Common file operations in php notes_PHP tutorial
//Common file operation functions
// The first part is file reading, writing, creation, deletion, renaming, etc.
//Before operating the file, let’s first determine whether it is a file and whether it is executable, readable and writable
$file="test.txt";
if(file_exists($file))//Whether the disk file exists
{
echo "The file exists
";
}else
{
echo "The file does not Exists, created";
$fp=fopen($file,"w");//Read-only mode creation
fclose($fp);
}
if(is_file($file ))
{
echo "is a file
";
}
if(is_dir($file))
{
echo "is a directory
" ;
}
if(is_executable($file))
{
echo "File executable
";
}
if(is_readable($file ))
{
echo "The file is readable
";
}
if(is_writable($file))
{
echo "The file is writable
}
chmod($file,0777);//Full permissions
//Mode Description The number 1 means to make the file executable, the number 2 means to make the file writable, and the number 4 means to make the file Readable - the addition of modes represents permissions
$fp=fopen("test.txt","a+");//Open with append read and write methods
//When opening a remote file
/ /$fp=fopen("test.txt","a+b"); Remember to add b;
$content=fread($fp,70);//Read 70 bytes
echo "1 .{$content}
";//Output
fwrite($fp,"I amRecommendation$content=file_get_contents("test.txt");//Reading files It is recommended to use this function to read remote files
//$content=file_get_contents( "http://www.jianlila.com");
echo "2.{$content}
";
file_put_contents("test.txt","I amLove My Parentsasddddddddddddddddddddddddddddxxxxxxxxx");
//Output to file
fclose($fp);//Close the file handle
$fp=fopen("test.txt","a+");
$content=fread($fp,filesize("test.txt"));
//Read all content filesize($file )//Number of file bytes
echo "3.{$content}
";
$fp=fopen("test.txt","r");
echo "One character ".fgetc($fp)."
";//Read one character
$fp=fopen("test.txt","r");
echo "One line".fgets( $fp)."
";//Read a line of characters
$fp=fopen("test.txt","r");
echo "remaining data";
fpassthru( $fp);
echo "
";//The remaining data can be used to output binary files
copy("test.txt","Jianlila.txt");
/ /File copy
if(file_exists("Love My Parents.txt"))
{
unlink("Love My Parents.txt");
//Delete the file if it exists
}
rename("Recommendation gift.txt","Love my parents.txt");
//File rename
if(file_exists("Recommendation gift") )
{
rmdir("Recommended gift");//Delete folder
}else
{
mkdir("Recommended gift");//Create folder
}
//Get file information function
$file="test.txt";
echo "File size".filesize($file)."bytes
echo "File type".filetype($file)."
";
//The file type here is not the .txt we see, which refers to fifo, char, dir, block, link, file and unknown
$fp=fopen($file,"r");//Open the file
print_r(fstat($fp));//Print file information
echo "Current file path information".__FILE__."
";
echo "The directory where the current file is located".dirname(__FILE__)."
";
echo "Current file name". basename(__FILE__)."
";
print_r(stat($file));//Print file information
?>

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


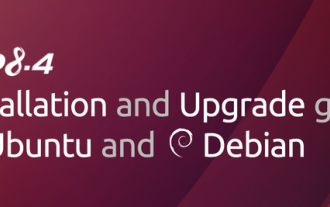
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
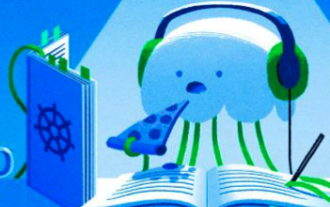
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
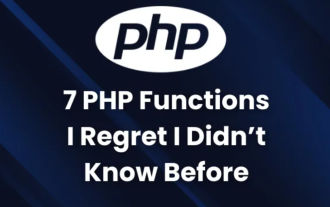
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
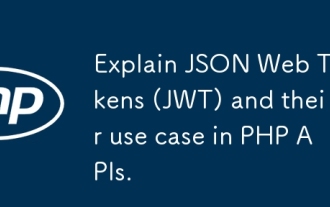
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
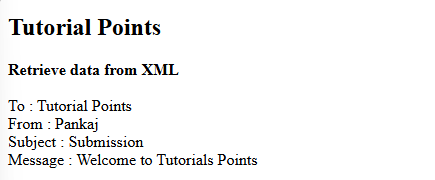
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
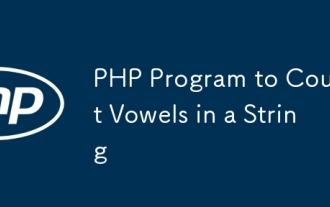
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
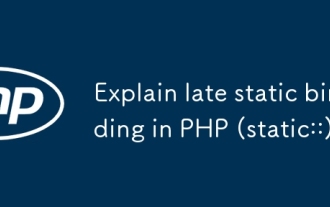
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
