5 ways to create a namespace in JavaScript_PHP Tutorial
Global variables in JavaScript often cause naming conflicts, and sometimes even rewriting variables is not in the order you imagine. You can take a look at the following example:
var sayHello = function() {
return 'Hello var';
};
function sayHello(name) {
Return 'Hello function';
};
sayHello();
The final output is
> "Hello var"
Why is this? According to StackOverFlow's explanation, JavaScript is actually parsed in the following order.
function sayHello(name) {
Return 'Hello function';
};
var sayHello = function() {
Return 'Hello var';
};
sayHello();
Function declarations without var are parsed in advance, so modern JS writing recommends that you always use prefixed var to declare all variables;
The best way to avoid global variable name conflicts is to create a namespace. Here are several common ways to create namespaces in JS.
1. Create via function
This is a relatively common way of writing. It is implemented by declaring a function, setting initial variables in the function, and writing public methods into the prototype, such as:
var NameSpace = NameSpace || {};
/*
Function
*/
NameSpace.Hello = function() {
this.name = 'world';
};
NameSpace.Hello.prototype.sayHello = function(_name) {
Return 'Hello ' + (_name || this.name);
};
var hello = new NameSpace.Hello();
hello.sayHello();
This way of writing is verbose and not conducive to code compression (jQuery uses fn instead of prototype), and it needs to be instantiated (new) before calling. Using Object to write it in JSON format can make it more compact:
2. Create Object through JSON object
/*
Object
*/
var NameSpace = NameSpace || {};
NameSpace.Hello = {
Name: 'world'
, sayHello: function(_name) {
Return 'Hello ' + (_name || this.name);
}
};
Call
NameSpace.Hello.sayHello('JS');
> Hello JS;
This way of writing is relatively compact. The disadvantage is that all variables must be declared as public, so all references to these variables need to be added with this to indicate the scope, and the writing method is also slightly redundant.
3. Implementation through Closure and Object
Declare all variables and methods in the closure, and return the public interface through a JSON Object:
var NameSpace = NameSpace || {};
NameSpace.Hello = (function() {
//Public object to be returned
var self = {};
//Private variables or methods
var name = 'world';
//Public methods or variables
self.sayHello = function(_name) {
Return 'Hello ' + (_name || name);
};
//Returned public object
return self;
}());
4. Improved writing methods of Object and closure
In the previous example, the internal call to the public method also needs to add self, such as: self.sayHello(); Here you can finally return the JSON objects of all public interfaces (methods/variables).
var NameSpace = NameSpace || {};
NameSpace.Hello = (function() {
var name = 'world';
var sayHello = function(_name) {
Return 'Hello ' + (_name || name);
};
Return {
SayHello: sayHello
};
}());
5. Concise writing of Function
This is a relatively simple implementation with a compact structure. It uses function instances and does not require instantiation (new) when calling. The solution comes from stackoverflow:
var NameSpace = NameSpace || {};
NameSpace.Hello = new function() {
var self = this;
var name = 'world';
self.sayHello = function(_name) {
Return 'Hello ' + (_name || name);
};
};
Additions are welcome.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


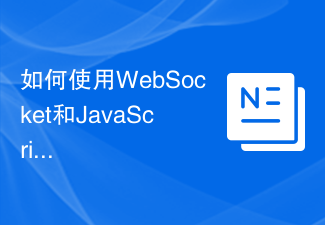
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
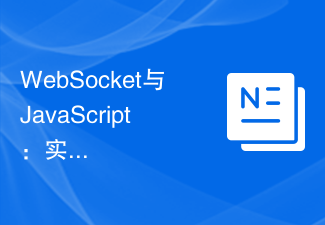
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
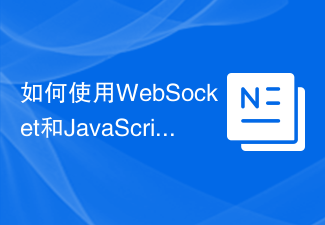
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
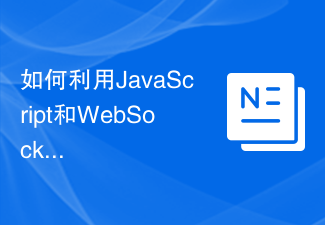
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
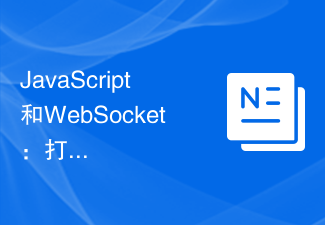
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
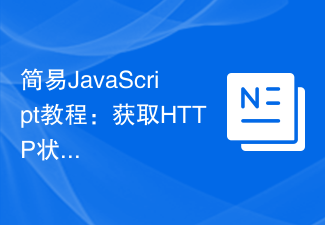
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest
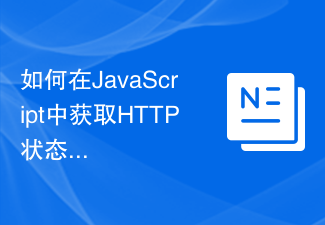
Introduction to the method of obtaining HTTP status code in JavaScript: In front-end development, we often need to deal with the interaction with the back-end interface, and HTTP status code is a very important part of it. Understanding and obtaining HTTP status codes helps us better handle the data returned by the interface. This article will introduce how to use JavaScript to obtain HTTP status codes and provide specific code examples. 1. What is HTTP status code? HTTP status code means that when the browser initiates a request to the server, the service
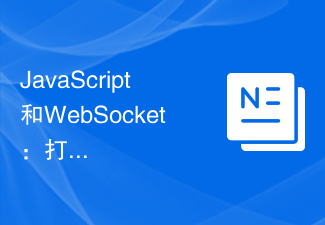
JavaScript is a programming language widely used in web development, while WebSocket is a network protocol used for real-time communication. Combining the powerful functions of the two, we can create an efficient real-time image processing system. This article will introduce how to implement this system using JavaScript and WebSocket, and provide specific code examples. First, we need to clarify the requirements and goals of the real-time image processing system. Suppose we have a camera device that can collect real-time image data
