


PHP WeChat public platform development Custom menu function_PHP tutorial
[PHP WeChat public platform development series]
01. Configure WeChat interface
02. Public platform sample code analysis
03. Subscription event (subscribe) processing
04. Development of simple reply function
05. Weather forecast function development
06. Translation function development
07. Chatbot function development
08. Custom menu function
URL of this article: http://www.phpchina.com/archives/view-43404-1.html
This series is contributed by PHPChina's specially invited author @David_Tang. Please indicate the author's information and the address of this article when reprinting.
1. Introduction
The WeChat public platform service account and subscription account that successfully applied for internal beta qualification before have the function of customizing menus. Developers can use this function to add a custom menu to the bottom of the public account's conversation interface. Users can click on the options in the menu to bring up the corresponding reply information or web link. The customized menu interface will provide more possibilities for the information display space of public accounts. This article will do a simple development application for custom menus for readers’ reference.
2. Official description
After developers obtain the usage credentials, they can use the credentials to create, query, and delete the custom menu of the public account. The custom menu interface can implement the following types of buttons:
click (click event):
After the user clicks the click type button, the WeChat server will push the click event to the developer through the message interface (event type), and bring the key value filled in by the developer in the button. The developer can reply to the message through the customized key value.
view (visit web page):
After the user clicks the view type button, it will jump directly to the URL specified by the developer.
After creating a custom menu, it will take 24 hours for the WeChat client to display it due to cache on the WeChat client. It is recommended that when testing, you can try to unfollow the public account and follow it again, and you can see the effect after creation.
Document address: http://mp.weixin.qq.com/wiki/index.php?title=%E8%87%AA%E5%AE%9A%E4%B9%89%E8%8F%9C%E5% 8D%95%E6%8E%A5%E5%8F%A3
3. Obtain usage voucher
3.1 Get appid and appsecret
Find the appid and appsecret in WeChat Public Platform > Advanced Functions > Development Mode.
3.2 Use appid and appsecret to request access_token from the WeChat credential acquisition interface
Request address: https://api.weixin.qq.com/cgi-bin/token?grant_type=client_credential&appid=APPID&secret=APPSECRET
Request parameter description:
grant_type: Get access_token and fill in client_credential
appid: unique credential for third-party users
secret: The unique credential key for third-party users, namely appsecret
Return instructions:
Correct Json return result:
{"access_token":"ACCESS_TOKEN","expires_in":7200}
Return parameter description:
access_token: Obtained certificate
expires_in: voucher validity time, unit: seconds
3.3 Specific implementation
a. Print out the format

<?php $APPID="wxdxxxxxxxxxxxxxxx"; $APPSECRET="96xxxxxxxxxxxxxxxxxxxxxxxxxxxxxx"; $TOKEN_URL="https://api.weixin.qq.com/cgi-bin/token?grant_type=client_credential&appid=".$APPID."&secret=".$APPSECRET; $json=file_get_contents($TOKEN_URL); $result=json_decode($json,true); print_r($result); ?>

The results are as follows:
b. Get access_token

<?php $APPID="wxdxxxxxxxxxxxxxxx"; $APPSECRET="96xxxxxxxxxxxxxxxxxxxxxxxxxxxxxx"; $TOKEN_URL="https://api.weixin.qq.com/cgi-bin/token?grant_type=client_credential&appid=".$APPID."&secret=".$APPSECRET; $json=file_get_contents($TOKEN_URL); $result=json_decode($json,true); $ACC_TOKEN=$result['access_token']; echo $ACC_TOKEN; ?>

Note: access_token corresponds to the public account and is a globally unique ticket. Repeated acquisition will cause the last access_token to become invalid.
4. Create menu
Method: POST a specific structure to create a custom menu on the WeChat client.
Request address: https://api.weixin.qq.com/cgi-bin/menu/create?access_token=ACCESS_TOKEN
Request example:

{ "button":[ { "name":"公共查询", "sub_button":[ { "type":"click", "name":"天气查询", "key":"tianQi" }, { "type":"click", "name":"公交查询", "key":"gongJiao" }, { "type":"click", "name":"翻译", "key":"fanYi" }] }, { "name":"苏州本地", "sub_button":[ { "type":"click", "name":"爱上苏州", "key":"loveSuzhou" }, { "type":"click", "name":"苏州景点", "key":"suzhouScenic" }, { "type":"click", "name":"苏州美食", "key":"suzhouFood" }, { "type":"click", "name":"住在苏州", "key":"liveSuzhou" }] }, { "type":"click", "name":"联系我们", "key":"lianxiUs" }] }

Example description:
Menu structure and description:

{ "button":[ //button定义该结构为一个菜单 { "name":"分支主菜单名", "sub_button":[ //sub_button定义子菜单 { "type":"click", //按钮类型 "name":"分支子菜单名1", //菜单名称 "key":"loveSuzhou" //菜单key值 }, { "type":"click", "name":"分支子菜单名2", "key":"liveSuzhou" }] }, //菜单之间用 , 分隔 { "type":"click", "name":"独立菜单", "key":"lianxiUs" }] }

Return instructions:
Correct Json return result:
{"errcode":0,"errmsg":"ok"}
Submit menu:
Submit the above menu data through curl, the code is as follows:

$MENU_URL="https://api.weixin.qq.com/cgi-bin/menu/create?access_token=".$ACC_TOKEN; $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $MENU_URL); curl_setopt($ch, CURLOPT_CUSTOMREQUEST, "POST"); curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, FALSE); curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, FALSE); curl_setopt($ch, CURLOPT_USERAGENT, 'Mozilla/5.0 (compatible; MSIE 5.01; Windows NT 5.0)'); curl_setopt($ch, CURLOPT_FOLLOWLOCATION, 1); curl_setopt($ch, CURLOPT_AUTOREFERER, 1); curl_setopt($ch, CURLOPT_POSTFIELDS, $data); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $info = curl_exec($ch); if (curl_errno($ch)) { echo 'Errno'.curl_error($ch); } curl_close($ch); var_dump($info);

运行结果:
测试结果:
菜单创建成功。
五、查询菜单
查询当前使用的自定义菜单结构。
请求地址:https://api.weixin.qq.com/cgi-bin/menu/get?access_token=ACCESS_TOKEN
curl 代码如下:

$MENU_URL="https://api.weixin.qq.com/cgi-bin/menu/get?access_token=".$ACC_TOKEN; $cu = curl_init(); curl_setopt($cu, CURLOPT_URL, $MENU_URL); curl_setopt($cu, CURLOPT_RETURNTRANSFER, 1); $menu_json = curl_exec($cu); $menu = json_decode($menu_json); curl_close($cu); echo $menu_json;

运行结果:
菜单查询成功。
六、删除菜单
取消当前使用的自定义菜单。
请求地址:https://api.weixin.qq.com/cgi-bin/menu/delete?access_token=ACCESS_TOKEN
curl 代码如下:

$MENU_URL="https://api.weixin.qq.com/cgi-bin/menu/delete?access_token=".$ACC_TOKEN; $cu = curl_init(); curl_setopt($cu, CURLOPT_URL, $MENU_URL); curl_setopt($cu, CURLOPT_RETURNTRANSFER, 1); $info = curl_exec($cu); $res = json_decode($info); curl_close($cu); if($res->errcode == "0"){ echo "菜单删除成功"; }else{ echo "菜单删除失败"; }

运行结果:
测试结果:
菜单删除成功。

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


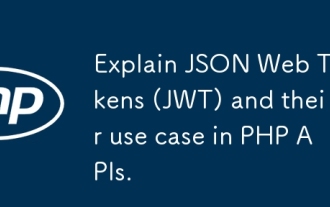
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
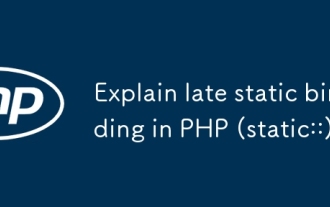
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
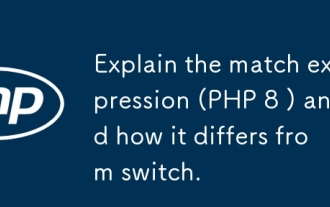
In PHP8, match expressions are a new control structure that returns different results based on the value of the expression. 1) It is similar to a switch statement, but returns a value instead of an execution statement block. 2) The match expression is strictly compared (===), which improves security. 3) It avoids possible break omissions in switch statements and enhances the simplicity and readability of the code.

In PHP, you can effectively prevent CSRF attacks by using unpredictable tokens. Specific methods include: 1. Generate and embed CSRF tokens in the form; 2. Verify the validity of the token when processing the request.
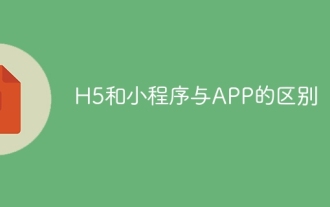
H5. The main difference between mini programs and APP is: technical architecture: H5 is based on web technology, and mini programs and APP are independent applications. Experience and functions: H5 is light and easy to use, with limited functions; mini programs are lightweight and have good interactiveness; APPs are powerful and have smooth experience. Compatibility: H5 is cross-platform compatible, applets and APPs are restricted by the platform. Development cost: H5 has low development cost, medium mini programs, and highest APP. Applicable scenarios: H5 is suitable for information display, applets are suitable for lightweight applications, and APPs are suitable for complex functions.
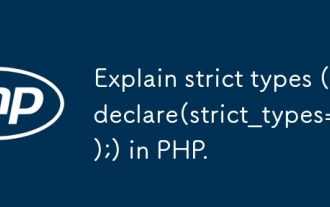
Strict types in PHP are enabled by adding declare(strict_types=1); at the top of the file. 1) It forces type checking of function parameters and return values to prevent implicit type conversion. 2) Using strict types can improve the reliability and predictability of the code, reduce bugs, and improve maintainability and readability.
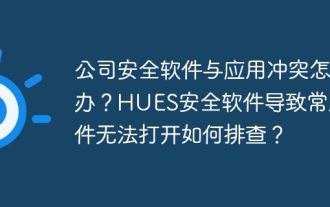
Compatibility issues and troubleshooting methods for company security software and application. Many companies will install security software in order to ensure intranet security. However, security software sometimes...
