


PHP program to generate thumbnails in equal proportions_PHP tutorial
PHP program to generate thumbnails in equal proportions This program is easy to implement, but it is only used to generate thumbnails in equal proportions. You need to upload the file to the server and then operate it with this function. Friends in need can refer to it.
PHP tutorial program to generate thumbnails in equal proportions
This program is easy to implement, but it is only used to generate thumbnails in equal proportions. You need to upload the file to the server and then operate it with this function. Friends in need can refer to it.
function reSizeImg($imgSrc, $resize_width, $resize_height, $isCut=false) {
//Type of picture
$type = substr ( strrchr ( $imgSrc, "." ), 1 );
//Initialize image
if ($type == "jpg") {
$im = imagecreatefromjpeg ( $imgSrc );
}
if ($type == "gif") {
$im = imagecreatefromgif ($imgSrc);
}
if ($type == "png") {
$im = imagecreatefrompng ( $imgSrc );
}
//Target image address
$full_length = strlen ( $imgSrc );
$type_length = strlen ( $type );
$name_length = $full_length - $type_length;
$name = substr ( $imgSrc, 0, $name_length - 1 );
$dstimg = $name . "_s." . $type;$width = imagesx ( $im );
$height = imagesy ($im);//Generate image
//The proportion of the changed image
$resize_ratio = ($resize_width) / ($resize_height);
//Proportion of actual image
$ratio = ($width) / ($height);
if (($isCut) == 1) //Cut image
{
if ($ratio >= $resize_ratio) //High priority
{
$newimg = imagecreatetruecolor ( $resize_width, $resize_height );
Imagecopyresampled ( $newimg, $im, 0, 0, 0, 0, $resize_width, $resize_height, (($height) * $resize_ratio), $height );
ImageJpeg ( $newimg, $dstimg );
}
if ($ratio < $resize_ratio) //width first
{
$newimg = imagecreatetruecolor ( $resize_width, $resize_height );
Imagecopyresampled ( $newimg, $im, 0, 0, 0, 0, $resize_width, $resize_height, $width, (($width) / $resize_ratio) );
ImageJpeg ( $newimg, $dstimg );
}
} else //Do not crop image
{
if ($ratio >= $resize_ratio) {
$newimg = imagecreatetruecolor ( $resize_width, ($resize_width) / $ratio );
Imagecopyresampled ( $newimg, $im, 0, 0, 0, 0, $resize_width, ($resize_width) / $ratio, $width, $height );
ImageJpeg ( $newimg, $dstimg );
}
if ($ratio < $resize_ratio) {
$newimg = imagecreatetruecolor ( ($resize_height) * $ratio, $resize_height );
Imagecopyresampled ( $newimg, $im, 0, 0, 0, 0, ($resize_height) * $ratio, $resize_height, $width, $height );
ImageJpeg ( $newimg, $dstimg );
}
}
ImageDestroy ( $im );
}
The calling method is simple, just reSizeImg directly, the reference is very simple.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


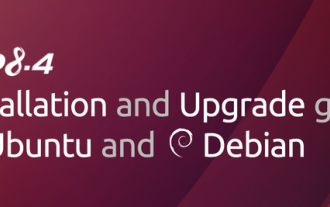
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
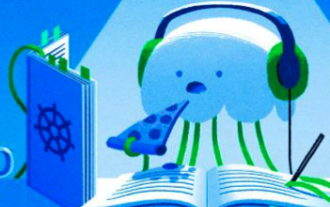
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
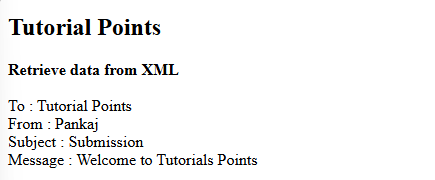
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
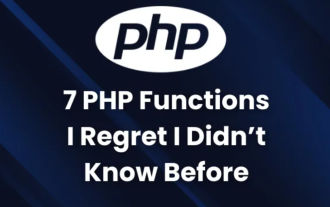
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
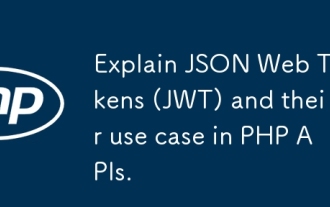
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
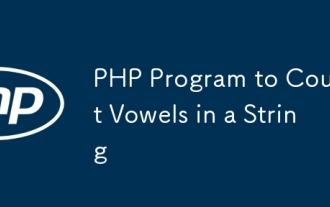
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
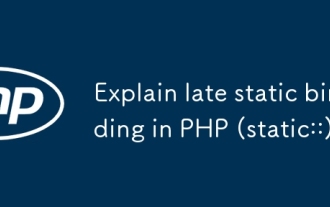
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
