


Detailed explanation of multi-server sharing of Session data in Memcache_PHP tutorial
A related introduction
1. Introduction to multi-server data sharing of memcache + memcache, please see http://www.guigui8.com/index.php/ archives/206.html
2.session mechanism:
The session mechanism is a server-side mechanism. The server uses a structure similar to a hash table (or may use a hash table) to Save information.
When the program needs to create a session for a client's request, the server first checks whether the client's request already contains a session identifier - called sessionid. If it already contains a sessionid, it means that this client has been used before. If the client has created a session, the server will retrieve the session and use it according to the sessionid (if it cannot be retrieved, it may create a new one). If the client request does not contain the sessionid, a session will be created for the client and a session will be generated related to the session. The associated sessionid. The value of sessionid should be a string that is neither repeated nor easy to find patterns to counterfeit. This sessionid will be returned to the client for storage in this response.
Cookie can be used to save this sessionid, so that during the interaction process, the browser can automatically display this identification to the server according to the rules.
Generally, the name of this cookie is similar to SEEESIONID. For example, for the cookie generated by weblogic, PHPSESSID=ByOK3vjFD75aPnrF3K2HmdnV6QZcEbzWoWiBYEnLerj, its name is PHPSESSID.
Second motivation
In an actual web production environment, an application system often distributes different business applications to different servers for processing.
When tracking current online user information, if it is the same primary domain name, you can use global cookies to handle the sharing of related data; if it is under different primary domains, you can solve the corresponding problem through the central concept of the observer mode. Problems, there are many solutions extended by this concept, and what I am going to talk about today is the former one, which uses memcache's multi-server data sharing technology to simulate sessions to share the current online user data with multiple servers.
Regarding the unified session information of multiple servers, the requirements are as follows:
1. Be able to save session information on several servers specified by memcached (through the memcache introduced earlier Multi-server data sharing);
2. You can customize the value of session_id through session_id ($sessid) before session_start() defined by zend.
3. It is easy to switch between session information stored in memcached and session information stored in files while the system is running.
Three codes
The implementation method is very simple. It uses memcache to simulate the session mechanism. It just uses memcache to replace the storage medium with the memory of a shared server to achieve multiple distribution The purpose of sharing session information among servers deployed in a formal manner. The calling interface is different from the session operation function provided by zend, so you can easily switch between memcache and file session information operations.
The following code has been tested many times and can meet the above functional requirements. Paste it below first:
/**
*=------------------------------------------------ ----------------------------------=
* -------------------------------------------------- --------------------=
*
* Implement the Session function based on Memcache storage
* (Simulate the session mechanism, just use memcache to store The medium is replaced by the memory of the shared server)
*
* Disadvantages: There is currently no implementation strategy for introducing the session sharing mechanism of different main domains. That is, only implementations in the same main domain are supported.
*
* Copyright(c) 2008 by guigui. All rights reserved.
* @author guigui
* @version $Id: MemcacheSession.class.php, v 1.0 2008/12/22 $
* @package systen
* @link http://www.guigui8.com
*/
/**
* class MemcacheSession
*
* 1. Set the client's cookie to save the SessionID
* 2. Save the user's data on the server side, and use the Session Id in the cookie to determine whether a data is User’s
*/
class MemcacheSession
{
// {{{ class members Property definition
public $memObject = null; //memcache operation object handle
private $_sessId = '';
private $_sessKeyPrefix = 'sess_';
private $_sessExpireTime = 86400;
private $_cookieDomain = '.guigui8.com'; //Global cookie domain name
private $_cookieName = '_PROJECT_MEMCACHE_SESS';
private $_cookieExpireTime = '';
private $_memServers = array(' 192.168.0.3' => 11211, '192.168.0.4' => 11211);
private $_sessContainer = array(); //Current user’s session information
private static $_instance = null; // Singleton object of this class
// }}}
/**
* Static method to obtain singleton object.
* (You can provide the server parameters for memcache information storage by the way)
*
* @param string $host - the server ip for memcache data storage
* @param integer $port - the server for memcache data storage Port number
* @param bool $isInit - Whether to start Session when instantiating the object
*/
public static function getInstance($host='', $port=11211, $isInit = true) {
if (null === self::$_instance) {
self::$_instance = new self($host, $port, $isInit);
}
return self::$ _instance;
}
/**
* Constructor
*
* @param bool $isInit - Whether to start Session when instantiating the object
*/
private function __construct($host='', $port=11211, $isInit = false){
!empty( $host) && $this->_memServers = array(trim($host) => $port);
$isInit && $this->start();
}
/**
*=-----------------------------------------------------------------------=
*=-----------------------------------------------------------------------=
* Public Methods
*=-----------------------------------------------------------------------=
*=-----------------------------------------------------------------------=
*/
/**
* Start Session operation
*
* @param int $expireTime - Session expiration time, the default is 0, it will expire when the browser is closed, the value unit is seconds
*/
public function start($expireTime = 0){
$_sessId = $_COOKIE[$this->_cookieName ];
if (!$_sessId){
$this->_sessId = $this->_getId();
$this->_cookieExpireTime = ($expireTime > 0) ? time () + $expireTime : 0;
this->_initMemcacheObj();
$this->_sessContainer = array(); $this-> ;_sessId = $_sessId;
🎜> public function setSessId($sess_id){
$_sessId = trim($sess_id);
if (!$_sessId){
return false;
} else {
$this- & gt; _Sessid = $ _Sessid;
$ This-& GT; _SessContainer = $ This-& GT; _getSession ($ _ SESSID);
public function isRegistered($varName){
if (!isset($this->_sessContainer[$varName])){
return false;
}
return true;
}
/**
* Register a Session variable
*
* @param string $varName - The name of the variable that needs to be registered as Session
* @param mixed $varValue - The value of the variable that needs to be registered as Session
* @ return bool - the variable name already exists and returns false, if the registration is successful, it returns true
*/
public function set($varName, $varValue){
$this->_sessContainer[$varName] = $varValue;
$this->_saveSession();
return true;
}
/**
* Get a registered Session variable value
*
* @param string $varName - the name of the Session variable
* @return mixed - returns false if the variable does not exist, returns the variable value if the variable exists
*/
public function get($varName){
if (!isset($this->_sessContainer[$varName])){
return false;
}
return $this->_sessContainer[$varName];
}
/**
* Destroy a registered Session variable
*
* @param string $varName - the name of the Session variable that needs to be destroyed
* @return bool If the destruction is successful, return true
*/
public function delete($varName){
unset($this->_sessContainer[$varName]);
$this->_saveSession();
return true;
}
/**
* Destroy all registered Session variables
*
* @return true if destroyed successfully
*/
public function destroy(){
$this->_sessContainer = array();
$this->_saveSession();
return true;
}
/**
* Get all Session variables
*
* @return array - Return all registered Session variable values
*/
public function getAll(){
return $this->_sessContainer;
}
/**
* Get the current Session ID
*
* @return string Get the SessionID
*/
public function getSid(){
return $this->_sessId;
}
/**
* Get Memcache server information
*
* @return array Memcache configuration array information
*/
public function getMemServers(){
return $this->_memServers;
}
/**
* Set the Memcache server information
*
* @param string $host - the IP of the Memcache server
* @param int $port - the port of the Memcache server
*/
public function setMemServers($arr){
$this->_memServers = $arr;
}
/**
* Add Memcache server
*
* @param string $host - IP of Memcache server
* @param int $port - Port of Memcache server
*/
public function addMemServer($host, $port){
$this->_memServers[trim($host)] = trim($port);
$this->memObject->addServer($host, $port);
}
/**
* Remove the Memcache server (note that this only removes the configuration and cannot actually remove it from the memcached connection pool)
*
* @param string $host - the IP of the Memcache server
* @param int $port - the port of the Memcache server
*/
public function removeMemServer($host){
unset($this->_memServers[trim($host)]);
}
/**
*=-----------------------------------------------------------------------=
*=-----------------------------------------------------------------------=
* Private Methods
*=-----------------------------------------------------------------------=
*=-----------------------------------------------------------------------=
*/
/**
* Generate a Session ID
*
* @return string Return a 32-bit Session ID
*/
private function _getId(){
return md5(uniqid(microtime()));
}
/**
* Get a Session Key saved in Memcache
*
* @param string $_sessId - whether to specify the Session ID
* @return string Get the Session Key
*/
private function _getSessKey($_sessId = ''){
$sessKey = ($_sessId == '') ? $this->_sessKeyPrefix.$this->_sessId : $this->_sessKeyPrefix.$_sessId;
return $sessKey;
}
/**
* Check whether the path to save Session data exists
*
* @return bool Returns true if successful
*/
private function _initMemcacheObj(){
if (!class_exists('Memcache') || !function_exists('memcache_connect')){
$this->_showMessage('Failed: Memcache extension not install, please from http://pecl.php.net download and install');
}
if ($this->memObject && is_object($this->memObject)){
return true;
}
$this->memObject = new Memcache;
if (!empty($this->_memServers)) {
foreach ($this->_memServers as $_host => $_port) {
$this->memObject->addServer($_host, $_port);
}
}
return true;
}
/**
* Get the data in the Session file
*
* @param string $_sessId - The SessionId that needs to get the Session data
* @return unknown
*/
private function _getSession($_sessId = ''){
$this->_initMemcacheObj();
$sessKey = $this->_getSessKey($_sessId);
$sessData = $this->memObject->get($sessKey);
if (!is_array($sessData) || empty($sessData)){
//this must be $_COOKIE['__SessHandler'] error!
return array();
}
return $sessData;
}
/**
* Save the current Session data to Memcache
*
* @param string $_sessId - Session ID
* @return Return true on success
*/
private function _saveSession($_sessId = ''){
$this->_initMemcacheObj();
$sessKey = $this->_getSessKey($_sessId);
if (empty($this->_sessContainer)){
$ret = @$this->memObject->set($sessKey, $this->_sessContainer, false, $this->_sessExpireTime);
}else{
$ret = @$this->memObject->replace($sessKey, $this->_sessContainer, false, $this->_sessExpireTime);
}
if (!$ret){
_showMessage('Failed: Save sessiont data failed, please check memcache server');
}
return true;
}
/**
* Display prompt message
*
* @param string $strMessage - the message content to be displayed
* @param bool $isFailed - whether it is a failure message, the default is true
*/
private function _showMessage($strMessage, $isFailed = true){
return;
if ($isFailed){
echo ($strMessage);
}
echo $strMessage;
}
Four Applications
1 .Local session storage operates in the same manner as the original session, without any changes. For example:
session_start();
$_SESSION['file_session_info' ]= 'session information saved in local file'; //session saved in local file
2.memcache shared server's session storage
$mem= MemcacheSession::getInstance('192.168.0.4', 11211);
$mem->addMemServer( '192.168.0.4',11211);
$mem->addMemServer('192.168.0.5',11211);
//If the cookie function is not available, set the mapping based on the unique information passed by other parameters for session_id
if(1) {
$sn= '838ece1033bf7c7468e873e79ba2a3ec';
$mem->setSessId($sn);
}
$mem->set('name ','guigui'); //session shared by multiple memcache servers
$mem->set('addr','wuhan'); //session shared by multiple memcache servers
//$ mem->destroy();
3. Get the session information stored locally and in memcache respectively
$addr= $mem->get('addr');
$_MEM_SESSION= $mem->getAll();
echo "
localhost file session:";
var_dump($_SESSION);
echo"
memcache session:";
var_dump($_MEM_SESSION);
//$res = $mem->delete('name');

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
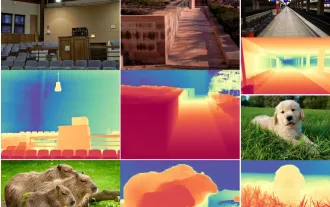
0.What does this article do? We propose DepthFM: a versatile and fast state-of-the-art generative monocular depth estimation model. In addition to traditional depth estimation tasks, DepthFM also demonstrates state-of-the-art capabilities in downstream tasks such as depth inpainting. DepthFM is efficient and can synthesize depth maps within a few inference steps. Let’s read about this work together ~ 1. Paper information title: DepthFM: FastMonocularDepthEstimationwithFlowMatching Author: MingGui, JohannesS.Fischer, UlrichPrestel, PingchuanMa, Dmytr
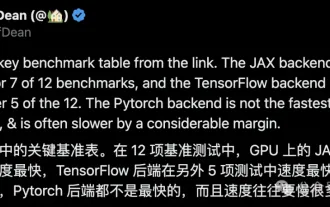
The performance of JAX, promoted by Google, has surpassed that of Pytorch and TensorFlow in recent benchmark tests, ranking first in 7 indicators. And the test was not done on the TPU with the best JAX performance. Although among developers, Pytorch is still more popular than Tensorflow. But in the future, perhaps more large models will be trained and run based on the JAX platform. Models Recently, the Keras team benchmarked three backends (TensorFlow, JAX, PyTorch) with the native PyTorch implementation and Keras2 with TensorFlow. First, they select a set of mainstream
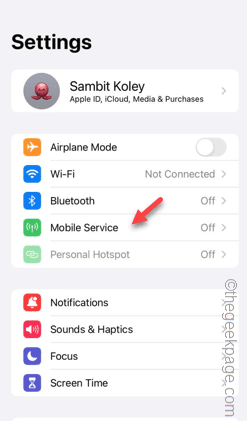
Facing lag, slow mobile data connection on iPhone? Typically, the strength of cellular internet on your phone depends on several factors such as region, cellular network type, roaming type, etc. There are some things you can do to get a faster, more reliable cellular Internet connection. Fix 1 – Force Restart iPhone Sometimes, force restarting your device just resets a lot of things, including the cellular connection. Step 1 – Just press the volume up key once and release. Next, press the Volume Down key and release it again. Step 2 – The next part of the process is to hold the button on the right side. Let the iPhone finish restarting. Enable cellular data and check network speed. Check again Fix 2 – Change data mode While 5G offers better network speeds, it works better when the signal is weaker
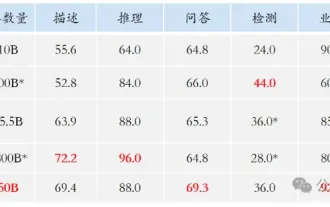
I cry to death. The world is madly building big models. The data on the Internet is not enough. It is not enough at all. The training model looks like "The Hunger Games", and AI researchers around the world are worrying about how to feed these data voracious eaters. This problem is particularly prominent in multi-modal tasks. At a time when nothing could be done, a start-up team from the Department of Renmin University of China used its own new model to become the first in China to make "model-generated data feed itself" a reality. Moreover, it is a two-pronged approach on the understanding side and the generation side. Both sides can generate high-quality, multi-modal new data and provide data feedback to the model itself. What is a model? Awaker 1.0, a large multi-modal model that just appeared on the Zhongguancun Forum. Who is the team? Sophon engine. Founded by Gao Yizhao, a doctoral student at Renmin University’s Hillhouse School of Artificial Intelligence.
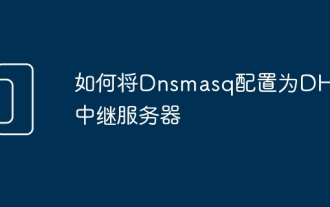
The role of a DHCP relay is to forward received DHCP packets to another DHCP server on the network, even if the two servers are on different subnets. By using a DHCP relay, you can deploy a centralized DHCP server in the network center and use it to dynamically assign IP addresses to all network subnets/VLANs. Dnsmasq is a commonly used DNS and DHCP protocol server that can be configured as a DHCP relay server to help manage dynamic host configurations in the network. In this article, we will show you how to configure dnsmasq as a DHCP relay server. Content Topics: Network Topology Configuring Static IP Addresses on a DHCP Relay D on a Centralized DHCP Server
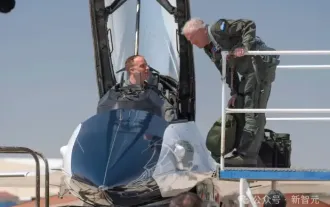
Recently, the military circle has been overwhelmed by the news: US military fighter jets can now complete fully automatic air combat using AI. Yes, just recently, the US military’s AI fighter jet was made public for the first time and the mystery was unveiled. The full name of this fighter is the Variable Stability Simulator Test Aircraft (VISTA). It was personally flown by the Secretary of the US Air Force to simulate a one-on-one air battle. On May 2, U.S. Air Force Secretary Frank Kendall took off in an X-62AVISTA at Edwards Air Force Base. Note that during the one-hour flight, all flight actions were completed autonomously by AI! Kendall said - "For the past few decades, we have been thinking about the unlimited potential of autonomous air-to-air combat, but it has always seemed out of reach." However now,
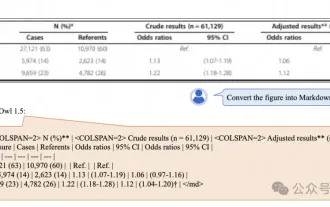
New SOTA for multimodal document understanding capabilities! Alibaba's mPLUG team released the latest open source work mPLUG-DocOwl1.5, which proposed a series of solutions to address the four major challenges of high-resolution image text recognition, general document structure understanding, instruction following, and introduction of external knowledge. Without further ado, let’s look at the effects first. One-click recognition and conversion of charts with complex structures into Markdown format: Charts of different styles are available: More detailed text recognition and positioning can also be easily handled: Detailed explanations of document understanding can also be given: You know, "Document Understanding" is currently An important scenario for the implementation of large language models. There are many products on the market to assist document reading. Some of them mainly use OCR systems for text recognition and cooperate with LLM for text processing.
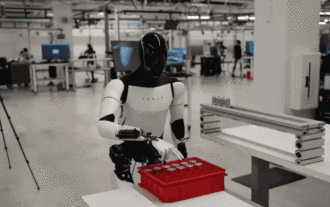
The latest video of Tesla's robot Optimus is released, and it can already work in the factory. At normal speed, it sorts batteries (Tesla's 4680 batteries) like this: The official also released what it looks like at 20x speed - on a small "workstation", picking and picking and picking: This time it is released One of the highlights of the video is that Optimus completes this work in the factory, completely autonomously, without human intervention throughout the process. And from the perspective of Optimus, it can also pick up and place the crooked battery, focusing on automatic error correction: Regarding Optimus's hand, NVIDIA scientist Jim Fan gave a high evaluation: Optimus's hand is the world's five-fingered robot. One of the most dexterous. Its hands are not only tactile
