How to lock files under PHP_PHP Tutorial
/*
*lock_thisfile: Obtain exclusive lock
*@param $tmpFileStr The file name used as the shared lock file (you can give it any name)
*@param $locktype lock type, the default is false (non-blocking, that is, once the lock fails, it will directly return false). If set to true, it will wait for the lock to be successful before returning
*@return If the lock is successful, the lock instance is returned (this parameter is required when using the unlock_thisfile method), if the lock fails, false is returned.
*/
function lock_thisfile($tmpFileStr,$locktype=false){
if($locktype == false)
$locktype = LOCK_EX|LOCK_NB;
$can_write = 0;
$lockfp = @fopen($tmpFileStr.".lock","w");
if($lockfp){
$can_write = @flock($lockfp,$locktype);
}
if($can_write){
return $lockfp;
}
else{
if($lockfp){
@fclose($lockfp);
@unlink($tmpFileStr.".lock");
}
return false;
}
}
/**
*unlock_thisfile: Unlock the previously obtained lock instance
*@param $fp The return value of the lock_thisfile method
*@param $tmpFileStr The file name used as the shared lock file (you can give it any name)
*/
function unlock_thisfile($fp,$tmpFileStr){
@flock($fp,LOCK_UN);
@fclose($fp);
@fclose($fp);
@unlink($tmpFileStr.".lock");
}
?>
// Usage example
$tmpFileStr = "/tmp/mylock.loc";
// Wait for the operation permission to be obtained. If you want to return immediately, set the second parameter to false.
$lockhandle = lock_thisfile($tmpFileStr,true);
if($lockhandle){
// All transaction processing that needs to be exclusive is done here.
// ... ...
// Transaction completed.
unlock_thisfile($lockhandle,$tmpFileStr);
}
?>

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
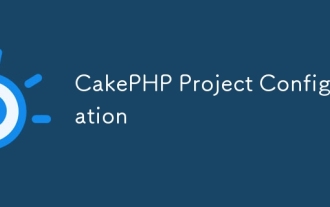
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
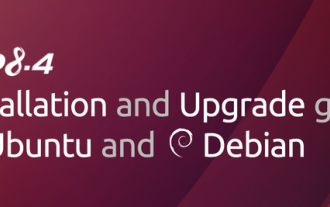
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
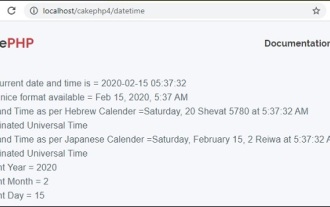
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
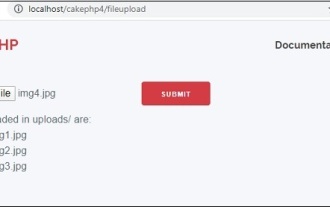
To work on file upload we are going to use the form helper. Here, is an example for file upload.
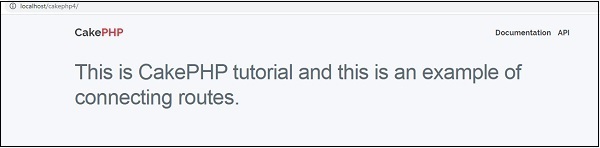
In this chapter, we are going to learn the following topics related to routing ?
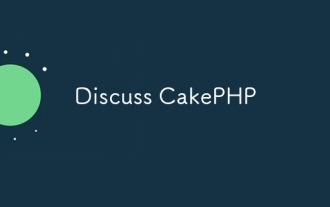
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
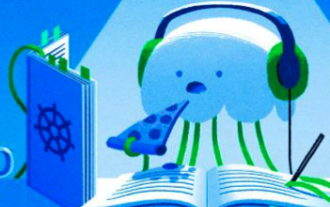
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
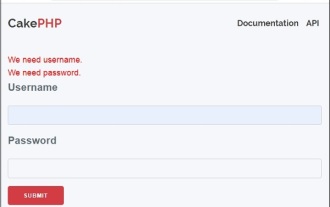
Validator can be created by adding the following two lines in the controller.
