


PHP exception error handling mechanism and error handling (1/2)_PHP tutorial
The most common error mechanism we use in PHP is try catch{}, which can easily catch errors. However, PHP also provides error viewing and error closing for many things. This can be found in php.ini During processing, you can also add error_display(0); at the beginning of the file to not display errors
The code is as follows | Copy code | ||||||||||||||||||||
$f=fopen('test.txt','r'); //Close after use
|
The code is as follows | Copy code |
if(!file_exists('aa.txt')){ die('File does not exist'); } else { //Perform operation } //If die() above is triggered, then the echo connection here will not be executed echo 'ok'; |
The code is as follows | Copy code |
file_exits('aaa.txt') or die('File does not exist'); echo 'ok'; |
B. Custom errors and error triggers
1. Error handler (custom error, generally used for syntax error handling)
Create a custom error function (handler) that must be able to handle at least two parameters (error_level and errormessage), but can accept up to five parameters (error_file, error_line, error_context)
Syntax:
function error_function($error_level,$error_message,$error_file,$error_line,$error_context)
//After creation, you need to rewrite the set_error_handler(); function
set_error_handler('error_function',E_WARNING); //Here error_function corresponds to the custom handler name created above, and the second parameter is the error level using the custom error handler;
Error reporting level (just understand it)
These error reporting levels are different types of errors that error handlers are designed to handle:
Value Constant Description
2 E_WARNING Nonfatal run-time error. Do not pause script execution.
8 E_NOTICE Run-time notification.
Script discovery errors may occur, but may also occur while the script is running normally.
256 E_USER_ERROR Fatal user-generated error. This is similar to E_ERROR set by the programmer using the PHP function trigger_error().
512 E_USER_WARNING Non-fatal user-generated warning. This is similar to the E_WARNING set by the programmer using the PHP function trigger_error().
1024 E_USER_NOTICE User-generated notification. This is similar to E_NOTICE set by the programmer using the PHP function trigger_error().
4096 E_RECOVERABLE_ERROR Trapable fatal error. Like E_ERROR, but can be caught by a user-defined handler. (see set_error_handler())
8191 E_ALL All errors and warnings except level E_STRICT.
(In PHP 6.0, E_STRICT is part of E_ALL)
2. Error trigger (generally used to handle logical errors)
Requirement: For example, if you want to receive an age, if the number is greater than 120, it is considered an error
Traditional method:
if($age>120){
echo 'Wrong age';exit();
}
Use triggers:
if($age>120){
//trigger_error('error message'[,'error level']); The error level here is optional and is used to define the level of the error
//User-defined levels include the following three types: E_USER_WARNING, E_USER_ERROR, E_USER_NOTICE
trigger_error('age error');//This is the default error handling method of the calling system, we can also use a custom processor
}
//Custom processor, same as above
function myerror($error_level,$error_message){
echo 'error text';
}
//At the same time, the system default processing function needs to be changed
set_error_handler('myerror',E_USER_WARNING);//Same as above, the first parameter is the name of the custom function, and the second parameter is the error level [The error levels here are usually the following three: E_USER_WARNING, E_USER_ERROR, E_USER_NOTICE]
//Now you can use the custom error handling function by using trigger_error
Practice questions:
The code is as follows | Copy code | ||||
$info= "Error number: $error_leveln"; } else { echo 'File'.$filename.'Not writable'; }
exit();
} <🎜>
set_error_handler('myerror',E_WARNING); <🎜>
$fp=fopen('aaa.txt','r'); <🎜>
?>
|

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


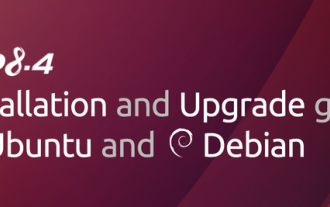
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
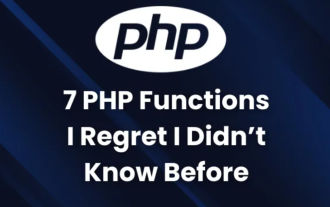
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
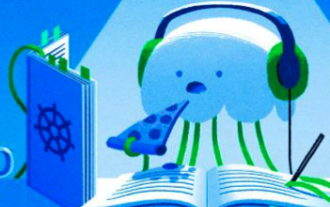
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
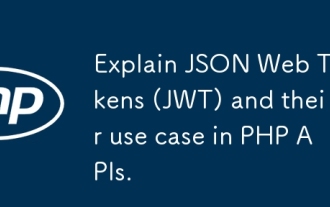
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
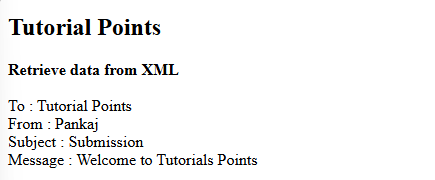
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
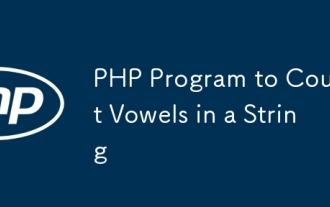
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
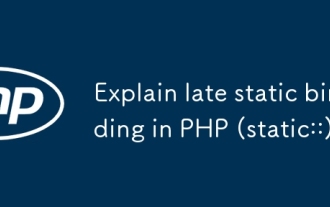
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
