Implement a simple ACL_PHP tutorial
Php代码
/**
* Simple ACL permission control function
*
* Table definition
*
* 1. Resource definition (rsid,access,desc)
* 2. Role definition (id,rolename,desc)
* 3. Resource-role association (rsid, role_id)
* 4. User-role association (user_id, role_id)
*
* Depends on db.php sqlobject.php
*
* @author vb2005xu.iteye.com
*/
class AclBase {
/**
* No one is allowed to access
*/
const NOBODY = 0;
/**
* Allow anyone to access
*/
const EVERYONE = 1;
/**
* * Allow users with roles to access
*/
const HAS_ROLE = 2;
/**
* Allow users without roles to access
*/
const NO_ROLE = 3;
/**
* * Only roles defined in the resource-role association can access
*/
const ALLOCATE_ROLES = 4;
// 定义相关的 表名
public $tbResources = 'aclresources';
public $tbRoles = 'aclroles';
public $tbRefResourcesRoles = 'aclresources_aclroles';
public $tbRefUsersRoles = 'users_aclroles';
/**
* Format resource access permissions and return
* *
* @return int
*/
static function formatAccessValue($access){
static $arr = array(self::NOBODY,self::EVERYONE,self::HAS_ROLE,self::NO_ROLE,self::ALLOCATE_ROLES);
return in_array($access,$arr) ? $access : self::NOBODY;
}
/**
* Create a resource and return the resource record primary key
* *
* @param string $rsid
* @param int $access
* @param string $desc
* *
* @return int
*/
function createResource($rsid,$access,$desc){
if (emptyempty($rsid)) return false;
$resource = array(
'rsid' => $rsid,
'access' => self::formatAccessValue($access),
'desc' => $desc,
'created_at' => CURRENT_TIMESTAMP
);
return SingleTableCRUD::insert($this->tbResources,$resource);
}
/**
* Modify resources and return success status
* *
* @param array $resource
* @return int
*/
function updateResource(array $resource){
if (!isset($resource['rsid'])) return false;
$resource['updated_at'] = CURRENT_TIMESTAMP;
return SingleTableCRUD::update($this->tbResources,$resource,'rsid');
}
/**
* Delete resources
* *
* @param string $rsid
* @return int
*/
function deleteResource($rsid){
if (emptyempty($rsid)) return false;
return SingleTableCRUD::delete($this->tbResources,array('rsid'=>$rsid));
}
/**
* Create a role and return the primary key of the role record
* *
* @param string $rolename
* @param string $desc
* *
* @return int
*/
function createRole($rolename,$desc){
if (emptyempty($rolename)) return false;
$role = array(
'rolename' => $rolename,
'desc' => $desc,
'created_at' => CURRENT_TIMESTAMP
);
return SingleTableCRUD::insert($this->tbRoles,$role);
}
/**
* Modify the role and return the success status
* *
* @param array $role
* @return int
*/
function updateRole(array $role){
if (!isset($role['id'])) return false;
if (isset($role['rolename'])) unset($role['rolename']);
$role['updated_at'] = CURRENT_TIMESTAMP;
return SingleTableCRUD::update($this->tbRoles,$role,'id');
}
/**
* Delete character
* *
* @param int $role_id
* @return int
*/
function deleteRole($role_id){
if (emptyempty($role_id)) return false;
return SingleTableCRUD::delete($this->tbRoles,array('role_id'=>(int) $role_id));
}
/**
* Assign roles to resources, and remove all relevant records from the table before inserting them
* *
* @param int $rsid
* @param mixed $roleIds
* @param boolean $setNull Whether to clear resources from the association table when the role id does not exist
*/
function allocateRolesForResource($rsid,$roleIds,$setNull=false,$defaultAccess=-1){
if (emptyempty($rsid)) return false;
$roleIds = normalize($roleIds,',');
if (emptyempty($roleIds)){
if ($setNull){
SingleTableCRUD::delete($this->tbRefResourcesRoles,array('rsid'=>$rsid));
if ($defaultAccess != -1){
$defaultAccess = self::formatAccessValue($defaultAccess);
$this->updateResource(array('rsid'=>$rsid,'access'=>$defaultAccess));
}
return true;
}
return false;
}
SingleTableCRUD::delete($this->tbRefResourcesRoles,array('rsid'=>$rsid));
$roleIds = array_unique($roleIds);
foreach ($roleIds as $role_id){
SingleTableCRUD::insert($this->tbRefResourcesRoles,array('rsid'=>$rsid,'role_id'=>(int)$role_id));
}
return true;
}
function cleanRolesForResource($rsid){
if (emptyempty($rsid)) return false;
return SingleTableCRUD::delete($this->tbRefResourcesRoles,array('rsid'=>$rsid));
}
function cleanResourcesForRole($role_id){
if (emptyempty($role_id)) return false;
return SingleTableCRUD::delete($this->tbRefResourcesRoles,array('role_id'=>(int) $role_id));
}
/**
* * To allocate resources to roles, remove all relevant records from the table before inserting them
* *
* @param int $role_id
* @param mixed $rsids
* *
* @return boolean
*/
function allocateResourcesForRole($role_id,$rsids){
if (emptyempty($role_id)) return false;
$role_id = (int) $role_id;
$rsids = normalize($rsids,',');
if (emptyempty($rsids)){
return false;
}
SingleTableCRUD::delete($this->tbRefResourcesRoles,array('role_id'=>$role_id));
$rsids = array_unique($rsids);
foreach ($rsids as $rsid){
SingleTableCRUD::insert($this->tbRefResourcesRoles,array('rsid'=>$rsid,'role_id'=>$role_id));
}
return true;
}
/**
* Assign roles to users, and remove all relevant records from the table before inserting them
* *
* * There may be performance issues here when there are many users... I will think about how to optimize it later
* *
* @param int $user_id
* @param mixed $roleIds
* *
* @return boolean
*/
function allocateRolesForUser($user_id,$roleIds){
if (emptyempty($user_id)) return false;
$user_id = (int) $user_id;
$rsids = normalize($rsids,',');
if (emptyempty($rsids)){
return false;
}
SingleTableCRUD::delete($this->tbRefUsersRoles,array('user_id'=>$user_id));
$roleIds = array_unique($roleIds);
foreach ($roleIds as $roleId){
SingleTableCRUD::insert($this->tbRefUsersRoles,array('user_id'=>$user_id,'role_id'=>$role_id));
}
return true;
}
function cleanRolesForUser($user_id){
if (emptyempty($user_id)) return false;
return SingleTableCRUD::delete($this->tbRefUsersRoles,array('user_id'=>(int) $user_id));
}
function cleanUsersForRole($role_id){
if (emptyempty($role_id)) return false;
return SingleTableCRUD::delete($this->tbRefUsersRoles,array('role_id'=>(int) $role_id));
}
}
/**
* Perform acl verification on resources
*
* @param string $rsid resource identifier
* @param array $user Specific user, if not specified, the current user will be verified
*
* @return boolean
*/
function aclVerity($rsid,array $user = null){
if (emptyempty($rsid)) return false;
}
Java代码
/*
* 校验步骤如下:
*
* 1. 先校验 资源本身access 属性
* EVERYONE => true,NOBODY => false * 其它的属性在下面继续校验
* 2. 从session(或者 用户session表)中获取角色id集合
* 3. If the user has a role, then HAS_ROLE => true, NO_ROLE => false; vice versa
* 4. If resource access == ALLOCATE_ROLES
* 1. Obtain the role id set corresponding to the resource from the cache (or $tbRefResourcesRoles)
* 2. Find the intersection of the role id set owned by the user and the role id set corresponding to the resource
* 3. Intersection exists=> true; otherwise=> false
*/
It took me half an hour and I’m so dizzy that I’ll find time to perfect it tomorrow....

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
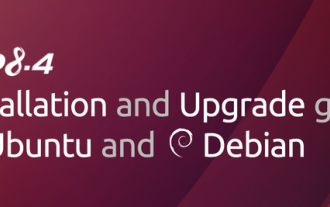
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
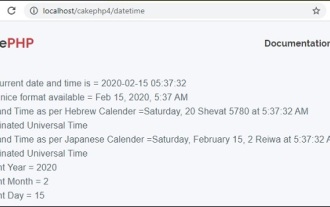
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
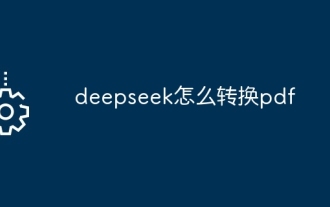
DeepSeek cannot convert files directly to PDF. Depending on the file type, you can use different methods: Common documents (Word, Excel, PowerPoint): Use Microsoft Office, LibreOffice and other software to export as PDF. Image: Save as PDF using image viewer or image processing software. Web pages: Use the browser's "Print into PDF" function or the dedicated web page to PDF tool. Uncommon formats: Find the right converter and convert it to PDF. It is crucial to choose the right tools and develop a plan based on the actual situation.
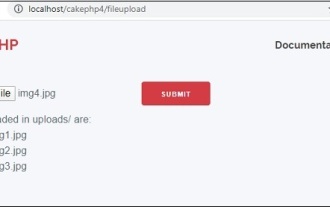
To work on file upload we are going to use the form helper. Here, is an example for file upload.
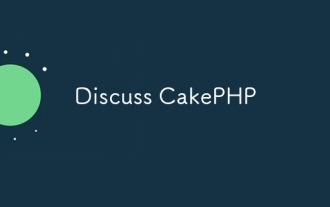
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
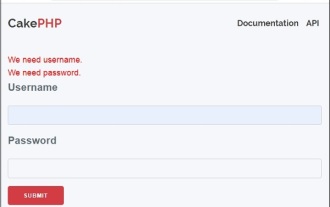
Validator can be created by adding the following two lines in the controller.
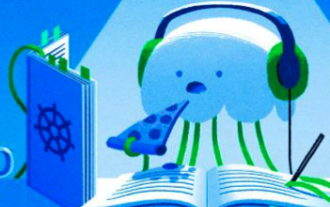
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
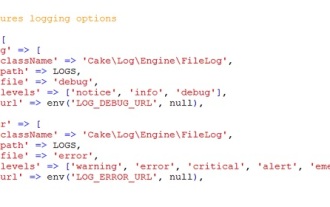
Logging in CakePHP is a very easy task. You just have to use one function. You can log errors, exceptions, user activities, action taken by users, for any background process like cronjob. Logging data in CakePHP is easy. The log() function is provide
