Example tutorial on communication between java and php through socket
The simple function implemented by
demo is to accept the string written by the PHP end, and then return it to the output end as it is. The code is as follows:
The code is as follows:
import java.io.*; import java.net.*; public class Server { public static void main ( String [] args) throws IO Exception { System.out. print ln("Server started !\n"); ServerSocket server= new ServerSocket(5678); while (true){ Socket client=server.accept(); System.out.println("client coming!\n"); PrintWriter printer = new PrintWriter(client.getOutputStream()); BufferedReader reader = new BufferedReader(new InputStreamReader(client.getInputStream())); String m = reader. readLine (); System.out.println("get infomation " + m + "\n from " + client.getInetAddress().toString()); printer.println(m); printer. flush (); printer.close(); printer.close(); client.close(); System.out.println("client leaving!\n"); } } }
After running, the java program will listen to the 5678 port. When the message is received, the received message will be returned to the client as it is...
The PHP code is as follows:
The code is as follows:
<?php $socket = socket_create ( AF_INET, SOCK_STREAM, SOL_TCP ) or die ( 'could not create socket' ); $connect = socket_connect ( $socket, '127.0.0.1', 5678 ); //向服务端发送数据 socket_write ( $socket, 'Hello' . "\n" ); //接受服务端返回数据 $str = socket_read ( $socket, 1024, PHP_NORMAL_READ ); echo $str; //关闭 socket_close($socket);
The PHP program connects to the 5678 port of the local machine, writes Hello, and then reads the returned data...will return Data is output to the browser...
First run the java server, and then use the browser to access the PHP page. You will see the Hello returned from the server
[Related recommendations]
1. Detailed explanation of the valueOf method example in java
2. Share the example code in java that handles sticky packets during socket communication
3. Java instance-ServerSocket and Socket communication instance

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


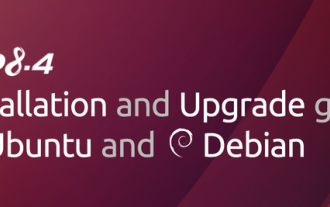
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
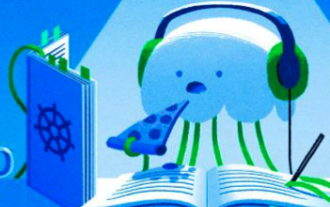
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
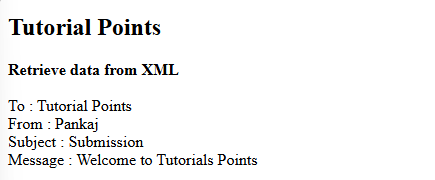
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
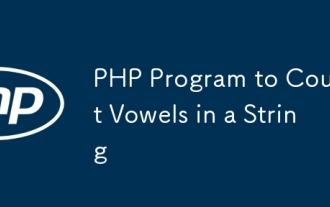
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
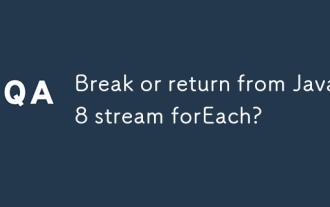
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
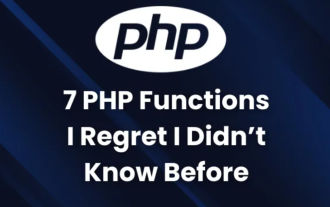
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
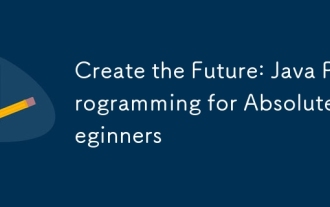
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
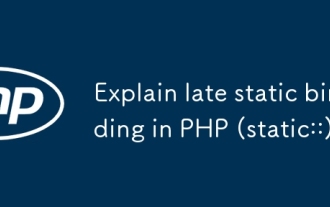
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
