


Comprehensive implementation of PHP sorting algorithm_PHP tutorial
When learning PHP, you may encounter PHP sorting problems. Here we will introduce the solution to PHP sorting problems, and share them with you here. I always have to look at the data structure every three or five times every year, and every time I always feel that I haven't learned a lot of things well, alas.
<ol class="dp-xml"> <li class="alt"><span><span class="tag"><?</SPAN><SPAN> </SPAN></SPAN><LI class=""><SPAN>//插入排序(一维数组) </SPAN><LI class=alt><SPAN>function insert_sort($arr){ </SPAN><LI class=""><SPAN>$</SPAN><SPAN class=attribute>count</SPAN><SPAN class=attribute-value>count</SPAN><SPAN> = count($arr); </SPAN></SPAN><LI class=alt><SPAN>for($</SPAN><SPAN class=attribute>i</SPAN><SPAN>=</SPAN><SPAN class=attribute-value>1</SPAN><SPAN>; $i</SPAN><SPAN class=tag><</SPAN><SPAN>$count; $i++){ </SPAN></SPAN><LI class=""><SPAN>$</SPAN><SPAN class=attribute>tmp</SPAN><SPAN> = $arr[$i]; </SPAN></SPAN><LI class=alt><SPAN>$</SPAN><SPAN class=attribute>j</SPAN><SPAN> = $i - 1; </SPAN></SPAN><LI class=""><SPAN>while($arr[$j] </SPAN><SPAN class=tag>></span><span> $tmp){ </span></span></li> <li class="alt"><span>$arr[$j+1] = $arr[$j]; </span></li> <li class=""><span>$arr[$j] = $tmp; </span></li> <li class="alt"><span>$j--; </span></li> <li class=""><span>} </span></li> <li class="alt"><span>} </span></li> <li class=""><span>return $arr; </span></li> <li class="alt"><span>} </span></li> <li class=""><span> </span></li> <li class="alt"><span> </span></li> <li class=""><span>//选择排序(一维数组) </span></li> <li class="alt"><span>function select_sort($arr){ </span></li> <li class=""> <span>$</span><span class="attribute">count</span><span class="attribute-value">count</span><span> = count($arr); </span> </li> <li class="alt"> <span>for($</span><span class="attribute">i</span><span>=</span><span class="attribute-value">0</span><span>; $i</span><span class="tag"><</SPAN><SPAN>$count; $i++){ </SPAN></SPAN><LI class=""><SPAN>$</SPAN><SPAN class=attribute>k</SPAN><SPAN> = $i; </SPAN></SPAN><LI class=alt><SPAN>for($</SPAN><SPAN class=attribute>j</SPAN><SPAN>=$i+1; $j</SPAN><SPAN class=tag><</SPAN><SPAN>$count; $j++){ </SPAN></SPAN><LI class=""><SPAN>if ($arr[$k] </SPAN><SPAN class=tag>></span><span> $arr[$j]) </span> </li> <li class="alt"> <span>$</span><span class="attribute">k</span><span> = $j; </span> </li> <li class=""><span>if ($k != $i){ </span></li> <li class="alt"> <span>$</span><span class="attribute">tmp</span><span> = $arr[$i]; </span> </li> <li class=""><span>$arr[$i] = $arr[$k]; </span></li> <li class="alt"><span>$arr[$k] = $tmp; </span></li> <li class=""><span>} </span></li> <li class="alt"><span>} </span></li> <li class=""><span>} </span></li> <li class="alt"><span>return $arr; </span></li> <li class=""><span>} </span></li> <li class="alt"><span> </span></li> <li class=""><span>//冒泡排序(一维数组) </span></li> <li class="alt"><span>function bubble_sort($array){ </span></li> <li class=""> <span>$</span><span class="attribute">count</span><span class="attribute-value">count</span><span> = count($array); </span> </li> <li class="alt"> <span>if ($count </span><span class="tag"><</SPAN><SPAN>= 0) return false; </SPAN></SPAN><LI class=""><SPAN> </SPAN><LI class=alt><SPAN>for($</SPAN><SPAN class=attribute>i</SPAN><SPAN>=</SPAN><SPAN class=attribute-value>0</SPAN><SPAN>; $i</SPAN><SPAN class=tag><</SPAN><SPAN>$count; $i++){ </SPAN></SPAN><LI class=""><SPAN>for($</SPAN><SPAN class=attribute>j</SPAN><SPAN>=$count-1; $j</SPAN><SPAN class=tag>></span><span>$i; $j--){ </span> </li> <li class="alt"> <span>if ($array[$j] </span><span class="tag"><</SPAN><SPAN> $array[$j-1]){ </SPAN></SPAN><LI class=""><SPAN>$</SPAN><SPAN class=attribute>tmp</SPAN><SPAN> = $array[$j]; </SPAN></SPAN><LI class=alt><SPAN>$array[$j] = $array[$j-1]; </SPAN><LI class=""><SPAN>$array[$j-1] = $tmp; </SPAN><LI class=alt><SPAN>} </SPAN><LI class=""><SPAN>} </SPAN><LI class=alt><SPAN>} </SPAN><LI class=""><SPAN>return $array; </SPAN><LI class=alt><SPAN>} </SPAN><LI class=""><SPAN> </SPAN><LI class=alt><SPAN>//快速排序(一维数组) </SPAN><LI class=""><SPAN>function quick_sort($array){ </SPAN><LI class=alt><SPAN>if (count($array) </SPAN><SPAN class=tag><</SPAN><SPAN>= 1) return $array; </SPAN></SPAN><LI class=""><SPAN> </SPAN><LI class=alt><SPAN>$</SPAN><SPAN class=attribute>key</SPAN><SPAN> = $array[0]; </SPAN></SPAN><LI class=""><SPAN>$</SPAN><SPAN class=attribute>left_arr</SPAN><SPAN> = </SPAN><SPAN class=attribute-value>array</SPAN><SPAN>(); </SPAN></SPAN><LI class=alt><SPAN>$</SPAN><SPAN class=attribute>right_arr</SPAN><SPAN> = </SPAN><SPAN class=attribute-value>array</SPAN><SPAN>(); </SPAN></SPAN><LI class=""><SPAN>for ($</SPAN><SPAN class=attribute>i</SPAN><SPAN>=</SPAN><SPAN class=attribute-value>1</SPAN><SPAN>; $i</SPAN><SPAN class=tag><</SPAN><SPAN class=tag-name>count</SPAN><SPAN>($array); $i++){ </SPAN></SPAN><LI class=alt><SPAN>if ($array[$i] </SPAN><SPAN class=tag><</SPAN><SPAN>= $key) </SPAN></SPAN><LI class=""><SPAN>$left_arr[] = $array[$i]; </SPAN><LI class=alt><SPAN>else </SPAN><LI class=""><SPAN>$right_arr[] = $array[$i]; </SPAN><LI class=alt><SPAN>} </SPAN><LI class=""><SPAN>$</SPAN><SPAN class=attribute>left_arr</SPAN><SPAN> = </SPAN><SPAN class=attribute-value>quick_sort</SPAN><SPAN>($left_arr); </SPAN></SPAN><LI class=alt><SPAN>$</SPAN><SPAN class=attribute>right_arr</SPAN><SPAN> = </SPAN><SPAN class=attribute-value>quick_sort</SPAN><SPAN>($right_arr); </SPAN></SPAN><LI class=""><SPAN> </SPAN><LI class=alt><SPAN>return array_merge($left_arr, array($key), $right_arr); </SPAN><LI class=""><SPAN>} </SPAN><LI class=alt><SPAN> </SPAN><LI class=""><SPAN></SPAN><SPAN class=tag>?></span><span> </span> </li> </ol>

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
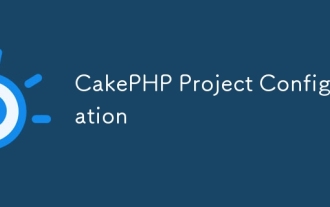
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
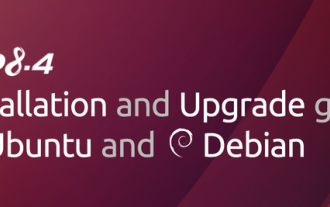
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
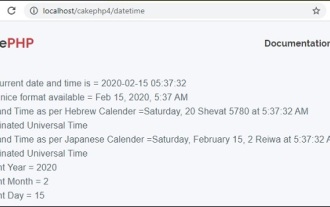
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
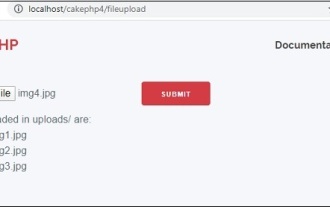
To work on file upload we are going to use the form helper. Here, is an example for file upload.
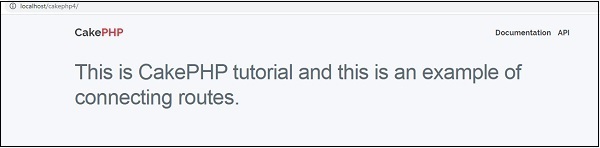
In this chapter, we are going to learn the following topics related to routing ?
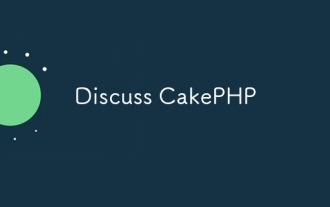
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
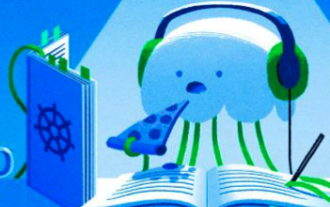
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
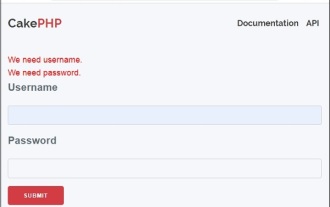
Validator can be created by adding the following two lines in the controller.
