


Several program applications of PHP substr() function_PHP tutorial
Introduction to substr() function
The substr() function returns a part of a string.
Syntax: substr(string,start,length).
- string: required. Specifies a part of the string to be returned.
- start: required. Specifies where in the string to begin. Positive number - starts at the specified position in the string; negative number - starts at the specified position from the end of the string; 0 - starts at the first character in the string.
- charlist: optional. Specifies the length of the string to be returned. The default is until the end of the string. Positive number - returned from the position of the start parameter; negative number - returned from the end of the string.
Note: If start is a negative number and length is less than or equal to start, length is 0.
Program List: negative start parameter
<?php $rest = substr("abcdef", -1); // returns "f" echo $rest.'<br />'; $rest = substr("abcdef", -2); // returns "ef" echo $rest.'<br />'; $rest = substr("abcdef", -3, 1); // returns "d" echo $rest.'<br />'; ?>
Program execution result:
f ef d
Program List: negative length parameter
starts from the start position. If length is a negative value, it starts counting from the end of the string. If substr("abcdef", 2, -1), it starts from c, and then -1 means to intercept to e, which means to intercept cde.
<?php $rest = substr("abcdef", 0, -1); // returns "abcde" echo $rest.'<br />'; $rest = substr("abcdef", 2, -1); // returns "cde" echo $rest.'<br />'; $rest = substr("abcdef", 4, -4); // returns "" echo $rest.'<br />'; $rest = substr("abcdef", -3, -1); // returns "de" echo $rest.'<br />'; ?>
Program execution result:
abcde cde de
Program List: Basic substr() function usage
<?php echo substr('abcdef', 1); // bcdef echo '<br />'; echo substr('abcdef', 1, 3); // bcd echo '<br />'; echo substr('abcdef', 0, 4); // abcd echo '<br />'; echo substr('abcdef', 0, 8); // abcdef echo '<br />'; echo substr('abcdef', -1, 1); // f echo '<br />'; // Accessing single characters in a string // can also be achieved using "square brackets" $string = 'abcdef'; echo $string[0]; // a echo '<br />'; echo $string[3]; // d echo '<br />'; echo $string[strlen($string)-1]; // f echo '<br />'; ?>
Program execution result:
bcdef bcd abcd abcdef f a d f
Program List: Remove suffix
<?php //removes string from the end of other function removeFromEnd($string, $stringToRemove) { // 获得需要移除的字符串的长度 $stringToRemoveLen = strlen($stringToRemove); // 获得原始字符串的长度 $stringLen = strlen($string); // 计算出需要保留字符串的长度 $pos = $stringLen - $stringToRemoveLen; $out = substr($string, 0, $pos); return $out; } $string = 'bkjia.jpg.jpg'; $result = removeFromEnd($string, '.jpg'); echo $result; ?>
Program execution result:
bkjia.jpg
Program List: If the string is too long, only the beginning and end will be displayed, and ellipses will be used instead
<?php $file = "Hellothisfilehasmorethan30charactersandthisfayl.exe"; function funclongwords($file) { if (strlen($file) > 30) { $vartypesf = strrchr($file,"."); // 获取字符创总长度 $vartypesf_len = strlen($vartypesf); // 截取左边15个字符 $word_l_w = substr($file,0,15); // 截取右边15个字符 $word_r_w = substr($file,-15); $word_r_a = substr($word_r_w,0,-$vartypesf_len); return $word_l_w."...".$word_r_a.$vartypesf; } else return $file; } // RETURN: Hellothisfileha...andthisfayl.exe $result = funclongwords($file); echo $result; ?>
Program execution result:
Hellothisfileha...andthisfayl.exe
Program List: Display extra text as ellipsis
Many times we need to display a fixed number of words, and the extra words are replaced with ellipses.
<?php $text = 'welcome to bkjia, I hope you can find something you wanted.'; $result = textLimit($text, 30); echo $result; function textLimit($string, $length, $replacer = '...') { if(strlen($string) > $length) return (preg_match('/^(.*)\W.*$/', substr($string, 0, $length+1), $matches) ? $matches[1] : substr($string, 0, $length)) . $replacer; return $string; } ?>
Program execution result:
welcome to bkjia, I hope...
Program List: Format string
Sometimes we need to format strings, such as phone numbers.
<?php function str_format_number($String, $Format) { if ($Format == '') return $String; if ($String == '') return $String; $Result = ''; $FormatPos = 0; $StringPos = 0; while ((strlen($Format) - 1) >= $FormatPos) { //If its a number => stores it if (is_numeric(substr($Format, $FormatPos, 1))) { $Result .= substr($String, $StringPos, 1); $StringPos++; //If it is not a number => stores the caracter } else { $Result .= substr($Format, $FormatPos, 1); } //Next caracter at the mask. $FormatPos++; } return $Result; } // For phone numbers at Buenos Aires, Argentina // Example 1: $String = "8607562337788"; $Format = "+00 0000 0000000"; echo str_format_number($String, $Format); echo '<br />'; // Example 2: $String = "8607562337788"; $Format = "+00 0000 00.0000000"; echo str_format_number($String, $Format); echo '<br />'; // Example 3: $String = "8607562337788"; $Format = "+00 0000 00.000 a"; echo str_format_number($String, $Format); echo '<br />'; ?>
Program execution result:
+86 0756 2337788 +86 0756 23.37788 +86 0756 23.377 a

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


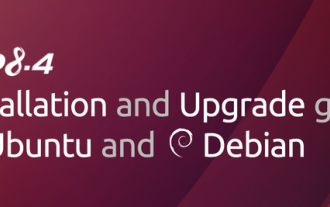
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
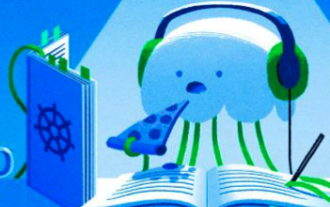
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
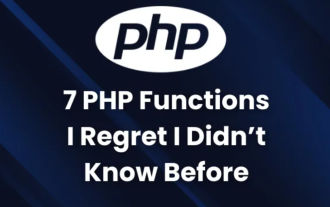
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
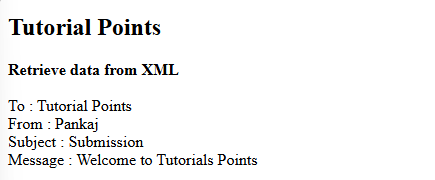
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
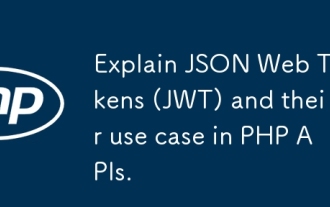
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
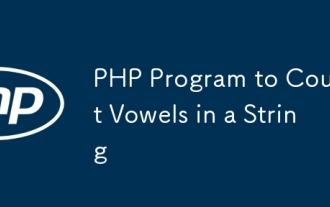
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
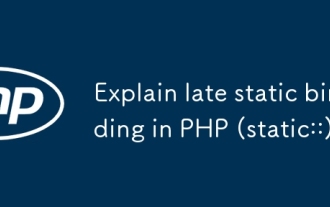
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
