


In-depth understanding of PHP arrays (traversal order) Laruence Original_PHP Tutorial
People often ask me, if a PHP array is accessed using foreach, is the order of traversal fixed? In what order should it be traversed?
For example:
$arr['laruence'] = 'huixinchen';
$arr['yahoo'] = 2007;
$arr['baidu'] = 2008;
foreach ($arr as $key => $val) {
//What is the result?
}
Another example:
$arr[2] = ' huixinchen';
$arr[1] = 2007;
$arr[0] = 2008;
foreach ($arr as $key => $val) {
//Now the result is again What is it?
}
To fully understand this issue, I think you should first understand the internal implementation structure of PHP arrays...
PHP arrays
In PHP, arrays are implemented using a HASH structure (HashTable). PHP uses some mechanisms to enable the addition and deletion of arrays in O(1) time complexity, and supports both linear traversal and random access. .
We have also discussed PHP’s HASH algorithm in previous articles. Based on this, we will make a further extension.
Before getting to know HashTable, let us first take a look at the structure definition of HashTable. I Comments are added for everyone to understand:
typedef struct _hashtable {
uint nTableSize; / * Hash table size, range of Hash values*/
uint nTableMask; /* Equal to nTableSize -1, used for quick positioning*/
uint nNumOfElements; /* The actual number of elements in the HashTable*/
ulong nNextFreeElement; /* Numeric index of the next free available position*/
Bucket *pInternalPointer; /* Internal position pointer, which will be reset, current and these traversal functions use */
Bucket *pListHead; /* Head element , used for linear traversal*/
Bucket *pListTail; /* tail element, used for linear traversal*/
Bucket **arBuckets; /* actual storage container*/
dtor_func_t pDestructor;/* element Destructor (pointer) */
zend_bool persistent;
unsigned char nApplyCount; /* Loop traversal protection*/
zend_bool bApplyProtection;
#if ZEND_DEBUG
int inconsistent;
#endif
} HashTable;
Regarding the meaning of nApplyCount, we can understand it through an example:
$arr = array(1,2,3,4,5,);
$arr[] = &$arr;
var_export($arr); //Fatal error: Nesting level too deep - recursive dependency?
This field is set up to prevent infinite loops caused by circular references.
Looking at the above structure, we can see that for HashTable, the key element is arBuckets, which is the actual storage container. Let us take a look at its structure definition:
typedef struct bucket {
ulong h; /* Numeric index/hash value*/
uint nKeyLength; /* Character index Length*/
void *pData; /* data*/
void *pDataPtr; /* data pointer*/
struct bucket *pListNext; /* next element, for linear traversal*/
struct bucket *pListLast; /* Previous element, used for linear traversal*/
struct bucket *pNext; /* Next element in the same zipper*/
struct bucket *pLast; /* In The previous element in the same zipper*/
char arKey[1]; /* Tips to save memory and facilitate initialization*/
} Bucket;
We noticed that the last element is a flexible array technique, which can save memory and facilitate initialization. Friends who are interested can google flexible array.
h is the Hash value of the element , for numerically indexed elements, h is the direct index value (numeric index represented by nKeyLength=0). For string indexes, the index value is stored in arKey, and the length of the index is stored in nKeyLength.
In the Bucket, the actual data is stored in the memory block pointed to by the pData pointer. Usually this memory block is allocated separately by the system. But there is an exception, that is, when the data saved by the Bucket is a pointer, the HashTable will not request the system to allocate space to save the pointer, but directly save the pointer to pDataPtr, and then point pData to the member of this structure. address. This improves efficiency and reduces memory fragmentation. From this we can see the subtleties of PHP HashTable design. If the data in the Bucket is not a pointer, pDataPtr is NULL (this paragraph comes from Altair's "Zend HashTable Detailed Explanation")
Combined with the above HashTable structure, let's illustrate the overall structure diagram of the HashTable:

The pListhHead of HashTable points to the first element in the linear list form. In the above picture, it is element 1. pListTail points to the last element 0. For each element, pListNext is the next element of the linear structure drawn by the red line. And pListLast is the previous element.
pInternalPointer points to the position of the current internal pointer. When sequentially traversing the array, this pointer indicates the current element.
When linear (sequential) ) when traversing, it will start from pListHead, follow pListNext/pListLast in Bucket, and move pInternalPointer according to the movement of pInternalPointer to achieve a linear traversal of all elements.
For example, for foreach, if we look at the generated opcode sequence, we can find that before foreach, there will first be a FE_RESET to reset the internal pointer of the array, which is pInternalPointer (for foreach, please refer to the in-depth understanding of PHP principles: foreach), and then increment pInternalPointer through each FE_FETCH, thus Implement sequential traversal.
Similarly, when we use the each/next series of functions to traverse, we also implement sequential traversal by moving the internal pointer of the array. There is a problem here, such as:
$arr = array(1,2,3,4,5 );
foreach ($arr as $v) {
//can get
}
while (list($key, $v) = each($arr)) {
//Cannot obtain
}
?>
Understand the knowledge I just introduced, then this problem will be very clear, because foreach will automatically reset, and The while block will not be reset, so after foreach ends, pInternalPointer points to the end of the array, and of course the while statement block cannot access it. The solution is to reset the internal pointer of the array before each.
And in During random access, the head pointer position in the hash array will be determined by the hash value, and then the characteristic element will be found through pNext/pLast.
When adding elements, the elements will be inserted into the same Hash element chain The head and the tail of the linear list. In other words, the elements are traversed according to the order of insertion during linear traversal. This special design makes it so that in PHP, when using numerical indexes, the order of elements is determined by adding is determined by the order, not the index order.
In other words, the order in which arrays are traversed in PHP is related to the order in which elements are added. So, now we clearly know the problem at the beginning of the article The output of >
So, if you want to iterate by index size in a numerically indexed array, then you should use for, not foreach
for($i=0,$l=count($arr); $i<$l; $i++) {
//At this time, it cannot be considered a sequence Traversal (linear traversal)
}
Original text: http://www.laruence.com/2009/08/23/1065.html
true
http: //www.bkjia.com/PHPjc/325561.html
TechArticle
People often ask me, if a PHP array is accessed using foreach, is the order of traversal fixed? In what order should it be traversed? For example: Copy the code as follows: ?php $arr['laruence'] =...

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


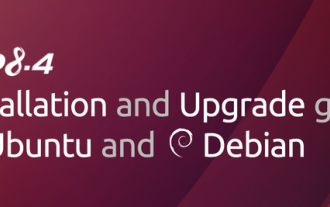
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
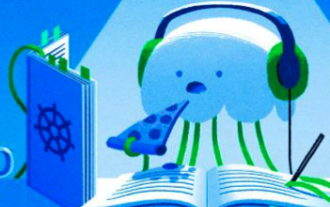
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
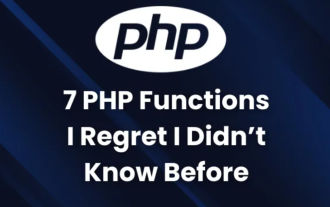
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
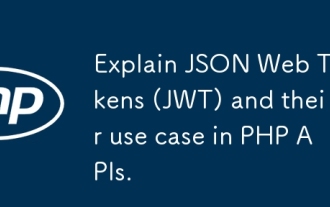
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
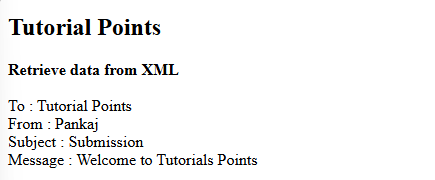
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
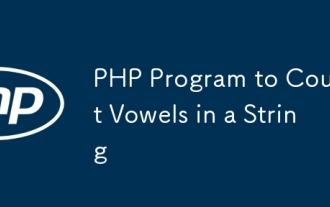
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
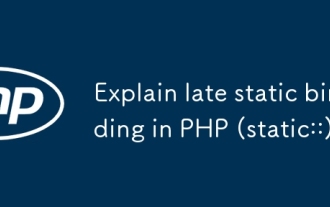
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
