


Examples of php combining forms to implement some simple functions_PHP tutorial
Jul 21, 2016 pm 03:29 PM
例子一(POST提交表单):
<html>
<head>
<title>
Chunkify Form
</title>
</head>
<body>
<form action="chunkify.php" method="POST">
Enter a word:
<input type="text" name="word"/><br/>
How long should be the chunks be?
<input type="text" name="number"/><br />
<input type="submit" value="Chunkify">
</form>
</body>
</html>
<html>
<head>
<title>
Chunkify Word
</title>
</head>
<?php
$word=$_POST['word'];
$number=$_POST['number'];
$chunks=ceil(strlen($word)/$number);
echo "The $number-letter chunks of '$word' are:<br/>n";
for ($i = 0;$i<$chunks;$i++){
$chunk=substr($word,$i*$number,$number);
printf("%d: %s<br />n",$i+1,$chunk);
}
?>
</body>
</html>
html显示出来的页面。
提交表单后php处理出来的页面。在这个例子中,我输入一个单词,然后给定一个长度,将单词等分成该长度的块。
演示了通过POST方法提交表单。
例子二(单选,GET接受表单):
<form action="<?php echo $_SERVER['PHP_SELF'] ?>" method="GET">
Select your personality attributes:<br/>
<select name="att[]" >
<option value="perky">perky</option>
<option value="morese">morose</option>
<option value="thinking">thinking</option>
<option value="feeling"> feeling</option>
<option value="thrifty">speed-thrift</option>
<option value="prodigal">shopper</option>
</select>
<br>
<input type ="submit" name="s" value="Record my personality">
</form>
<?php
if (array_key_exists('s',$_GET)){
$des = implode(' ', $_GET['att']);
echo "You have a $des personality.";
}
?>
例子三(多选,GET接受表单):
注意到此时<select name="att[]" multiple> 下划线告诉GET你传输的是个数组,黑体字部分则是表示改选择框为多选框
<form action="<?php echo $_SERVER['PHP_SELF'] ?>" method="GET">
Select your personality attributes:<br/>
<select name="att[]" multiple>
<option value="perky">perky</option>
<option value="morese">morose</option>
<option value="thinking">thinking</option>
<option value="feeling"> feeling</option>
<option value="thrifty">speed-thrift</option>
<option value="prodigal">shopper</option>
</select>
<br>
<input type ="submit" name="s" value="Record my personality">
</form>
<?php
if (array_key_exists('s',$_GET)){
$des = implode(' ', $_GET['att']);
echo "You have a $des personality.";
}
?>
Example 4 (checkbox): Similarly name="att[]" tells GET that what you transmit is an array, checked means that the option is the initial default Selection, the same as the above example, adding selected="selected" in the tag can also
make the initial default selection for multiple selections.
<form action="<?php echo $_SERVER['PHP_SELF'] ?>" method="GET">
Select your personality attributes:<br/>
perky<input type="checkbox" name="att[]" value="perky" checked / > <br/>
morose<input type="checkbox" name="att[]" value="morose" checked /> <br/>
thinking<input type=" checkbox" name="att[]" value="thinking" /> <br/>
feeling<input type="checkbox" name="att[]" value="feeling" /> < ;br/>
<br>
<input type ="submit" name="s" value="Record my personality">
</form>
<?php
if (array_key_exists('s',$_GET)){
echo "<pre>";
print_r($_GET);
echo "</pre> ;";
if (is_null($_GET['att'])) exit;
$des = implode(' ', $_GET['att']);
echo "You have a $des personality.";
}
?>
Example 5 (radio button): Note that the same option can be used The selected names must be equal
<form>
Male:
< input type="radio" checked="checked" name="Sex" value="male" />
<br />
Female:
<input type="radio" name ="Sex" value="female" />
<br>
<hr>
Male:
<input type="radio" checked="checked" name=" Se" value="male" />
<br />
Female:
<input type="radio" name="Se" value="female" />
</form>
<p>When the user clicks a radio button, the button becomes selected and all other buttons become unselected. </p>
Example 6 (stick form): How can a form realize that the previously entered values still exist after the page is refreshed? As follows
<?php
$f = $_POST['fa'] ;
?>
<form action = "<?php echo $_SERVER['PHP_SELF']; ?> " method="POST">
temperature :
<input type="text" name="fa" value="<?php echo $f;?>" />;
<br/>
< input type="submit" name="Convert to Celsius" />
</form>
<?php
if (!is_null($f)){
$c = ($f-32)*5/9;
printf("%.2lf is %.2lfC",$f,$c);
}
?>
It’s all simple form processing~

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
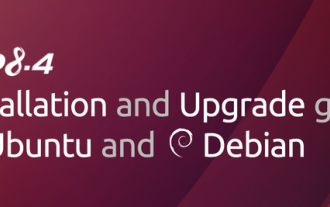
PHP 8.4 Installation and Upgrade guide for Ubuntu and Debian
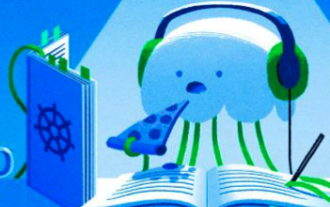
How To Set Up Visual Studio Code (VS Code) for PHP Development
