


PHP Object-Oriented Journey: Static Variables and Methods_PHP Tutorial
The static keyword declares that an attribute or method is related to the class, rather than to a specific instance of the class. Therefore, this type of attribute or method is also called "class attribute" or "class method" ".
If access control permissions allow, you can call it directly using the class name plus two colons "::" without creating an object of this class.
The static keyword can be used to modify variables and methods.
You can directly access the static attributes and static methods in the class without instantiation.
Static properties and methods can only access static properties and methods, and non-static properties and methods cannot be accessed by class. Because when static properties and methods are created, there may not yet be any instances of this class that can be called.
Static attributes have only one copy in memory and are shared by all instances.
Use the self:: keyword to access static members of the current class.
Public properties of static properties
All instances of a class share static properties in the class.
In other words, even if there are multiple instances in the memory, there is only one copy of the static attributes.
In the following example, a counter $count attribute is set, with private and static modifications. In this way, the outside world cannot directly access the $count property. As a result of the program running, we also see that multiple instances are using the same static $count attribute.
Copy the code as follows
class user{
private static $count = 0; //Record the login status of all users.
public function __construct(){
self::$count = self::$count + 1;
}
public function getCount(){
return self::$count;
}
public function __destruct(){
self::$count = self::$count -1;
}
}
$user1 = new user();
$user2 = new user();
$user3 = new user();
echo "now here have ".$user1->getCount()." user";
echo "
";
unset( $user3) ;
echo "now here have ".$user1->getCount()." user";
?>
Program running result:
1
2
now here have 3 user
now here have 2 user bKjia.c0m
Static attribute calls directly
Static properties can be used directly without instantiation, and can be used directly before the class is created.
The method used is class name::static property name.
The code is as follows Copy the code
class Math{
public static $pi = 3.14;
}
//Find a garden with a radius of 3 area.
$r = 3;
echo "The area of radius $r is
";
echo Math::$pi * $r * $r ;
echo "
";
//I think 3.14 is not accurate enough here, so I set it to be more accurate.
Math::$pi = 3.141592653589793;
echo "The area of radius $r is
";
echo Math::$pi * $r * $r ;
?> ;
Program running results:
1
2
3
4
The area with radius 3 is
28.26
The area with radius 3 is
28.2743338823
The class is not created, and the static attributes can be used directly. When are static properties created in memory? I haven't seen any relevant information in PHP. Citing concepts in Java to explain should also be universal.
Static properties and methods, created when the class is called. When a class is called, it means that the class is created or any static member in the class is called.
Static method
Static methods can be used directly without the class being instantiated.
The method used is class name:: static method name.
Let’s continue writing this Math class to perform mathematical calculations. We design a method to calculate the maximum value. Since it is a mathematical operation, we do not need to instantiate this class. It would be much more convenient if this method can be taken and used.
We designed this class just to demonstrate the static method. www.111Cn.net provides the max() function in PHP to compare values.
Copy the code as follows
class Math{
: $num2;
}
}
$a = 99;
$b = 88;
echo "Show the maximum value of $ a and $ b is";
echo "
";
echo Math::Max($a,$b);
echo "
";echo "
";echo "
";
$a = 99;
$b = 100;
echo "Show the maximum value of $a and $b is";
echo "
";
echo Math ::Max($a,$b);
?>
Program running results:
The maximum value shown in $a and $b is
99
The maximum value shown in $a and $b is
100
Static How to call static method
The first example, when a static method calls other static methods, use the class name directly.
The code is as follows Copy the code
// Math class that implements maximum value comparison.
class Math{
. function Max3($num1,$num2,$num3){
$num1 = Math::Max($num1,$num2);
$num2 = Math::Max($num2,$num3);
$num1 = Math::Max($num1,$num2);
return $num1;
}
}
$a = 99;
$b = 77;
$c = 88;
echo "Show the maximum value in $a $b $c is";
echo "
";
echo Math::Max3($a,$b ,$c);
?>
Program running result:
1
2
Displays that the maximum value among 99 77 88 is
99
You can also use self:: to call other static methods in the current class. (Suggestion)
// Math class that implements maximum value comparison.
. function Max3($num1,$num2,$num3){
$num1 = self::Max($num1,$num2);
$num2 = self::Max($num2,$num3);
$num1 = self::Max($num1,$num2);
return $num1;
}
}
$a = 99;
$b = 77;
$c = 88;
echo "Show the maximum value in $a $b $c is";
echo "
";
echo Math::Max3($a,$b ,$c);
?>
Program running result:
1
2
Displays that the maximum value among 99 77 88 is
99
Static method calls static property www.111Cn.Net
Use class name::static property name to call the static properties in this class.
The code is as follows Copy the code
//
class Circle{
public static function circleAcreage($r){
}
}
$r = 3;
echo "The area of a circle with radius $r is" . Circle::circleAcreage ($r);
?>
Program execution result:
1
The area of a circle with radius 3 is 28.26
Use self:: to call the static properties of this class. (Suggestion)
The code is as follows Copy the code
//
class Circle{
public static function circleAcreage($r){
}
}
$r = 3;
echo "The area of a circle with radius $r is" . Circle::circleAcreage ($r);
?>
Program running result:
1
The area of a circle with radius 3 is 28.26
Static methods cannot call non-static properties
Static methods cannot call non-static properties. Non-static properties cannot be called using self::.
The code is as follows Copy the code
//
class Circle{
public static function circleAcreage($r){
}
}
$r = 3;
echo "The area of a circle with radius $r is" . Circle::circleAcreage($ r);
?>
Program running result:
1
Fatal error: Undefined class constant 'pi' in E:PHPProjectstest.php on line 7
You also cannot use $this to get the value of a non-static property.
//
class Circle{
public static function circleAcreage($r) {
return $r * $r * $this->pi;
. ::circleAcreage($r);
?>
Program running result:
1
Fatal error: Using $this when not in object context in E:PHPProjectstest.php on line 7
Static method calls non-static method
In PHP5, the $this identifier cannot be used in static methods to call non-static methods.
The code is as follows Copy the code
// Math class that implements maximum value comparison.
class Math{
public function Max($num1,$num2){
echo "bad
";
return $num1 > $num2 ? $num1 : $num2;
}
public static function Max3($num1,$num2,$num3){
$num1 = $this->Max($num1,$num2);
$num2 = $this-> ;Max($num2,$num3);
$num1 = $this->Max($num1,$num2);
return $num1;
}
}
$a = 99;
$b = 77;
$c = 188;
echo "Show the maximum value in $a $b $c is";
echo "
";
echo Math::Max3($a,$b,$c);
?>
Program execution result:
The maximum value displayed among 99 77 188 is
Fatal error: Using $this when not in object context in E:wwW.111cn.neT test.php on line 10
When a non-static method in a class is called by self::, the system will automatically convert this method into a static method.
The following code was executed and produced results. Because the Max method is converted into a static method by the system.
The code is as follows Copy the code
// Math class that implements maximum value comparison.
class Math{
public function Max($num1,$num2){ return $num1 > $num2 ? $num1 : $num2;
}
public static function Max3($ num1,$num2,$num3){
$num1 = self::Max($num1,$num2);
$num2 = self::Max($num2,$num3);
$num1 = self::Max($num1,$num2); 188;
echo "Show the maximum value in $a $b $c is";
echo "
";
echo Math::Max3($a,$b,$c) ;
?>
Program running result:
1
2
Displays that the maximum value among 99 77 188 is
188
In the following example, we let the static method Max3 use self:: to call the non-static method Max, and let the non-static method Max call the non-static property $pi through $this.
An error was reported when running. This error is the same as the previous example 3-1-9.php. This time, the non-static method Max reported an error of calling non-static properties by a static method.
This proves something. The non-static method Max we defined here is automatically converted into a static method by the system.
The code is as follows Copy the code
// Math class that implements maximum value comparison.
class Math{public $pi = 3.14;
public function Max($num1,$num2){
echo self::$pi; //The call here does not seem to work There should be a problem. Return $num1 > $num2 ? $num1 : $num2;
}
public static function Max3($num1,$num2,$num3){
$num1 = self ::Max($num1,$num2);
$num2 = self::Max($num2,$num3);
$num1 = self::Max($num1,$num2);
return $num1;
}
}
$a = 99;
$b = 77;
$c = 188;
echo "Show $a $b $c The maximum value is ";
echo "
";
echo Math::Max3($a,$b,$c);
?>
The program running result:
1
2
The maximum value displayed among 99 77 188 is
Fatal error: Access to undeclared static property: Math::$pi in E: PHPProjectstest.php on line 7
For more details, please check: http://www.bKjia.c0m/phper/php/56640.htm

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
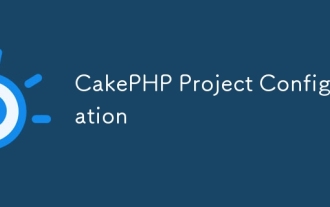
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
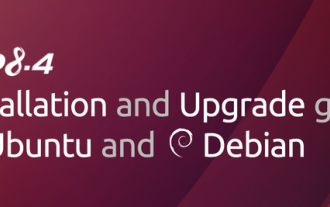
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
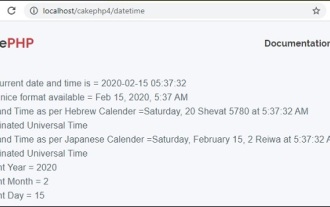
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
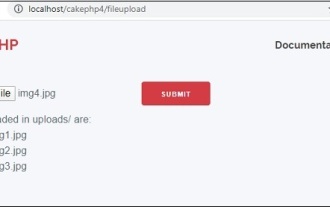
To work on file upload we are going to use the form helper. Here, is an example for file upload.
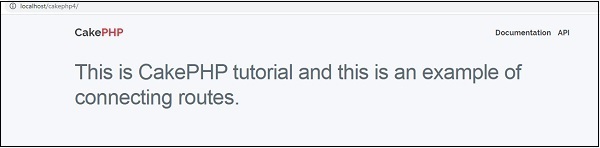
In this chapter, we are going to learn the following topics related to routing ?
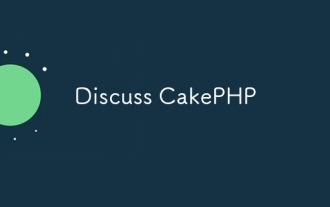
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
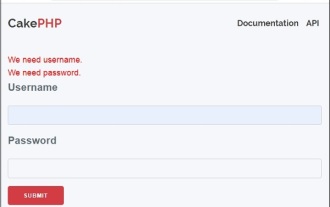
Validator can be created by adding the following two lines in the controller.
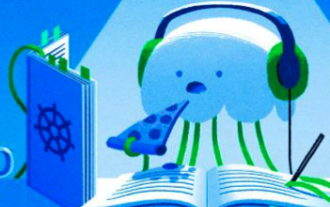
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
