


Use php to send emails with attachments-(Analysis of examples using PHPMailer)_PHP tutorial
/*PHPMailer is a PHP function package for sending emails. The functions it provides include:
*. Specify multiple recipients, CC addresses, BCC addresses and reply addresses when sending emails
*. Supports multiple email encodings including: 8bit, base64, binary and quoted-printable
*. Support SMTP authentication
*. Support redundant SMTP server
*. Support emails with attachments and emails in Html format
*. Customize email headers
*. Support embedding images in emails
*. Flexible debugging
*. Tested and compatible SMTP servers include: Sendmail, qmail, Postfix, Imail, Exchange, etc.
*. Can run on any platform
phpMailer is a very powerful php email class. You can set the sending email address, reply address, email subject, rich text content, upload attachments,...
Official website: http:// phpmailer.worxware.com/
Download address: http://code.google.com/a/apache-extras.org/p/phpmailer/downloads/list
*/
require_once('include/ PHPMailer/class.phpmailer.php'); //Import PHPMAILER class
$mail = new PHPMailer(); //Create instance
$mail -> CharSet='utf-8'; //Set characters Set
$mail -> SetLanguage('ch','include/PHPMailer/language/'); //Set the language type and the directory where the language file is located
$mail -> IsSMTP(); //Use Send via SMTP
$mail -> SMTPAuth = true; //Set whether the server requires SMTP authentication
$mail -> Host = SMTP_SERVER; //SMTP host address
$mail -> Port = SMTP_SERVER_PORT; //SMTP host port
$mail -> From = SMTP_USER_MAIL; //Sender's EMAIL address
$mail -> FromName = 'jasonxu'; //The sender is in the SMTP host Username
$mail -> Username = SMTP_USER_NAME; //Sender’s name
$mail -> Password = SMTP_USER_PASS; //Sender’s password in the SMTP host
$mail -> Subject = 'Test email title'; //Email subject
$mail -> AltBody = 'text/html'; //Set the backup display when the email body does not support HTML
$mail -> Body = 'Content of test email'; //Mail content is made
$mail -> IsHTML(true); //Is it an HTML email
$mail -> AddAddress('chinajason2008# gmail.com','jasonxu'); //Recipient's address and name
$mail -> AddReplyTo('chinajason2008#gmail.com','jasonxu'); //Reply when the recipient replies The given address and name
$mail -> AddAttachment('include/id.csv','att.csv');//The path and name of the attachment
if(!$mail -> Send ()) //Send mail
var_dump($mail -> ErrorInfo); //View the error message sent
Note: If phpmailer adds an attachment, it must be in the attachment name Please specify the attachment suffix. If you do not specify the attachment suffix, the default attachment suffix will be .txt.
For example $mail -> AddAttachment('include/id.csv','att');//The path and name of the attachment
If you add an attachment and send it as above, the attachment you finally receive may It is att.txt.
AddAttachment can set the attachment encoding method and attachment type. For example, the above attachment addition can also be set to
$mail -> AddAttachment('include/id.csv','att.csv',"binary", "text/comma-separated-values");//The path and name of the attachment,
There are probably several encoding methods for attachments: 8bit, base64, binary, and quoted-printable encoding is supported
The MIME Types accepted by CSV
· application/octet-stream
· text/comma-separated-values (recommended)
· text/csv
So, the attachment type of csv format file can It is any one of the above three
An example of email sending in a previous project, compiled into an abbreviated version for easy application:
$body=$_smtp_body;
$mail=new PHPMailer();//Get a PHPMailer instance
//$mail->SMTPSecure='tls';
$mail ->CharSet="utf-8"; //Set encoding
$mail->IsSMTP();//Set to use SMTP to send emails
$mail->Host=$_smtp_server;// Set the address of the SMTP mail server
$mail->Port=$_smtp_port; //Set the port of the mail server, the default is 25
$mail->From=$_smtp_from_mail; //Set the sender's port Email address
$mail->FromName=$_smtp_from_name;//Set the sender’s name
$mail->Username=$_smtp_username;
$mail->Password=$_smtp_password;
$mail->AddAddress("$email","");//Set the recipient's address (parameter 1) and name (parameter 2)
$mail->SMTPAuth=true;//Enable SMTP authentication
$mail->Subject=$_smtp_subject;//Set the title of the email
//$mail->AltBody="text/html";
$mail->Body=$ body;//Email content
$mail->IsHTML(true);//Set whether the content is html type
//$mail->WordWrap=50; 🎜>//$mail->AddReplyTo("samzhang@tencent.com","samzhang"); //Set the address of the reply recipient
$mail->SMTPDebug=0;
if ($mail->Send()){//Send mail
exit 'ok';
}else{
exit 'fail';
}
In addition, the contents of the problems that occurred at that time are organized as follows:
1. Error: Could not connect to SMTP host
Reason 1: The SMTP requests required by the mail system are different, but all allow uppercase letters, and some do not support lowercase letters, such as NetEase and Tencent mailboxes. (As for whether this is the case, I have not tested it. Anyway, it will not affect if it is changed to uppercase)
Solution:
$this- >Mailer ='SMTP'; // Change smtp ->SMTP; that is, it was originally lowercase and now it is uppercase.
}
// Choose the mailer and send through it
switch($this->Mailer) {
case 'sendmail':
return $this->SendmailSend($header, $body) ;
case 'SMTP':// also changes smtp ->SMTP ; that is, it was originally lowercase, but now it is uppercase.
return $this->SmtpSend($header, $body);
case 'mail':
default:
return $this->MailSend($header, $body);
}
Cause: Some virtual hosts or servers have blocked the "fsockopen() function" for security reasons, resulting in failure to send Email
Solution:
Enable fsockopen function
First, remove the two semicolons below in php.ini
;extension=php_sockets.dll
;extension=php_openssl.dll
Replace fsockopen function
You can replace the fsockopen function in the class.smtp.php file with the pfsockopen function
3. Could not instantiate mail function
Reason:
Solution:
$mail->SMTPSecure = ‘tls’; //Just add this sentence
Note: I have never encountered this kind of error, so in the above example, I added comments to this content. If you encounter this kind of error, you can try this sentence.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


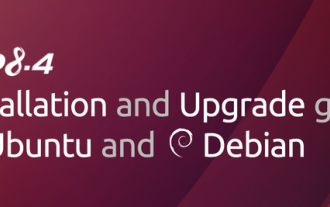
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
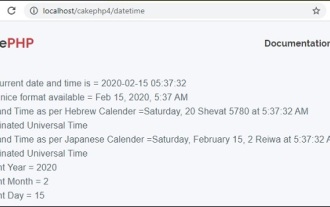
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
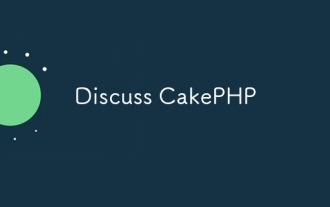
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
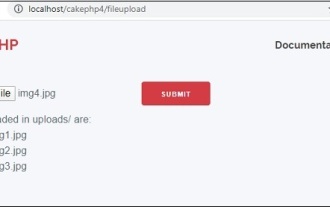
To work on file upload we are going to use the form helper. Here, is an example for file upload.
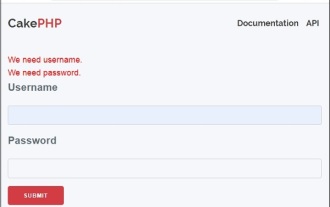
Validator can be created by adding the following two lines in the controller.
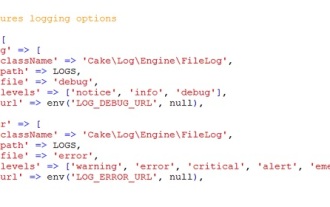
Logging in CakePHP is a very easy task. You just have to use one function. You can log errors, exceptions, user activities, action taken by users, for any background process like cronjob. Logging data in CakePHP is easy. The log() function is provide
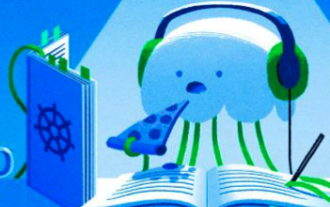
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
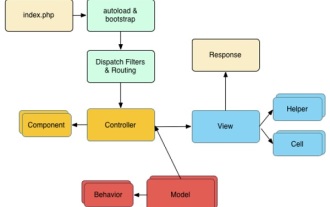
CakePHP is an open source MVC framework. It makes developing, deploying and maintaining applications much easier. CakePHP has a number of libraries to reduce the overload of most common tasks.
