My PHP study notes (graduation project)_PHP tutorial
PHP has simple syntax, very good applications, and powerful class libraries. It can indeed write a very powerful server side. For someone like me who just needs a small server, it couldn't be better.
Simply speaking, when it comes to learning PHP, I think it’s better to read the manual. I spent a few days looking at the syntax. Because I have a programming foundation, it seems to be faster now. I just finished writing a simple server in PHP, for a purpose of course, to support the client of a ticket booking system. Below are my notes on the learning process. It would be nice to have a review in the future.
When there is no object of a certain class, you can call a method in a certain class through the scope discriminator (::);
When accessing a method in a base class, you can write parent::method() ;
serialize() returns a string containing a byte stream representation of any value that can be stored in PHP.
unserialize() can use this string to reconstruct the original variable value.
Using serialization to save objects can save all variables in the object. The functions in the object are not saved, only the name of the class.
When serializing and deserializing the same object, you can use the definition file method that contains the same object.
This is because "new" does not return a reference by default, but returns a copy.
php5
Characteristics of classes and objects:
visibility: visibility
Attribute access limits: public: This attribute can be accessed anywhere,
protect derived classes or parent classes can access to this attribute, or an item within any class that defines this attribute)
private: only accessible within the class
A member declared as static can not be accessed with
an instantiated class object (though a static method can).
Static members and methods cannot be re-defined in subclasses.
(If a member is defined as static, then the member cannot be accessed by the instantiated object,
Static members cannot redefined in subclasses).
Static definition must be after accessing properties, such as: protect static
Static methods can be called without instantiation, so the $this parameter cannot be used when using static methods.
Static members cannot be accessed using ->.
constant: constant keyword, const is used to define immutable constants, and there is no need to use the $ symbol when defining.
The definition method is generally: const aconstant = 'constant';
The variables defined by glob in php are used throughout the page, including pages included in require and pages included in include.
Abstract class:
Abstract class cannot be instantiated. Any class with abstract methods must be defined as an abstract class.
If you inherit an abstract class, any abstract method in the abstract class must be overridden. The access limit of these methods can only be
the same as or lower than the access limit of the abstract parent class's methods.
Both abstract classes and abstract methods use abstract as the keyword.
Object interface (object interface)
Object interface allows you to specify which methods must be implemented, rather than letting you define which methods are captured.
Object interface is defined using the interface keyword. It is a standard class, but none of its methods are implemented.
Any method in an interface object must be public, which is what interface objects must follow.
To implement an interface, you must use the implements mark, so the interface method implementation must be in a class. A class can implement multiple interfaces.
Overloaded:
Iterator:
Iterator can access all public object members in the class.
Implement the iterator interface in PHP5, which allows you to define how objects are accessed iteratively.
Design pattern:
Design pattern provides a good framework to implement some functional organization.
Factory pattern: Instantiate a required object during runtime.
Simple interest mode: The most obvious example is: database connection object. The following is an example of the best singleton pattern:
Singleton Function
class Example
{
// Hold an instance of the class
private static $instance;
// A private constructor; prevents direct creation of object
private function __construct()
{
echo 'I am constructed';
}
// The singleton method
public static function singleton()
{
if (!isset(self::$instance)) {
$c = __CLASS__;
self::$instance = new $c;
}
return self::$instance;
}
// Example method
public function bark()
{
echo 'Woof!';
}
// Prevent users to clone the instance
public function __clone()
{
trigger_error('Clone is not allowed.', E_USER_ERROR);
}
}
你还可以实现php5里面的iteratoraggregate接口对象来定义自己的迭代方法。
魔术函数:
The function names __construct, __destruct (see Constructors and Destructors),
__call, __get, __set, __isset, __unset (see Overloading), __sleep, __wakeup,
__toString, __clone and __autoload are magical in PHP classes.
这些函数在存在于每一个php类中。你不要随意使用__来定义函数,除非你真的想这个函数具有魔术功能。
__tostring()函数,这个函数将决定一个对象转换为字符的时候将发生的事。
final关键字:
final关键字用来阻止应用final关键字声明的类或者方法被继承,被覆盖。
参数类型强制:
可以在参数前面加上类名类控制传入的参数类型。
require() 和 include() 除了怎样处理失败之外在各方面都完全一样。
include() 产生一个警告而 require() 则导致一个致命错误。
换句话说,如果想在丢失文件时停止处理页面,那就别犹豫了,用 require() 吧。
require_once() 语句在脚本执行期间包含并运行指定文件。
此行为和 require() 语句类似,
唯一区别是如果该文件中的代码已经被包含了,
则不会再次包含。有关此语句怎样工作参见 require() 的文档。
PHP 有一个类型运算符:instanceof。instanceof 用来测定一个给定的对象是否来自指定的对象类。
代码范例:
class A { }
class B { }
$thing = new A;
if ($thing instanceof A) {
echo 'A';
}
if ($thing instanceof B) {
echo 'B';
}
?>
的 PHP 代码段结束标记可以不要,有些情况下当使用输出缓冲和
include() 或者 require() 时省略掉会更好些。
include() 就不是这样,脚本会继续运行。同时也要确认设置了合适的include_path。
__CLASS__ :指的是当前类。
异常处理,根据需要扩展异常处理类exception
require()语句包含并运行指定文件;

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










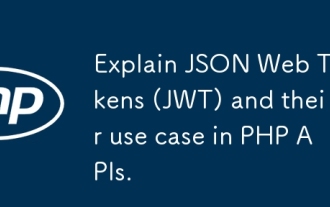
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
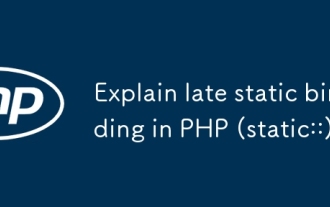
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
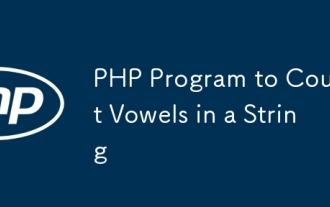
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
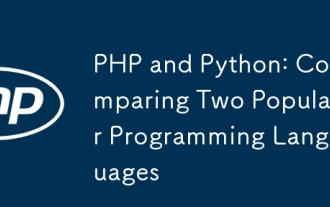
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
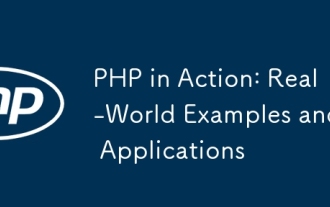
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
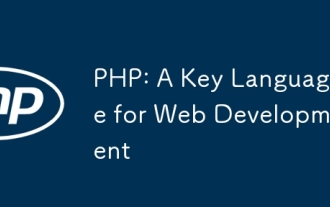
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
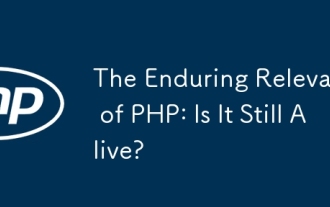
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
