PHP memory leak problem and garbage collection_PHP tutorial
Jul 13, 2016 am 10:34 AMWhen you write a php script, you generally don’t have to worry about memory leaks and garbage collection, because usually your script will finish executing and exit quickly.
But when the running time is long and the amount of data is large, after the program runs for a period of time, the php script takes up too much memory, and then exits with an error (PHP Fatal error: Allowed memory size of 134217728 bytes exhausted). Generally speaking, after each page processing is completed, the newly created simple_html_dom object should be destroyed - but in fact it is not. Obviously, a memory leak has occurred.
PHP’s garbage collection mechanism
The garbage collection mechanism used before PHP 5.3 is a simple "reference counting", that is, each memory object is allocated a counter. When the memory object is referenced by a variable, the counter + 1; when the variable reference is removed, the counter -1; when the counter = 0, it indicates that the memory object is not used, the memory object is destroyed, and garbage collection is completed.
There is a problem with "reference counting", that is, when two or more objects refer to each other to form a ring, the counter of the memory object will not be reduced to 0; at this time, this group of memory objects is no longer useful, but It cannot be recycled, causing memory leaks.
Starting from php5.3, a new garbage collection mechanism is used. Based on reference counting, a complex algorithm is implemented to detect the existence of reference rings in memory objects to avoid memory leaks.
Check whether the memory is leaked
To see if the memory that should be released has not been released, you can simply call the memory_get_usage function to check the memory usage; the memory usage data returned by the memory_get_usage function is said to be not very accurate. You can use php's xdebug extension to get more accurate Informative memory usage.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
|
The above constructs an example that will generate a circular reference. Every time an instance a of the A object is created, a creates an instance b of the B object, and lets b refer to a. In this way, each A object is always referenced by an object B, and each B object is simultaneously referenced by an object A. This is how a reference loop is created.
When you execute this code in the php5.2 environment, you will find that the memory usage is increasing monotonically, and there is no "A/B destructurt" information output after the destructors of A and B are executed; until the memory is exhausted, Output "PHP Fatal error: Allowed memory size of 134217728 bytes exhausted (tried to allocate 40 bytes)".
When this code is executed in the php5.3 environment, it is found that the memory usage jumps up and down, but it never exceeds a limit. The program will also output a large number of "A/B desctruct", which indicates that the destructor has been called.
My colleague's program has this kind of reference loop, and his script is actually executed under php5.2.3. In the simple_html_dom tool, there are two classes, namely simple_html_dom and simple_html_dom_node. The former has an array member variable nodes, and each element in the array is a simple_html_dom_node object; and each simple_html_dom_node object has a member variable dom, which is The value is the previous simple_html_dom object - thus forming a beautiful reference loop, causing a memory leak. The solution is also very simple, that is, when the simple_html_dom object is finished using, actively call its clear function and clear its member variable nodes, the loop will be broken, and memory leaks will not occur.
Others
1. Timing of garbage collection
In PHP, when the reference count is 0, the memory is released immediately. In other words, if there is no circular reference to a variable, the memory will be released immediately after leaving the scope of the variable. Circular reference detection is triggered when certain conditions are met, so in the above example, you will see large fluctuations in the memory used. You can also proactively detect circular references through the gc_collect_cycles function.
2. The influence of & symbol
Explicitly referencing a variable will increase the reference count of the memory:
1 2 |
|
At this time unset($a), but there is still a reference to $b pointing to the memory area, and the memory will not be released.
3. The impact of unset function
Unset just disconnects a variable from a memory area and reduces the reference count of the memory area by -1; in the above example, inside the loop body, $a=new A(); unset($a) ;Does not reduce $a’s reference count to zero;
4. The impact of = null operation;
$a = null directly nulls the data structure pointed to by $a and returns its reference count to 0.
5. Impact of the end of script execution
When the script execution ends, all memory used in the script will be released, regardless of whether there is a reference cycle.

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
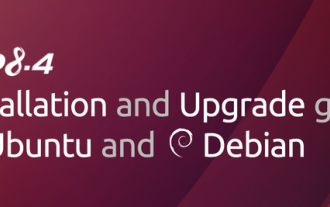
PHP 8.4 Installation and Upgrade guide for Ubuntu and Debian
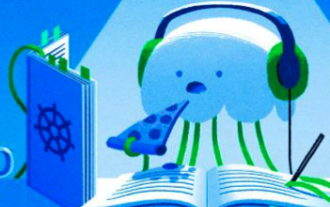
How To Set Up Visual Studio Code (VS Code) for PHP Development
