


Complete collection of PHP image processing functions (recommended collection)_PHP tutorial
1. Create image resources
imagecreatetruecolor(width,height);
imagecreatefromgif(image name);
imagecreatefrompng(image name);
imagecreatefromjpeg(image name) ;Draw various images imagegif (image resource, save path);
imagepng()
imagejpeg();
2. Get image attributes
imagesx(res//width
imagesy(res//height
getimagesize(file path)
Returns a four-unit Array. Index 0 contains the pixel value of the image width, index 2 contains the pixel value of the image type: 1 = GIF, 2 = JPG, 3 = PNG, 4 = SWF, 5 = PSD, 6 = BMP, 7 = TIFF (intel byte order), 8 = TIFF (motorola byte order), 9 = JPC, 10 = JP2, 11 = JPX, 12 = JB2, 13 = SWC, 14 = IFF, 15 = WBMP, 16 = XBM. These tags correspond to the new IMAGETYPE constant in PHP 4.3.0. Index 3 is a text string with the content "height="yyy" width="xxx"" and can be used directly to destroy the image. Resource
imagedestroy(picture resource);
3. Transparency processing
PNG and jpeg transparent colors are normal, only gif is abnormalimagecolortransparent(resource image [,int color])//Set a certain color to be transparent Color
imagecolorstotal()
imagecolorforindex();
4. Image cropping
imagecopyresized()imagecopyresampled();
5. Add watermark (text, picture)
String encoding conversion string iconv ( string $in_charset , string $out_charset , string $str )
6. Image rotation
imagerotate();//Flip the image at a specified angle
7. Flip of the picture
Flip along the X-axis along the Y-axis
8. Sharpening
imagecolorsforindex()imagecolorat()
Draw graphics on the picture $img=imagecreatefromgif("./images/map.gif");
* Image sharpening
*/
$ red= imagecolorallocate($img, 255, 0, 0);
imageline($img, 0, 0, 100, 100, $red);
imageellipse($img, 200, 100, 100 , 100, $red);
imagegif($img, "./images/map2.gif");
imagedestroy($img);
Normal image scaling
The code is as follows:
$filename="./images/hee.jpg";
$per=0.3;
list($width, $height)=getimagesize($filename);
$n_w=$width *$per;
$n_h=$width*$per;
$new=imagecreatetruecolor($n_w, $n_h);
$img=imagecreatefromjpeg($filename);
//Copy part Image and adjust
imagecopyresized($new, $img,0, 0,0, 0,$n_w, $n_h, $width, $height);
//Image output new image, save as
imagejpeg($new, "./images/hee2.jpg");
imagedestroy($new);
imagedestroy($img);
The image is scaled proportionally and the transparent color is not processed
The code is as follows:
function thumn($background, $width, $height, $newfile) {
list($s_w, $s_h)=getimagesize($background);//Get the original image height and width
if ($width && ($s_w < $s_h)) {
$width = ($height / $s_h) * $s_w;
} else {
$height = ($width / $s_w) * $s_h;
}
$new=imagecreatetruecolor($width, $height);
$img=imagecreatefromjpeg($background);
imagecopyresampled($new, $img, 0, 0, 0, 0, $width, $height, $s_w, $s_h);
imagejpeg($new, $newfile);
imagedestroy($new);
imagedestroy($img) ;
}
thumn("images/hee.jpg", 200, 200, "./images/hee3.jpg");
GIF transparent color processing
The code is as follows:
function thumn($background, $width, $height, $newfile) {
list($s_w, $s_h)=getimagesize($background);
if ($width && ($s_w < $ s_h)) {
$width = ($height / $s_h) * $s_w;
} else {
$height = ($width / $s_w) * $s_h;
}
$new=imagecreatetruecolor($width, $height);
$img=imagecreatefromgif($background);
$otsc=imagecolortransparent($img);
if($otsc >=0 && $ otst < imagecolorstotal($img)){//Judge index color
$tran=imagecolorsforindex($img, $otsc);//Index color value
$newt=imagecolorallocate($new, $tran[" red"], $tran["green"], $tran["blue"]);
imagefill($new, 0, 0, $newt);
imagecolortransparent($new, $newt);
}
imagecopyresized($new, $img, 0, 0, 0, 0, $width, $height, $s_w, $s_h);
imagegif($new, $newfile);
imagedestroy($new);
imagedestroy($img);
}
thumn("images/map.gif", 200, 200, "./images/map3.gif");
/**
* Image cropping
* edit by www.jbxue.com
*/
function cut($background, $cut_x, $cut_y, $cut_width, $cut_height, $location){
$back=imagecreatefromjpeg($background);
$new=imagecreatetruecolor($cut_width, $cut_height);
imagecopyresampled($new, $back, 0, 0, $cut_x, $cut_y, $cut_width, $cut_height,$cut_width,$cut_height);
imagejpeg($new, $location);
imagedestroy($new);
imagedestroy($back);
}
cut("./images/hee.jpg", 440, 140, 117, 112, "./images/hee5.jpg");
?>
图片加水印 文字水印
/**
*
* Add text watermark to pictures
*/
function mark_text($background, $text, $x, $y){
$back=imagecreatefromjpeg($background);
$color=imagecolorallocate($back, 0, 255, 0);
imagettftext($back, 20, 0, $x, $y, $color, "simkai.ttf", $text);
imagejpeg($back, "./images/hee7.jpg");
imagedestroy($back);
}
mark_text("./images/hee.jpg", "细说PHP", 150, 250);
//图片水印
function mark_pic($background, $waterpic, $x, $y){
$back=imagecreatefromjpeg($background);
$water=imagecreatefromgif($waterpic);
$w_w=imagesx($water);
$w_h=imagesy($water);
imagecopy($back, $water, $x, $y, 0, 0, $w_w, $w_h);
imagejpeg($back,"./images/hee8.jpg");
imagedestroy($back);
imagedestroy($water);
}
mark_pic("./images/hee.jpg", "./images/gaolf.gif", 50, 200);
图片旋转
/**
* Image rotation
*/
$back=imagecreatefromjpeg("./images/hee.jpg");
$new=imagerotate($back, 45, 0);
imagejpeg($new, "./images/hee9.jpg");
?>
图片水平翻转垂直翻转
/**
* Flip the image horizontally and vertically
*/
function turn_y($background, $newfile){
$back=imagecreatefromjpeg($background);
$width=imagesx($back);
$height=imagesy($back);
$new=imagecreatetruecolor($width, $height);
for($x=0; $x < $width; $x++){
imagecopy($new, $back, $width-$x-1, 0, $x, 0, 1, $height);
}
imagejpeg($new, $newfile);
imagedestroy($back);
imagedestroy($new);
}
function turn_x($background, $newfile){
$back=imagecreatefromjpeg($background);
$width=imagesx($back);
$height=imagesy($back);
$new=imagecreatetruecolor($width, $height);
for($y=0; $y < $height; $y++){
imagecopy($new, $back,0, $height-$y-1, 0, $y, $width, 1);
}
imagejpeg($new, $newfile);
imagedestroy($back);
imagedestroy($new);
}
turn_y("./images/hee.jpg", "./images/hee11.jpg");
turn_x("./images/hee.jpg", "./images/hee12.jpg");
?>

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
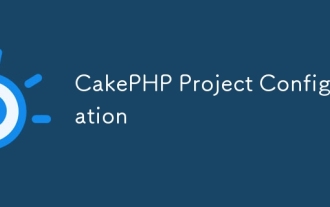
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
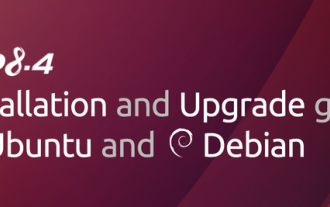
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
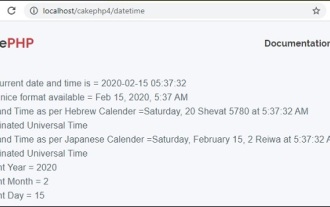
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
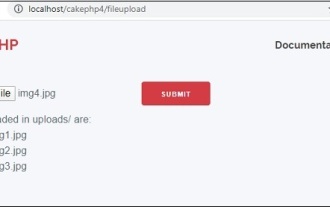
To work on file upload we are going to use the form helper. Here, is an example for file upload.
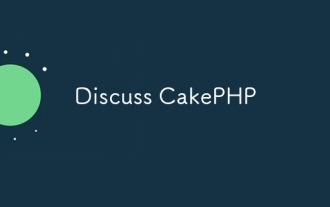
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
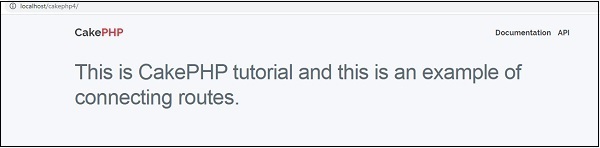
In this chapter, we are going to learn the following topics related to routing ?
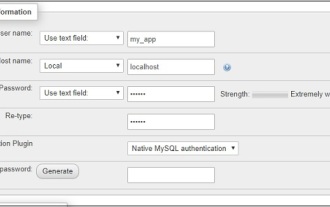
Working with database in CakePHP is very easy. We will understand the CRUD (Create, Read, Update, Delete) operations in this chapter.
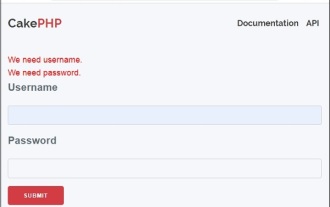
Validator can be created by adding the following two lines in the controller.
