


PHP accesses MYSQL database encapsulation class (with function description)_PHP tutorial
/*
MYSQL database access encapsulation class
MYSQL Data access method, php4 supports the process access method starting with mysql_, php5 starts to support the process starting with mysqli_ and the mysqli object-oriented
access method. This encapsulation class uses mysql_ to encapsulate the general process of
data access:
1, connect to the database mysql_connect or mysql_pconnect
2, select the database mysql_select_db
3, execute the SQL query mysql_query
4, process the returned data mysql_fetch_array mysql_num_rows mysql_fetch_assoc mysql_fetch_row etc
*/
class db_mysql
{
var $querynum = 0; //The number of times the current page process queries the database
var $dblink; //Database connection resources
//Link database
function connect($dbhost ,$dbuser,$dbpw,$dbname='',$dbcharset='utf-8',$pconnect=0 , $halt=true)
{
$func = empty($pconnect) ? 'mysql_connect ' : 'mysql_pconnect' ;
$this->dblink = @$func($dbhost,$dbuser,$dbpw) ;
if ($halt && !$this->dblink)
{
$this->halt("Unable to connect to database!");
}
//Set query character set
mysql_query("SET character_set_connection={$dbcharset},character_set_results={$dbcharset },character_set_client=binary",$this->dblink) ;
//Select database
$dbname && @mysql_select_db($dbname,$this->dblink) ;
}
/ /Select database
function select_db($dbname)
{
return mysql_select_db($dbname,$this->dblink);
}
//Execute SQL query
function query ($sql)
{
$this->querynum++ ;
return mysql_query($sql,$this->dblink) ;
}
//Return the latest connection handle The number of record rows affected by the associated INSERT, UPDATE or DELETE query
function affected_rows()
{
return mysql_affected_rows($this->dblink);
}
//Get the result The number of concentrated rows is only valid for the result set of select query
function num_rows($result)
{
return mysql_num_rows($result) ;
}
//Get a single-cell query Result
function result($result,$row=0)
{
return mysql_result($result,$row);
}
//Get the ID generated by the previous INSERT operation, Only valid for operations with AUTO_INCREMENT ID on the table
function insert_id()
{
return ($id = mysql_insert_id($this->dblink)) >= 0 ? $id : $this-> ;result($this->query("SELECT last_insert_id()"), 0);
}
//Extract the current row from the result set and return it in the form of an associative array represented by a number as the key
function fetch_row($result)
{
return mysql_fetch_row($result) ;
}
//Extract the current row from the result set and return it in the form of an associative array represented by the field name key
function fetch_assoc($result)
{
return mysql_fetch_assoc($result);
}
//Extract the current row from the result set and return it in the form of an associative array represented by the field name and number as key
function fetch_array($result)
{
return mysql_fetch_array($result);
}
//Close the link
function close()
{
return mysql_close($ this->dblink) ;
}
//Output a simple error html prompt message and terminate the program
function halt($msg)
{
$message = " nn" ;
$message .= "n" ;
$message .= "n" ;
$message .= "n" ;
$message .= "Database error: ".htmlspecialchars($msg)."n" ;
$message .= "$message .= "" ;
echo $message ;
exit ;
}
}
?>

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
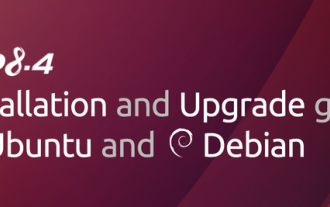
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati

One of the major changes introduced in MySQL 8.4 (the latest LTS release as of 2024) is that the "MySQL Native Password" plugin is no longer enabled by default. Further, MySQL 9.0 removes this plugin completely. This change affects PHP and other app
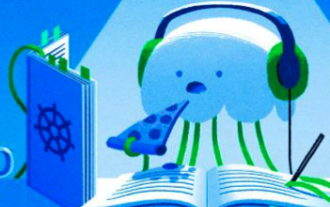
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
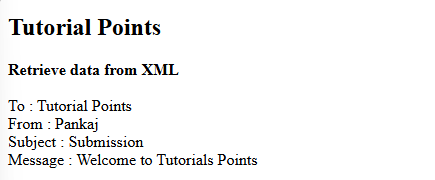
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
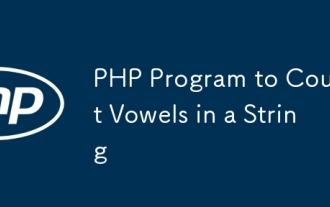
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
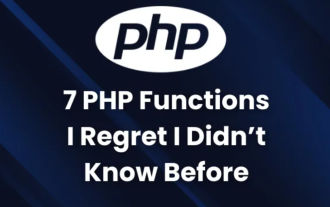
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
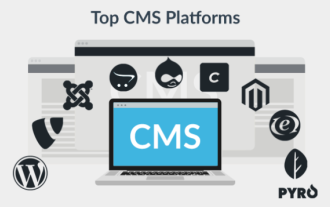
CMS stands for Content Management System. It is a software application or platform that enables users to create, manage, and modify digital content without requiring advanced technical knowledge. CMS allows users to easily create and organize content
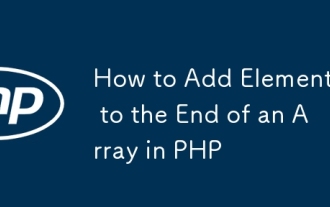
Arrays are linear data structures used to process data in programming. Sometimes when we are processing arrays we need to add new elements to the existing array. In this article, we will discuss several ways to add elements to the end of an array in PHP, with code examples, output, and time and space complexity analysis for each method. Here are the different ways to add elements to an array: Use square brackets [] In PHP, the way to add elements to the end of an array is to use square brackets []. This syntax only works in cases where we want to add only a single element. The following is the syntax: $array[] = value; Example
