


Detailed interpretation of PHP comparison operators_PHP tutorial
Table 1. PHP comparison operators
Example name result
$a == $b equals TRUE if $a equals $b.
$a === $b is congruent TRUE if $a is equal to $b and they are of the same type. (Introduced in PHP 4)
$a != $b not equal TRUE if $a is not equal to $b.
$a <> $b not equal TRUE if $a is not equal to $b.
$a !== $b Not Congruent TRUE if $a is not equal to $b, or their types are different. (PHP 4 only)
$a < $b is less than TRUE if $a is strictly less than $b.
$a > $b is greater than TRUE if $a is strictly $b.
$a <= $b is less than or equal to TRUE if $a is less than or equal to $b.
$a >= $b is greater than or equal to TRUE if $a is greater than or equal to $b.
If the PHP comparison operator compares an integer and a string, the string will be converted to an integer. If comparing two numeric strings, compare as integers. This rule also applies to switch statements.
<ol class="dp-xml"><li class="alt"><span><span class="tag"><</SPAN><SPAN> ?php </SPAN></SPAN><LI><SPAN>var_dump(</SPAN><SPAN class=attribute>0</SPAN><SPAN> == "a"); // </SPAN><SPAN class=attribute>0</SPAN><SPAN> == 0 -</SPAN><SPAN class=tag>></span><span> true </span><li class="alt"> <span>var_dump("1" == "01"); // </span><span class="attribute">1</span><span> == 1 -</span><span class="tag">></span><span> true </span> </li> <li><span>switch ("a") { </span></li> <li class="alt"><span>case 0: </span></li> <li><span>echo "0"; </span></li> <li class="alt"><span>break; </span></li> <li><span>case "a": // never reached because "a" is already matched with 0 </span></li> <li class="alt"><span>echo "a"; </span></li> <li><span>break; </span></li> <li class="alt"><span>} </span></li> <li> <span class="tag">?></span><span> </span> </li></span></li></ol>
If the operands are of different types, they are compared (in order) according to the following table.
Table 2. PHP comparison operators compare different types
Operator 1 Type Operand 1 Type result
null or string string Convert NULL to "" , perform numerical or lexical comparison
bool or null Any other type is converted to bool, FALSE < TRUE
object object Built-in classes can define their own comparisons, different classes cannot be compared, and attributes of the same class and arrays are compared in the same way ( PHP 4), PHP 5 has its own instructions
string, resource or number string, resource or number Convert strings and resources to numbers, compared by normal math
array array has fewer members The array is smaller. If the key in operand 1 does not exist in operand 2, the array cannot be compared, otherwise the values are compared one by one (see the example below)
array Any other type of array is always larger
object Any other type of object is always larger
Example 1. Standard array comparison code
<OL class=dp-xml><LI class=alt><SPAN><SPAN class=tag><</SPAN><SPAN> ?php </SPAN></SPAN><LI><SPAN>// 数组是用标准比较运算符这样比较的 </SPAN><LI class=alt><SPAN>function standard_array_compare($op1, $op2) </SPAN><LI><SPAN>{ </SPAN><LI class=alt><SPAN>if (count($op1) </SPAN><SPAN class=tag><</SPAN><SPAN> </SPAN><SPAN class=tag-name>count</SPAN><SPAN>($op2)) { </SPAN><LI><SPAN>return -1; // $op1 </SPAN><SPAN class=tag><</SPAN><SPAN> $op2 </SPAN><LI class=alt><SPAN>} elseif (count($op1) </SPAN><SPAN class=tag>><span> count($op2)) { </span></p> <li> <span>return 1; // $op1 </span><span class="tag">></span><span> $op2 </span> </li> <li class="alt"><span>} </span></li> <li> <span>foreach ($op1 as $</span><span class="attribute">key</span><span> =</span><span class="tag">></span><span> $val) { </span> </li> <li class="alt"><span>if (!array_key_exists($key, $op2)) { </span></li> <li><span>return null; // uncomparable </span></li> <li class="alt"> <span>} elseif ($val </span><span class="tag"><</SPAN><SPAN> $op2[$key]) { </SPAN><LI><SPAN>return -1; </SPAN><LI class=alt><SPAN>} elseif ($val </SPAN><SPAN class=tag>></span><span> $op2[$key]) { </span> </li> <li><span>return 1; </span></li> <li class="alt"><span>} </span></li> <li><span>} </span></li> <li class="alt"> <span>return 0; // $</span><span class="attribute">op1</span><span> == $op2 </span> </li> <li><span>} </span></li> <li class="alt"> <span class="tag">?></span><span> </span> </li> <p><strong>PHP comparison operator ternary operator </strong></p> <p>Another conditional operator is the "?:" (or ternary) operator. Example 2. Assign default value </p> <pre class="brush:php;toolbar:false"><ol class="dp-xml"><li class="alt"><span><span class="tag"><</SPAN><SPAN> ?php </SPAN></SPAN><LI><SPAN>// Example usage for: Ternary Operator </SPAN><LI class=alt><SPAN>$</SPAN><SPAN class=attribute>action</SPAN><SPAN> = (empty($_POST['action'])) <br>? 'default' : $_POST['action']; </SPAN><LI><SPAN>// The above is identical to <br>this if/else statement </SPAN><LI class=alt><SPAN>if (empty($_POST['action'])) { </SPAN><LI><SPAN>$</SPAN><SPAN class=attribute>action</SPAN><SPAN> = </SPAN><SPAN class=attribute-value>'default'</SPAN><SPAN>; </SPAN><LI class=alt><SPAN>} else { </SPAN><LI><SPAN>$</SPAN><SPAN class=attribute>action</SPAN><SPAN> = $_POST['action']; </SPAN><LI class=alt><SPAN>} </SPAN><LI><SPAN class=tag>?></span><span> </span></span></li></ol>
Expression (expr1) ? (expr2) : (expr3) When expr1 evaluates to TRUE, the value is expr2, and when expr1 evaluates to FALSE, the value is expr3.
Note: Note that the ternary operator is a statement, so its evaluation is not a variable, but the result of the statement. This is important if you want to return a variable by reference. The statement return $var == 42 ? $a : $b; in a function that returns by reference will not work, and a future version of PHP will issue a warning about this.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


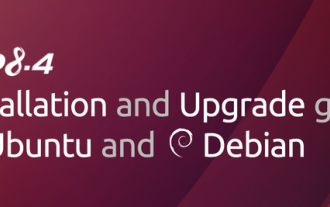
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
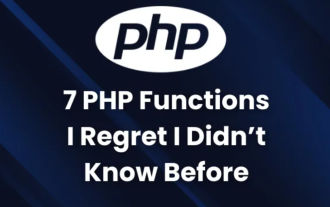
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
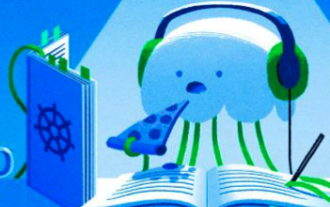
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
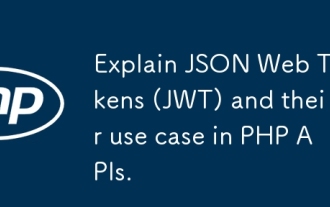
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
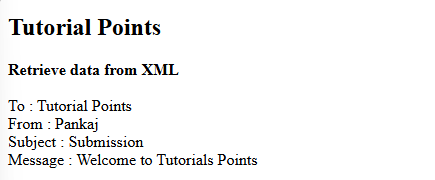
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
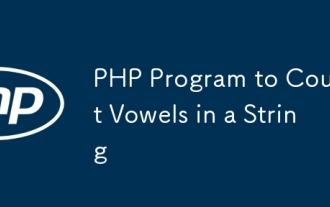
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
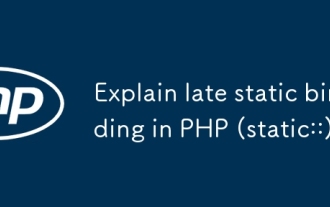
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
