


PHP generates watermark, including position and transparency_PHP tutorial
/*
Recommend it to everyone! It mainly includes image cropping, scaling, watermarking, etc. Here are some simple ways to use it.
include("imageclass.php"); //Pay attention to the path of imageclass.php here
$imgs=new image();
$imgs->param($tempFile);
if(!$imgs->thumb($targetFile,600,500))die('--Image upload failed!'); //Zoom the image
$imgs->water($targetFile,$water,$pos=0,$pct=50); mainly includes two parameters, $targetFile is the file path that has been uploaded to the server, $water is the watermark image, $pos is Watermark image position, $pct is transparency;
$pos location description:
0://random
1://1 is the top left
2://2 is top and center
3://3 means top right
4://4 means the center is on the left
5://5 is centered in the middle
6://6 means the center is on the right
7://7 means the bottom is on the left
8://8 is bottom-centered
9://9 means the bottom is on the right
Save the contents of // in imageclass.php and you can call it.
////////////////////////////////////////////////////// /////////////
*/
?>
/* +-------------------------------------------------- ---------------+
* | Copyright (c) 2008-2009 Diqiye.Com All rights reserved.
* +------------------------------------------------ -------------+
* | Info: Image processing class
* +------------------------------------------------ -------------+
*/
class image {
// The current picture <<>
protected $img; // Image type correspondence table
protected $types = array(
1 = & gt; 'gif',
2 = & gt; 'jpg',
3 = & gt; 'png',
6 = & gt; 'BMP'
);
// image
public function __construct($img=''){
!$img && $this->param($img);
}
// Info
public function param($img){
$this->img = $img;
return $this;
}
// imageInfo
public function getImageInfo($img){
$info = @getimagesize($img);
If(isset($this->types[$info[2]])){
$info['ext'] = $info['type'] = $this->types[$info[2]];
} else{
$info['ext'] = $info['type'] = 'jpg';
}
$info['type'] == 'jpg' && $info['type'] = 'jpeg';
$info['size'] = @filesize($img);
return $info;
}
// thumb(new image address, width, height, crop, allow zooming in)
Public function thumb($filename,$new_w=160,$new_h=120,$cut=0,$big=0){
// Get original image information
$info = $this->getImageInfo($this->img);
If(!empty($info[0])) {
$old_w = $info[0];
$old_h = $info[1];
$type = $info['type'];
$ext = $info['ext'];
unset($info);
// If the original image is smaller than the thumbnail and no enlargement is allowed
If($old_w < $new_h && $old_h < $new_w && !$big){
return false;
}
if($cut == 0){ // Equiproportional sequence
$scale = min($new_w/$old_w, $new_h/$old_h); // Calculate the scaling ratio
$width = (int)($old_w*$scale); // Thumbnail size
$height = (int)($old_h*$scale);
$start_w = $start_h = 0;
$end_w = $old_w;
$end_h = $old_h;
} elseif($cut == 1){ // center center crop
$scale1 = round($new_w/$new_h,2);
$scale2 = round($old_w/$old_h,2);
If($scale1 > $scale2){
$end_h = round($old_w/$scale1,2);
$end_w = round($old_h*$scale1,2);
$start_w = ($old_w-$end_w)/2;
$start_h = 0;
$end_h = $old_h;
}
$width = $new_w;
$height= $new_h;
} elseif($cut == 2){ // left top 裁剪
$scale1 = round($new_w/$new_h,2);
$scale2 = round($old_w/$old_h,2);
if($scale1 > $scale2){
$end_h = round($old_w/$scale1,2);
$end_w = $old_w;
} else{
$end_w = round($old_h*$scale1,2);
$end_h = $old_h;
}
$start_w = 0;
$start_h = 0;
$width = $new_w;
$height= $new_h;
}
// 载入原图
$createFun = 'ImageCreateFrom'.$type;
$oldimg = $createFun($this->img);
// 创建缩略图
if($type!='gif' && function_exists('imagecreatetruecolor')){
$newimg = imagecreatetruecolor($width, $height);
} else{
$newimg = imagecreate($width, $height);
}
// 复制图片
if(function_exists("ImageCopyResampled")){
ImageCopyResampled($newimg, $oldimg, 0, 0, $start_w, $start_h, $width, $height, $end_w,$end_h);
} else{
ImageCopyResized($newimg, $oldimg, 0, 0, $start_w, $start_h, $width, $height, $end_w,$end_h);
}
//Set interlacing for jpeg graphics
$type == 'jpeg' && imageinterlace($newimg,1);
$imageFun = 'image'.$type;
!@$imageFun($newimg,$filename,100) && die('Save failed! Check whether the directory exists and is writable?');
ImageDestroy($newimg);
ImageDestroy($oldimg);
return $filename;
}
return false;
}
// water(save address, watermark image, watermark position, transparency)
public function water($filename,$water,$pos=0,$pct=50){
$info = $this->getImageInfo($water);
If(!empty($info[0])){
$water_w = $info[0];
$water_h = $info[1];
$type = $info['type'];
$fun = 'imagecreatefrom'.$type;
$waterimg = $fun($water);
} else{
return false;
}
$info = $this->getImageInfo($this->img);If(!empty($info[0])){
$old_h = $info[1];
$type = $info['type'];
$fun = 'imagecreatefrom'.$type;
$oldimg = $fun($this->img);
} else{
return false;
}
$water_w >$old_w && $water_w = $old_w;
$water_h >$old_h && $water_h = $old_h;
// Watermark position
switch($pos){
case 0://random
$posX = rand(0,($old_w - $water_w));
$posY = rand(0,($old_h - $water_h));
break;
case 1://1 is the top left
$posX = 0;
$posY = 0;
break;
case 2://2 is top-centered
$posX = ($old_w - $water_w) / 2;
$posY = 0;
break;
case 3://3 is the top right
$posX = $old_w - $water_w;
$posY = 0;
break;
case 4://4 is the center on the left
$posX = 0;
$posY = ($old_h - $water_h) / 2;
break;
case 5://5 is centered in the middle
$posX = ($old_w - $water_w) / 2;
$posY = ($old_h - $water_h) / 2;
break;
case 6://6 is the center on the right
$posX = $old_w - $water_w;
$posY = ($old_h - $water_h) / 2;
break;
case 7://7 means the bottom is on the left
$posX = 0;
$posY = $old_h - $water_h;
break;
case 8://8 is the bottom center
$posX = ($old_w - $water_w) / 2;
$posY = $old_h - $water_h;
break;
case 9://9 is the bottom right
$posX = $old_w - $water_w;
$posY = $old_h - $water_h;
break;
Default: // Random
$posX = rand(0,($old_w - $water_w));
$posY = rand(0,($old_h - $water_h));
break;
}
// Set the color blending mode of the image
Imagealphablending($oldimg, true);
Imagecopymerge($oldimg, $waterimg, $posX, $posY, 0, 0, $water_w,$water_h,$pct);
$fun = 'image'.$type;
!@$fun($oldimg, $filename,100) && die('Save failed! Check whether the directory exists and is writable?');
imagedestroy($oldimg);
imagedestroy($waterimg);
return $filename;
}
}
$imgs=new image();
$tempFile = "./images/hdb.jpg";
$water = "./images/bamiluo.jpg";
$imgs->param($tempFile);
//if(!$imgs->thumb($targetFile,600,500))die('fail');
$imgs->water($targetFile,$water,0,10);
echo "aaa";
?>
Excerpted from: ws07_byyy’s column

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
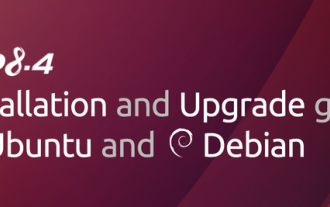
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
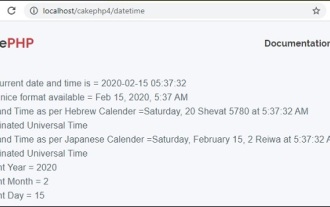
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
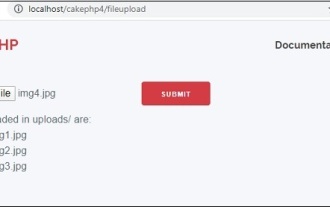
To work on file upload we are going to use the form helper. Here, is an example for file upload.
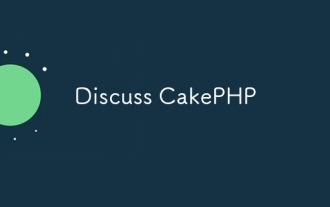
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
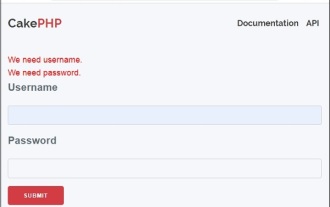
Validator can be created by adding the following two lines in the controller.
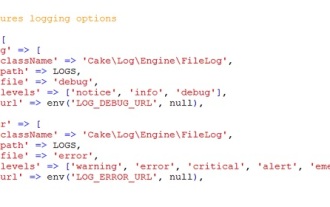
Logging in CakePHP is a very easy task. You just have to use one function. You can log errors, exceptions, user activities, action taken by users, for any background process like cronjob. Logging data in CakePHP is easy. The log() function is provide
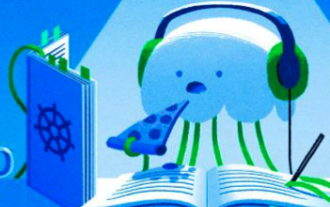
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
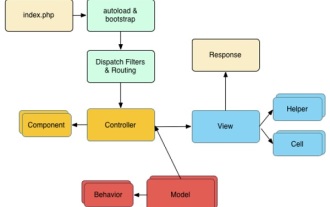
CakePHP is an open source MVC framework. It makes developing, deploying and maintaining applications much easier. CakePHP has a number of libraries to reduce the overload of most common tasks.
