


Advanced traversal and operation processing methods of PHP array_PHP tutorial
I have talked about simple array traversal before, which is based on statements such as foreach and for. Now I will introduce the advanced traversal method of array. Friends can refer to it. These arrays are really useful for development, with strong practical performance and complex It's also higher.
PHP’s handling of arrays can be called one of the most attractive features of the language. It supports more than 70 array-related functions. Whether you want to flip an array, determine whether a value exists in an array, convert an array to a string, or calculate the size of an array, you can do it simply by executing an existing function. However, there are also some array-related tasks that have higher requirements for developers. Just knowing a certain function in the manual cannot solve it. These tasks require some in-depth understanding of the original features of PHP and some imagination to solve the problem. force.
Multidimensional associative array sorting
PHP provides some array sorting functions, such as sort(), ksort(), and asort(), but it does not provide sorting of multi-dimensional associative arrays.
For example, an array like this:
Array
(
[0] => Array
(
[name] => chess
[price] => 12.99
)
[1] => Array
(
[name] => checkers
[price] => 9.99
)
[2] => Array
(
[name] => backgammon
[price] => 29.99
)
)
To sort the array in ascending order, you need to write a function yourself to compare prices, and then pass the function as a callback function to the usort() function to implement this function:
The code is as follows | Copy code | ||||
|
After executing this program fragment, the array will be sorted, and the result is as follows:
Array
(
[0] => Array
(
[name] => checkers
[price] => 9.99
)
[1] => Array
(
[name] => chess
[price] => 12.99
)
[2] => Array
(
[name] => backgammon
[price] => 29.99
)
)
To sort the array in descending order, just swap the positions of the two subtracted numbers in the comparePrice() function.
Traverse the array in reverse order
PHP’s While Loop and For Loop are the most commonly used methods to traverse an array. But how do you iterate over an array like the one below?
Array
(
[0] => Array
(
[name] => Board
[games] => Array
(
[0] =>
(
[name] => chess
[price] =>
)
[1] => Array
[Name] = & gt; checker
[price] => 9.99
)
)
)
)
There is an iterator class for collections in the PHP standard library. It can not only be used to traverse some heterogeneous data structures (such as file systems and database query result sets), but can also be used to iterate some embedded objects of unknown size. Traversal of arrays. For example, to traverse the above array, you can use the RecursiveArrayIterator iterator:
The code is as follows | Copy code | ||||
$iterator = new RecursiveArrayIterator($games); iterator_apply($iterator, 'navigateArray', array($iterator));
|
Executing this code will give the following results:
name: Board
name: chess
price: 12.99
name: checkers
price: 9.99
Filter the results of associative arrays
Suppose you are given the following array, but you only want to operate on elements whose price is less than $11.99:
Array
(
[0] => Array
(
[name] => checkers
[price] => 9.99
)
[1] => Array
(
[name] => chess
[price] => 12.99
)
[2] => Array
(
[name] => backgammon
[price] => 29.99
)
)
It can be easily implemented using the array_reduce() function:
The code is as follows | Copy code | ||||
return ($game['price'] < 11.99); }
|
The array_reduce() function will filter out all elements that do not satisfy the callback function. The callback function in this example is filterGames. Any element with a price lower than 11.99 will be kept, and the others will be eliminated. The execution result of this code segment:
Array
(
[1] => Array (
[name] => checkers
[price] => 9.99
)
)
代码如下 | 复制代码 |
class Game { $game = new Game(); print_r(array($game)); 执行该例子就会产生如下结果: Array |
Convert object to array
代码如下 | 复制代码 |
class Game { public function setPrice($price) { $game = new Game(); print_r(array($game));执行该代码片段: Array |
The code is as follows | Copy code |
class Game { public $name; public $price; } $game = new Game(); $game->name = 'chess'; $game->price = 12.99; print_r(array($game)); Executing this example will produce the following results: Array ( [0] => Game Object ( [name] => chess [price] => 12.99 ) ) |
The code is as follows | Copy code |
class Game { public $name; private $_price; public function setPrice($price) { $this->_price = $price; } } $game = new Game(); $game->name = 'chess'; $game->setPrice(12.99); print_r(array($game));Execute this code snippet: Array ( [0] => Game Object ( [name] => chess [_price:Game:private] => 12.99 ) ) |
As you can see, in order to distinguish, the keys of the private variables saved in the array are automatically changed.
“Natural ordering” of arrays
PHP’s sorting results for “alphanumeric” strings are undefined. For example, suppose you have many image names stored in an array:
The code is as follows | Copy code | ||||||||||||
0=>'madden2011.png', 1=>'madden2011-1.png',
3=>'madden2012.png'
);
|
The code is as follows | Copy code |
$arr = array(<🎜>
0=>'madden2011.png',
1=>'madden2011-1.png',
2=>'madden2011-2.png',
3=>'madden2012.png'
);
natsort($arr);
echo ""; print_r($arr); echo ""; ?> Run result: Array ( [1] => madden2011-1.png [2] => madden2011-2.png [0] => madden2011.png [3] => madden2012.png ) |
The code is as follows | Copy code |
$array = array("A"=>1, "B"=>1, "C"=>1, "D"=>1); foreach($array as &$value) $value = 2; print_r($array); ?> |
The output of the above code is as follows:
Array ( [A] => 2 [B] => 2 [C] => 2 [D] => 2 )
As you can see, the values corresponding to each key in $array have been modified to 2. It seems this approach actually works.
Use key values to operate the elements of the array
Sometimes, the array may represent some interrelated elements. If you encounter one of these interrelated elements and mark other elements, the above reference will definitely not work. At this time, when modifying these associated elements, you must use their corresponding key values. Try it first and see if it works:
The code is as follows | Copy code | ||||||||||||
If($key == "B"){
echo '
If($value === "CHANGE") echo $value.'';
|
The code is as follows | Copy code |
$array = array("A"=>1, "B"=>1, "C"=>1, "D"=>1);
foreach($array as $key => $value){
If($key == "B"){
$array["A"] = "CHANGE";
$array["D"] = "CHANGE";
print_r($array);
echo ' '; } If($array[$key] === "CHANGE") echo $value.' '; } print_r($array); ?> |
The code is as follows | Copy code |
Array ( [A] => CHANGE [ B] => 1 [C] => 1 [D] => CHANGE ) 1 Array ( [A] => CHANGE [B] => 1 [C] => 1 [D] => CHANGE ) |
Then it is indeed 1.
What is the reason for this? Turning to the foreach page of the PHP document, I suddenly realized:
Note: Unless the array is referenced, foreach operates on a copy of the specified array, not the array itself. foreach has some side effects on array pointers. Do not rely on the value of an array pointer during or after a foreach loop unless it is reset.
It turns out that foreach operates on a copy of the specified array. No wonder, getting $value doesn’t work! After understanding this, the above problem is solved. As long as in foreach, you can directly take the elements in $array according to the key and perform various judgment and assignment operations.
Summary and extension
PHP's array traversal and operation capabilities are indeed very powerful, but the solutions to some slightly more complex problems are not so obvious. In fact, this is the case in any field. A language and grammar provide basic operations. Solutions to complex problems require developers’ own thinking, imagination and code writing.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


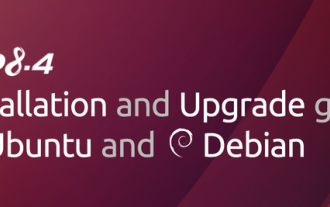
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
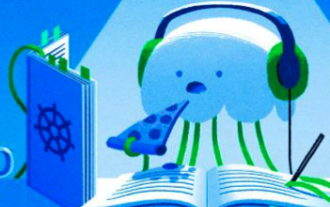
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
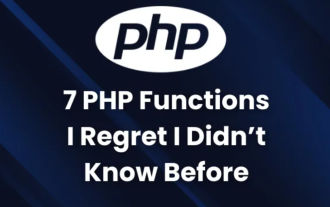
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
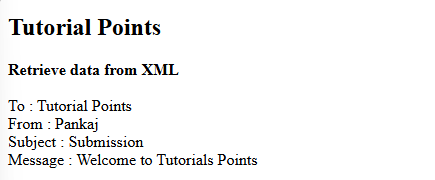
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
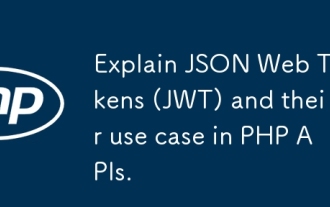
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
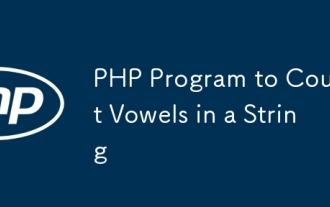
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
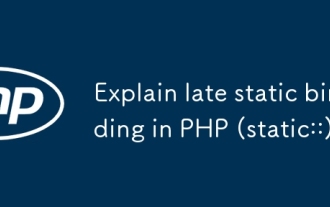
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
