


A brief discussion on the use and precautions of variable parameters of custom functions in Python
Variable parameters
Python has two types of variable parameters, one is the list type and the other is the dictionary type. The list type is similar to the variable parameter in C and is defined as
def test_list_param(*args) : for arg in args : print arg
where args is a tuple.
Variable parameters of dictionary type:
def test_dict_param(**args) : for k, v in args.iteritems() : print k, v
where args is a dictionary
You can pass tuple and dictionary to the corresponding variable parameters respectively, the format is as follows
a = (1, 2, 3) b = {"a":1, "b":2, "msg":"hello"} test_list_param(*a) test_dict_param(**b)
Function with default parameters
The parameters with default values of the function can be very convenient for us to use: Under normal circumstances, you can omit the parameter passing and use the default value of the parameter, or you can actively pass the parameter; when calling, you don’t need to care about the order of the parameters for convenience, and Direct and explicit; it can even be used as a magic value to perform some logical control.
But since Python’s default value parameter will only be parsed once at the function definition, every time the function is called thereafter, the default value parameter will be this value. It's okay when you encounter some immutable data types such as integers, strings, ancestors, etc., but if you encounter mutable types of data such as arrays, some unexpected things will happen.
Let’s give a simple example to illustrate:
def add_to(num, target=[]): target.append(num) print id(target), target add_to(1) # Output: 39003656, [1] add_to(2) # Output: 39003656, [1, 2] add_to(3) # Output: 39003656, [1, 2, 3]
Obviously, if you want to get a new array containing the expected results every time you call the function, you will definitely not be able to do so. The parameter target of the function add_to will be assigned to an empty array when the function is parsed for the first time, because it will only be parsed once. Every subsequent call will be based on the target variable and the id value of the variable. Exactly the same. To get the expected results, you can specify a None to represent a null value for this variable data type parameter:
a = (1, 2, 3) b = {"a":1, "b":2, "msg":"hello"} test_list_param(*a) test_dict_param(**b)
In the python world, parameters are passed by identifier (a rough explanation is that they are passed by reference). What you need to worry about is whether the type of the parameter is variable:
>>> def test(param1, param2): ... print id(param1), id(param2) ... param1 += 1 ... param2 += 1 ... print id(param1), id(param2) ... >>> var1 = 1 >>> var2 = 2 >>> print id(var1), id(var2) 36862728 36862704 >>> test(var1, var2) 36862728 36862704 36862704 36862680
For mutable data types, any changes in the local scope of the function will be retained in the data; for immutable data types, any changes will only be reflected in newly generated local variables, as in the above example Readers can compare the effects shown.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
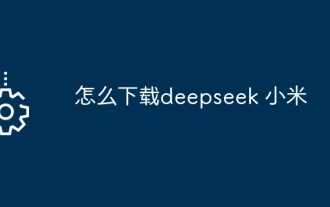
How to download DeepSeek Xiaomi? Search for "DeepSeek" in the Xiaomi App Store. If it is not found, continue to step 2. Identify your needs (search files, data analysis), and find the corresponding tools (such as file managers, data analysis software) that include DeepSeek functions.
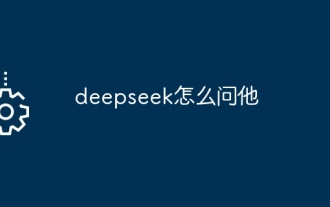
The key to using DeepSeek effectively is to ask questions clearly: express the questions directly and specifically. Provide specific details and background information. For complex inquiries, multiple angles and refute opinions are included. Focus on specific aspects, such as performance bottlenecks in code. Keep a critical thinking about the answers you get and make judgments based on your expertise.
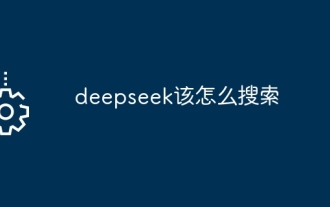
Just use the search function that comes with DeepSeek. Its powerful semantic analysis algorithm can accurately understand the search intention and provide relevant information. However, for searches that are unpopular, latest information or problems that need to be considered, it is necessary to adjust keywords or use more specific descriptions, combine them with other real-time information sources, and understand that DeepSeek is just a tool that requires active, clear and refined search strategies.
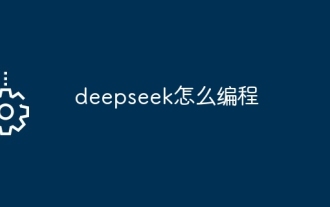
DeepSeek is not a programming language, but a deep search concept. Implementing DeepSeek requires selection based on existing languages. For different application scenarios, it is necessary to choose the appropriate language and algorithms, and combine machine learning technology. Code quality, maintainability, and testing are crucial. Only by choosing the right programming language, algorithms and tools according to your needs and writing high-quality code can DeepSeek be successfully implemented.
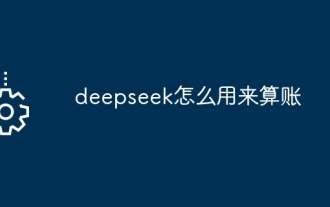
Question: Is DeepSeek available for accounting? Answer: No, it is a data mining and analysis tool that can be used to analyze financial data, but it does not have the accounting record and report generation functions of accounting software. Using DeepSeek to analyze financial data requires writing code to process data with knowledge of data structures, algorithms, and DeepSeek APIs to consider potential problems (e.g. programming knowledge, learning curves, data quality)
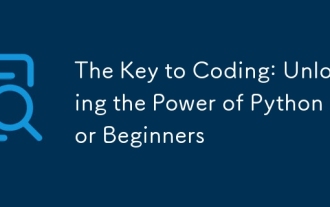
Python is an ideal programming introduction language for beginners through its ease of learning and powerful features. Its basics include: Variables: used to store data (numbers, strings, lists, etc.). Data type: Defines the type of data in the variable (integer, floating point, etc.). Operators: used for mathematical operations and comparisons. Control flow: Control the flow of code execution (conditional statements, loops).
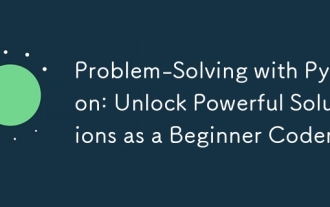
Pythonempowersbeginnersinproblem-solving.Itsuser-friendlysyntax,extensivelibrary,andfeaturessuchasvariables,conditionalstatements,andloopsenableefficientcodedevelopment.Frommanagingdatatocontrollingprogramflowandperformingrepetitivetasks,Pythonprovid
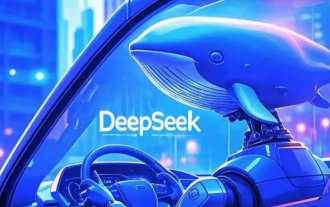
Detailed explanation of DeepSeekAPI access and call: Quick Start Guide This article will guide you in detail how to access and call DeepSeekAPI, helping you easily use powerful AI models. Step 1: Get the API key to access the DeepSeek official website and click on the "Open Platform" in the upper right corner. You will get a certain number of free tokens (used to measure API usage). In the menu on the left, click "APIKeys" and then click "Create APIkey". Name your APIkey (for example, "test") and copy the generated key right away. Be sure to save this key properly, as it will only be displayed once
