


PHP multiple serialization/deserialization methods_PHP tutorial
Serialization is the process of converting a variable into a string that can be saved or transmitted; deserialization is to convert this string into the original variable at the appropriate time for use. These two processes combine to easily store and transfer data, making the program more maintainable.
1. serialize and unserialize functions
These two are common functions for serializing and deserializing data in PHP.
<?php $a = array('a' => 'Apple' ,'b' => 'banana' , 'c' => 'Coconut'); //序列化数组 $s = serialize($a); echo $s; //输出结果:a:3:{s:1:"a";s:5:"Apple";s:1:"b";s:6:"banana";s:1:"c";s:7:"Coconut";} echo '<br /><br />'; //反序列化 $o = unserialize($s); print_r($o); //输出结果 Array ( [a] => Apple [b] => banana [c] => Coconut ) ?>
When array values contain characters such as double quotes, single quotes, or colons, problems may occur after they are deserialized. To overcome this problem, a neat trick is to use base64_encode and base64_decode.
$obj = array(); //序列化 $s = base64_encode(serialize($obj)); //反序列化 $original = unserialize(base64_decode($s));
But base64 encoding will increase the length of the string. To overcome this problem, it can be used with gzcompress.
//定义一个用来序列化对象的函数 function my_serialize( $obj ) { return base64_encode(gzcompress(serialize($obj))); } //反序列化 function my_unserialize($txt) { return unserialize(gzuncompress(base64_decode($txt))); }
2. json_encode and json_decode
Using JSON format serialization and deserialization is a good choice:
- Using json_encode and json_decode format output is much faster than serialize and unserialize format.
- JSON format is readable.
- JSON format returns smaller data results than serialize.
- The JSON format is open and portable. Other languages can use it as well.
$a = array('a' => 'Apple' ,'b' => 'banana' , 'c' => 'Coconut'); //序列化数组 $s = json_encode($a); echo $s; //输出结果:{"a":"Apple","b":"banana","c":"Coconut"} echo '<br /><br />'; //反序列化 $o = json_decode($s);
In the above example, the json_encode output length is obviously shorter than the serialize output length in the previous example.
3. var_export and eval
The var_export function outputs the variable as a string; eval executes the string as a PHP code and deserializes it to obtain the content of the original variable.
$a = array('a' => 'Apple' ,'b' => 'banana' , 'c' => 'Coconut'); //序列化数组 $s = var_export($a , true); echo $s; //输出结果: array ( 'a' => 'Apple', 'b' => 'banana', 'c' => 'Coconut', ) echo '<br /><br />'; //反序列化 eval('$my_var=' . $s . ';'); print_r($my_var);
4. wddx_serialize_value and wddx deserialize
The wddx_serialize_value function can serialize array variables and output them as XML strings.
$a = array('a' => 'Apple' ,'b' => 'banana' , 'c' => 'Coconut'); //序列化数组 $s = wddx_serialize_value($a); echo $s; //输出结果(查看输出字符串的源码):<wddxPacket version='1.0'><header/><data><struct><var name='a'><string>Apple</string></var><var name='b'><string>banana</string></var><var name='c'><string>Coconut</string></var></struct></data></wddxPacket> echo '<br /><br />'; //反序列化 $o = wddx_deserialize($s); print_r($o); //输出结果:Array ( [a] => Apple [b] => banana 1 => Coconut )
It can be seen that there are many XML tag characters, so the serialization of this format still takes up a lot of space.
Summary
All the above functions can be executed normally when serializing array variables, but it is different when applied to objects. For example, json_encode serialized objects will fail. When deserializing an object, unserialize and eval will have different effects.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
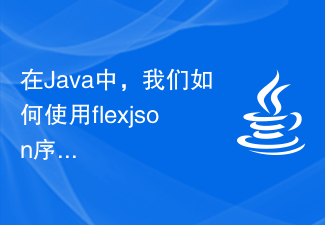
Flexjson is a lightweight library for serializing and deserializing Java objects to and from JSON format. We can serialize a list of objects using the serialize() method of the JSONSerializer class. This method performs shallow serialization on the target instance. We need to pass a list of objects of list type as a parameter to the serialize() method. Syntax publicStringserialize(Objecttarget) example importflexjson.JSONSerializer;importjava.util.*;publicclassJsonSerial
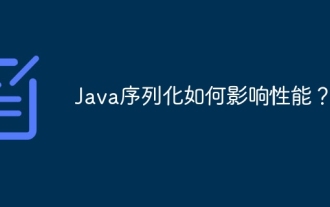
The impact of serialization on Java performance: The serialization process relies on reflection, which will significantly affect performance. Serialization requires the creation of a byte stream to store object data, resulting in memory allocation and processing costs. Serializing large objects consumes a lot of memory and time. Serialized objects increase load when transmitted over the network.

@JsonPropertyOrder is an annotation used at class level. It takes as an attribute a list of fields that defines the order in which the fields appear in the string generated by the JSON serialization of the object. Properties included in the annotation declaration can be serialized first (in the order they are defined), followed by any properties not included in the definition. Syntax public@interfaceJsonPropertyOrder Example importcom.fasterxml.jackson.core.*;importcom.fasterxml.jackson.databind.*;importcom.fasterxml.jac
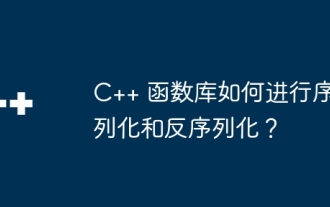
C++ Library Serialization and Deserialization Guide Serialization: Creating an output stream and converting it to an archive format. Serialize objects into archive. Deserialization: Creates an input stream and restores it from archive format. Deserialize objects from the archive. Practical example: Serialization: Creating an output stream. Create an archive object. Create and serialize objects into the archive. Deserialization: Create an input stream. Create an archive object. Create objects and deserialize them from the archive.
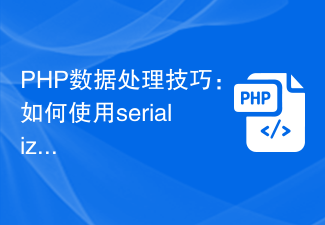
PHP data processing skills: How to use the serialize and unserialize functions to implement data serialization and deserialization Serialization and deserialization are one of the commonly used data processing skills in computer science. In PHP, we can use the serialize() and unserialize() functions to implement data serialization and deserialization operations. This article will give you a detailed introduction to how to use these two functions and provide relevant code examples. 1. What is serialization and deserialization in computer programming?
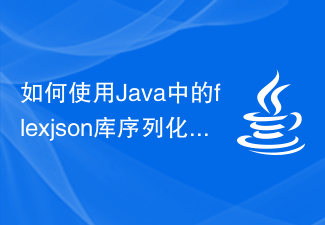
Flexjson is a lightweight library for serializing and deserializing Java objects to and from JSON format. We can also serialize a Map using the serialize() method of the JSONSerializer class, which performs shallow serialization on the target instance. Syntax publicStringserialize(Objecttarget) example importflexjson.JSONSerializer;importjava.util.*;publicclassJsonSerializeMapTest{ publ
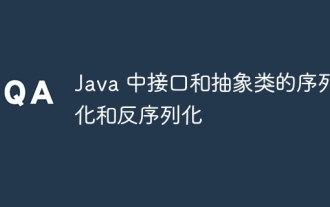
Interfaces cannot be serialized directly. Abstract classes can be serialized but only if they do not contain non-static, non-transient fields or override the writeObject() and readObject() methods. Specific instances can be implemented through concrete classes that implement the interface or override writeObject() and readObject. Abstract class implementation of () method.
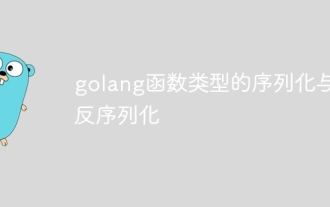
GoLang function types can be serialized and deserialized through the encoding/gob package. Serialization: Register a custom type and use gob.NewEncoder to encode the function type into a byte array. Deserialization: Use gob.NewDecoder to deserialize function types from byte arrays.
