


PHP connects to MySQL database and adds records to the database_PHP tutorial
First you need to connect to the MySQL database through PHP:
#Connect to database
The following is the simplest PHP code to connect to the MySQL database:
The code is as follows | Copy code | ||||
$link=mysql_connect("localhost","root","password"); if (!$link) echo "connect error"; else echo "connect ok"; ?>
|
To make it easier to use in the future, standardize the connection code:
代码如下 | 复制代码 |
$link_host='localhost'; $link_user='root'; $link_pass='password'; $link=mysql_connect($link_host,$link_user,$link_pass); if ($link) { echo "connect ok!"; } else { echo "connect fail!"; } ?> |
Use three variables to read the server address, username and password to facilitate future form reading and assignment.
#Create database code
代码如下 | 复制代码 |
include ("conn.php"); $link_db='link_system'; //设置要建立的数据库的名字,一定不能跟已有的数据库名称相同 if ($link) { echo "connect ok! "; if (mysql_query("create database ".$link_db,$link)) { echo "database created! "; } else { echo "database create fail!"; } } else { echo "connect error!"; } ?> |
After establishing the link_system database, you need to create a table.
#Create database table
//Set the table that needs to be built as link_table. The following is the name of the table that needs to be built to store different data. You can set it according to your own needs.
link_id data id
link_name Friendly link name
link_url Friendly link URL
link_detail Introduction
link_contact Contact information
link_show whether to display
link_order sort order
link_sort Category
//Because there are categories in our friend link list, we need to create a category table link_sorts. My idea is to save the location where the friend link is displayed, such as the homepage or channel page, inner page, etc.
sort_id data id
sort_name category name
The complete PHP code to create the table is as follows:
The code is as follows | Copy code |
代码如下 | 复制代码 |
//选择操作的数据库 mysql_select_db($link_db,$link); //建立表格 $link_table = "create table link_table ( link_id int unsigned primary key not null auto_increment, link_name varchar(20) not null, link_url varchar(50) not null, link_detail varchar(100) not null, link_contact varchar(100) not null, link_show int unsigned not null, link_order int unsigned not null, link_sort int unsigned not null )"; $sort_table = "create table sort_table ( sort_id int unsigned primary key not null auto_increment, sort_name varchar(20) not null )"; //执行建表操作 if(!mysql_query($link_table,$link)){ echo "Create link_table error :" . mysql_error() . " "; } else { echo "link_table Created!" . " "; } if(!mysql_query($sort_table,$link)){ echo "Create sort_table error :" . mysql_error() . " "; } else { echo "sort_table Created!" . " "; } //执行完毕关闭数据库连接 mysql_close($link); ?> |
mysql_select_db($link_db,$link);
//Create a table
$link_table = "create table link_table代码如下 | 复制代码 |
//insert.php
|
"; } else { echo "link_table Created!" . "
"; } if(!mysql_query($sort_table,$link)){ echo "Create sort_table error :" . mysql_error() . "
"; } else { echo "sort_table Created!" . "
"; } //Close the database connection after execution mysql_close($link); ?> First create a form to fill in the data that needs to be written to the MySQL database: #Write to database
The code is as follows | Copy code |
//insert.php |
is entered using text boxes, and whether to display or not is determined by using check boxes, which are selected by default.
Program page to execute writing
The code is as follows
|
Copy code
|
||||
//insert_ok.php |
include ("conn.php");
//Read the data in the form on the previous page
$link_name=$_POST[site_name];
$link_url=$_POST[site_url];
$link_contact=$_POST[site_contact];
$link_detail=$_POST[site_detail];
$link_order=$_POST[site_order];
$link_sort=$_POST[site_sort];
$link_show=$_POST[site_show];
if (!$link_show=="1") $link_show="0";
//Whether the check box is selected, if it is not selected, the value is 0
mysql_select_db("link_system", $link); //Select database link_system
if($_POST)
{
$sql = "INSERT INTO link_table (link_name,link_url,link_contact,link_detail,link_order,link_sort,link_show) VALUES ('$link_name','$link_url','$link_contact','$link_detail','$link_order',' $link_sort','$link_show')";
If(!mysql_query($sql,$link))
{
echo "Failed to add data:".mysql_error();
}
else
{
echo "Added data successfully!";
echo $_POST[site_name]."
".$_POST[site_url]."
".$_POST[site_contact]."
".$_POST[site_detail]."
".$_POST[site_sort]."
".$_POST[site_show];
}
}
?>
true

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
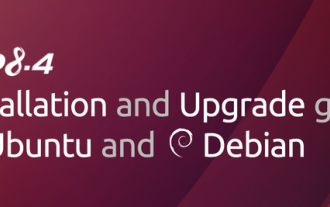
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
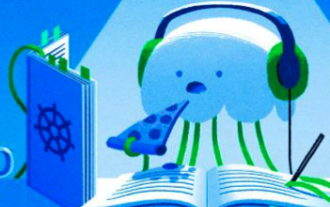
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c

One of the major changes introduced in MySQL 8.4 (the latest LTS release as of 2024) is that the "MySQL Native Password" plugin is no longer enabled by default. Further, MySQL 9.0 removes this plugin completely. This change affects PHP and other app
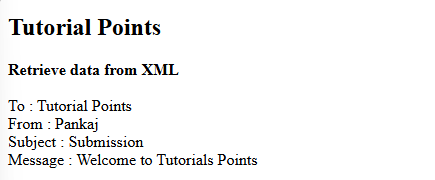
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
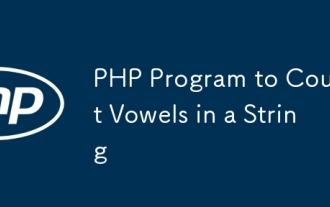
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
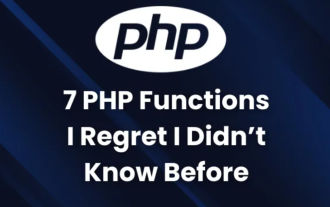
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
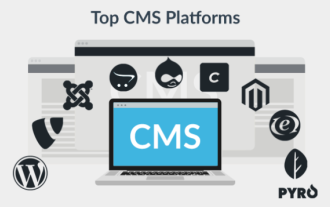
CMS stands for Content Management System. It is a software application or platform that enables users to create, manage, and modify digital content without requiring advanced technical knowledge. CMS allows users to easily create and organize content
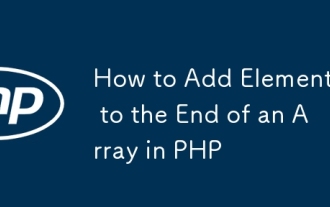
Arrays are linear data structures used to process data in programming. Sometimes when we are processing arrays we need to add new elements to the existing array. In this article, we will discuss several ways to add elements to the end of an array in PHP, with code examples, output, and time and space complexity analysis for each method. Here are the different ways to add elements to an array: Use square brackets [] In PHP, the way to add elements to the end of an array is to use square brackets []. This syntax only works in cases where we want to add only a single element. The following is the syntax: $array[] = value; Example
