


Sharing of 2 more classic PHP encryption and decryption functions_PHP Tutorial
Sometimes in the project we need to use PHP to encrypt specific information, that is, to generate an encrypted string through the encryption algorithm. This encrypted string can be decrypted through the decryption algorithm to facilitate the program to process the decrypted information. .
The most common applications are in user login and some API data exchange scenarios.
The author has collected some classic PHP encryption and decryption function codes to share with you. The principle of encryption and decryption is generally to use a certain encryption and decryption algorithm, add the key to the algorithm, and finally obtain the encryption and decryption results.
1. Very powerful authcode encryption function, Discuz! Classic code (with detailed explanation):
function authcode($string, $operation = 'DECODE', $key = '', $expiry = 0) {
// Dynamic key length, the same plaintext will generate different ciphertext, relying on the dynamic key
$ckey_length = 4;
// Key
$key = md5($key ? $key : $GLOBALS['discuz_auth_key']);
// Key a will participate in encryption and decryption
$keya = md5(substr($key, 0, 16));
// Key b will be used for data integrity verification
$keyb = md5(substr($key, 16, 16));
// Key c is used to change the generated ciphertext
$keyc = $ckey_length ? ($operation == 'DECODE' ? substr($string, 0, $ckey_length):
substr(md5(microtime()), -$ckey_length)) : '';
// Keys involved in calculations
$cryptkey = $keya.md5($keya.$keyc);
$key_length = strlen($cryptkey);
// Plain text, the first 10 bits are used to save the timestamp, and the data validity is verified during decryption, and the 10 to 26 bits are used to save $keyb (key b),
//Data integrity will be verified through this key when decrypting
// If it is decoding, it will start from the $ckey_length bit, because the $ckey_length bit before the ciphertext stores the dynamic key to ensure correct decryption
$string = $operation == 'DECODE' ? base64_decode(substr($string, $ckey_length)) :
sprintf('%010d', $expiry ? $expiry + time() : 0).substr(md5($string.$keyb), 0, 16).$string;
$string_length = strlen($string);
$result = '';
$box = range(0, 255);
$rndkey = array();
// Generate keybook
for($i = 0; $i <= 255; $i++) {
$rndkey[$i] = ord($cryptkey[$i % $key_length]); }
// Use a fixed algorithm to scramble the key book and increase randomness. It seems very complicated, but in fact it will not increase the strength of the ciphertext
for($j = $i = 0; $i < 256; $i++) {
$j = ($j + $box[$i] + $rndkey[$i]) % 256; $tmp = $box[$i]; $box[$i] = $box[$j]; $box[$j] = $tmp; }
// Core encryption and decryption part
for($a = $j = $i = 0; $i < $string_length; $i++) {
$a = ($a + 1) % 256; $j = ($j + $box[$a]) % 256; $tmp = $box[$a]; $box[$a] = $box[$j]; $box[$j] = $tmp; // Get the key from the key book, perform XOR, and then convert it into characters
$result .= chr(ord($string[$i]) ^ ($box[($box[$a] + $box[$j]) % 256])); }
If($operation == 'DECODE') {
// To verify data validity, please see the format of unencrypted plaintext
If((substr($result, 0, 10) == 0 || substr($result, 0, 10) - time() > 0) &&
substr($result, 10, 16) == substr(md5(substr($result, 26).$keyb), 0, 16)) {
return substr($result, 26); return ''; } else {
//Save the dynamic key in the ciphertext, which is why the same plaintext can be decrypted after producing different ciphertexts
// Because the encrypted ciphertext may contain some special characters and may be lost during the copying process, base64 encoding is used
return $keyc.str_replace('=', '', base64_encode($result)); }
}
Usage:
$key = 'www.helloweba.com';
echo authcode($str,'ENCODE',$key,0); //Encryption
$str = '56f4yER1DI2WTzWMqsfPpS9hwyoJnFP2MpC8SOhRrxO7BOk';
echo authcode($str,'DECODE',$key,0); //Decrypt
2. Encryption and decryption function encrypt():
$key_length=strlen($key);
$string=$operation=='D'?base64_decode($string):substr(md5($string.$key),0,8).$string;
$string_length=strlen($string);
$rndkey=$box=array();
$result='';
for($i=0;$i<=255;$i++){
$rndkey[$i]=ord($key[$i%$key_length]);
$box[$i]=$i;
}
for($j=$i=0;$i<256;$i++){
$j=($j+$box[$i]+$rndkey[$i])%256;
$tmp=$box[$i];
$box[$i]=$box[$j];
$box[$j]=$tmp;
}
for($a=$j=$i=0;$i<$string_length;$i++){
$a=($a+1)%256;
$j=($j+$box[$a])%256;
$tmp=$box[$a];
$box[$a]=$box[$j];
$box[$j]=$tmp;
$result.=chr(ord($string[$i])^($box[($box[$a]+$box[$j])%256]));
}
If($operation=='D'){
If(substr($result,0,8)==substr(md5(substr($result,8).$key),0,8)){
return substr($result,8);
}else{
return'';
}
}else{
return str_replace('=','',base64_encode($result));
}
}
Usage:
$key = 'www.helloweba.com';
$token = encrypt($str, 'E', $key);
echo 'Encryption:'.encrypt($str, 'E', $key);
echo 'Decrypt:'.encrypt($str, 'D', $key);

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
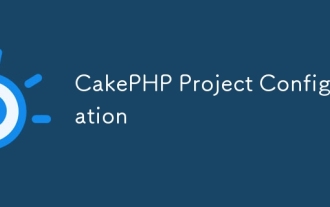
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
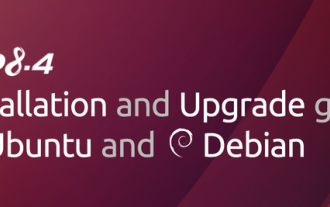
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
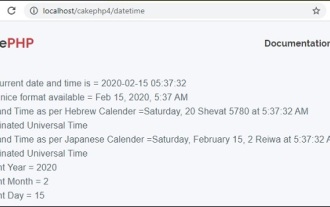
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
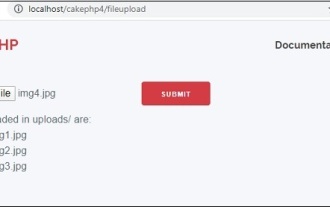
To work on file upload we are going to use the form helper. Here, is an example for file upload.
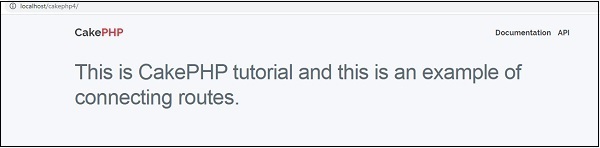
In this chapter, we are going to learn the following topics related to routing ?
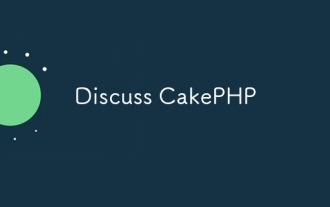
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
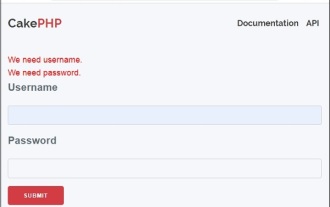
Validator can be created by adding the following two lines in the controller.
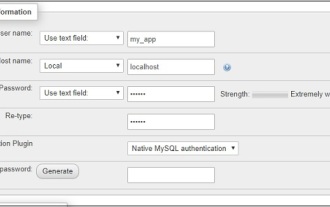
Working with database in CakePHP is very easy. We will understand the CRUD (Create, Read, Update, Delete) operations in this chapter.
