Basics of Getting Started with PHP - Grammar_PHP Tutorial
This is a simple introduction to PHP syntax. Friends in need can refer to it.
1. Double quotation marks and single quotation marks
Variables can be executed in double quotes, but constants using define are not executable in both single and double quotes:
The code is as follows | Copy code | ||||||||
echo 'im $var'; // => 'im $var' echo "im $var"; // => 'im sofish'
|
No variables can be rendered within quotes in JS.
2. Definition of variables
Use the $ sign instead of the var keyword; have real constants, use the define function, and once defined, can be changed.
The code is as follows | Copy code | ||||||||
// In JS we use var name = 'sofish';
// In JS we use var NAME = sofish; and it can be changed define('NAME', 'sofish');
define('NAME', 'error'); |
Sort by:
代码如下 | 复制代码 |
echo 'im sofish,' . PHP_EOL; |
The code is as follows | Copy code |
$arr = array('name' => 'sofish', 'age' => '25', 'gender' => 'male'); |
The code is as follows | Copy code |
$arr = array('1' => ; 'sofish', '25', 'male') $arr = array('1' => 'sofish', '2' => '25', '3' => 'male') |
The code is as follows | Copy code |
echo 'im sofish,' . PHP_EOL; echo "25 years old, n"; echo 'male'; |
// Imagine the following paragraph, the most popular answer on stackoverflow
// Personally, I don’t like mixing two types of quotation marks. It doesn’t look good, but it’s not necessarily bad. lol?!
echo 'im sofish' . "n" . '25 ...' In JS, writing n inside quotation marks is feasible.
6. if statement
In PHP, the other branch uses elseif and in JS, there is a space difference.
UPDATE: 2012.02.29 5:28 pm: Andor: "In fact, it is possible to use elseif and else if on the other branch of the if branch."
7. Function
In PHP, you cannot use functions like (function(){})() to directly run an anonymous function, but you can create a function with default parameters, such as:
The code is as follows | Copy code | ||||||||
echo $greet . $name; };
|
// When no value is passed, the default value is displayed, the result is >> 'good morning sofish'
代码如下 | 复制代码 |
$a = 'hello'; $b = function(){ echo $a . ' sofish.'; }; $b(); |
fn('sofish', 'good afternoon '); scope, I still prefer closures in JS. In PHP, let's take a look at the following code:
The code is as follows | Copy code | ||||
$a = 'hello';
echo $a . ' sofish.'; };$b(); |
Our expected result is, ‘hello sofish.’. However, there are 2 $b in me, and the results often surprise us. In PHP, variables cannot be used within custom functions, nor can externally defined variables (WTF) be used inside functions unless the global keyword is used. The above function can be used if modified as follows:
The code is as follows | Copy code | ||||
$a = 'hello'; $b = function(){ global $a;
|
The code is as follows | Copy code |
var cursorPos = (function(){ // render ... Return [posX, posY]; })(); |
In PHP, you can use list() to assign values to a set of variables based on the contents of the array, so that we can use variables to access the values we want to use instead of using subscripts:
// Use variables in brackets to access the value returned by the function `cursorPos`
list($posX, $posY) = cursorPos(); In addition, another interesting function worth mentioning isset(), which determines whether a value is NULL. There are also functions such as is_array / is_string, which are not provided in JS. Type judgment is considered a high-level content in JS. Only programmers who are familiar with it and have accumulated experience know how to do it reasonably.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


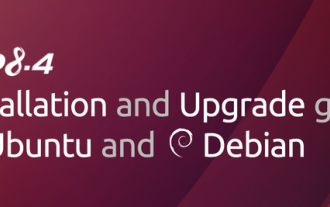
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
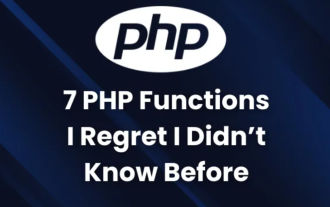
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
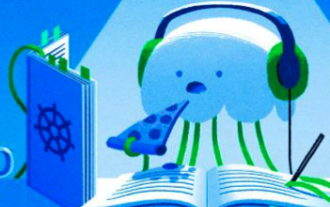
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
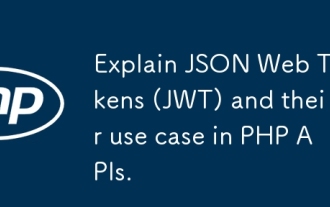
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
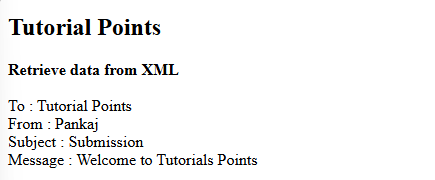
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
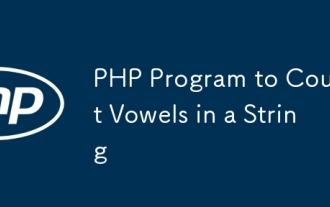
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
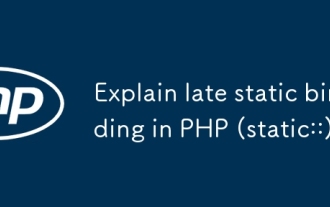
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
