


Basic Introduction to PHP - File and Directory Operations_PHP Tutorial
An article about the various operating functions and example applications of files and directories for friends who are getting started with PHP. Friends in need can simply refer to it.
This chapter can be regarded as a continuation of the previous chapter, introducing additional information besides the actual content of the file, including the file size, directory, access permissions, etc. Some functions in the file system are only valid when the server is a specific system, such as the function symlink() that changes symbolic links, the function chmod() that sets file access permissions, and the function umask() that sets directory access permissions. Etc. These are only valid in Linux systems, not Windows systems. The DirectoryIterator class provided by PHP5 and later also encapsulates many practical directory operations
The code is as follows | Copy code | ||||
foreach(new directoryIterator('/usr/local/images') as $file){ print $file->getPathname()."n"; } //------------- Implementation method of previous versions of PHP5 ------------- $d = opendir('/usr/local/images') or die($php_errormsg); while(false !==($f = readdir($d))){ print $f."n"; } |
closedir($d);File information function
Function name What file information does the function provide?
file_exists() Whether the file exists
fileatime() last access time
filectime() The last modification time of file inode
filegroup() gets the file group (returns an integer)
fileinode() gets the number of information nodes of the file (returns an integer)
filemtime() gets the time when the file data block was last written (returns Unix timestamp)
fileowner() gets the owner of the file (returns user ID)
fileperms() obtains file permissions
filesize() gets the file size in bytes
filetype() gets the file type, may return fifo, char, dir, block, link, file and unknown
is_dir() determines whether the given file name is a directory
is_executable() determines whether the given file name is executable (available for Windows since PHP5.0.0)
is_file() determines whether the given file name is a normal file
is_link() determines whether the given file name is a symbolic link
is_readable() determines whether the given file name is readable
is_writable() determines whether the given file name is writable
Directory related functions
Function name What file information does the function provide?
mkdir() creates a new directory, the second parameter can be used to set access permissions
rmdir() delete directory
rename() renames a file or directory
Directory class related methods
The DirectoryIterator class encapsulates many directory-related methods
Method name What directory information does the function provide?
isDir() determines whether the given DirectoryIterator item object is a directory
isDot() determines whether the current DirectoryIterator item object is ‘.’ or ‘..’
isFile() determines whether the current DirectoryIterator item object is a valid file
isLink() determines whether the current DirectoryIterator item object is a connection
isReadable() determines whether the current DirectoryIterator item object is readable
isWritable() determines whether the current DirectoryIterator item object is writable
isExecutable() determines whether the current DirectoryIterator item object is executable
getATime() gets the last access time of the current Iterator item
getCTime() Gets the last modification time of the current Iterator item
getMTime() Gets the time when the current Iterator item file data block was last written
getFilename() Gets the current Iterator item file name (with extension)
getPathname() gets the current Iterator item path name
getPath() gets the current Iterator item path name and file name
getGroup() gets the current Iterator item group ID
getOwner() gets the current Iterator item owner ID
getPerms() Gets the current Iterator item permissions
getSize() gets the current Iterator item file size
getType() gets the current Iterator item type, which may be file, link or dir
getInode() gets the inode node number of the current Iterator item
File timestamps solved
The touch() function modifies the update time of the file
The fileatime() function returns the last time the file was opened for reading or writing
The filemtime() function returns the last time the file content was modified
The filectime() function returns the last time the file content or metadata was modified
Get file information
An array containing file-related information can be obtained through stat(). Similar to this function is the fstat() function. This function takes a file handle as a parameter (returned by fopen() or popen()). lstat() is used to Get information about a symbol or file connection.
Numeric index String index Description
0 dev device number
1 ino information node number
2 mode protection mode
3 nlink Number of connected connections
4 uid owner user ID
5 gid group ID
6 rdev device type, if it is an inode device
7 size file size in bytes
8 atime time of last access (Unix timestamp)
9 mtime Last modified time (Unix timestamp)
10 ctime Last changed time (Unix timestamp)
11 blksize file system IO block size
12 blocks The number of blocks occupied
Modify file permissions
The chmod() function modifies file permissions
The chown() function modifies the owner of the file
The chgrp() function modifies the group to which the file belongs
Note: The above 3 functions are invalid in Windows system
Get information about each part of the file name
The basename() function can obtain the file name, the dirname() function can obtain the path name, and pathinfo() obtains the associative array of the directory name, complete file name, extension, and file name (that is, without extension). The key names are [ dirname], [basename], [extension], [filename]
The current directory path (physical path, often used to reference other PHP files) is often obtained through the combination of dirname(__FILE__)
Delete files
Use the unlink() function to delete a file. If the deletion fails, an E_WARNING error will be generated
Tip: After PHP5.0.0, this function can also be used to delete remote files, such as FTP, etc.
Copy or move files
Use the copy(old_dir, new_dir) function to copy files, and use rename(old_dir, new_dir) to move files. The new_dir here can rename the file name.
Pattern matching file name list (fuzzy query)
If you want to query all jpg files (*.jpg) in a directory like the command line, you can use the FileterIterator subclass accept() method or glob() function of the DirectoryIterator class to obtain matching file names.
//Implementation code of FileterIterator
The code is as follows
|
Copy code
|
||||||||
Public function accept() {
|
foreach (new ImageFilter(new DirectoryIterator('/usr/local/images')) as $img) {
}

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




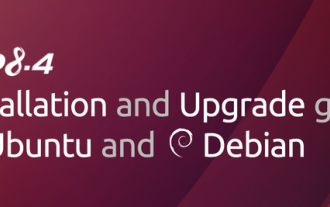
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
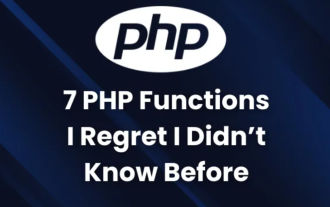
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
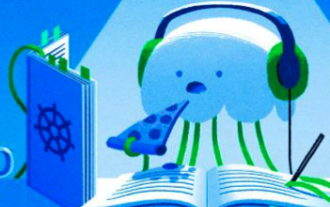
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
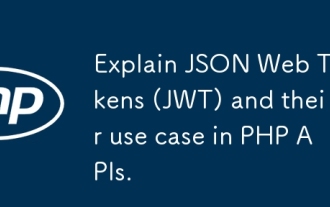
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
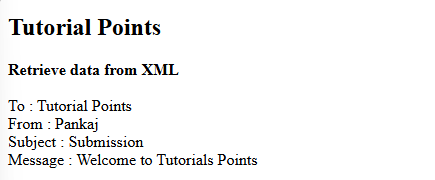
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
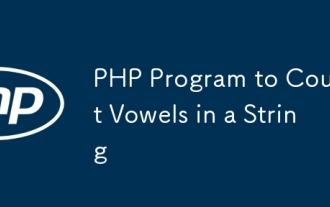
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
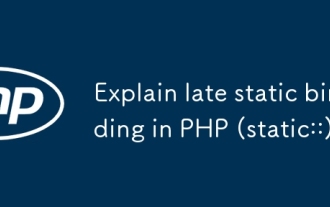
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
