


Introduction to the usage of php custom functions_PHP tutorial
This article introduces the usage of PHP custom functions in detail, including 1. The function name cannot have the same name as an existing function name. 2. Function names can only contain letters, numbers and underscores. 3. The function name cannot start with a number
Custom function
7.2.1 Basic principles of function naming:
1. The function name cannot have the same name as an existing function name.
2. Function names can only contain letters, numbers and underscores.
3. The function name cannot start with a number
7.2.2 Basic usage: declare with function
The code is as follows | Copy code | ||||||||||||||||||||||||||||||||||||
function funcCountArea($radius) {
}
'; $area2 = funcCountArea(30); echo $area2; ?> output 1256.63706144 2827.43338823 7.2.3 Passing parameters by value
7.2.6 Another function call that returns multiple values (practical: recommended)
$userInfo [] = $username; $arr[] = funcUserInfo ( 'Mark', '925472' ); print_r ( $arr ); |

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
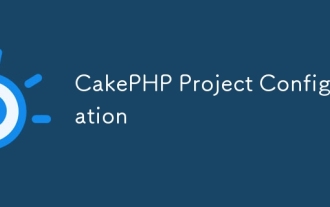
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
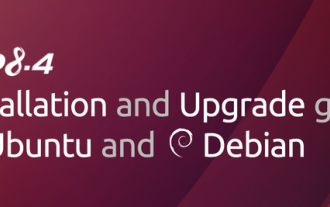
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
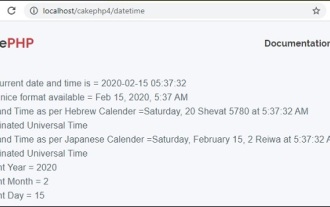
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
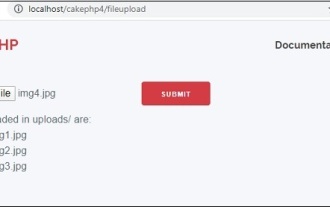
To work on file upload we are going to use the form helper. Here, is an example for file upload.
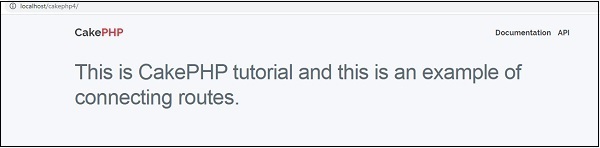
In this chapter, we are going to learn the following topics related to routing ?
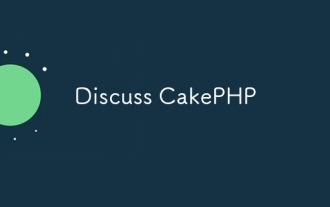
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
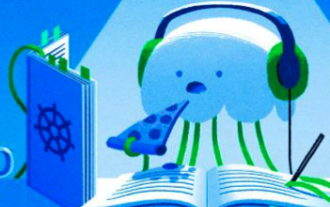
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
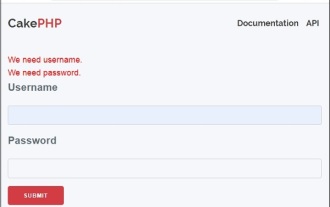
Validator can be created by adding the following two lines in the controller.
