


Detailed explanation of form validation based on PHP+Ajax_PHP tutorial
First, use keyboard response to verify whether the form input is legal without refreshing the page
Users trigger response events through onkeydown and onkeyup events. The usage method is similar to the onclick event. onkeydown means that it is triggered when a key on the keyboard is pressed, and onkeyup is just the opposite, triggering when a key on the keyboard is pressed and then lifted.
Two commonly used calling methods:
(1) Add the event to the page element. When the user completes inputting the information and clicks any key, the onkeydown event is triggered and the refer() function is called.
This method is the simplest and most direct. The format is as follows:
(2) Loaded through window.onload, when the page is loaded, the event is loaded. When the user enters information, this event will be triggered every time a letter is entered. In the function called by the event, the user's input information is judged.
window.onload = function(){
$('regname ').onkeydown = function (){
name = $('regname').value;
}
}
Using the onkeydown event can also achieve specific key Control, including the
2. Registration information verification
General function, returns the triggered id element object
function $(id){
return document.getElementById(id);
}
window.onload event, indicating the current window Fired when loaded. function(){...} represents the operation to be performed when the current page is loaded.
window.onload = function(){
...
}
function() function analysis;
First position the focus on User name text box to facilitate user operations. Next, 5 variables are declared, which represent the results of the 5 data to be detected. When the test data is qualified, set the variable value to "yes".
$( 'regname').focus();
var cname1,cname2,cpwd1,cpwd2; //Five variables are declared, indicating the five items of data to be detected. The chkreg() function is called every time a keyboard event is triggered. , this function determines the values of 5 variables. Only when all variables are "yes", the registration button will be activated.
function chkreg(){
if((cname1 == 'yes') && (cname2 == 'yes') && (cpwd1 == 'yes') && (cpwd2 == 'yes')){
$('regbtn').disabled = false;
}else{
$('regbtn').disabled = true;
}
}
Next, verify the user name. When the user enters the registration name, this function will make a regular judgment on each input of the user, and set different cname1 values based on the results.
$('regname').onkeyup = function (){
name = $('regname').value; //Get the registered name
cname2 = '';
if(name.match(/^[a-zA-Z_]*/) == ''){
$('namediv').innerHTML = 'Must start with a letter or underscore';
cname1 = '';
}else if(name.length <= 3){
$('namediv').innerHTML = 'The registered name must be greater than 3 characters';
cname1 = '';
}else{
$('namediv').innerHTML = 'The registered name meets the standards';
cname1 = 'yes';
}
chkreg(); //Call the chkreg() function to determine whether the five variables are correct
}
When the username text box loses focus, that is, when the user completes input and moves to other elements on the page, it will detect whether the username is repeated. The user name judgment uses Ajax technology to call chkname.php (the user name verification code of this page will be posted later) and the judgment result is displayed in the div tag based on the return value of chkname.php.
$('regname').onblur = function(){
name = $('regname').value; //Get the registered name
if(cname1 == 'yes'){ //Only do this step when the format of the user name is qualified
xmlhttp.open('get ','chkname.php?name='+name,true); //open() creates XMLHttpRequest to initialize the connection, and Ajax creates a new request
xmlhttp.onreadystatechange = function(){ //When XMLHttpRequest is specified as asynchronous transmission (false), if any state change occurs, the object will call the function specified by onreadystatechange
if(xmlhttp.readyState == 4){ //XMLHttpRequest processing status, 4 means processing is completed
if(xmlhttp. status == 200){ //HTTP code of the server response, 200 means normal
var msg = xmlhttp.responseText; //Get the content of the response page
if(msg == '1'){ //chkname .php page searches the database, the database does not have the user and returns 1
. > cname2 = 'yes';
}else if(msg == '2'){ //The user exists in the database and returns 0
$('namediv').innerHTML=" Username is occupied! ";
cname2 = '';
}
}
}
}
xmlhttp.send(null);
chkreg(); // Detect whether the registration button is activated
}
}
Verify the password. When verifying the password, in addition to limiting the length of the password, you can also judge the strength of the password.
Copy code
$('pwddiv1').innerHTML = '< ;font color=red>The password length needs to be at least 6 characters';
cpwd1 = '';
}else if(pwd.length >= 6 && pwd.length < 12){
$('pwddiv1').innerHTML = 'The password meets the requirements. Password strength: weak';
cpwd1 = 'yes';
}else if((pwd.match(/^[0-9]*$/)!=null) || ( pwd.match(/^[a-zA-Z]*$/) != null )){
$('pwddiv1').innerHTML = 'The password meets the requirements. Password strength: Medium';
cpwd1 = 'yes';
}else{
$('pwddiv1').innerHTML = 'The password meets the requirements. Password strength: high';
cpwd1 = 'yes';
}
if(pwd2 != '' && pwd != pwd2){
$('pwddiv2') .innerHTML = 'The two passwords are inconsistent!';
cpwd2 = '';
}else if(pwd2 != '' && pwd == pwd2){
$('pwddiv2').innerHTML = 'The password is entered correctly';
cpwd2 = 'yes';
}
chkreg();
}
Judgement of the secondary password is relatively simple, just judge whether the second password input is equal to the first input.
Copy code
$('pwddiv2').innerHTML = 'The two passwords are inconsistent!';
cpwd2 = '';
}else{
$('pwddiv2').innerHTML = ' The password is entered correctly';
cpwd2 = 'yes';
}
chkreg();
}
The above information must be filled in. If the user wants to fill in more detailed information, they can click the "Details Button"
$('morebtn').onclick = function(){
if($('morediv').style.display == ''){
$('morediv' ).style.display = 'none';
}else{
$('morediv').style.display = '';
}
}
E-mail format verification, the input string must contain @ and ., and the positions of these two strings cannot be at the beginning or end or connected together
$('email').onkeyup = function(){
emailreg = /^w+([-+.]w+)*@w+([- .]w+)*.w+([-.]w+)*$/;
$('email').value.match(emailreg);
if($('email').value.match (emailreg) == null){
$('emaildiv').innerHTML = 'wrong email format';
cemail = '';
}else{
$('emaildiv').innerHTML = 'Enter correctly';
cemail = 'yes';
}
chkreg (); 🎜>Copy code
The code is as follows:
session_start();
include_once "conn/conn.php";$reback = '0';$sql = "select * from tb_member where name='".$_GET['name']."'";
}
echo $reback;
?>
4. XMLHttpRequest function initialization
Copy code
The code is as follows:
// JavaScript Document var xmlhttp = false;
if (window.XMLHttpRequest) { //Mozilla, Safari and other browsers xmlhttp = new XMLHttpRequest();}
}
}
http://www.bkjia.com/PHPjc/327864.html
www.bkjia.com
true
http: //www.bkjia.com/PHPjc/327864.html
1. Use keyboard response to verify whether the form input is legal without refreshing the page. The user passes onkeydown and onkeyup event to trigger a response event. Usage methods and onclick event classes...

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
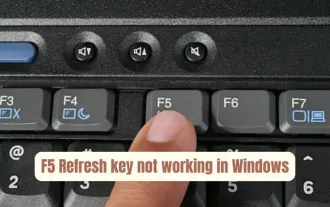
Is the F5 key not working properly on your Windows 11/10 PC? The F5 key is typically used to refresh the desktop or explorer or reload a web page. However, some of our readers have reported that the F5 key is refreshing their computers and not working properly. How to enable F5 refresh in Windows 11? To refresh your Windows PC, just press the F5 key. On some laptops or desktops, you may need to press the Fn+F5 key combination to complete the refresh operation. Why doesn't F5 refresh work? If pressing the F5 key fails to refresh your computer or you are experiencing issues on Windows 11/10, it may be due to the function keys being locked. Other potential causes include the keyboard or F5 key
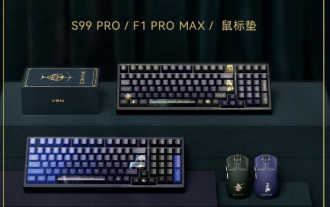
According to news from this site on August 12, VGN launched the co-branded "Elden Ring" keyboard and mouse series on August 6, including keyboards, mice and mouse pads, designed with a customized theme of Lani/Faded One. The current series of products It has been put on JD.com, priced from 99 yuan. The co-branded new product information attached to this site is as follows: VGN丨Elden Law Ring S99PRO Keyboard This keyboard uses a pure aluminum alloy shell, supplemented by a five-layer silencer structure, uses a GASKET leaf spring structure, has a single-key slotted PCB, and the original height PBT material Keycaps, aluminum alloy personalized backplane; supports three-mode connection and SMARTSPEEDX low-latency technology; connected to VHUB, it can manage multiple devices in one stop, starting at 549 yuan. VGN丨Elden French Ring F1PROMAX wireless mouse the mouse
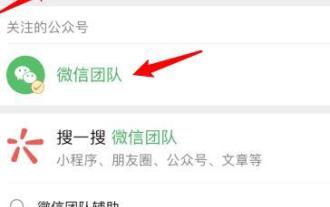
1. After opening WeChat, click the search icon, enter WeChat team, and click the service below to enter. 2. After entering, click the self-service tool option in the lower left corner. 3. After clicking, in the options above, click the option of unblocking/appealing for auxiliary verification.
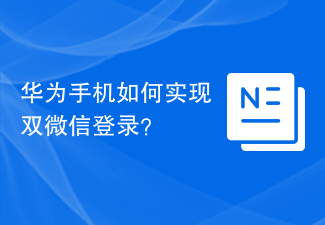
How to implement dual WeChat login on Huawei mobile phones? With the rise of social media, WeChat has become one of the indispensable communication tools in people's daily lives. However, many people may encounter a problem: logging into multiple WeChat accounts at the same time on the same mobile phone. For Huawei mobile phone users, it is not difficult to achieve dual WeChat login. This article will introduce how to achieve dual WeChat login on Huawei mobile phones. First of all, the EMUI system that comes with Huawei mobile phones provides a very convenient function - dual application opening. Through the application dual opening function, users can simultaneously
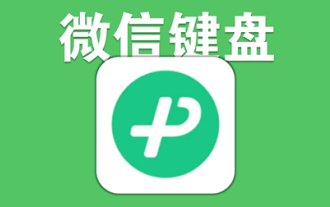
How to set the skin for WeChat keyboard? WeChat Keyboard is a very smart mobile phone input method software. This software has many user-friendly functions. It allows users to choose their own input mode and find the expressions they want as quickly as possible on this software. send out. This software also allows users to change the skin of the keyboard themselves. Many users are not sure how to change the skin. The editor below has compiled the skin changing methods for your reference. How to set the WeChat keyboard skin In WeChat, SMS or other applications that require the use of the keyboard on your phone, you can click the input method settings icon in the upper left corner of the keyboard to enter the settings page to view the function setting options for various input methods. 2. Click "Personalized Skin" on the settings page of the input method.
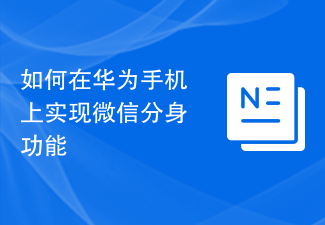
How to implement the WeChat clone function on Huawei mobile phones With the popularity of social software and people's increasing emphasis on privacy and security, the WeChat clone function has gradually become the focus of people's attention. The WeChat clone function can help users log in to multiple WeChat accounts on the same mobile phone at the same time, making it easier to manage and use. It is not difficult to implement the WeChat clone function on Huawei mobile phones. You only need to follow the following steps. Step 1: Make sure that the mobile phone system version and WeChat version meet the requirements. First, make sure that your Huawei mobile phone system version has been updated to the latest version, as well as the WeChat App.
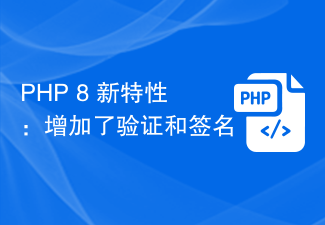
PHP8 is the latest version of PHP, bringing more convenience and functionality to programmers. This version has a special focus on security and performance, and one of the noteworthy new features is the addition of verification and signing capabilities. In this article, we'll take a closer look at these new features and their uses. Verification and signing are very important security concepts in computer science. They are often used to ensure that the data transmitted is complete and authentic. Verification and signatures become even more important when dealing with online transactions and sensitive information because if someone is able to tamper with the data, it could potentially
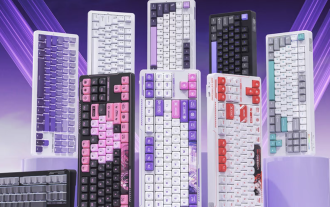
According to news from this website on July 19, Lingbao K87/PRO three-mode mechanical keyboard will be on sale at 8 o'clock tonight. The main differences between the two versions are the shaft/color/battery differences. The standard version is equipped with 4000 mAh. Battery, the PRO version is equipped with an 8000 mAh battery. The price information compiled by this website is as follows: K87 Light Cloud White: 99 yuan K87 Night Purple/Xiaguang Purple/Sunny Mountain Blue: 149 yuan K87 Dust Gray/Star Daisy Purple: 199 yuan K87PRO : 249 yuan According to reports, this keyboard adopts a Gasket structure, uses an 80% arrangement layout, is available in a variety of colors, has built-in lower light RGB lights, and supports three-mode connection 2.4G (1KHz)/wired (1KHz)/Bluetooth (125Hz) ). In terms of specifications, series keyboards are optional
