


List of ThinkPHP's CURD methods and query methods_PHP tutorial
The so-called CURD. That is, the four basic operations (CURD) of database operations: C:create (create), U:update (update), R:read (read) and D:detele (delete).
In ThinkPHP, methods do not necessarily have these names. Here are the common methods: select, find, findAll, save, create, etc.:
D read:
select->() queries the data set, which is the same as findAll->(). For example:
$User->where(‘status=1′)->order(‘create_time’)->limit(10)->select();
Note: Except for the select method, which must be placed last in a coherent operation, the order of calling methods of other consecutive operations is not sequential. For example, the following code is equivalent to the above:
$User->order(‘create_time’)->where(‘status=1′)->limit(10)->select();
If you are not used to using continuous operations, the new version also supports the method of directly using parameters to perform queries. For example, the above code can be changed to
Written as:
$User->select(array('order'=>'create_time', 'where'=>'status=1', 'limit'=>'10'));
The find->() method is similar to the above two methods. The difference is that only one piece of data is returned. Can be used together with getField->() to obtain a field value of a record.
Select has the same effect as findall and returns a two-dimensional array. Such as
array(1) {
[0] => array(8)
{ ["rank_id"] => string(3) “151″
["rank_name"] => string(7) "Test 9"
["rank_memo"] => string(3) “123″
["uid"] => string(5) “59471″
["rank_kw"] => string(6) "Important"
["rank_uptime"] => string(10) “1280202914″
["isverify"] => string(1) “0″
["ishot"] => string(1) “0″
}
}
The effect of find is as follows, returning a one-dimensional array:
array(8) {
["rank_id"] => string(3) “151″
["rank_name"] => string(7) "Test 9"
["rank_memo"] => string(3) “123″
["uid"] => string(5) “59471″
["rank_kw"] => string(6) "Important"
["rank_uptime"] => string(10) “
1280202914″ ["isverify"] => string(1) “0″
["ishot"] => string(1) “0″
}
Where method: used to query or update the definition of conditions
Table method: Define the name of the data table to be operated
$Model->Table(‘think_user user’)->where(‘status>1′)->select();
field method: define the field to be queried
The parameters of the field method support strings and arrays, for example,
$Model->field(‘id,nickname as name’)->select();
$Model->field(array(‘id’,’nickname’=>’name’))->select();
If you do not use the field method to specify a field, the default is equivalent to using field(‘*’).
U updated, C created:
data, add, save methods: assign, add, and save data objects. For example:
$data['name'] = 'ThinkPHP';
$data['email'] = ‘ThinkPHP@gmail.com’;
$Model->data($data)->add();//Newly added, equivalent to insert, coherent writing
$Model->add($data); //New, equivalent to insert, non-coherent writing
$Model->data($data)->where(‘id=3′)->save(); //Modify, equivalent to update
It should be noted that in the save method, if the data has not changed, the default return operation is FALSE. But this save execution is OK, this needs attention.
create->() automatically forms data in the form of $data from the POST fields
$User=D("User");
$User->create(); //Create by default using data submitted by the form
$User->add(); //Add
If your primary key is of the auto-increasing type, and if the data is successfully inserted, the return value of the Add method is the latest entered primary key value, which can be obtained directly.
Data objects created using the data method will not be automatically verified and filtered, please handle it yourself. But those who are practicing add command
During the save operation, fields that do not exist in the data table and illegal data types (such as objects, arrays and other non-scalar data) will automatically
With automatic filtering, you don't have to worry about SQL errors caused by writing to non-data table fields.
The command verification, automatic verification and automatic completion (we will look at the related usage later) functions that we are familiar with are actually
Must pass the create method to take effect. The data object created by the Create method is stored in memory and is not actually written to the database
, just use the add or save method. If you just want to simply create a data object, there is no need to complete some additional functions
If so, you can use the data method to simply create a data object.
setInc and setDec methods. For updates to statistical fields (usually numeric types):
$Model->setInc(‘score’,’id=5′,3); // Add 3 to the user’s points
$Model->setInc(‘score’,’id=5′); // Add 1 to the user’s points
$Model->setDec(‘score’,’id=5′,5); // The user’s points are reduced by 5
$Model->setDec(‘score’,’id=5′); // The user’s points are reduced by 1
D Delete:
delete->() delete data
$User->where(‘status=0′)->order(‘create_time’)->limit(’5′)->delete();
Other common methods of Model:
order method: result sorting Example:
order(‘id desc’)
The sorting method supports sorting multiple fields
order(‘status desc,id asc’)
The parameters of the order method support strings and arrays. The usage of arrays is as follows:
order(array(‘status’=>’desc’,’id’))
limit method: result limit
limit(’1,10′)
If using limit(’10′) it is equivalent to limit(’0,10′)
Page method: Query paging. The usage of the Page method is similar to the limit method. The format is:
Page(‘page[,listRows]‘)
Page represents the current page number, and listRows represents the number of records displayed on each page. For example, if 10 records are displayed on each page, get the data on page 2:
Page(’2,10′)
If listRow is not written, the value of limit(‘length’) will be read. For example, if 25 records are displayed on each page, the data on page 3 will be obtained:
limit(25)->page(3);
If limit is not set, the default is to display 20 records per page.
Join method: Query Join support. The parameters of the Join method support strings and arrays, and the join method is the only method that can be called multiple times in a coherent operation. For example:
$Model->join(‘ work ON artist.id = work.artist_id’)->join(‘card ON artist.card_id = card.id’)->select();
The LEFT JOIN method is used by default. If you need to use other JOIN methods, you can change it to
$Model->join(‘RIGHT JOIN work ON artist.id = work.artist_id’)->select();
Distinct method: Query Disiinct support. Perform unique filtering when querying data
$Model->Distinct(true)->select();
Relation method: related query support
$Model->Relation(true)->select();
Conditional query
$map->put('name','php'); //name='php'
('name',array('like','think')); //name like '...'
('id',array('in',array(1,2,4)));
('id',array('10','3','or')); //id>=10 or <=3
thinkphp multi-table query statement
1. table() function
thinkphp provides a table() function. For specific usage, please refer to the following statement:
$list=$Demo->table('think_blog blog,think_type type')->where('blog.typeid=type.id')->field('blog.id as id,blog.title,blog .content,type.typename as type')->order('blog.id desc' )->limit(5)->select();
echo $Demo->getLastSql(); //Print the SQL statement and check it
2. join() function
Take a look at the code:
$Demo = M('artist');
$Demo->join('RIGHT JOIN think_work ON think_artist.id = think_work.artist_id' );
//You can use INNER JOIN or LEFT JOIN. Be sure to pay attention to the prefix of the table name here!
echo $Demo->getLastSql(); //Print the SQL statement and check it
Attached is the simplest code for adding, deleting and checking
[php]
// Query data
public function index(){
$Form = M("Form");
$list = $Form->limit(5)->order('id desc')->findAll();
$this->assign('list',$list);
$this->display();
}
//Write data
public function insert() {
$Form = D("Form");
If($vo = $Form->create()) {
$list=$Form->add();
If($list!==false){
$this->success('Data saved successfully!');
}else{
$this->error('Data writing error!');
}
}else{
$this->error($Form->getError());
}
}
//Update data
public function update() {
$Form = D("Form");
If($vo = $Form->create()) {
$list=$Form->save();
If($list!==false){
$this->success('Data updated successfully!');
}else{
$this->error("No data updated!");
}
}else{
$this->error($Form->getError());
}
}
// Delete data
public function delete() {
If(!emptyempty($_POST['id'])) {
$ Form = m ("form");
$ Result = $ form-& gt; delete ($ _ post ['id']);
If(false !== $result) {
$this->ajaxReturn($_POST['id'],'Delete successfully!',1);
}else{
$this->error('Deletion error!');
}
}else{
$this->error('The deleted item does not exist!');
}
}

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
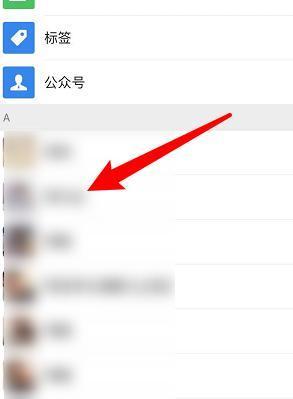
Unfortunately, people often delete certain contacts accidentally for some reasons. WeChat is a widely used social software. To help users solve this problem, this article will introduce how to retrieve deleted contacts in a simple way. 1. Understand the WeChat contact deletion mechanism. This provides us with the possibility to retrieve deleted contacts. The contact deletion mechanism in WeChat removes them from the address book, but does not delete them completely. 2. Use WeChat’s built-in “Contact Book Recovery” function. WeChat provides “Contact Book Recovery” to save time and energy. Users can quickly retrieve previously deleted contacts through this function. 3. Enter the WeChat settings page and click the lower right corner, open the WeChat application "Me" and click the settings icon in the upper right corner to enter the settings page.
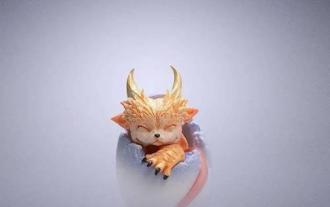
Mobile games have become an integral part of people's lives with the development of technology. It has attracted the attention of many players with its cute dragon egg image and interesting hatching process, and one of the games that has attracted much attention is the mobile version of Dragon Egg. To help players better cultivate and grow their own dragons in the game, this article will introduce to you how to hatch dragon eggs in the mobile version. 1. Choose the appropriate type of dragon egg. Players need to carefully choose the type of dragon egg that they like and suit themselves, based on the different types of dragon egg attributes and abilities provided in the game. 2. Upgrade the level of the incubation machine. Players need to improve the level of the incubation machine by completing tasks and collecting props. The level of the incubation machine determines the hatching speed and hatching success rate. 3. Collect the resources required for hatching. Players need to be in the game
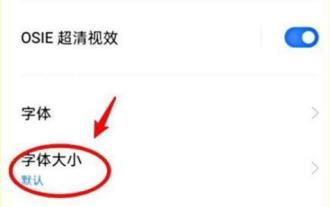
Setting font size has become an important personalization requirement as mobile phones become an important tool in people's daily lives. In order to meet the needs of different users, this article will introduce how to improve the mobile phone use experience and adjust the font size of the mobile phone through simple operations. Why do you need to adjust the font size of your mobile phone - Adjusting the font size can make the text clearer and easier to read - Suitable for the reading needs of users of different ages - Convenient for users with poor vision to use the font size setting function of the mobile phone system - How to enter the system settings interface - In Find and enter the "Display" option in the settings interface - find the "Font Size" option and adjust it. Adjust the font size with a third-party application - download and install an application that supports font size adjustment - open the application and enter the relevant settings interface - according to the individual
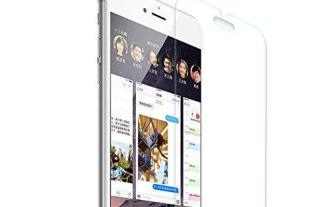
Mobile phone film has become one of the indispensable accessories with the popularity of smartphones. To extend its service life, choose a suitable mobile phone film to protect the mobile phone screen. To help readers choose the most suitable mobile phone film for themselves, this article will introduce several key points and techniques for purchasing mobile phone film. Understand the materials and types of mobile phone films: PET film, TPU, etc. Mobile phone films are made of a variety of materials, including tempered glass. PET film is relatively soft, tempered glass film has good scratch resistance, and TPU has good shock-proof performance. It can be decided based on personal preference and needs when choosing. Consider the degree of screen protection. Different types of mobile phone films have different degrees of screen protection. PET film mainly plays an anti-scratch role, while tempered glass film has better drop resistance. You can choose to have better
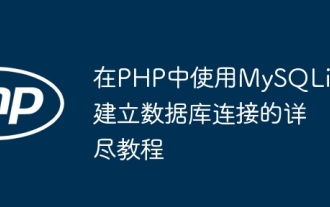
How to use MySQLi to establish a database connection in PHP: Include MySQLi extension (require_once) Create connection function (functionconnect_to_db) Call connection function ($conn=connect_to_db()) Execute query ($result=$conn->query()) Close connection ( $conn->close())
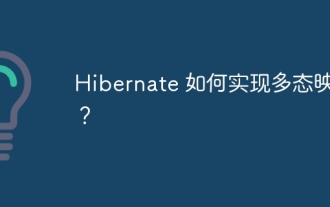
Hibernate polymorphic mapping can map inherited classes to the database and provides the following mapping types: joined-subclass: Create a separate table for the subclass, including all columns of the parent class. table-per-class: Create a separate table for subclasses, containing only subclass-specific columns. union-subclass: similar to joined-subclass, but the parent class table unions all subclass columns.
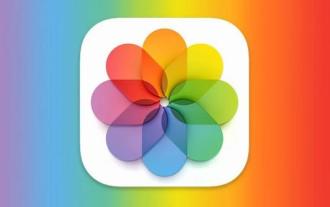
Apple's latest releases of iOS18, iPadOS18 and macOS Sequoia systems have added an important feature to the Photos application, designed to help users easily recover photos and videos lost or damaged due to various reasons. The new feature introduces an album called "Recovered" in the Tools section of the Photos app that will automatically appear when a user has pictures or videos on their device that are not part of their photo library. The emergence of the "Recovered" album provides a solution for photos and videos lost due to database corruption, the camera application not saving to the photo library correctly, or a third-party application managing the photo library. Users only need a few simple steps
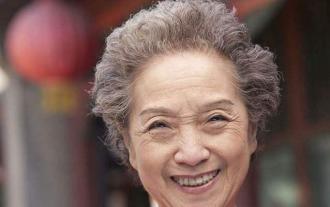
Age spots are a common skin problem that tend to appear on the faces of older adults on exposed areas such as the neck and back of hands. It will also make people look older. It not only brings trouble to people's appearance. With the advancement of science and technology and the development of beauty technology, there are now many simple and quick ways to remove age spots, however. To restore young and healthy skin and help you eliminate age spots quickly, this article will share some effective skin care tips. 1. The importance of actively protecting the skin from ultraviolet damage, so it is very important to avoid prolonged exposure to the sun. Ultraviolet rays are one of the main causes of age spots. 2. Choose skin care products reasonably and use products containing antioxidants and whitening ingredients. Skin care products containing antioxidants and whitening ingredients can help reduce the appearance of age spots.
