In-depth explanation of PHP variable storage_PHP tutorial
1.1.1 zval structure
Zend uses the zval structure to store the value of PHP variables. The structure is as follows:
typedef union _zvalue_value {
long lval; /* long value */
double dval; /* double value */
struct {
char *val;
int len;
} str;
HashTable *ht; /* hash table value */
zend_object_value obj;
} zvalue_value;
struct _zval_struct {
/* Variable information */
zvalue_value value; /* value */
zend_uint refcount;
zend_uchar type; /* active type */
zend_uchar is_ref;
};
typedef struct _zval_struct zval;
Zend根据type值来决定访问value的哪个成员,可用值如下:
IS_NULL |
N/A |
IS_LONG |
对应value.lval |
IS_DOUBLE |
对应value.dval |
IS_STRING |
对应value.str |
IS_ARRAY |
对应value.ht |
IS_OBJECT |
对应value.obj |
IS_BOOL |
对应value.lval. |
IS_RESOURCE |
对应value.lval |
According to this table, two interesting things can be found: first, the array in PHP is actually a HashTable, which explains why PHP can support associative arrays; secondly, Resource is a long value, which usually stores a A pointer, the index of an internal array, or something else known only to the creator can be considered a handle.
1.1.2 Reference counting
Reference counting is widely used in garbage collection, memory pools, strings, etc. Zend implements typical reference counting. Multiple PHP variables can share the same zval through the reference counting mechanism. The remaining two members of zval, is_ref and refcount, are used to support this sharing.
Obviously, refcount is used for counting. When the reference is increased or decreased, this value is also incremented and decremented accordingly. Once it decreases to zero, Zend will recycle the zval.
What about is_ref?
1.1.3 zval status
In PHP, there are two types of variables - reference and non-reference, and they are all stored in Zend using reference counting. For non-reference variables, it is required that the variables are independent of each other. When modifying one variable, it cannot affect other variables. This conflict can be solved by using the Copy-On-Write mechanism - when trying to write a variable, Zend will find If the zval pointed to by this variable is shared by multiple variables, a zval with a refcount of 1 will be copied to it, and the refcount of the original zval will be decremented. This process is called "zval separation". However, for reference variables, the requirements are opposite to those for non-reference types. Variables assigned by reference must be bundled. Modifying one variable modifies all bundled variables.
It can be seen that it is necessary to point out the status of the current zval to deal with these two situations respectively. is_ref is for this purpose. It points out whether all the variables currently pointing to the zval are assigned by reference - either all references or Not at all. At this time, another variable is modified. Only when it is found that the is_ref of its zval is 0, that is, it is not a reference, Zend will execute Copy-On-Write.
1.1.4 zval status switching
When all assignment operations on a zval are references or non-references, one is_ref is enough to cope with it. However, the world is not always so beautiful. PHP cannot impose such restrictions on users. When we mix reference and non-reference assignments, special handling must be carried out.
Situation I, look at the following PHP code:
$a = 1;
$b = $a;
$c = $b;
$d = &$c; // In a bunch of non-reference assignments, insert a reference
? >
This code first performs an initialization, which will create a new zval, is_ref=0, refcount=1, and point a to this zval; followed by two non-reference assignments , as mentioned before, just point b and c to the zval of a; the last line is a reference assignment, which requires is_ref to be 1, but Zend found that the zval pointed to by c is not a reference, so it creates a separate zval for c , and at the same time point d to the zval.
Essentially, this can also be seen as a Copy-On-Write, not just value, is_ref is also a protected object.
The whole process is illustrated as follows:
For case II, look at the following PHP code:
$a = 1;
$b = &$a;
$c = &$b;
$d = $c; // Insert a non-reference
into a bunch of reference assignments ?>
The first three sentences of this code will point a, b and c to a zval, whose is_ref=1, refcount=3; the fourth sentence is a non-reference assignment, usually Just increase the reference count. However, the target zval is a reference variable. Simply increasing the reference count is obviously wrong. Zend's solution is to generate a separate copy of zval for d.
The whole process is as follows:
1.1.5 Parameter passing
The passing of PHP function parameters is the same as variable assignment. Non-reference passing is equivalent to non-reference assignment, and reference passing is equivalent to reference assignment, and may also cause Perform zval state switching. This will be mentioned later.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


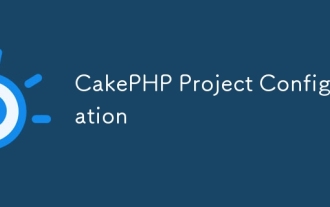
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
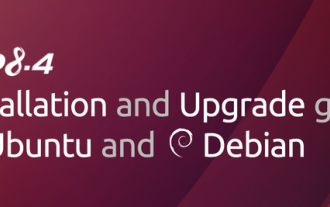
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
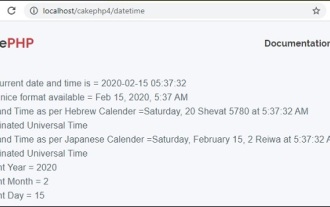
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
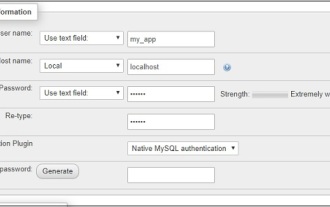
Working with database in CakePHP is very easy. We will understand the CRUD (Create, Read, Update, Delete) operations in this chapter.
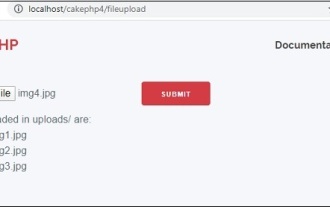
To work on file upload we are going to use the form helper. Here, is an example for file upload.
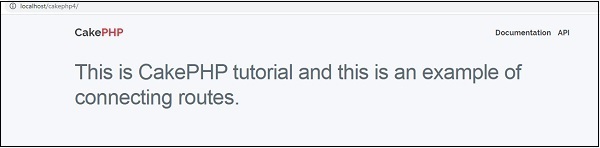
In this chapter, we are going to learn the following topics related to routing ?
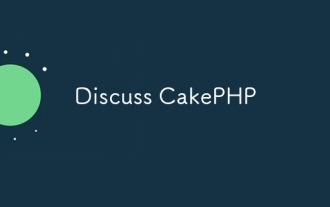
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
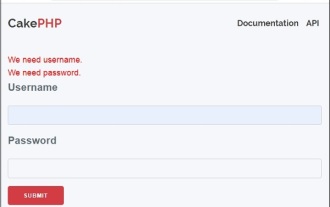
Validator can be created by adding the following two lines in the controller.
