http://blog.csdn.net/morewindows/article/details/7102362
Tips for reading and writing XML files with PHP_PHP tutorial
Commonly used lines are as follows:
header("content-type:text/html; charset=utf-8"); //Specify PHP to use UTF-8 encoding
$xml = simplexml_load_file("example.xml"); //Read xml file
$newxml = $xml->asXML(); //Standardize $xml
$fp = fopen("newxml.xml", "w"); //New xml file
fwrite($fp, $newxml); //Write -------xml file
fclose($fp);
PHP can easily generate and read XML files. PHP mainly completes XML reading and writing operations through DOMDocument, DOMElement and DOMNodeList. Below is a brief explanation of how to use these classes.
1. Generate XML file
For an XML file as follows.
[html]
http://blog.csdn.net/morewindows/article/details/7102362
http://blog.csdn.net/morewindows/article/details/7102362
Let’s see how to generate it using PHP:
First create a new DOMDocument object and set the encoding format.
$dom = newDOMDocument('1.0', 'UTF-8');
$dom->formatOutput= true;
Create the
$rootelement =$dom->createElement("article");
$title =$dom->createElement("title", "PHP Access MySql Database - Elementary");
Then create a node with text content
$link =$dom->createElement("link","http://blog.csdn.net/morewindows/article/details/7102362");
You can also generate a node first and then add text content to it.
$link = $dom->createElement("link");
$linktext =$dom->createTextNode('http://blog.csdn.net/morewindows/article/details/7102362');
$link->appendChild($linktext);
Then add the
$rootelement->appendChild($title);
$rootelement->appendChild($link);
Finally, add the
$dom->appendChild($rootelement);
A complete XML is now generated. Then regenerate the entire XML,
echo $dom->saveXML() ;
saveXML() can also input only part of the XML text. For example, echo $dom->saveXML($link); will only output the node: http://blog.csdn. net/morewindows/article/details/7102362
The following is a complete example of outputting data content to an XML file in PHP. This example will output a PHP array to an XML file.
[php]
//Output the array to an XML file
// by MoreWindows( http://blog.csdn.net/MoreWindows )
$article_array = array(
"First article" => array(
"title"=>"PHP Access MySql Database Beginner",
"link"=>"http://blog.csdn.net/morewindows/article/details/7102362"
),
"Second article" => array(
"title"=>"PHP Access MySql Database Intermediate Smarty Technology",
"link"=>"http://blog.csdn.net/morewindows/article/details/7094642"
),
"Part 3" => array(
"title"=>"PHP Access MySql Database Advanced AJAX Technology",
"link"=>"http://blog.csdn.net/morewindows/article/details/7086524"
),
);
$dom = new DOMDocument('1.0', 'UTF-8');
$dom->formatOutput = true;
$rootelement = $dom->createElement("MoreWindows");
foreach ($article_array as $key=>$value)
{
$article = $dom->createElement("article", $key);
$title = $dom->createElement("title", $value['title']);
$link = $dom->createElement("link", $value['link']);
$article->appendChild($title);
$article->appendChild($link);
$rootelement->appendChild($article);
}
$dom->appendChild($rootelement);
$filename = "D:test.xml";
echo 'XML file size' . $dom->save($filename) . 'Bytes';
?>
//Output the array to an XML file
// by MoreWindows( http://blog.csdn.net/MoreWindows )
$article_array = array(
"First article" => array(
"title"=>"PHP Access MySql Database Beginner",
"link"=>"http://blog.csdn.net/morewindows/article/details/7102362"
),
"Second article" => array(
"title"=>"PHP Access MySql Database Intermediate Smarty Technology",
"link"=>"http://blog.csdn.net/morewindows/article/details/7094642"
),
"Part 3" => array(
"title"=>"PHP Access MySql Database Advanced AJAX Technology",
"link"=>"http://blog.csdn.net/morewindows/article/details/7086524"
),
);
$dom = new DOMDocument('1.0', 'UTF-8');
$dom->formatOutput = true;
$rootelement = $dom->createElement("MoreWindows");
foreach ($article_array as $key=>$value)
{
$article = $dom->createElement("article", $key);
$title = $dom->createElement("title", $value['title']);
$link = $dom->createElement("link", $value['link']);
$article->appendChild($title);
$article->appendChild($link);
$rootelement->appendChild($article);
}
$dom->appendChild($rootelement);
$filename = "D:test.xml";
echo 'XML file size' . $dom->save($filename) . 'Bytes';
?>
Running this PHP will generate the test.xml file on the D drive (Win7 + XAMPP + IE9.0 test passed)
2. Read XML file
Take reading the D:test.xml generated in the previous article as an example:
[php]
//Read XML file
// by MoreWindows( http://blog.csdn.net/MoreWindows )
$filename = "D:test.xml";
$article_array = array();
$dom = new DOMDocument('1.0', 'UTF-8');
$dom->load($filename);
//Get the
$articles = $dom->getElementsByTagName("article");
echo '
foreach ($articles as $article)
{
$id = $article->getElementsByTagName("id")->item(0)->nodeValue;
$title = $article->getElementsByTagName("title")->item(0)->nodeValue;
$link = $article->getElementsByTagName("link")->item(0)->nodeValue;
$article_array[$id] = array('title'=>$title, 'link'=>$link);
}
//Output results
echo ""; <br>
var_dump($article_array); <br>
echo "
";
?>
//Read XML file
// by MoreWindows( http://blog.csdn.net/MoreWindows )
$filename = "D:test.xml";
$article_array = array();
$dom = new DOMDocument('1.0', 'UTF-8');
$dom->load($filename);
//Get
$articles = $dom->getElementsByTagName("article");
echo '
foreach ($articles as $article)
{
$id = $article->getElementsByTagName("id")->item(0)->nodeValue;
$title = $article->getElementsByTagName("title")->item(0)->nodeValue;
$link = $article->getElementsByTagName("link")->item(0)->nodeValue;
$article_array[$id] = array('title'=>$title, 'link'=>$link);
}
//Output results
echo "
";<br> var_dump($article_array);<br> echo "";
?>

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


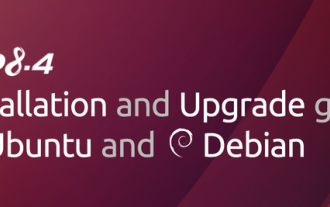
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
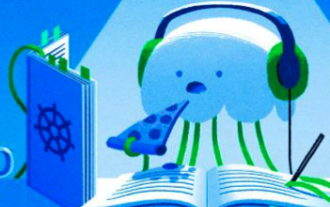
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
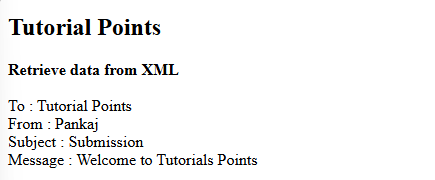
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
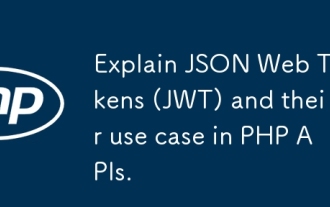
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
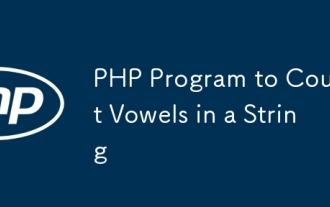
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
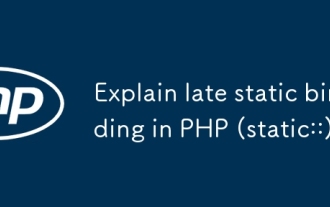
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
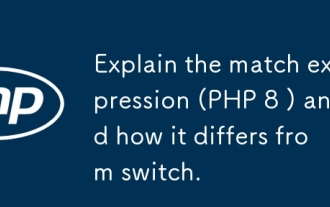
In PHP8, match expressions are a new control structure that returns different results based on the value of the expression. 1) It is similar to a switch statement, but returns a value instead of an execution statement block. 2) The match expression is strictly compared (===), which improves security. 3) It avoids possible break omissions in switch statements and enhances the simplicity and readability of the code.
