


PHP multifunctional image processing class sharing (php image scaling class)_PHP tutorial
/**
* Basic image processing, used to complete image indentation and watermark addition
* When the watermark image exceeds the target image size, the watermark image can automatically adapt to the target image and shrink
* The watermark image can be set to match the background Merger degree
*/
/*
Usage:
Automatic cropping:
The program will follow the shape of the picture Size: Cut the largest square from the middle and shrink it according to the target size
$t--->setSrcImg("img/test.jpg");
$t->setCutType( 1);//This sentence is OK
$t->setDstImg("tmp/new_test.jpg");
$t->createImg(60,60);
Manual cutting:
The program will take the image from the source image according to the specified position
$t->setSrcImg("img/test.jpg");
$t->setCutType (2);//Indicate manual cutting
$t->setSrcCutPosition(100, 100);//Coordinates of the starting point of the source image
$t->setRectangleCut(300, 200);//Cut Cut size
$t->setDstImg("tmp/new_test.jpg");
$t->createImg(300,200);
*/
class ThumbHandler
{
var $dst_img;// Target file = 100;// Image generation quality
var $img_display_quality = 80;// Image display quality, the default is 75
var $img_scale = 0;// Image scaling ratio
var $src_w = 0;/ / Original image width
var $src_h = 0;// Original image height
var $dst_w = 0;// New image total width
var $dst_h = 0;// New image total height
var $fill_w;// width of filled graphics
var $fill_h;// height of filled graphics
var $copy_w;// width of copied graphics
var $copy_h;// height of copied graphics
var $src_x = 0;// The starting abscissa of the original image drawing
var $src_y = 0;// The starting ordinate of the original image drawing
var $start_x;// The starting abscissa of the new image drawing
var $start_y;// The vertical coordinate of the starting point for drawing a new picture
var $mask_pos_y = 0;// vertical coordinate of the watermark
var $mask_offset_x = 5;// horizontal offset of the watermark
var $mask_offset_y = 5;// vertical offset of the watermark
var $font_w ;//Watermark font width
var $font_h;// Watermark font height
var $mask_w;// Watermark width
var $mask_h;// Watermark height
var $mask_font_color = "#ffffff ";// Watermark text color
var $mask_font = 2;// Watermark font
var $font_size;// Size
var $mask_position = 0;// Watermark position
var $mask_img_pct = 50;// The degree of image merging, the larger the value, the lower the merging process.
var $mask_txt_pct = 50;// The degree of text merging, the smaller the value, the lower the merging process.
var $img_border_size = 0;// Image border size
var $img_border_color;// Image border color
var $_flip_x=0;// Number of horizontal flips
var $_flip_y=0;// Number of vertical flips
var $cut_type=0;// Cut type
var $img_type;//File type
// File type definition, and points out the function for outputting images => array("output"=>"imagegif"),
"png" => array("output"=>"imagepng"),
"wbmp" => array(" output"=>"image2wbmp"), function ThumbHandler()
{ $this->mask_font_color = "#ffffff";
$this->font = 2;
$this->font_size = 12;
} /* *
* Constructor
*/
function getImgHeight($src)
{
return imagesy($src); function setSrcImg($src_img, $img_type=null)
if(!empty($img_type))
{ $this->img_type = $img_type;
else
{ = $this->_getImgType($src_img);
} }
$this->_checkValid($this->img_type);
// file_get_contents function requires php version >4.3.0
}
else
{
$handle = fopen ($src_img, "r");
while (!feof ($handle))
{
$src .= fgets($fd, 4096);
}
fclose ($handle);
}
if(empty($src))
{
die("图片源为空");
}
$this->h_src = @ImageCreateFromString($src);
$this->src_w = $this->getImgWidth($this->h_src);
$this->src_h = $this->getImgHeight($this->h_src);
}
/** */
function setDstImg($dst_img)
{
$arr = explode('/',$dst_img);
$last = array_pop($arr);
$path = implode('/',$arr);
$this->_mkdirs($path);
$this->dst_img = $dst_img;
}
/** */
function setImgDisplayQuality($n)
{
$this->img_display_quality = (int)$n;
}
/** */
function setImgCreateQuality($n)
{
$this->img_create_quality = (int)$n;
}
/** $color Watermark font color
*/
function setMaskWord($word)
{
$this->mask_word .= $word;
}
/** */
function setMaskFontColor($color="#ffffff")
{
$this->mask_font_color = $color;
}
/** */
function setMaskFont($font=2)
{
if(!is_numeric($font) && !file_exists($font))
{
die("字体文件不存在");
}
$this->font = $font;
}
/**
* Set the text font size, only valid for truetype fonts
*/
function setMaskFontSize($size = "12")
{
$this->font_size = $size;
}
/** */
function setMaskImg($img)
{
$this->mask_img = $img;
}
/** */
function setMaskOffsetX($x)
{
$this->mask_offset_x = (int)$x;
}
/** */
function setMaskOffsetY($y)
{
$this->mask_offset_y = (int)$y;
}
/**
* Specify the watermark position
* * @param integer $position position, 1: upper left, 2: lower left, 3: upper right, 0/4: lower right
*/
function setMaskPosition($position=0)
{
$this->mask_position = (int)$position;
}
/** */
function setMaskImgPct($n)
{
$this->mask_img_pct = (int)$n;
}
/** */
function setMaskTxtPct($n)
{
$this->mask_txt_pct = (int)$n;
}
/** */
function setDstImgBorder($size=1, $color="#000000")
{
$this->img_border_size = (int)$size;
$this->img_border_color = $color;
}
/** */
function flipH()
{
$this->_flip_x++;
}
/**
* Vertical flip
*/
function flipV()
{
$this->_flip_y++;
}
/** */
function setCutType($type)
{
$this->cut_type = (int)$type;
}
/** */
function setRectangleCut($width, $height)
{
$this->fill_w = (int)$width;
$this->fill_h = (int)$height;
}
/** */
function setSrcCutPosition($x, $y)
{
$this->src_x = (int)$x;
$this->src_y = (int)$y;
}
/**
* Create image, main function * @param integer $a When the second parameter is missing, this parameter will be used as a percentage,
* Otherwise, it will be used as the width value
* @param integer $b The height of the image after scaling
*/
function createImg($a, $b=null)
{ $num = func_num_args();
if(1 == $num) die("The image scaling ratio must not be less than 1");
🎜> if(2 == $num)
🎜> die("Target width cannot is 0"); _setNewImgSize($w, $h); H($this- >h_src); gt;h_src) ;
destroy($this-> h_src) && imagedestroy($this->h_dst))
{
Return true;
}
else
{
Return false;
}
}
/**
* To generate a watermark, two methods are called to generate watermark text and watermark image.
*/
function _createMask()
{
if($this->mask_word)
{
// 获取字体信息
$this->_setFontInfo();
if($this->_isFull())
{
die("水印文字过大");
}
else
{
$this->h_dst = imagecreatetruecolor($this->dst_w, $this->dst_h);
$white = ImageColorAllocate($this->h_dst,255,255,255);
imagefilledrectangle($this->h_dst,0,0,$this->dst_w,$this->dst_h,$white);// 填充背景色
$this->_drawBorder();
imagecopyresampled( $this->h_dst, $this->h_src,
$this->start_x, $this->start_y,
$this->src_x, $this->src_y,
$this->fill_w, $this->fill_h,
$this->copy_w, $this->copy_h);
$this->_createMaskWord($this->h_dst);
}
}
if($this->mask_img)
{ $this->_loadMaskImg();//When loading, get the width and height
if( $this- > 🎜> $this->h_dst = imagecreatetruecolor($this->dst_w, $this->dst_h); >h_dst,0, 0,$this->dst_w,$this->dst_h,$white);// Fill background color this->h_src, ->src_x, $this->src_y, $this->start_y,
else
{
// 创建新图并拷贝
$this->h_dst = imagecreatetruecolor($this->dst_w, $this->dst_h);
$white = ImageColorAllocate($this->h_dst,255,255,255);
imagefilledrectangle($this->h_dst,0,0,$this->dst_w,$this->dst_h,$white);// 填充背景色
$this->_drawBorder();
imagecopyresampled( $this->h_dst, $this->h_src,
$this->start_x, $this->start_y,
$this->src_x, $this->src_y,
$this->fill_w, $this->fill_h,
$this->copy_w, $this->copy_h);
$this->_createMaskImg($this->h_dst);
}
}
if(empty($this->mask_word) && empty($this->mask_img))
{
$this->h_dst = imagecreatetruecolor($this->dst_w, $this->dst_h);
$white = ImageColorAllocate($this->h_dst,255,255,255);
imagefilledrectangle($this->h_dst,0,0,$this->dst_w,$this->dst_h,$white);// 填充背景色
$this->_drawBorder();
imagecopyresampled( $this->h_dst, $this->h_src,
$this->start_x, $this->start_y,
$this->src_x, $this->src_y,
$this->fill_w, $this->fill_h,
$this->copy_w, $this->copy_h);
}
}
/** */
function _drawBorder()
{
if(!empty($this->img_border_size))
{
$c = $this->_parseColor($this->img_border_color);
$color = ImageColorAllocate($this->h_src,$c[0], $c[1], $c[2]);
imagefilledrectangle($this->h_dst,0,0,$this->dst_w,$this->dst_h,$color);// 填充背景色
}
}
/**
* Generate watermark text
*/
function _createMaskWord($src)
{
$this->_countMaskPos();
$this->_checkMaskValid();
$c = $this->_parseColor($this->mask_font_color);
$color = imagecolorallocatealpha($src, $c[0], $c[1], $c[2], $this->mask_txt_pct);
if(is_numeric($this->font))
{
imagestring($src,
$this->font,
$this->mask_pos_x, $this->mask_pos_y,
$this->mask_word,
$color);
}
else
{
imagettftext($src,
$this->font_size, 0,
$this->mask_pos_x, $this->mask_pos_y,
$color,
$this->font,
$this->mask_word);
}
}
/**
* Generate watermark image
*/
function _createMaskImg($src)
{
$this->_countMaskPos();
$this->_checkMaskValid();
imagecopymerge($src,
$this->h_mask,
$this->mask_pos_x ,$this->mask_pos_y,
0, 0,
$this->mask_w, $this->mask_h,
$this->mask_img_pct);
imagedestroy($this->h_mask);
}
/**
* Load watermark image
*/
function _loadMaskImg()
{
$mask_type = $this->_getImgType($this->mask_img);
$this->_checkValid($mask_type);
// file_get_contents函数要求php版本>4.3.0
$src = '';
if(function_exists("file_get_contents"))
{
$src = file_get_contents($this->mask_img);
}
else
{
$handle = fopen ($this->mask_img, "r");
while (!feof ($handle))
{
$src .= fgets($fd, 4096);
}
fclose ($handle);
}
if(empty($this->mask_img))
{
die("水印图片为空");
}
$this->h_mask = ImageCreateFromString($src);
$this->mask_w = $this->getImgWidth($this->h_mask);
$this->mask_h = $this->getImgHeight($this->h_mask);
}
/** */
function _output()
{
$img_type = $this->img_type;
$func_name = $this->all_type[$img_type]['output'];
if(function_exists($func_name))
{
// 判断浏览器,若是IE就不发送头
if(isset($_SERVER['HTTP_USER_AGENT']))
{
$ua = strtoupper($_SERVER['HTTP_USER_AGENT']);
if(!preg_match('/^.*MSIE.*)$/i',$ua))
{
header("Content-type:$img_type");
}
}
$func_name($this->h_dst, $this->dst_img, $this->img_display_quality);
}
else
{
Return false;
}
}
/**
* 分析颜色
*
* @param string $color 十六进制颜色
*/
function _parseColor($color)
{
$arr = array();
for($ii=1; $ii
{
switch($this->mask_position)
{
case 1:
// 左上
$this->mask_pos_x = $this->mask_offset_x + $this->img_border_size;
$this->mask_pos_y = $this->mask_offset_y + $this->img_border_size;
break;
case 2:
// 左下
$this->mask_pos_x = $this->mask_offset_x + $this->img_border_size;
$this->mask_pos_y = $this->src_h - $this->mask_h - $this->mask_offset_y;
break;
case 3:
// 右上
$this->mask_pos_x = $this->src_w - $this->mask_w - $this->mask_offset_x;
$this->mask_pos_y = $this->mask_offset_y + $this->img_border_size;
break;
case 4:
// 右下
$this->mask_pos_x = $this->src_w - $this->mask_w - $this->mask_offset_x;
$this->mask_pos_y = $this->src_h - $this->mask_h - $this->mask_offset_y;
break;
default:
// 默认将水印放到右下,偏移指定像素
$this->mask_pos_x = $this->src_w - $this->mask_w - $this->mask_offset_x;
$this->mask_pos_y = $this->src_h - $this->mask_h - $this->mask_offset_y;
break;
}
}
else
{
switch($this->mask_position)
{
case 1:
// 左上
$this->mask_pos_x = $this->mask_offset_x + $this->img_border_size;
$this->mask_pos_y = $this->mask_offset_y + $this->img_border_size;
break;
case 2:
// 左下
$this->mask_pos_x = $this->mask_offset_x + $this->img_border_size;
$this->mask_pos_y = $this->dst_h - $this->mask_h - $this->mask_offset_y - $this->img_border_size;
break;
case 3:
// 右上
$this->mask_pos_x = $this->dst_w - $this->mask_w - $this->mask_offset_x - $this->img_border_size;
$this->mask_pos_y = $this->mask_offset_y + $this->img_border_size;
break;
case 4:
// 右下
$this->mask_pos_x = $this->dst_w - $this->mask_w - $this->mask_offset_x - $this->img_border_size;
$this->mask_pos_y = $this->dst_h - $this->mask_h - $this->mask_offset_y - $this->img_border_size;
break;
default:
// 默认将水印放到右下,偏移指定像素
$this->mask_pos_x = $this->dst_w - $this->mask_w - $this->mask_offset_x - $this->img_border_size;
$this->mask_pos_y = $this->dst_h - $this->mask_h - $this->mask_offset_y - $this->img_border_size;
break;
}
}
}
/**
* Set font information
*/
function _setFontInfo()
{
if(is_numeric($this->font))
{
$this->font_w = imagefontwidth($this->font);
$this->font_h = imagefontheight($this->font);
// 计算水印字体所占宽高
$word_length = strlen($this->mask_word);
$this->mask_w = $this->font_w*$word_length;
$this->mask_h = $this->font_h;
}
else
{
$arr = imagettfbbox ($this->font_size,0, $this->font,$this->mask_word);
$this->mask_w = abs($arr[0] - $arr[2]);
$this->mask_h = abs($arr[7] - $arr[1]);
}
}
/**
* Set new image size
* * @param integer $img_w Target width
* @param integer $img_h Target height
*/
function _setNewImgSize($img_w, $img_h=null)
{
$num = func_num_args();
if(1 == $num)
{
$this->img_scale = $img_w;// 宽度作为比例
$this->fill_w = round($this->src_w * $this->img_scale / 100) - $this->img_border_size*2;
$this->fill_h = round($this->src_h * $this->img_scale / 100) - $this->img_border_size*2;
// 源文件起始坐标
$this->src_x = 0;
$this->src_y = 0;
$this->copy_w = $this->src_w;
$this->copy_h = $this->src_h;
// 目标尺寸
$this->dst_w = $this->fill_w + $this->img_border_size*2;
$this->dst_h = $this->fill_h + $this->img_border_size*2;
}
if(2 == $num)
{ $fill_w = (int)$img_w - $this->img_border_size*2;
$fill_h = (int)$img_h -$ this-& gt; img_border_size*2;
if ($ fill_w & lt; 0 || $ fill_h & lt; 0)
{
DIE ("The picture frame is too large, it has exceeded the width of the picture" " );

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


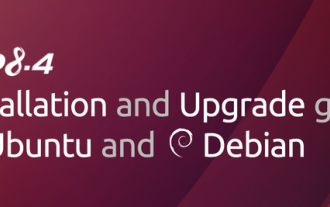
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
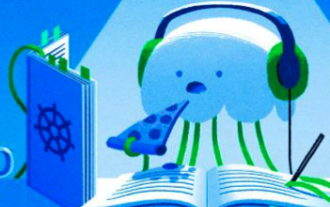
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
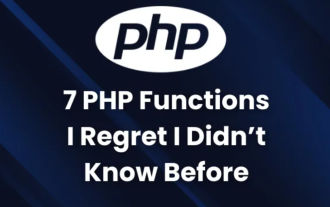
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
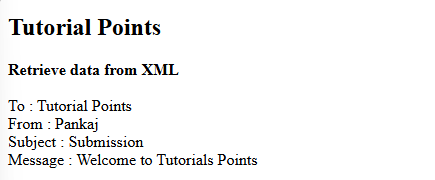
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
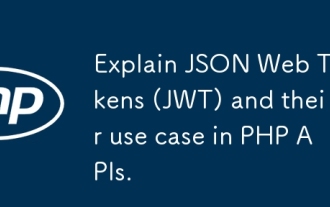
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
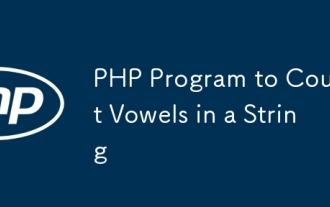
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
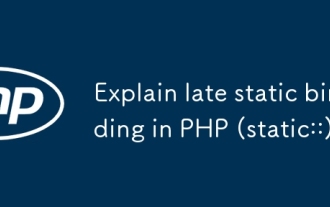
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
