


PHP functions urlencode(), urldecode(), rawurlencode(), rawurldecode() to solve the problem of URL encoding_PHP tutorial
In PHP, there are functions such as urlencode(), urldecode(), rawurlencode(), and rawurldecode() to solve the problem of web page URL encoding and decoding.
Understanding urlencode:
urlencode: refers to an encoding conversion method for Chinese characters in web page URLs. The most common one is that when Chinese queries are entered in search engines such as Baidu and Google, an encoded web page URL is generated. There are generally two ways of urlencoding: one is the traditional GB2312-based Encode (used by Baidu, Yisou, etc.), and the other is utf-8-based Encode (used by Google, Yahoo, etc.). This article analyzes the two methods of Encode and Decode respectively.
Chinese -> Encode of GB2312 -> %D6%D0%CE%C4
Chinese -> Encode of utf-8 -> %E4%B8%AD%E6%96%87
urlencode in Html:
In the html file encoded as GB2312:
http://www.phpernote.com/中文.rar -> The browser automatically converts to -> http://www.phpernote.com/%D6%D0%CE%C4.rar
Note: Firefox does not have good support for Chinese URLs in GB2312 Encode because it sends URLs in utf-8 encoding by default, but the ftp:// protocol is fine. This should be considered a bug in Firefox.
In html file encoded as utf-8:
http://www.phpernote.com/中文.rar -> The browser automatically converts to -> http://www.phpernote.com/%E4%B8%AD%E6%96%87.rar
urlencode in PHP:
1 2 3 4 5 |
|
All non-alphanumeric characters except -_. will be replaced with a percent sign (%) followed by two hexadecimal digits.
The difference between urlencode and rawurlencode:
urlencode encodes spaces as plus signs (+)
rawurlencode encodes spaces as plus signs (%20)
My last version of the txt file splitter (online) code used urlencode. I have never found this problem. As a result, a serious bug occurred today. All URLs with spaces cannot be solved Analyzed, resulting in the split file being unable to be downloaded. Using the rawurlencode() function solves this problem.
If you want to use utf-8 Encode, there are two methods:
1. Save the file as a utf-8 file, just use urlencode or rawurlencode directly.
2. Use the mb_convert_encoding function.
1 2 3 4 |
|
Application examples:
1 2 3 4 5 6 7 8 9 10 |
|
Articles you may be interested in
- Comparison and analysis of JavaScript string encoding functions escape, encodeURI, encodeURIComponent
- PHP string replacement function str_replace is faster than preg_replace Quickly
- PHP generates continuous numeric (letter) array function range() analysis, PHP lottery program function
- String Functions in PHP Full summary
- php function to extract the birthday date from the ID number and verify whether it is a minor
- php finds whether a certain value exists in the array (in_array(), array_search(), array_key_exists())
- Use the PHP function memory_get_usage to obtain the current PHP memory consumption to optimize program performance
- Send email SMTP Error Could not connect to SMTP host. Send fail solution

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
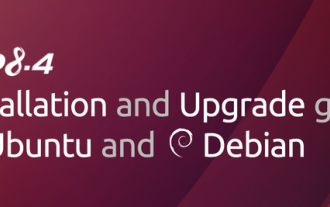
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
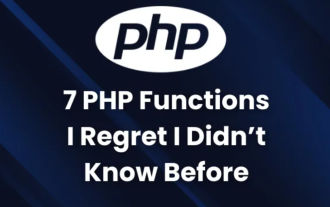
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
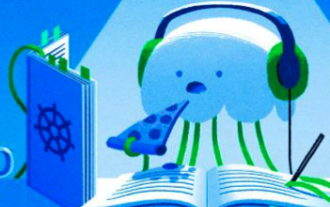
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
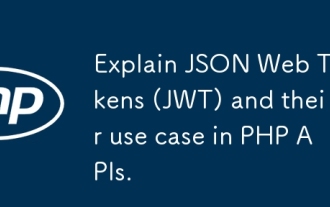
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
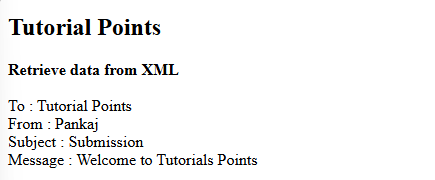
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
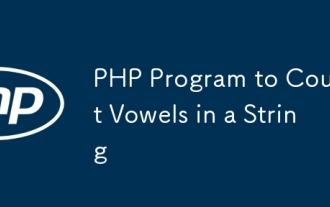
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
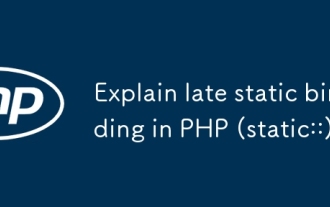
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
