


Two methods of merging two-dimensional arrays in PHP_PHP tutorial
Yesterday I also wrote a technical article about array merging. There I introduced the merging of one-dimensional arrays. Here I will introduce the merging method of two-dimensional arrays in PHP. I hope it will be helpful to all students.
Example 1
Custom method to merge arrays
Look at a two-dimensional array first:
The code is as follows | Copy code | ||||
|
The merging method is as follows:
The code is as follows | Copy code | ||||
$store_count=count($showinfo); //Count the number of times displayed. $showinfo here is a variable when I get the database content, and then print it out as it did at the beginning for($i=0;$i<$store_count;$i++) { $stores[]=array( ‘name’ =>$showinfo[$i]['value'], ‘owner’=>$showinfo[$j]['value'], ); $i = $j; // The function here is equivalent to $i getting the value whose key value is an even number } ?> |
In this way you can get the above results!
In order to let everyone see it more clearly, I wrote out the database table structure. The main fields are as follows:
key value
store_name online store one
store_owner Xiaofeng
store_name online store 2
store_owner Xiao Lei
After using the above method, the front page can be displayed in a row, as follows
name owner
Online Shop 1 Xiaofeng
Online Shop 2 Xiao Lei
Example 3
The code is as follows
|
Copy code | ||||
(
0 => array(
'1@01,02',
'2@01,02',
'4@ALL',
'3@01',
'5@01,02,04',
),
1 => array(
'1@01,02,03',
'2@01,02,04',
'3@ALL',
'4@01,02',
'111@01,05',
'5@03',
),
2 => array(
'1@01,02,03',
'2@02,03,05',
'3@ALL',
'4@01,02,03',
'111@01,05',
'5@03',
),
);
$result = array();
foreach($arr as $items){
If(is_array($items)){
foreach($items as $item){
$item = explode('@', $item);
If(count($item) != 2){
continue ;
}
$result[$item[0]] .= $item[1].',';
}
}
}
function reJoin(&$item,$key,$seq){
$list = array_unique(explode($seq,$item));
If (in_array('ALL', $list)){
$item = $key.'@ALL';
}else{
$item = $key.'@'.join($seq,$list);
}
}
array_walk($result, 'reJoin',',');
sort($result);
var_export($result);
/**
* array (
* 0 => '111@01,05,',
* 1 => '1@01,02,03,',
* 2 => '2@01,02,04,03,05,',
* 3 => '3@ALL',
* 4 => '4@ALL',
* 5 => '5@01,02,04,03,',
* )
*/
?>

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
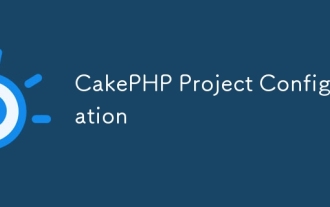
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
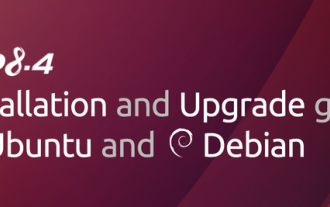
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
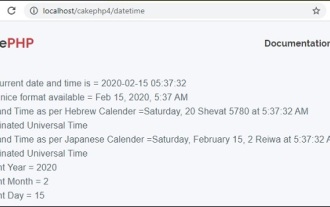
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
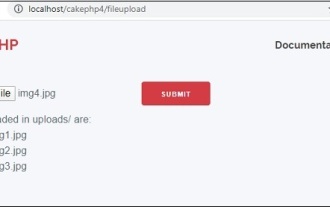
To work on file upload we are going to use the form helper. Here, is an example for file upload.
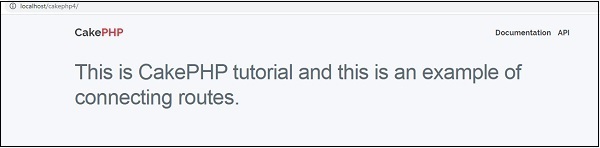
In this chapter, we are going to learn the following topics related to routing ?
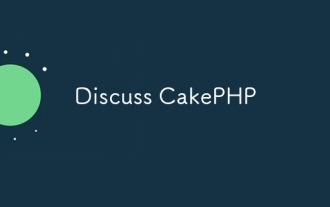
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
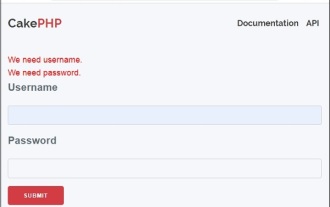
Validator can be created by adding the following two lines in the controller.
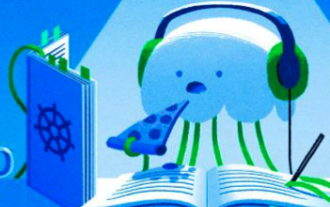
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
