Photos cannot open this file because the format is not currently supported, or the file
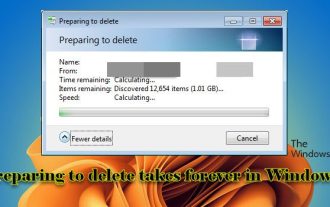
In this article, we will introduce how to solve the problem of "Ready to delete" prompt when deleting files or folders in Windows system. This prompt means that the system is performing some background operations, such as checking file permissions, verifying whether the file is occupied by other programs, calculating the size of the item to be deleted, etc. We will provide you with some workarounds to ensure that you can successfully delete your files without waiting too long. Why does Windows take so long to delete files? The time it takes Windows to prepare a file for deletion is affected by a variety of factors, including file size, storage device speed, and background processes. A long or stuck "Preparing to delete" prompt may indicate insufficient system resources, disk errors, or file system issues. exist
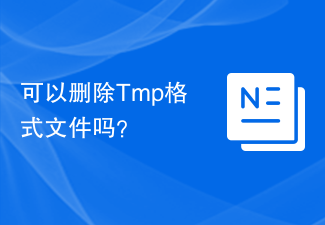
Tmp format files are a temporary file format usually generated by a computer system or program during execution. The purpose of these files is to store temporary data to help the program run properly or improve performance. Once the program execution is completed or the computer is restarted, these tmp files are often no longer necessary. Therefore, for Tmp format files, they are essentially deletable. Moreover, deleting these tmp files can free up hard disk space and ensure the normal operation of the computer. However, before deleting Tmp format files, we need to
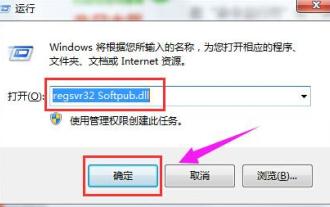
When deleting or decompressing a folder on your computer, sometimes a prompt dialog box "Error 0x80004005: Unspecified Error" will pop up. How should you solve this situation? There are actually many reasons why the error code 0x80004005 is prompted, but most of them are caused by viruses. We can re-register the dll to solve the problem. Below, the editor will explain to you the experience of handling the 0x80004005 error code. Some users are prompted with error code 0X80004005 when using their computers. The 0x80004005 error is mainly caused by the computer not correctly registering certain dynamic link library files, or by a firewall that does not allow HTTPS connections between the computer and the Internet. So how about
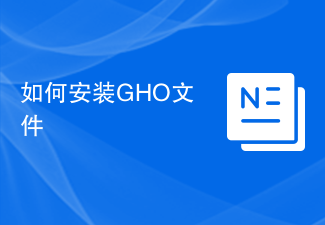
The gho file is a GhostImage image file, which is usually used to back up the entire hard disk or partition data into a file. In some specific cases, we need to reinstall this gho file back to the hard drive to restore the hard drive or partition to its previous state. The following will introduce how to install the gho file. First, before installation, we need to prepare the following tools and materials: Entity gho file: Make sure you have a complete gho file, which usually has a .gho suffix and contains a backup
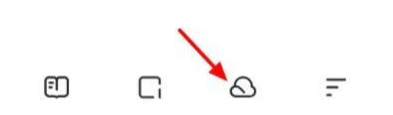
Quark Netdisk and Baidu Netdisk are currently the most commonly used Netdisk software for storing files. If you want to save the files in Quark Netdisk to Baidu Netdisk, how do you do it? In this issue, the editor has compiled the tutorial steps for transferring files from Quark Network Disk computer to Baidu Network Disk. Let’s take a look at how to operate it. How to save Quark network disk files to Baidu network disk? To transfer files from Quark Network Disk to Baidu Network Disk, you first need to download the required files from Quark Network Disk, then select the target folder in the Baidu Network Disk client and open it. Then, drag and drop the files downloaded from Quark Cloud Disk into the folder opened by the Baidu Cloud Disk client, or use the upload function to add the files to Baidu Cloud Disk. Make sure to check whether the file was successfully transferred in Baidu Cloud Disk after the upload is completed. That's it
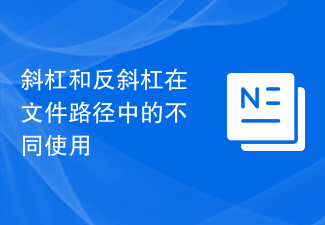
A file path is a string used by the operating system to identify and locate a file or folder. In file paths, there are two common symbols separating paths, namely forward slash (/) and backslash (). These two symbols have different uses and meanings in different operating systems. The forward slash (/) is a commonly used path separator in Unix and Linux systems. On these systems, file paths start from the root directory (/) and are separated by forward slashes between each directory. For example, the path /home/user/Docume
