


How to generate PHP QR code (google API, PHP class library, libqrencode, etc.)_PHP tutorial
================================================PHP How to use================================================ ==
1. Use Google Open API to complete
[php]
$urlToEncode="name:Liu Xinming,phone:18792448443";
generateQRfromGoogle($urlToEncode);
/**
* google api QR code generation [QRcode can store up to 4296 alphanumeric characters of any text, please check the QR code data format for details]
* @param string $chl The information contained in the QR code can be numbers, characters, binary information, or Chinese characters. Data types cannot be mixed, and the data must be UTF-8 URL-encoded. If the information that needs to be transferred exceeds 2K bytes, please use the POST method
* @param int $widhtHeight Size setting for generating QR code
* @param string $EC_level Optional error correction level. QR code supports four levels of error correction, which is used to recover lost, misread, ambiguous, and data.
* L-Default: Can identify 7% of the data that has been lost
* M-Can identify data that has lost 15%
* Q-Can identify data that has lost 25%
* H-Can identify data that has lost 30%
* @param int $margin The distance between the generated QR code and the image border
*/
function generateQRfromGoogle($chl,$widhtHeight ='150',$EC_level='L',$margin='0')
{
$chl = urlencode($chl);
echo '';
}
For more details, please refer to Baidu Library: http://wenku.baidu.com/view/b2a6ffc60c22590102029dae.html
2. PHP class library PHP QR Code
PHP QR Code is open source (LGPL) library for generating QR Code,
2-dimensional barcode. Based on libqrencode C library,
provides API for creating QR Code barcode images (PNG, JPEG thanks to GD2).
Implemented purely in PHP, with no external dependencies (except GD2 if needed).
PS: Due to time constraints, I only tested Google’s open API and it was normal. Others can be tested by yourself.
=============================================== Learn about the next two The principle of QR code============================================== ====
2-dimensional bar code is a black and white graphic that uses a specific geometric figure distributed on a plane according to certain rules to record data and information.
Commonly used code systems include: Data Matrix, Maxi Code, Aztec, QR Code, Vericode, PDF417, Ultracode, Code 49, Code 16K, etc.
①: Stacked/lined 2D barcode, such as Code 16K, Code 49, PDF417 (as shown below), etc.
②: Matrix QR code, the most popular is QR CODE
QR codes store a larger amount of data; they can contain mixed content such as numbers, characters, and Chinese text; they have a certain fault tolerance (can be read normally after some damage); they have high space utilization, etc.
③:Introduction to QR CODE
As shown above, the basic structure of a qrcode, where:
Position detection graphics, position detection graphics separators, positioning graphics: used to locate QR codes. For each QR code, the location is fixed, but the size and specifications will vary;
Correction graphics: Once the specifications are determined, the number and location of the correction graphics are also determined;
Format information: Indicates the error correction level of the QR code, divided into L, M, Q, H;
Version information: that is, the specifications of the QR code. There are 40 matrix specifications of QR code symbols (usually black and white), from 21x21 (version 1) to 177x177 (version 40). Each version symbol is larger than the previous one. Version adds 4 modules per side.
Data and error correction code words: the actual saved QR code information, and error correction code words (used to correct errors caused by damage to the QR code).
Brief coding process:
1. Data analysis: Determine the encoded character type and convert it into symbolic characters according to the corresponding character set; Select the error correction level. Under certain conditions, the higher the error correction level, the smaller the real data capacity.
2. Data encoding: Convert data characters into a bit stream, with one codeword for every 8 bits, forming a data codeword sequence as a whole. In fact, knowing this data code word sequence means you know the data content of the QR code.
Data can be encoded according to a pattern for more efficient decoding, for example: Data: 01234567 encoding (version 1-H),
1) Group: 012 345 67
2) Convert to binary: 012→0000001100
3) Convert to sequence: 0000001100 0101011001 1000011
4) The number of characters is turned into binary: 8 → 0000001000
Add the mode indicator (the number in the picture above) 0001: 0001 0000001000 0000001100 0101011001 1000011
For letters, Chinese, Japanese, etc., only the grouping methods, modes and other contents are different. The basic method is the same
3. Error correction coding: Divide the above codeword sequence into blocks as needed, generate error correction codewords according to the error correction level and the block codewords, and add the error correction codewords to the data codeword sequence Later, it becomes a new sequence.
When the specifications and error correction level of the QR code are determined, the total number of code words and error correction code words that it can accommodate are also determined. For example: version 10, when the error correction level is H, can accommodate a total of 346 Codewords, including 224 error correction codewords.
The error correction capacity is: 112/346=32.4%
4. Construct the final data information: Under the conditions of determined specifications, put the sequences generated above into blocks in order
Divide the data into blocks according to regulations, and then calculate each block to obtain the corresponding error correction codeword block. The error correction codeword blocks are formed into a sequence in order and added to the end of the original data codeword sequence.
Such as: D1, D12, D23, D35, D2, D13, D24, D36, ... D11, D22, D33, D45, D34, D46, E1, E23, E45, E67, E2, E24, E46, E68,. ..
Construct matrix: Put detection graphics, separators, positioning graphics, correction graphics and codeword modules into the matrix.
Fill the above complete sequence into the area of the QR code matrix of the corresponding specifications
6. Masking: Use masking graphics for the coding area of the symbol so that the dark and light (black and white) areas in the QR code graphics can be distributed with optimal ratios.
A algorithm, no research, interested students can continue.
Versions 7-40 all contain version information, and those without version information are all 0. The two locations on the QR code contain version information, which are redundant.
The version information has a total of 18 bits, a 6X3 matrix, of which the 6-bit data is, for example, version number 8, the data bit information is 001000, and the following 12 bits are error correction bits.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


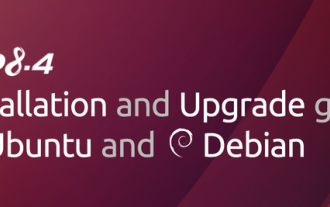
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
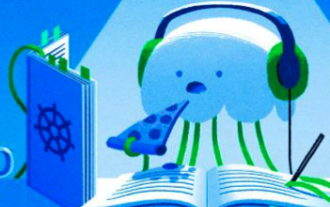
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
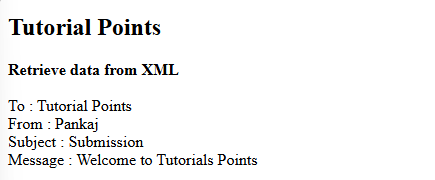
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
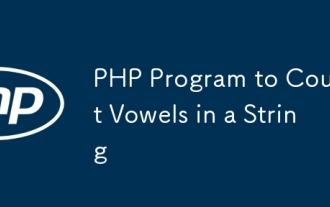
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
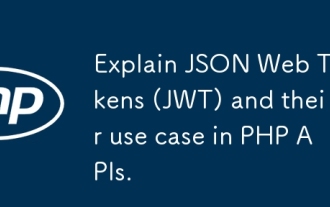
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
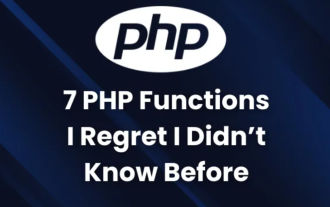
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
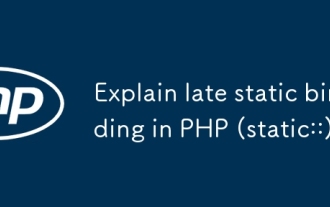
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
