


Summary of common PHP skills (with function code)_PHP tutorial
PHP file reading function
//File reading function
function PHP_Read($file_name) {
$fd=fopen($file_name,r);
while($bufline=fgets($fd, 4096)){
$buf.=$bufline;
}
fclose($fd);
return $buf;
}
?>
File writing function
//File writing function
function PHP_Write($file_name,$data,$method=”w” ) {
$filenum=@fopen($file_name,$method);
flock($filenum,LOCK_EX);
$file_data=fwrite($filenum,$data);
fclose($ filenum);
return $file_data;
}
?>
Static page generation function
//Static page generation function
function phptohtm($filefrom,$fileto,$u2u=1){
if($u2u== 1){
$data=PHP_Read($filefrom);
}else{
$data=$filefrom;
}
PHP_Write($fileto,$data);
return true;
}
?>
Specify condition information quantity retrieval function
//Specify condition information quantity retrieval function
function rec_exist($table,$where){
$query="select count(*) as num from $table " .$where;
$result=mysql_query($query) or die(nerror(1));
$rowcount=mysql_fetch_array($result);
$num=$rowcount["num"];
if ($num==0){
return false;
}
return $num;
}
?>
Directory deletion Function
//Directory deletion function
function del_DIR($directory){
$mydir=dir($directory);
while($file=$mydir->read()){
if((is_dir(“$directory/$file”)) AND ($ file!=”.”) AND ($file!=”..”)){
del_DIR(“$directory/$file”);
}else{
if(($file!= ”.”) AND ($file!=”..”)){
unlink(“$directory/$file”);
//echo “unlink $directory/$file ok “;
}
}
}
$mydir->close();
rmdir($directory);
//echo “rmdir $directory ok “;
}
?> >//Directory name legality detection
function isen($str){
for($i=0;$i $p=ord(substr($str,$ i,1));
if(($p<48 & $p!=45 & $p!=46) || ($p>57 & $p<65) || ($p>90 & $p<97 & $p!=95) || $p>122){
PHP paging function
Copy code
The code is as follows:
//Paging function
function splitlist($HALT,$LRLIST,$ECHOCNT,$paper,$table,$where,$page_id,$userid){
if($paper==”” || $sumcnt==””){
$query = “select count(*) as num from $table $where” ;
}
$sumpaper=($sumcnt-$sumcnt%$ECHOCNT )/$ECHOCNT;
if(($sumcnt%$ECHOCNT)!=0) $sumpaper+=1;
if($sumpaper==1 && $HALT==0) return($where);
$enwhere=base64_encode(base64_encode($where));
if(($LRLIST*2+1) < $sumpaper){
if(($paper-$LRLIST) < 2){
$tract=1;
$sub=$LRLIST*2+1;
}else if(($paper+$LRLIST) >= $sumpaper){
$tract=$sumpaper- ($LRLIST*2);
$sub=$sumpaper;
}else{
$tract=$paper-$LRLIST;
$sub=$paper+$LRLIST;
}
}else{
$tract=1;
$sub=$sumpaper;
}
$uppaper=$paper-1;
$downpaper=$paper+1;
$startcnt=($paper-1)*$ECHOCNT;
$where.=” limit ${ startcnt },${ ECHOCNT }”;
if($tract > 1) { $splitstr=” 【 << “; }
else $splitstr=”【 << “;
for($i=$tract;$i<=$sub;$i++){
if ( $i!=$paper) $splitstr.=”".$i.” “;
else $splitstr.=”".$i.” “;
}
if ($sub!= $sumpaper) $splitstr.=”>> 】”;
else $splitstr.=”>> 】”;
return($where);
}
?>
PHP instructions for using paging functions
/*
## ## Search paging function ####
Int $HALT - Whether (1/0) displays the page number bar when the search results are only divided into 1 page
Int $LRLIST - (The page number bar displays the page number - 1)/ 2
Int $ECHOCNT – the number of records displayed on each page during retrieval
Int $paper – number of pages, pre-extraction: $paper=$HTTP_GET_VARS[paper];
Varchar $table – data table name, pre-extraction Attached value: $table="db.table";
Varchar $where - retrieval conditions, pre-attached value: $where="where field='value'";
Varchar $enwhere - change the original $where After base64_encode() encoding twice, submit it in GET mode
Varchar $splitstr - page number bar output string, execute the function and execute echo $splitstr at the corresponding position;
Variables need to be obtained before the function call -
$paper=$HTTP_GET_VARS[paper];
$sumcnt=$HTTP_GET_VARS[sumcnt];
$enwhere=$HTTP_GET_VARS[enwhere];
Return (Varchar $where) – the search statement after paging Search conditions
Note: This function needs to call the error handling function nerror($error);
*/
PHP image file upload function
//Image file upload function
function upload_img($UploadFile,$UploadFile_name,$UploadFile_size,$UploadPath,$max_size=64){
//$TimeLimit=60; //Set the timeout limit. The default time is 30 seconds. When set to 0, there is no time limit
//set_time_limit($TimeLimit);
if(($UploadFile!= “none” )&&($UploadFile != “” )){
$FileName=$UploadPath.$UploadFile_name;
if($UploadFile_size <1024){
$FileSize=”(string)$UploadFile_size” . “byte”;
}elseif($UploadFile_size <(1024 * $max_size)){
$ FileSize=number_format((double)($UploadFile_size / 1024), 1) . ” KB”;
}else{
nerror(“The file exceeds the limit size!”);
}
// {
//$FileSize=”number_format((double)($UploadFile_size” / (1024 * 1024)), 1) . ” MB”;
// }
if(!file_exists($FileName )){
if(copy($UploadFile,$FileName)){
return “$UploadFile_name ($FileSize)”;
}else{
nerror(“File $UploadFile_name failed to upload!” );
}
unlink($UploadFile);
}else{
nerror("The file $UploadFile_name already exists!");
}
//set_time_limit(30); //Restore the default timeout setting
}
}
The following are some tips:
How does PHP determine the legality of the ip address
if(!strcmp (long2ip(sprintf(“%u”,ip2long($ip))),$ip)) echo “is ipn”;
——-
Email regularity judgment
eregi(“^[_. 0-9a-zA-Z-]+@([0-9a-zA-Z][0-9a-zA-Z_-]+.)+[a-zA-Z]$”, $email);
Example of checking whether the IP address and mask are legal
$ip = '192.168.0.84′;
$mask = '255.255.255.0′;
$network = '192.168.0′;
$ip = ip2long($ip);
$mask = ip2long($mask);
$network = ip2long($network);
if( ($ip & $mask) == $network) echo “valid ip and maskn”;
?>
—-
How to set the PHP file download header output
header(“Content-type: application/x-download” );
header(“Content-Disposition: attachment; filename=$file_download_name;”);
header(“Accept-Ranges: bytes”);
header(“Content-Length: $download_size”) ;
echo 'xxx'
PHP uses header to output ftp download method and supports breakpoint resume download
An example:
header('Pragma: public');
header('Cache -Control: private');
header('Cache-Control: no-cache, must-revalidate');
header('Accept-Ranges: bytes');
header('Connection: close ');
header(“Content-Type: audio/mpeg”);
header(“Location:ftp://download:1bk3l4s3k9s2@232.2.22.22/2222/web technology development knowledge base/cn_web.rmvb ");
PHP regular matching Chinese
ereg("^[".chr(0xa1)."-".chr(0xff)."]+$", $str);
Batch replace text Hyperlink inside
function urlParse($str = ”){
if (” == $str) return $str;
$types = array(“http”, “ftp”, “https” );
$replace = <<
”.htmlentities('1′).htmlentities('2′)."
EOPHP;
$ret = $str;
while (list(,$type) = each($types)){
$ret = preg_replace(“|($type://)([^s]*)|ie “, $replace, $ret);
}
return $ret;
}

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
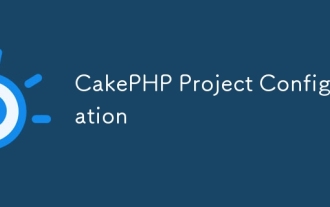
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
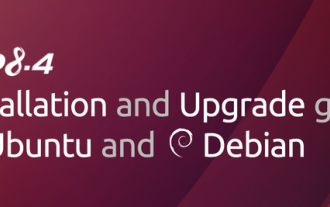
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
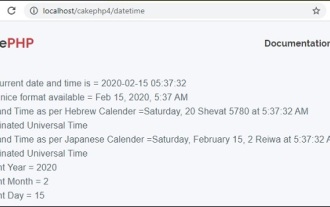
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
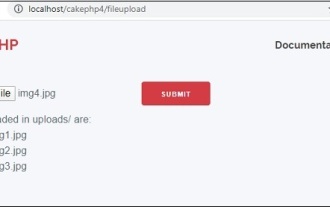
To work on file upload we are going to use the form helper. Here, is an example for file upload.
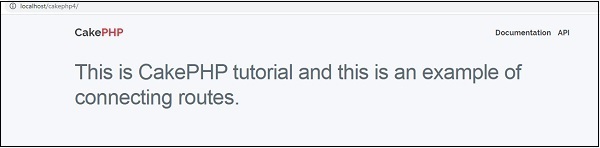
In this chapter, we are going to learn the following topics related to routing ?
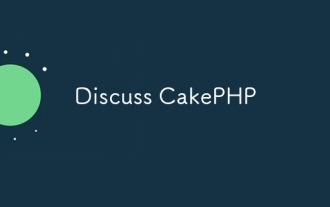
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
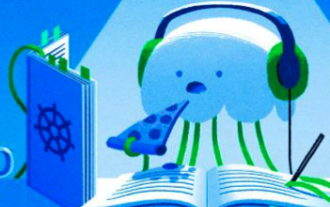
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
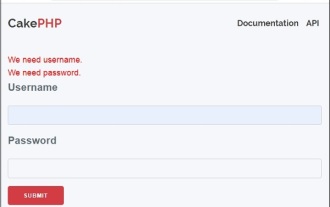
Validator can be created by adding the following two lines in the controller.
