


Full analysis of php global variables and classes used together_PHP tutorial
Case 1:
father.php is defined as follows:
$jack = 1000;
?>
children.php is defined as follows:
require("father.php");
$jack=123;
echo $jack."/n";
?>
php children.php
The running output is 123.
If $jack=123 is commented out, the running result is 1000. If $jack=123 is placed before require("father.php");, the running result is 1000.
Easier to understand: PHP explains and executes, wherever it is explained, it is executed. . Global variables like $jack are global variables. For example, in the first case, when it is initially used, it is 1000, which is in require
When running, the result was changed to 123, so the running result output is 123.
Case 2:
The children.php code is changed to the following:
require("father.php");
function testJack(){
if(!isset($jack)){
echo '$jack is null'."/n";
}
}//testJack
testJack();
?>
php children.php
The operation result is: $jack is null. That is to say, the $jack referenced in testJack() is a local variable.
If you use the global keyword and declare $jack as a global variable, the code will be changed to the following:
require("father.php");
function testJack(){
global $jack;
if(!isset($jack)){
echo '$jack is null'."/n";
}else{
echo '$jack is not null'."/n";
}
}//testJack
testJack();
?>
The result is $jack is not null!
Case 3:
The children.php code is as follows:
require("father.php");
class JackTest{
public function testJack(){
if(!isset($jack)){
echo '$jack is null'."/n";
}else{
echo '$jack is not null'."/n";
}
}//testJack
}
$jackTest = new JackTest();
$jackTest->testJack();
?>
Run result output: $jack is null
This is because $jack of this function in class is a local variable.
If you add global $jack; at the beginning of function testJack, then $jack is not null will be output.
Easier to understand.
Case 4:
Dynamic loading the file name as a parameter, the code is as follows:
$casefile = $_SERVER['argv'][1];
echo $casefile."/n";
require($casefile);
echo $jack."/n";
?>
Run php children.php father.php
The results are as follows:
father.php
1000
That is to say, our dynamic loader ran successfully. .
Case 5:
To combine dynamic loading with class definition:
The directory relationship is as follows:
|- c.php
|- Bfold - b.php
|- Afold - a.class.php (the functions inside refer to ../Bfold/b.php)
That is to say, class a.class is new in c.php, and a function of a.class.php requires b.php under the Bfold folder. This require (../Bfold/b.php ) error, Warning: ……
Because you let the server currently execute the c.php file, when php parses it, the path is relative to c.php. Try changing (../Bfold/b.php) to (Bfold/b .php) and you should see no error.
The following is a program example illustrating the use of require_once (A.php) inside a function.
Understanding of require_once:
Assume that require_once(A.php); is referenced in B.php. .
Then it is actually equivalent to calling the anonymous lambda function A.php to execute. As shown below:
C.php requires B.php in a function call------》
B.php requires A.php in a normal statement--------》
A.php
Now we call php B.php; because B.php uses require in a normal statement to call A.php, then A.php will register its variables that are global variables relative to A to B. php environment. Because B.php is the root calling file, its running environment is the global environment. So the variables in the A.php file can be used normally in B.php.
Now we call php C.php; then C calls B.php using require in the function, and then B calls A. It feels that during this calling process, the root operating environment of B and A is C's. The environment of the calling function, but if the calling function of C wants to use the variables in B and A, there is no way. .
If you use global $a, to reference, then because $a does not belong to the global variable in this case, it cannot be referenced.
If you use $a to reference, then $a will be treated as a local variable and cannot be referenced. . .

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


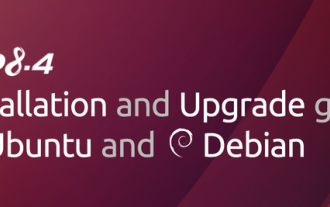
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
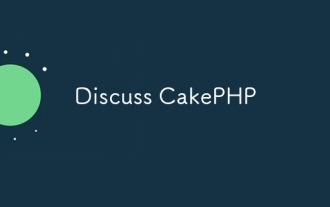
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
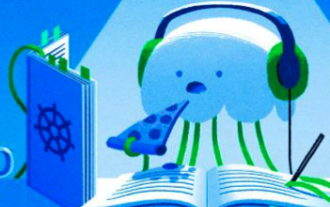
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
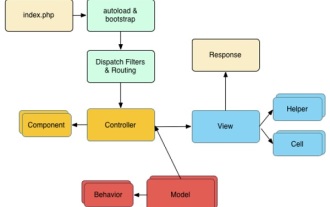
CakePHP is an open source MVC framework. It makes developing, deploying and maintaining applications much easier. CakePHP has a number of libraries to reduce the overload of most common tasks.
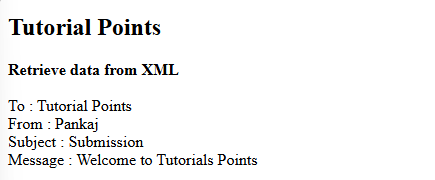
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
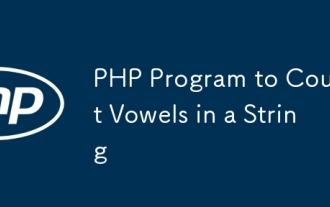
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
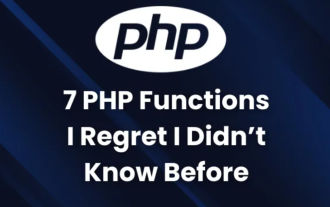
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
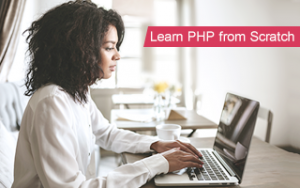
The following resources contain additional information on CakePHP. Please use them to get more in-depth knowledge on this.
