Summary and sharing of common PHP functions_PHP tutorial
Summary sharing of common PHP functions
I will summarize and share with you the commonly used array, string, time, and file operation functions of PHP. I hope it will be helpful to friends who are learning PHP development.
Array:【Key Point 1】
implode (separated, arr) concatenates array value data according to specified characters
For example:
$arr=array('1','2','3','4');
$str=implode('-',$arr);
explode([separate],arr) splits a string according to the specified rules, and the return value is an array. Alias join
array_merge() merges one or more arrays
array_combine(array keys, array values) creates an array, using the value of one array as its key name and the value of another array as its value
For example:
$a = array('green','red','yellow');
$b = array('avocado','apple','banana');
$c = array_combine($a, $b);
print_r($c);
/* Outputs:
Array
(
[green] => avocado
[red] => apple
[yellow] => banana
)
*/
array_push(arr,str) Push one or more cells to the end of the array (push)
array_unique(arr) removes duplicate values in the array
array_search() searches for a given value in the array, and returns the corresponding key name if successful
array_values() returns all values in the array
array_keys() returns all key names in the array
count(arr) counts the number of cells in the array or the number of attributes in the object sizeof()
is_array(arr) checks whether the variable is an array
sort(arr) Sort array
array_flip(arr) swaps keys and values in an array
Note that the value in trans needs to be a legal key name, for example, it needs to be integer or string. A warning will be emitted if the value is of the wrong type, and the key/value pairs in question will not be reversed.
key(arr) returns the key name of the current element in the array
current(arr) returns the value pointed by the current pointer
next returns the value pointed to by the next pointer
For example
The code is as follows:
$array = array (
'fruit1' => 'apple',
'fruit2' => 'orange',
'fruit3' => 'grape',
'fruit4' => 'apple',
'fruit5' => 'apple'
);
// this cycle echoes all associative array
// key where value equals "apple"
while ($fruit_name = current($array)) {
if ($fruit_name == 'apple') {
echo key($array).'
';
}
next($array);
}
The internal pointer of the reset(arr) array points to the first element
array_chunk( array input, int size [, bool preserve_keys])) Split an array into multiple
Split an array into multiple arrays, where the number of cells in each array is determined by size. The last array may have a few fewer elements. The resulting array is a cell in a multidimensional array, with indexes starting from zero.
Setting the optional preserve_keys parameter to TRUE causes PHP to preserve the original key names in the input array. If you specify FALSE, each result array will be indexed with a new number starting from zero. The default value is FALSE.
String [Key Point 2]
trim(str) removes spaces on both sides of a string
rtrim()
addslashes adds
before specifying predefined characters
strlen(str) takes the length of the string
substr(str,start,length) intercepts the characters of the specified length in the specified string
The strstr(str,search) function is used to obtain the substring from the first occurrence of a specified string in another string to the end of the latter. Same as strchr
strpos(str,search) finds the first occurrence of a character in a string
str_replace(search,replace,str) string replacement
ucfirst(str) capitalizes the first character of the string
strtolower becomes lowercase
ucwords(str) capitalizes the first letter of each word in the string
The strcmp(str1,str2) function is used to compare two strings
The substr_count() function retrieves the number of occurrences of a substring
Regular string related functions:
preg_match(pattern,subject,matches) searches the subject string for content that matches the regular expression given by pattern. The matched results are stored in matches. matches[0] matches all content, and matches[1] comes first. A pattern unit matches[1] the second pattern unit, and so on.
preg_match_all(pattern,subject,matches) matches globally, the rest of the preg_match functions are similar
preg_replace(pattern,replacement,str) performs regular expression search and replacement, three types [string,string][array,string][array,array]
preg_split(pattern,str) splits a string using regular expression
preg_grep(pattern,array) matches the value of the array with a regular expression and returns a new array information
Time【Point 3】
PHP date and time function date()
1. Year-month-day
echo date('Y-m-j');
2007-02-6
echo date('y-n-j');
07-2-6
An uppercase Y represents a four-digit year, while a lowercase y represents a two-digit year;
Lowercase m represents the number of the month (with a leading), while lowercase n represents the number of the month without the leading.
echo date('Y-M-j');
2007-Feb-6
echo date('Y-m-d');
2007-02-06
Uppercase M represents the 3 abbreviated characters of the month, while lowercase m represents the number of the month (with leading 0);
There is no uppercase J, only lowercase j represents the day of the month, without the leading o; if a leading month is required, use a lowercase d.
echo date('Y-M-j');
2007-Feb-6
echo date('Y-F-jS');
2007-February-6th
Capital M represents the 3 abbreviated characters of the month, while capital F represents the full English letter of the month. (no lowercase f)
Capital S represents the suffix of the date, such as "st", "nd", "rd" and "th", depending on the date number.
Summary:
You can use uppercase Y or lowercase y to indicate the year;
Months can be represented by uppercase F, uppercase M, lowercase m and lowercase n (two ways to represent characters and numbers respectively);
Lowercase d and lowercase j can be used to represent the day, and uppercase S represents the suffix of the date.
2, hours: minutes: seconds
By default, PHP interprets the displayed time as "Greenwich Mean Time", which is 8 hours different from our local time.
echo date('g:i:s a');
5:56:57 am
echo date('h:i:s A');
05:56:57 AM
A lowercase g indicates a 12-hour clock without leading 0s, while a lowercase h indicates a 12-hour clock with leading 0s.
When using the 12-hour clock, it is necessary to indicate morning and afternoon. Lowercase a represents lowercase "am" and "pm", and uppercase A represents uppercase "AM" and "PM".
echo date('G:i:s');
14:02:26
Capital G represents the hour in 24-hour format, but without leading; use capital H to represent hour in 24-hour format with leading
Summary:
The letter g represents the hour without leading, and the letter h represents the hour with leading;
Lowercase g and h represent the 12-hour format, while uppercase G and H represent the 24-hour format.
3, leap year, week, day
echo date('L');
Whether this year is a leap year: 0
echo date('l');
Today is: Tuesday
echo date('D');
Today is: Tue
Capital L indicates whether this year is a leap year, Boolean value, returns 1 if true, otherwise 0;
The lowercase l represents the full English word for the day of the week (Tuesday);
Instead, use a capital D to represent the 3-character abbreviation of the day of the week (Tue).
echo date('w');
Today’s week: 2
echo date('W');
This week is week 06 of the year
The lowercase w represents the day of the week, and the numeric form represents
Capital W represents the number of weeks in the year
echo date('t');
This month is 28 days
echo date('z');
Today is the 36th day of the year
Lowercase t represents the number of days in the current month
Lowercase z indicates what day of the year today is
4, other
echo date('T');
UTC
A capital T represents the server’s time locale
echo date('I');
0
Capital I means to determine whether the current daylight saving time is, if true, return 1, otherwise 0
echo date('U');
1170769424
A capital U represents the total number of seconds from January 1, 1970 to the present, which is the UNIX timestamp of the Unix time epoch.
echo date('c');
2007-02-06T14:24:43+00:00
Lowercase c represents an ISO8601 date, the date format is YYYY-MM-DD, the letter T is used to separate the date and time, the time format is HH:MM:SS, and the time zone is represented by the offset from Greenwich Mean Time (GMT).
echo date('r');
Tue, 06 Feb 2007 14:25:52 +0000
A lowercase r indicates the RFC822 date.
The small date() function shows the power and charm of PHP. Compare it to ASP, haha.
checkdate($month,$date,$year)
This function returns true if the applied value forms a valid date. For example, for the error date February 31, 2005, this function returns false.
This function can be used to check and validate a date before it is used in calculations or saved in the database.
The code is as follows:
// returns false
echo checkdate(2,30,2005) ? "valid" : "invalid";
// returns true
echo checkdate(4,6,2010) ? "valid" : "invalid";
?>
getdate($ts)
Without arguments, this function returns the current date and time as an array. Each element in the array represents a specific component of the date/time value. An optional time stamp argument can be submitted to the function to obtain a date/time value corresponding to the time stamp.
Apply this function to obtain a series of discrete, easily separable date/time values.
The code is as follows:
// get date as associative array
$arr = getdate();
echo "Date is " . $arr['mday'] . " " . $arr['weekday'] . " " . $arr['year'];
echo "Time is " . $arr['hours'] . ":" . $arr['minutes'];
?>
mktime($hour, $minute, $second, $month, $day, $year)
This function does the opposite of getdate(): it generates a UNIX timestamp (the number of seconds elapsed since January 1, 1970 GMT) from a series of date and time values. When no arguments are used, it generates a UNIX time stamp of the current time.
Use this function to obtain the UNIX time stamp of the immediate time. Such timestamps are commonly used in many databases and programming languages.
The code is as follows:
// returns timestamp for 13:15:23 7-Jun-2006
echo mktime(13,15,23,6,7,2006);
?>
date($format, $ts)
This function formats a UNIX time stamp into a human-readable date string. It is the most powerful function in the PHP date/time API and can be used to convert integer time labels into the required string format in a series of correction values.
This function is used when formatting a time or date for display.
The code is as follows:
// format current date
// returns "13-Sep-2005 01:16 PM"
echo date("d-M-Y h:i A", mktime());
?>
strtotime($str)
This function converts a human-readable English date/time string into a UNIX time label.
Apply this function to convert a non-standardized date/time string into a standard, compatible UNIX time stamp.
The code is as follows:
// returns 13-Sep-05
echo date("d-M-y", strtotime("today"));
// returns 14-Sep-05
echo date("d-M-y", strtotime("tomorrow"));
// returns 16-Sep-05
echo date("d-M-y", strtotime("today +3 days"));
?>
strftime($format,$ts)
As defined previously by the setlocale() function, this function formats the UNIX time stamp into a date string appropriate for the current environment.
Use this function to build a date string that is compatible with the current environment.
The code is as follows:
// set locale to France (on Windows)
setlocale(LC_TIME, "fra_fra");
// format month/day names
// as per locale setting
// returns "septembre" and "mardi"
echo strftime("Month: %B ");
echo strftime("Day: %A ");
?>
microtime()
As defined previously by the setlocale() function, this function formats the UNIX time stamp into a date string appropriate for the current environment.
Use this function to build a date string that is compatible with the current environment.
The code is as follows:
// get starting value
$start = microtime();
// run some code
for ($x=0; $x<1000; $x++) {
$null = $x * $x;
}
// get ending value
$end = microtime();
// calculate time taken for code execution
echo "Elapsed time: " . ($end - $start) ." sec";
?>
gmmktime($hour, $minute, $second, $month, $day, $year)
This function generates a UNIX time stamp from a series of date and time values expressed in GMT time. When no arguments are used, it generates a UNIX time stamp of the current GMT time.
Use this function to obtain the UNIX time stamp of the GMT real-time time.
The code is as follows:
// returns timestamp for 12:25:23 9-Jul-2006
echo gmmktime(12,25,23,7,9,2006);
?>
gmdate($format, $ts)
This function formats a UNIX time stamp into a human-readable date string. This date string is expressed in GMT (not local time).
This function is used when expressing time labels in GMT.
The code is as follows:
// format current date into GMT
// returns "13-Sep-2005 08:32 AM"
echo gmdate("d-M-Y h:i A", mktime());
?>
date_default_timezone_set($tz), date_default_timezone_get()
All date/time function calls thereafter set and restore the default time zone.
Note: This function is only available in PHP 5.1+.
This function is a convenient shortcut for setting the time zone for future time operations.
The code is as follows:
// set timezone to UTC
date_default_timezone_set('UTC');
?>
代码如下:
//今天
$today = date("Y-m-d");
//昨天
$yesterday = date("Y-m-d", strtotime(date("Y-m-d"))-86400);
//上周
$lastweek_start = date("Y-m-d H:i:s",mktime(0, 0 , 0,date("m"),date("d")-date("w")+1-7,date("Y")));
$lastweek_end = date("Y-m-d H:i:s",mktime(23,59,59,date("m"),date("d")-date("w")+7-7,date("Y")));
//本周
$thisweek_start = date("Y-m-d H:i:s",mktime(0, 0 , 0,date("m"),date("d")-date("w")+1,date("Y")));
$thisweek_end = date("Y-m-d H:i:s",mktime(23,59,59,date("m"),date("d")-date("w")+7,date("Y")));
//上月
$lastmonth_start = date("Y-m-d H:i:s",mktime(0, 0 , 0,date("m")-1,1,date("Y")));
$lastmonth_end = date("Y-m-d H:i:s",mktime(23,59,59,date("m") ,0,date("Y")));
//本月
$thismonth_start = date("Y-m-d H:i:s",mktime(0, 0 , 0,date("m"),1,date("Y")));
$thismonth_end = date("Y-m-d H:i:s",mktime(23,59,59,date("m"),date("t"),date("Y")));
//本季度未最后一月天数
$getMonthDays = date("t",mktime(0, 0 , 0,date('n')+(date('n')-1)%3,1,date("Y")));
//本季度/
$thisquarter_start = date('Y-m-d H:i:s', mktime(0, 0, 0,date('n')-(date('n')-1)%3,1,date('Y')));
$thisquarter_end = date('Y-m-d H:i:s', mktime(23,59,59,date('n')+(date('n')-1)%3,$getMonthDays,date('Y')));
文件操作【重点4】
file_exists(filename) 文件或目录是否存在
filesize(filename) 取得文件大小
pathinfo(filename) 返回目录名、基本名和扩展名的关联数组
$path_parts = pathinfo("/www/htdocs/www.jnwebseo.com/index.html");
echo $path_parts["dirname"] . "n";
echo $path_parts["basename"] . "n";
echo $path_parts["extension"] . "n";
/www/htdocs/www.jnwebseo.com/index.html
mkdir(dirname) 创建目录
rmdir(dirname) 删除空目录
fopen(filename,模式) 打开文件
fclose(fp) 关闭文件
fwrite(fp,str,length) 写入文件
file_put_contents(filename,content) 把内容存成文件
file_get_contents(filename) 从文件读出内容
fread(fp,length) 读取文件
fgets() 从文件指针中读取一行
fgetc() 从文件指针中读取字符
file()把整个文件读入一个数组中,数组中的每个单元都是文件中相应的一行
readfile() 读入一个文件并写入到输出缓冲
copy(filename1,filename2) 复制文件
unlink(filename) 删除文件
rename(filename1,filename2) 重命名文件或目录
$text = iconv('gbk','utf-8','曾志伟');///将gbk编码转换为utf-8

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
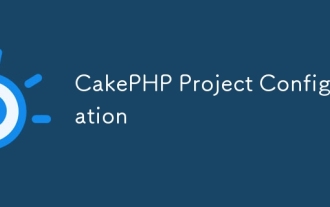
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
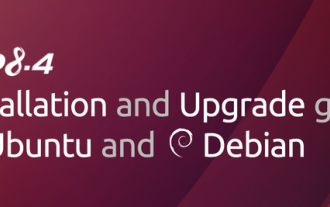
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
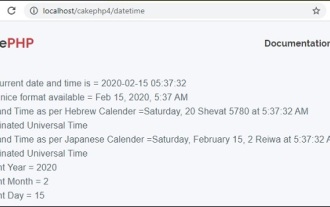
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
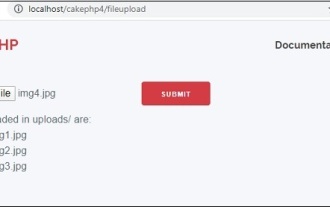
To work on file upload we are going to use the form helper. Here, is an example for file upload.
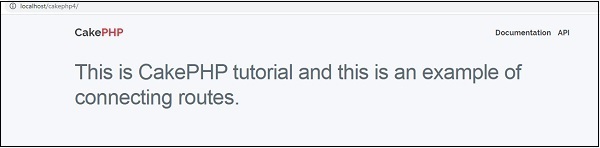
In this chapter, we are going to learn the following topics related to routing ?
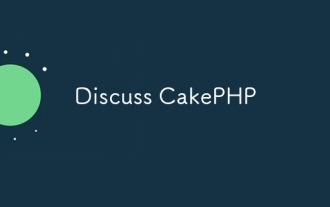
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
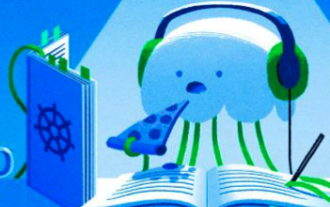
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
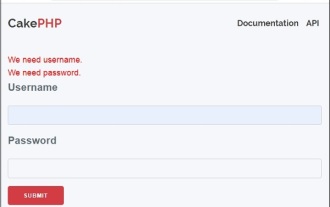
Validator can be created by adding the following two lines in the controller.
