


Agent and Exception Customization in PHP5 OOP Programming_PHP Tutorial
1. DBQuery object
Now, our DBQuery object simply emulates a stored procedure - once executed, it returns a result resource that must be saved; and if you want to use functions on that result set (such as num_rows() or fetch_row()), You have to pass the MySqlDB object. So, what is the effect if the DBQuery object implements the functions implemented by the MySqlDB object (which is designed to operate on the results of an executed query)? Let's continue using the code from the previous example; and let's assume that our result resources are now managed by a DBQuery object. The source code of the DBQuery class is shown in Listing 1.
Listing 1. Using the DBQuery class.
require mysql_db.php;
|
What we are most interested in in the modified code above are the catch statement and execute statement.
· The execute statement no longer returns a result resource, it now returns the DBQuery object itself.
· The DBQuery object now implements the num_rows() function—which we are already familiar with from the DB interface.
· If the query execution fails, it throws an exception of type QueryException. When converted to a string, it returns details of the error that occurred.
To do this, you need to use a proxy. In fact, you already use proxies in our DBQuery object, but now you'll use it in more depth to tie it tightly to the MySqlDB object. The DBQuery object has been initialized with an object that implements the DB interface, and it already contains a member function execute—which calls the query() method of the DB object to execute the query. The DBQuery object itself does not actually query the database, it leaves this task to the DB object. This is a proxy, which is a process by which an object can implement a specific behavior by sending messages to another object that implements the same or similar behavior.
To do this, you need to modify the DBQuery object to include all functions that operate on a result resource from the DB object. You need to use the stored results when executing a query to call the corresponding function of the DB object and return its results. The following functions will be added:
Listing 2: Extending the DBQuery class using proxies.
class DBQuery
..... public function fetch_array() { if (! is_resource($this->result)) { Throw new Exception(Query not executed.); } return $this->db->fetch_array($this->result);
|
public function __construct(DB $db) { $this->db = $db; } |
When using type hints, you can specify not only object types, but also abstract classes and interfaces.
3. Throw exception
You may have noticed from the above code that you are catching an exception called QueryException (we will implement this object later). An exception is similar to an error, but more general. The best way to describe an exception is to use emergency. Although an emergency may not be "fatal," it must still be dealt with. When an exception is thrown in PHP, the current scope of execution is quickly terminated, whether it is a function, try..catch block or the script itself. The exception then traverses the call stack—terminating each execution scope—until it is either caught in a try..catch block or it reaches the top of the call stack—at which point it generates a fatal error.
Exception handling is another new feature in PHP 5. When used in conjunction with OOP, it can achieve good control over error handling and reporting. A try..catch block is an important mechanism for handling exceptions. Once caught, script execution will continue from the next line of code where the exception was caught and handled.
If the query fails, you need to change your execute function to throw an exception. You will throw a custom exception object called QueryException - the DBQuery object that caused the error is passed to it.
Listing 3. Throw an exception.
/** *Execute current query * * Execute the current query—replacing any dot markers with the provided arguments * . * * @parameters: mixed $queryParams,... query parameters * @return: Resource A—reference describing the resource on which the query is executed. */ public function execute($queryParams = ) { //For example: SELECT * FROM table WHERE name=:1S AND type=:2I AND level=:3N $args = func_get_args(); if ($this->stored_procedure) { /*Call the compile function to get the query*/ $query = call_user_func_array(array($this, compile), $args); } else { /*A stored procedure has not been initialized, therefore, it is executed as a standard query*/ $query = $queryParams; } $result = $this->db->query($query); if (! $result) { throw new QueryException($this); |

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
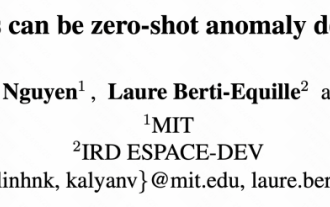
Today I would like to introduce to you an article published by MIT last week, using GPT-3.5-turbo to solve the problem of time series anomaly detection, and initially verifying the effectiveness of LLM in time series anomaly detection. There is no finetune in the whole process, and GPT-3.5-turbo is used directly for anomaly detection. The core of this article is how to convert time series into input that can be recognized by GPT-3.5-turbo, and how to design prompts or pipelines to let LLM solve the anomaly detection task. Let me introduce this work to you in detail. Image paper title: Largelanguagemodelscanbezero-shotanomalydete
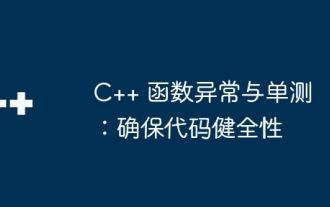
Exception handling and unit testing are important practices to ensure the soundness of C++ code. Exceptions are handled through try-catch blocks, and when the code throws an exception, it jumps to the catch block. Unit testing isolates code testing to verify that exception handling works as expected under different circumstances. Practical case: The sumArray function calculates the sum of array elements and throws an exception to handle an empty input array. Unit testing verifies the expected behavior of a function under abnormal circumstances, such as throwing an std::invalid_argument exception when an array is empty. Conclusion: By leveraging exception handling and unit testing, we can handle exceptions, prevent code from crashing, and ensure that the code behaves as expected under abnormal conditions.
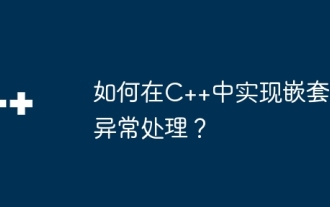
Nested exception handling is implemented in C++ through nested try-catch blocks, allowing new exceptions to be raised within the exception handler. The nested try-catch steps are as follows: 1. The outer try-catch block handles all exceptions, including those thrown by the inner exception handler. 2. The inner try-catch block handles specific types of exceptions, and if an out-of-scope exception occurs, control is given to the external exception handler.
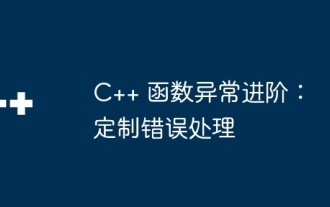
Exception handling in C++ can be enhanced through custom exception classes that provide specific error messages, contextual information, and perform custom actions based on the error type. Define an exception class inherited from std::exception to provide specific error information. Use the throw keyword to throw a custom exception. Use dynamic_cast in a try-catch block to convert the caught exception to a custom exception type. In the actual case, the open_file function throws a FileNotFoundException exception. Catching and handling the exception can provide a more specific error message.
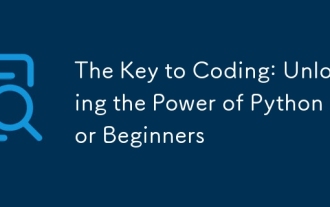
Python is an ideal programming introduction language for beginners through its ease of learning and powerful features. Its basics include: Variables: used to store data (numbers, strings, lists, etc.). Data type: Defines the type of data in the variable (integer, floating point, etc.). Operators: used for mathematical operations and comparisons. Control flow: Control the flow of code execution (conditional statements, loops).
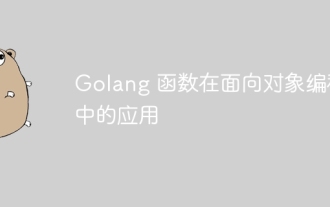
Go functions are available as methods of objects. Methods are functions associated with an object that provide access to the object's fields and methods. In Go, methods are defined using func(receiver_type)identifier(parameters)return_type syntax. This approach plays an important role in object-oriented programming by providing encapsulation, reuse, and extensibility.
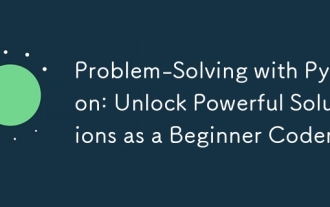
Pythonempowersbeginnersinproblem-solving.Itsuser-friendlysyntax,extensivelibrary,andfeaturessuchasvariables,conditionalstatements,andloopsenableefficientcodedevelopment.Frommanagingdatatocontrollingprogramflowandperformingrepetitivetasks,Pythonprovid
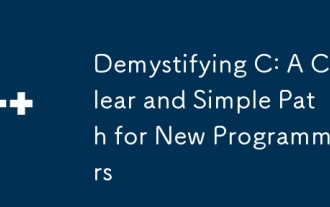
C is an ideal choice for beginners to learn system programming. It contains the following components: header files, functions and main functions. A simple C program that can print "HelloWorld" needs a header file containing the standard input/output function declaration and uses the printf function in the main function to print. C programs can be compiled and run by using the GCC compiler. After you master the basics, you can move on to topics such as data types, functions, arrays, and file handling to become a proficient C programmer.
