In NetBeans, the code prompt function of PHPRedis
Just put the file under phpruntime/.
1.redis.php
<?php class Redis { public function __construct () {} public function connect () {} public function pconnect () {} public function close () {} public function ping () {} /** * 取得键数据 * * @param string $key * @return string 如果 $key 不存在, 返回 (bool)false */ public function get ($key) {} /** * 给一个键设置字符串值 * SET keyname datalength data * (SET bruce 10 paitoubing:保存key为burce, * 字符串长度为10的一个字符串paitoubing到数据库), * data最大不可超过1G。 * * @param string $key * @param string $val * @return bool */ public function set ($key, $val) {} /** * SETNX与SET的区别是: * SET可以创建与更新key的value, * 而SETNX是如果key不存在,则创建key与value数据 * * @param string $key * @param string $val * @return bool */ public function setex ($key, $val) {} public function setnx () {} /** * 读取旧数据, 并新更新新数据. * 注意, 返回的是旧数据! * * @param string $key * @param mix $newValue * @return mix/false 如果没有旧数据, 返回 (bool)false */ public function getSet ($key, $newValue) {} public function randomKey () {} /** * 重命名key的名称, 如果 new key 已经存在, 执行失败. */ public function renameKey () {} public function renameNx () {} public function getMultiple () {} /** * 判断一个键是否存在 * @return bool */ public function exists ($key) {} public function delete () {} /** * 自增函数.具有原子操作,适合用了"计数器" * * @param string $key * @return int 自增后的数值 */ public function incr ($key) {} /** * 令键值自增指定数值 * @param string $key * @param int $int * @return int 自增后的数值 */ public function incrBy ($key, $int) {} /** * 自减键值 * * @param string $key * @return int 自减后的数值 */ public function decr ($key) {} /** * 令键值自减指定数值 * @param string $key * @param int $int * @return int 自减后的数值 */ public function decrBy ($key, $int) {} /** * 返回某个key元素的数据类型 * ( none:不存在,string:字符,list,set,zset,hash) * 可是, 我测试的结果却是 int 0/1 * @param string $key * @return int */ public function type ($key) {} public function append () {} public function getRange () {} public function setRange () {} public function getBit () {} public function setBit () {} public function strlen () {} public function getKeys () {} public function sort () {} public function sortAsc () {} public function sortAscAlpha () {} public function sortDesc () {} public function sortDescAlpha () {} public function lPush () {} public function rPush () {} public function lPushx () {} public function rPushx () {} public function lPop () {} public function rPop () {} public function blPop () {} public function brPop () {} public function lSize () {} public function lRemove () {} public function listTrim () {} public function lGet () {} public function lGetRange () {} public function lSet () {} public function lInsert () {} public function sAdd () {} public function sSize () {} public function sRemove () {} public function sMove () {} public function sPop () {} public function sRandMember () {} public function sContains () {} public function sMembers () {} public function sInter () {} public function sInterStore () {} public function sUnion () {} public function sUnionStore () {} public function sDiff () {} public function sDiffStore () {} public function setTimeout () {} public function save () {} public function bgSave () {} public function lastSave () {} public function flushDB () {} public function flushAll () {} /** * 返回当前数据库的key的总数 * * @return int */ public function dbSize () {} public function auth () {} /** * 查找某个键还有多长时间过期,返回时间秒 * 永不过期, 返回 -1; * * @param string $key * @return int */ public function ttl ($key) {} public function persist () {} /** * 返回 Redis 当前的状态. * 包括版本号,CPU占用, 内存占用等等... * @return array */ public function info () {} /** * 选择,切换到另一个数据库 * @param int $dbID */ public function select ($dbID=0) {} /** * 把一个键移动到另一个库中. */ public function move () {} public function bgrewriteaof () {} public function slaveof () {} public function mset () {} public function msetnx () {} public function rpoplpush () {} public function zAdd () {} public function zDelete () {} public function zRange () {} public function zReverseRange () {} public function zRangeByScore () {} public function zRevRangeByScore () {} public function zCount () {} public function zDeleteRangeByScore () {} public function zDeleteRangeByRank () {} public function zCard () {} public function zScore () {} public function zRank () {} public function zRevRank () {} public function zInter () {} public function zUnion () {} public function zIncrBy () {} public function expireAt () {} public function hGet () {} public function hSet () {} public function hSetNx () {} public function hDel () {} public function hLen () {} public function hKeys () {} public function hVals () {} public function hGetAll () {} public function hExists () {} public function hIncrBy () {} public function hMset () {} public function hMget () {} public function multi () {} public function discard () {} public function exec () {} public function pipeline () {} public function watch () {} public function unwatch () {} public function publish () {} public function subscribe () {} public function unsubscribe () {} public function getOption () {} public function setOption () {} public function open () {} public function popen () {} /** * 返回一个 List 的长度 * * @param string $key * @return 返回一个 List 的长度 */ public function lLen ($key) {} public function sGetMembers () {} public function mget () {} /** * 设置某个key的过期时间(秒), * (EXPIRE bruce 1000:设置bruce这个key1000秒后系统自动删除) * * @param string $key * @param int $ttl */ public function expire ($key,$ttl=-1) {} public function zunionstore () {} public function zinterstore () {} public function zRemove () {} public function zRem () {} public function zRemoveRangeByScore () {} public function zRemRangeByScore () {} public function zRemRangeByRank () {} public function zSize () {} /** * 截取一个键的子串 * * @param string $key 键名. * @param integer $start 开始位置 * @param integer $end 结束位置 */ public function substr (string $key, integer $start, integer $end) {} public function rename () {} /** * 删除一个键 * * @param string $key */ public function del (string $key) {} /** * 返回匹配的key列表 * foo*:查找foo开头的keys, 也支持 *ad*cd* 这样的方式 * @param string $pattern * @return array */ public function keys (string $pattern) {} public function lrem () {} public function ltrim () {} public function lindex () {} public function lrange () {} public function scard () {} public function srem () {} public function sismember () {} public function zrevrange () {} }

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
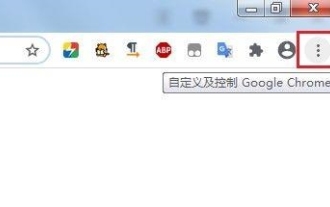
What should I do if Google Chrome prompts that the content of this tab is being shared? When we use Google Chrome to open a new tab, we sometimes encounter a prompt that the content of this tab is being shared. So what is going on? Let this site provide users with a detailed introduction to the problem of Google Chrome prompting that the content of this tab is being shared. Google Chrome prompts that the content of this tab is being shared. Solution: 1. Open Google Chrome. You can see three dots in the upper right corner of the browser "Customize and control Google Chrome". Click the icon with the mouse to change the icon. 2. After clicking, the menu window of Google Chrome will pop up below, and the mouse will move to "More Tools"
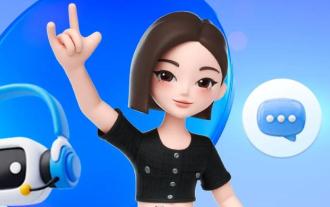
There will be many AI creation functions in the Doubao app, so what functions does the Doubao app have? Users can use this software to create paintings, chat with AI, generate articles for users, help everyone search for songs, etc. This function introduction of the Doubao app can tell you the specific operation method. The specific content is below, so take a look! What functions does the Doubao app have? Answer: You can draw, chat, write articles, and find songs. Function introduction: 1. Question query: You can use AI to find answers to questions faster, and you can ask any kind of questions. 2. Picture generation: AI can be used to create different pictures for everyone. You only need to tell everyone the general requirements. 3. AI chat: can create an AI that can chat for users,
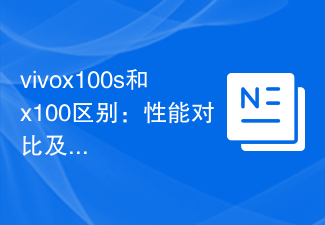
Both vivox100s and x100 mobile phones are representative models in vivo's mobile phone product line. They respectively represent vivo's high-end technology level in different time periods. Therefore, the two mobile phones have certain differences in design, performance and functions. This article will conduct a detailed comparison between these two mobile phones in terms of performance comparison and function analysis to help consumers better choose the mobile phone that suits them. First, let’s look at the performance comparison between vivox100s and x100. vivox100s is equipped with the latest
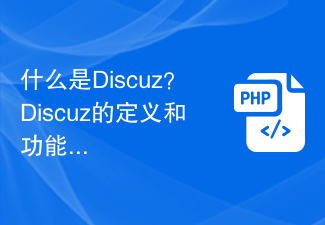
"Exploring Discuz: Definition, Functions and Code Examples" With the rapid development of the Internet, community forums have become an important platform for people to obtain information and exchange opinions. Among the many community forum systems, Discuz, as a well-known open source forum software in China, is favored by the majority of website developers and administrators. So, what is Discuz? What functions does it have, and how can it help our website? This article will introduce Discuz in detail and attach specific code examples to help readers learn more about it.
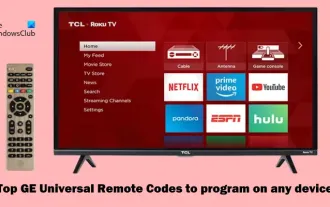
If you need to program any device remotely, this article will help you. We will share the top GE universal remote codes for programming any device. What is a GE remote control? GEUniversalRemote is a remote control that can be used to control multiple devices such as smart TVs, LG, Vizio, Sony, Blu-ray, DVD, DVR, Roku, AppleTV, streaming media players and more. GEUniversal remote controls come in various models with different features and functions. GEUniversalRemote can control up to four devices. Top Universal Remote Codes to Program on Any Device GE remotes come with a set of codes that allow them to work with different devices. you may
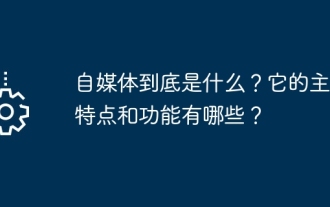
With the rapid development of the Internet, the concept of self-media has become deeply rooted in people's hearts. So, what exactly is self-media? What are its main features and functions? Next, we will explore these issues one by one. 1. What exactly is self-media? We-media, as the name suggests, means you are the media. It refers to an information carrier through which individuals or teams can independently create, edit, publish and disseminate content through the Internet platform. Different from traditional media, such as newspapers, television, radio, etc., self-media is more interactive and personalized, allowing everyone to become a producer and disseminator of information. 2. What are the main features and functions of self-media? 1. Low threshold: The rise of self-media has lowered the threshold for entering the media industry. Cumbersome equipment and professional teams are no longer needed.
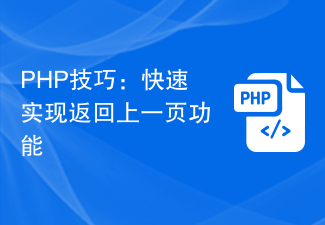
PHP Tips: Quickly implement the function of returning to the previous page. In web development, we often encounter the need to implement the function of returning to the previous page. Such operations can improve the user experience and make it easier for users to navigate between web pages. In PHP, we can achieve this function through some simple code. This article will introduce how to quickly implement the function of returning to the previous page and provide specific PHP code examples. In PHP, we can use $_SERVER['HTTP_REFERER'] to get the URL of the previous page
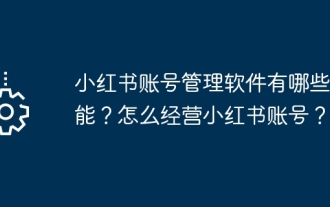
As Xiaohongshu becomes popular among young people, more and more people are beginning to use this platform to share various aspects of their experiences and life insights. How to effectively manage multiple Xiaohongshu accounts has become a key issue. In this article, we will discuss some of the features of Xiaohongshu account management software and explore how to better manage your Xiaohongshu account. As social media grows, many people find themselves needing to manage multiple social accounts. This is also a challenge for Xiaohongshu users. Some Xiaohongshu account management software can help users manage multiple accounts more easily, including automatic content publishing, scheduled publishing, data analysis and other functions. Through these tools, users can manage their accounts more efficiently and increase their account exposure and attention. In addition, Xiaohongshu account management software has
