


Using Smarty in PHP Part 6: Smarty built-in functions_PHP tutorial
Smarty’s built-in functions: Smarty comes with some built-in functions. Built-in functions are part of the template language. Users cannot create custom functions with the same name as built-in functions, nor can they modify built-in functions.
The built-in functions in Smarty are explained below and given examples:
The Smarty template engine initialization file init.inc.php and the main file index.php used in the example
init.inc.php
Define('ROOT_PATH', dirname(__FILE__)); //Set the website root directory
require ROOT_PATH.'/libs/Smarty.class.php'; //Load Smarty template engine
$_tpl = new Smarty(); //Create an instance object
$_tpl->template_dir = ROOT_PATH.'/tpl/'; //Respecify the template directory
$_tpl->compile_dir = ROOT_PATH.'./com/'; //Respecify the compilation directory
$_tpl->left_delimiter = '<{'; //Respecify the left delimiter
$_tpl->right_delimiter = '}>'; //Respecify the right delimiter
?>
index.php
require 'init.inc.php'; //Introduce template initialization file
global $_tpl;
$_tpl->display('index.tpl'); //Introduce template
?>
capture
Properties | Type | Is it necessary | Default value | Description |
---|---|---|---|---|
name | string | no | default | Data collection area name |
assign | string | No | n/a | Where is the data collection area assigned to the variable name [to be tested] |
The function of the capture function is to capture the data output by the template and store it in a variable instead of outputting them to the page. Any data between {capture name="foo"} and {/capture} will be stored in In the variable $foo, the variable is specified by the name attribute and is accessed through $smarty.capture.foo in the template. If the name attribute is not specified, the function will use "default" as the parameter by default. {capture} must appear in pairs, that is, end with {/capture}. The function cannot be nested. Please see the example template file below.
/tpl/index.tpl
<{capture name="foo"}>
This is the content in the capture function, which is not displayed by default.
<{/capture}>
<{$smarty.capture.foo}>
2. config_load
Properties | Type | Is it necessary | Default value | Description |
---|---|---|---|---|
file | string | Yes | n/a | The name of the configuration file to include |
section | string | No | n/a | The name of the part to be loaded in the configuration file |
scope | string | no | local | The scope of loading data, the value must be local, parent or global. local indicates that the scope of the variable is the current template. parent indicates that the scope of the variable is the current template and the parent template of the current template (the template that calls the current template) .global indicates that the scope of this variable is all templates. |
global | boolean | No | No | Indicates whether the loaded variable is globally visible, equivalent to scope=parent. Note: When the scope attribute is specified, this attribute can be set, but the template ignores the attribute value and the scope attribute prevails. |
The config_load function is used to load variables from the configuration file. Regarding the use of the config_load function, please see my "Using Smarty in PHP Part 2: The use of configuration files in template variables."
3. include
Properties | Type | Is it necessary | Default value | Description |
---|---|---|---|---|
file | string | Yes | n/a | Template file name to be included |
assign | string | No | n/a | This attribute specifies a variable to hold the output of the template to be included |
[var ...] | [var type] | No | n/a | Local parameters passed to the template to be included are only valid in the template to be included |
The include function is used to include other templates in the current template. The variables in the current template are available in the included template. The file attribute must be specified, which specifies the location of the template resource. If the assign attribute is set, the variable name corresponding to this attribute is used to save the output of the template to be included, so that the output of the template to be included will not be displayed directly. See the example below:
/tpl/index.tpl
{include file="header.tpl"}
{* body of template goes here *}
{include file="footer.tpl"}
4. if,elseif,else
The if statement in Smarty is as flexible and easy to use as the if statement in PHP, and has added several features to suit the template engine. if must appear in pairs with /if. Else and elseif clauses can be used.
The following conditional modifiers can be used: eq, ne, neq, gt, lt, lte, le, gte, ge, is even, is odd, is not even, is not odd, not, mod, div by, even by, odd by, ==, !=, >, <, <=, >=. When using these modifiers, they must be separated from variables or constants by spaces.
The meaning of these modifiers is explained below:
条件修饰符 | 作用描述 |
eq | == |
ne | != |
neq | != |
gt | > |
lt | < |
lte | <= |
le | <= |
gte | >= |
ge | >= |
is even | Is it an even number |
is odd | Is it an odd number |
is not even | Is it not an even number |
is not odd | Is it not an odd number |
not | != |
mod | Find the model |
div by | Is it divisible |
even by | Is the quotient an even number |
odd by | Whether the quotient is an odd number |
&& | with |
|| | or |
() | Parentheses change priority |
5. ldelim and rdelim
Used for output delimiters, which are braces "{" and "}". The template engine always tries to interpret the content inside the braces, so if you need to output braces, use this method. See the example below:
/tpl/index.tpl
<{ldelim}>funcname<{rdelim}> is a function in Smarty.
6. literal
The data in the literal tag area will be treated as text, and the template will ignore all character information inside it. This feature is used to display JavaScript scripts that may contain character information such as braces. When this information is in {literal}{ /literal} tags, the template engine will not analyze them and display them directly. In fact, according to the tag style in all my examples (because the left delimiter and right delimiter have been reset in the init.inc.php initialization file delimiter) instead of Smarty's default style, this situation will basically not occur. Regarding the use of this function, please see the example below
/tpl/index.tpl
<{literal}>
<{/literal}>
7. php
The php tag allows php scripts to be directly embedded in templates. This tag will treat the content inside the tag as a PHP script for parsing and execution. Please see the example below
/tpl/index.tpl
<{php}>
echo date("Y-m-d H:i:s");
<{/php}>
Web developers have many times encountered situations where spaces and carriage returns affect the HTML output, so that in order to get a specific result, you have to run all the tags in the template. This problem is usually encountered in templates that are difficult to understand or process. Smarty will remove any leading and trailing spaces and carriage returns from the data in {strip}{/strip} tags before displaying. This ensures that the template is easy to understand and you don’t have to worry about extra spaces causing problems.
The built-in functions in the Smarty template engine will be summarized first. The use of the two most important functions (foreach, foreachelse, section, sectionelse) among the built-in functions will be sorted out below.
Excerpted from: Lee.’s column
http://www.bkjia.com/PHPjc/478569.html

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
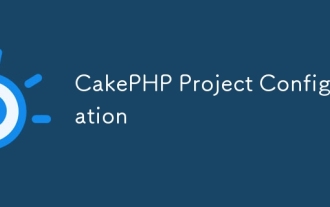
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
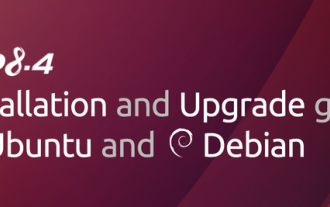
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
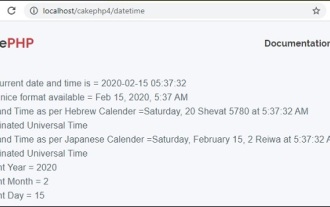
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
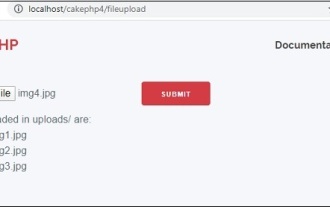
To work on file upload we are going to use the form helper. Here, is an example for file upload.
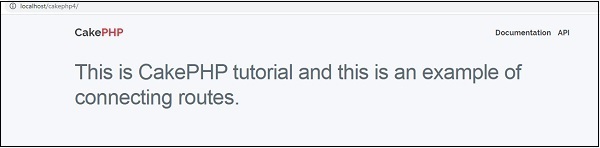
In this chapter, we are going to learn the following topics related to routing ?
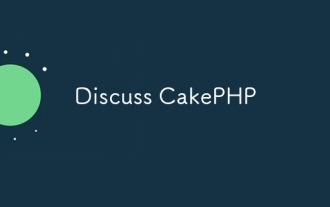
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
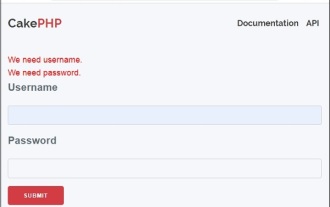
Validator can be created by adding the following two lines in the controller.
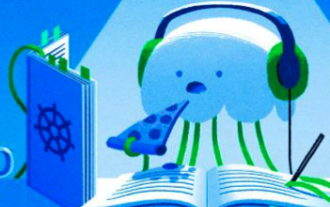
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
