


Useful MYSQL operation classes in PHP (Interpretation of design patterns 1)_PHP tutorial
It is very simple to use PHP to operate a database. Even those who have started with PHPER can do it. However, when dealing with a large number of table operations, we are tired of many MYSQL statements, so we are eager to encapsulate a large number of database operations. stand up. So database object mapping appeared.
First we create a new interface.
singleton.class.php
[php]
/**
* @author tomyjohn
* @link
* @license
* @version 1.0
* @copyright Copyright 2010 tomyjohn - tomyjohn.gicp.net
* @package singleton
*/
/**
* Database object
*/
interface singleton{
/**
* Generate database object
* @returns object data object;
* @access public
*/
Public static function getInstance();
}
Create a new abstract class. This abstract class simply uses 5 methods to abstract all databases.
db.class.php
[php]
/**
* @author tomyjohn
* @link
* @license
* @version 1.0
* @copyright Copyright 2010 tomyjohn - tomyjohn.gicp.net
* @package db
*/
/**
*Abstract DB class
*/
abstract class db{
/**
* Factory mode
* @param string $type sql type
* @returns object
* @access public
*/
Public static function factory($type){
return call_user_func(array($type,'getInstance'));
}
/**
* Execute SQL statement
* @param string $query sql statement
* @return object resource or false;
* @access public
*/
abstract public function execute($query);
/**
* Get the array returned by the SQL statement
* @param string $query sql statement
* @return object resource or false;
* @access public
*/
abstract public function get_array($query);
/**
* Get the execution ID of the previous statement
* @param string $query sql statement
* @return integer number or false;
* @access public
*/
abstract public function insert_get_id($query);
/**
* Convert special characters
* @param string $string
* @return string processed string
* @access public
*/
abstract public function clean($string);
}
I believe that after reading this, you will all think of how to use the call_user_func method. Don’t worry, read on
mysql.class.php
[html]
/**
* @author tomyjohn
* @link
* @license
* @version 1.0
* @copyright Copyright 2010 tomyjohn - tomyjohn.gicp.net
* @package db
*/
/**
*MYSQL database object
*/
class mysql extends db implements singleton{
/**
* @var $instance object
* @access current class
*/
protected static $instance = null;
/**
* @var $link resource
* @access current class
*/
protected $link;
/**
* Database instance
* @return $self::instance object
*/
Public static function getInstance(){
If(is_null(self::$instance)){
self::$instance = new self();
}
return self::$instance;
}
/**
* Constructor
*/
protected function __construct(){
global $current_conf;
$user = $current_conf['DBUSER'];
$pass = $current_conf['DBPWD'];
$host = $current_conf['DBHOST'];
$db = $current_conf['DBNAME'];
$this->link = mysql_connect($host,$user,$pass);
mysql_set_charset($current_conf['DBCHARSET'] , $this->link);
Mysql_select_db($db);
}
/**
* Convert special characters
* @param string $string
* @return string processed string
* @access public
*/
Public function clean($string){
return mysql_real_escape_string($string,$this->link);
}
/**
* Execute SQL statement
* @param string $query sql statement
* @return object resource or false;
* @access public
*/
Public function execute($query){
return mysql_query($query,$this->link);
}
/**
* Get the execution ID of the previous statement
* @param string $query sql statement
* @return integer number or false;
* @access public
*/
Public function insert_get_id($query){
$this->execute($query);
return mysql_insert_id($this->link);
}
/**
* Get the array returned by the SQL statement
* @param string $query sql statement
* @return object resource or false;
* @access public
*/
Public function get_array($query){
$result = $this->execute($query);
$return = array();
If($result){
while($row = mysql_fetch_array($result, MYSQL_ASSOC)){
$return[] =$row;
}
return $return;
}
}
[html] view plaincopy
$connection = db::factory('mysql');
$sql = "SELECT * FROM table";
$value_array = $connection->get_array($sql);
[html] view plaincopy
print_r($value_array);
Although this solves the problem of expansion and some repeated operations, it is not very convenient. We should go one step further and make the database table represented by objects, that is, database mapping
[html]
class dao{
/**
* @var $values array stores database objects
* @access current class
*/
protected $values = array();
/**
* @var $suffix array stores database objects
* @access public
*/
public $suffix = '';
/**
* Constructor
*/
public function __construct($qualifier = null){
global $current_conf;
$this->suffix = $current_conf['DBSUFFIX'];
if(!is_null($qualifier)){
$conditional = array();
if(is_numeric($qualifier)){
$conditional = array('id'=>$qualifier);
}
else if(is_array($qualifier)){
$conditional = $qualifier;
}
else{
throw new Exception('Invalid type of qualifier given!');
}
$this->populate($conditional);
}
}
public function __set($name , $value){
$this->values[$name] = $value;
}
public function __get($name){
if(isset($this->values[$name])){
return $this->values[$name];
}
else{
return null;
}
}
/**
* * Parse the parameters of the instance
* @param $conditional obj
*/
protected function populate($conditional){
$connection = db::factory('mysql');
$sql = "SELECT * FROM {$this->suffix}{$this->table} WHERE ";
$qualifier = '';
foreach($conditional as $column => $value){
if(!empty($qualifier)){
$qualifier .= ' AND ';
}
$qualifier .= "`{$column}`='" . $connection->clean($value) . "' ";
}
$sql .= $qualifier;
$value_array = $connection->get_array($sql);
if(!isset($value_array[0])){
$value_array[0] = array();
}
foreach($value_array[0] as $key => $value){
$this->values[$key] = $value;
}
}
/**
* Save data
*/
public function save(){
if(!$this->id){
$this->create();
}
else{
return $this->update();
}
}
/**
* Add data www.2cto.com
*/
public function create(){
$connection = db::factory('mysql');
$sql = "INSERT INTO {$this->suffix}{$this->table}(`";
$sql .= implode('`, `' , array_keys($this->values));
$sql .="`) VALUES('";
$clean = array();
foreach($this->values as $value){
$clean[] = $connection->clean($value);
}
$sql .= implode("', '" , $clean);
$sql .="')";
$this->id = $connection->insert_get_id($sql);
}
/**
* Update data
* @return returns the result of the operation
*/
public function update(){
$connection = db::factory('mysql');
$sql = "UPDATE {$this->suffix}{$this->table} set ";
$updates = array();
foreach($this->values as $key=>$value){
if($key!='id'){
$updates[] = "`{$key}`='" . $connection->clean($value) . "'";
}
}
$sql .= implode(',' , $updates);
$sql .= " WHERE id={$this->id}";
return $connection->execute($sql);
}
/**
* Delete data
* @return returns the result of the operation
*/
Public function delete(){
$connection = db::factory('mysql');
$sql = "DELETE FROM {$this->suffix}{$this->table} WHERE ";
$qualifier = 'id='.$this->id;
$sql .= $qualifier;
return $connection->execute($sql);
}
/**
* Convert object to array
* @return array
*/
Public function object_to_array(){
return $this->values;
}
}
I will write one
news.dao.class
class news extends dao{
protected $table = __CLASS__;
}

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
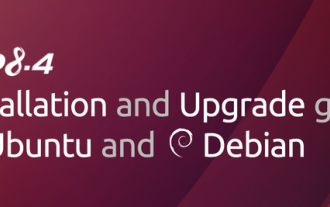
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati

One of the major changes introduced in MySQL 8.4 (the latest LTS release as of 2024) is that the "MySQL Native Password" plugin is no longer enabled by default. Further, MySQL 9.0 removes this plugin completely. This change affects PHP and other app
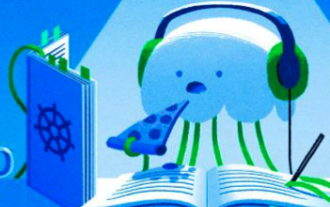
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
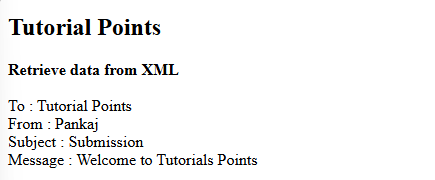
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
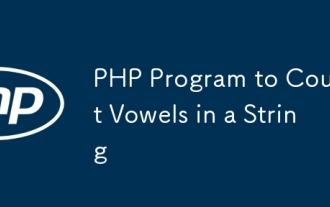
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
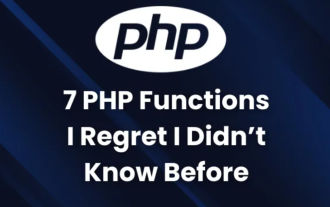
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
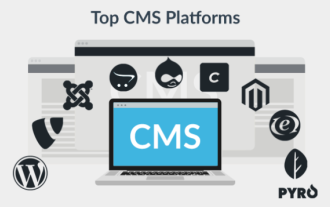
CMS stands for Content Management System. It is a software application or platform that enables users to create, manage, and modify digital content without requiring advanced technical knowledge. CMS allows users to easily create and organize content
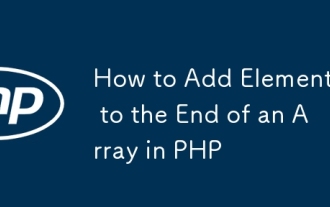
Arrays are linear data structures used to process data in programming. Sometimes when we are processing arrays we need to add new elements to the existing array. In this article, we will discuss several ways to add elements to the end of an array in PHP, with code examples, output, and time and space complexity analysis for each method. Here are the different ways to add elements to an array: Use square brackets [] In PHP, the way to add elements to the end of an array is to use square brackets []. This syntax only works in cases where we want to add only a single element. The following is the syntax: $array[] = value; Example
