


Detailed explanation of JS object-oriented programming_javascript skills
Because JavaScript is based on prototypes and does not have the concept of classes (ES6 has it, let’s not talk about it for now), all we have access to are objects, and everything is truly an object
So when we talk about objects, it is a bit vague. Many students will confuse the concepts of typed objects and objects themselves. We will not mention objects in the following terminology. We use a method similar to Java to facilitate understanding
Method 1
Class (function simulation)
function Person(name,id){ //实例变量可以被继承 this.name = name; //私有变量无法被继承 var id = id; //私有函数无法被继承 function speak(){ alert("person1"); } } //静态变量,无法被继承 Person.age = 18; //公有函数可以被继承 Person.prototype.say = function(){ alert("person2"); }
Inherit and call the parent class method
function Person(name,id){ //实例变量可以被继承 this.name = name; //私有变量无法被继承 var id = id; //私有函数无法被继承 function speak(){ alert("person1"); } } //静态变量,无法被继承 Person.age = 18; //公有静态成员可被继承 Person.prototype.sex = "男"; //公有静态函数可以被继承 Person.prototype.say = function(){ alert("person2"); } //创建子类 function Student(){ } //继承person Student.prototype = new Person("iwen",1); //修改因继承导致的constructor变化 Student.prototype.constructor = Student; var s = new Student(); alert(s.name);//iwen alert(s instanceof Person);//true s.say();//person2
Inherit, override the parent class method and implement super()
function Person(name,id){ //实例变量可以被继承 this.name = name; //私有变量无法被继承 var id = id; //私有函数无法被继承 function speak(){ alert("person1"); } } //静态变量,无法被继承 Person.age = 18; //公有静态成员可被继承 Person.prototype.sex = "男"; //公有静态函数可以被继承 Person.prototype.say = function(){ alert("person2"); } //创建子类 function Student(){ } //继承person Student.prototype = new Person("iwen",1); //修改因继承导致的constructor变化 Student.prototype.constructor = Student; //保存父类的引用 var superPerson = Student.prototype.say; //复写父类的方法 Student.prototype.say = function(){ //调用父类的方法 superPerson.call(this); alert("Student"); } //创建实例测试 var s = new Student(); alert(s instanceof Person);//true s.say();//person2 student
Inherited wrapper function
function extend(Child, Parent) { var F = function(){}; F.prototype = Parent.prototype; Child.prototype = new F(); Child.prototype.constructor = Child; Child.uber = Parent.prototype; }
uber means setting an uber attribute for the child object, which directly points to the prototype attribute of the parent object. (Uber is a German word meaning "up" or "one level up.") This is equivalent to opening a channel on the child object, and you can directly call the parent object's method. This line is placed here just to achieve the completeness of inheritance and is purely for backup purposes.
Method 2
Equivalent to copying, the object you want to inherit is carried through the defined _this object. In this case, inheritance can be achieved by passing _this, which is easier to understand than the above
//创建父类 function Person(name,id){ //创建一个对象来承载父类所有公有东西 //也就是说_this承载的对象才会被传递给子类 var _this = {}; _this.name = name; //这样的是不会传递下去的 this.id = id; //承载方法 _this.say = function(){ alert("Person"); } //返回_this对象 return _this; } //子类 function Student(){ //获取person的_this对象,从而模仿继承 var _this = Person("iwne",1); //保存父类的_this引用 var superPerson = _this.say; //复写父类的方法 _this.say = function(){ //执行父类的say superPerson.call(_this); alert("Student"); } return _this; } var s = new Student(); s.say();
I hope it will be helpful for everyone to learn javascript programming.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


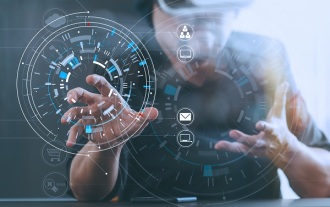
Face detection and recognition technology is already a relatively mature and widely used technology. Currently, the most widely used Internet application language is JS. Implementing face detection and recognition on the Web front-end has advantages and disadvantages compared to back-end face recognition. Advantages include reducing network interaction and real-time recognition, which greatly shortens user waiting time and improves user experience; disadvantages include: being limited by model size, the accuracy is also limited. How to use js to implement face detection on the web? In order to implement face recognition on the Web, you need to be familiar with related programming languages and technologies, such as JavaScript, HTML, CSS, WebRTC, etc. At the same time, you also need to master relevant computer vision and artificial intelligence technologies. It is worth noting that due to the design of the Web side
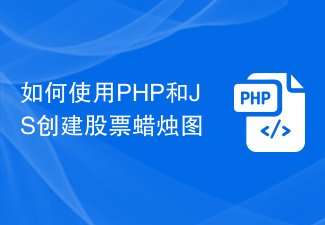
How to use PHP and JS to create a stock candle chart. A stock candle chart is a common technical analysis graphic in the stock market. It helps investors understand stocks more intuitively by drawing data such as the opening price, closing price, highest price and lowest price of the stock. price fluctuations. This article will teach you how to create stock candle charts using PHP and JS, with specific code examples. 1. Preparation Before starting, we need to prepare the following environment: 1. A server running PHP 2. A browser that supports HTML5 and Canvas 3
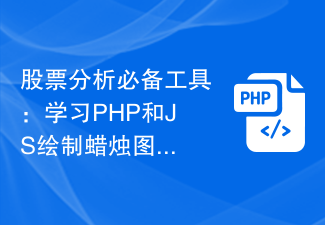
Essential tools for stock analysis: Learn the steps to draw candle charts in PHP and JS. Specific code examples are required. With the rapid development of the Internet and technology, stock trading has become one of the important ways for many investors. Stock analysis is an important part of investor decision-making, and candle charts are widely used in technical analysis. Learning how to draw candle charts using PHP and JS will provide investors with more intuitive information to help them make better decisions. A candlestick chart is a technical chart that displays stock prices in the form of candlesticks. It shows the stock price
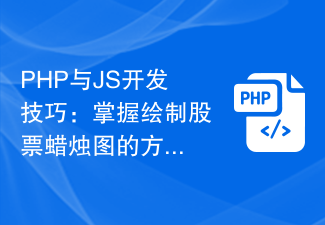
With the rapid development of Internet finance, stock investment has become the choice of more and more people. In stock trading, candle charts are a commonly used technical analysis method. It can show the changing trend of stock prices and help investors make more accurate decisions. This article will introduce the development skills of PHP and JS, lead readers to understand how to draw stock candle charts, and provide specific code examples. 1. Understanding Stock Candle Charts Before introducing how to draw stock candle charts, we first need to understand what a candle chart is. Candlestick charts were developed by the Japanese
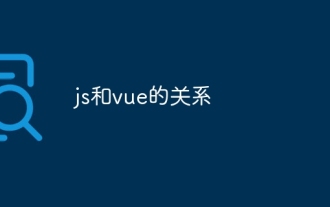
The relationship between js and vue: 1. JS as the cornerstone of Web development; 2. The rise of Vue.js as a front-end framework; 3. The complementary relationship between JS and Vue; 4. The practical application of JS and Vue.
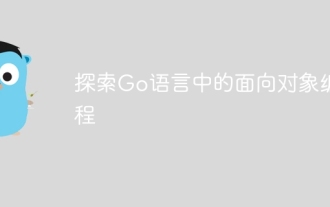
Go language supports object-oriented programming through type definition and method association. It does not support traditional inheritance, but is implemented through composition. Interfaces provide consistency between types and allow abstract methods to be defined. Practical cases show how to use OOP to manage customer information, including creating, obtaining, updating and deleting customer operations.
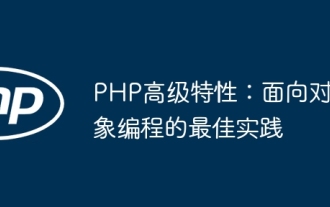
OOP best practices in PHP include naming conventions, interfaces and abstract classes, inheritance and polymorphism, and dependency injection. Practical cases include: using warehouse mode to manage data and using strategy mode to implement sorting.

The Go language supports object-oriented programming, defining objects through structs, defining methods using pointer receivers, and implementing polymorphism through interfaces. The object-oriented features provide code reuse, maintainability and encapsulation in the Go language, but there are also limitations such as the lack of traditional concepts of classes and inheritance and method signature casts.
