


The prompt message in the placeholder disappears after the input is clicked_javascript skills
In HTML, placeholder, as an attribute of input, plays the role of occupying a place in the input box and prompting.
However, in some browsers, such as Chrome, when the mouse clicks on the input box, the value of the placeholder does not disappear. It disappears only when the data is entered, which greatly reduces the front-end user experience.
After reading a lot of masters’ methods and writing long js, it seemed a bit difficult, so I thought of the following stupidest method to solve this problem.
html code:
<input type="text" placeholder="多个关键词空格隔开">
When the mouse clicks on the input, the prompt message in the placeholder disappears:
<input type="text" placeholder="多个关键词空格隔开" onfocus="this.placeholder=‘‘" onblur="this.placeholder=‘多个关键词空格隔开‘">
Two ways to implement PlaceHolder
The placeholder attribute is input in HTML5 added. Provide a placeholder on the input that displays a hint (hint) of the expected value of the input field in text form. The field will be displayed when the input is empty.
Such as
<input type="text" name="loginName" placeholder="邮箱/手机号/QQ号">
Current browser support
However, although IE10 supports the placeholder attribute , its performance is inconsistent with other browsers
•In IE10, the placeholder text disappears when the mouse is clicked (gets focus)
•Firefox/Chrome/Safari does not disappear when clicked, but the text disappears when keyboard input
This is quite disgusting, if the placeholder attribute is used. The product manager is still reluctant to give up, and will explain why the prompt text disappears when clicking on it in IE, but the prompt text disappears when typing on the keyboard in Chrome. Ask the front-end engineer to change it to the same form of expression. In view of this, the following two implementations do not use the native placeholder attribute.
Two ways of thinking
1. (Method 1) Use the value of the input as the display text
2. (Method 2) Do not use value, add an extra tag (span) to the body and then cover it with absolute positioning on the input
Both methods have their own advantages and disadvantages. Method one occupies the value attribute of the input, which is required when submitting the form. To do some extra judgment work, the second method uses additional labels.
Method 1
/** * PlaceHolder组件 * $(input).placeholder({ * word: // @string 提示文本 * color: // @string 文本颜色 * evtType: // @string focus|keydown 触发placeholder的事件类型 * }) * * NOTE: * evtType默认是focus,即鼠标点击到输入域时默认文本消失,keydown则模拟HTML5 placeholder属性在Firefox/Chrome里的特征,光标定位到输入域后键盘输入时默认文本才消失。 * 此外,对于HTML5 placeholder属性,IE10+和Firefox/Chrome/Safari的表现形式也不一致,因此内部实现不采用原生placeholder属性 */ $.fn.placeholder = function(option, callback) { var settings = $.extend({ word: '', color: '#ccc', evtType: 'focus' }, option) function bootstrap($that) { // some alias var word = settings.word var color = settings.color var evtType = settings.evtType // default var defColor = $that.css('color') var defVal = $that.val() if (defVal == '' || defVal == word) { $that.css({color: color}).val(word) } else { $that.css({color: defColor}) } function switchStatus(isDef) { if (isDef) { $that.val('').css({color: defColor}) } else { $that.val(word).css({color: color}) } } function asFocus() { $that.bind(evtType, function() { var txt = $that.val() if (txt == word) { switchStatus(true) } }).bind('blur', function() { var txt = $that.val() if (txt == '') { switchStatus(false) } }) } function asKeydown() { $that.bind('focus', function() { var elem = $that[0] var val = $that.val() if (val == word) { setTimeout(function() { // 光标定位到首位 $that.setCursorPosition({index: 0}) }, 10) } }) } if (evtType == 'focus') { asFocus() } else if (evtType == 'keydown') { asKeydown() } // keydown事件里处理placeholder $that.keydown(function() { var val = $that.val() if (val == word) { switchStatus(true) } }).keyup(function() { var val = $that.val() if (val == '') { switchStatus(false) $that.setCursorPosition({index: 0}) } }) } return this.each(function() { var $elem = $(this) bootstrap($elem) if ($.isFunction(callback)) callback($elem) }) }
Method 2
$.fn.placeholder = function(option, callback) { var settings = $.extend({ word: '', color: '#999', evtType: 'focus', zIndex: 20, diffPaddingLeft: 3 }, option) function bootstrap($that) { // some alias var word = settings.word var color = settings.color var evtType = settings.evtType var zIndex = settings.zIndex var diffPaddingLeft = settings.diffPaddingLeft // default css var width = $that.outerWidth() var height = $that.outerHeight() var fontSize = $that.css('font-size') var fontFamily = $that.css('font-family') var paddingLeft = $that.css('padding-left') // process paddingLeft = parseInt(paddingLeft, 10) + diffPaddingLeft // redner var $placeholder = $('') $placeholder.css({ position: 'absolute', zIndex: '20', color: color, width: (width - paddingLeft) + 'px', height: height + 'px', fontSize: fontSize, paddingLeft: paddingLeft + 'px', fontFamily: fontFamily }).text(word).hide() // 位置调整 move() // textarea 不加line-heihgt属性 if ($that.is('input')) { $placeholder.css({ lineHeight: height + 'px' }) } $placeholder.appendTo(document.body) // 内容为空时才显示,比如刷新页面输入域已经填入了内容时 var val = $that.val() if ( val == '' && $that.is(':visible') ) { $placeholder.show() } function hideAndFocus() { $placeholder.hide() $that[0].focus() } function move() { var offset = $that.offset() var top = offset.top var left = offset.left $placeholder.css({ top: top, left: left }) } function asFocus() { $placeholder.click(function() { hideAndFocus() // 盖住后无法触发input的click事件,需要模拟点击下 setTimeout(function(){ $that.click() }, 100) }) // IE有些bug,原本不用加此句 $that.click(hideAndFocus) $that.blur(function() { var txt = $that.val() if (txt == '') { $placeholder.show() } }) } function asKeydown() { $placeholder.click(function() { $that[0].focus() }) } if (evtType == 'focus') { asFocus() } else if (evtType == 'keydown') { asKeydown() } $that.keyup(function() { var txt = $that.val() if (txt == '') { $placeholder.show() } else { $placeholder.hide() } }) // 窗口缩放时处理 $(window).resize(function() { move() }) // cache $that.data('el', $placeholder) $that.data('move', move) } return this.each(function() { var $elem = $(this) bootstrap($elem) if ($.isFunction(callback)) callback($elem) }) }
Method 2 is not suitable for the following scenarios
1. Initial hiding of input
At this time, the offset of the input cannot be obtained, and the span cannot be positioned on the input.
2. The dom structure of the page containing the input changes
For example, some elements are deleted or added to the page, causing the input to shift upward or downward. At this time, the span has no offset (span is positioned relative to the body). This is disgusting. You can consider using span as a sibling element of input, that is, positioned relative to the inner p (instead of body). But this must force the addition of position:relative to the outer p, which may have a certain impact on the page layout.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


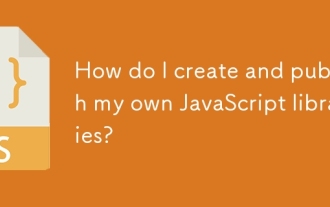
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
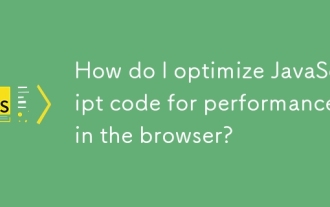
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
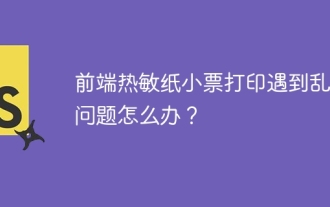
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
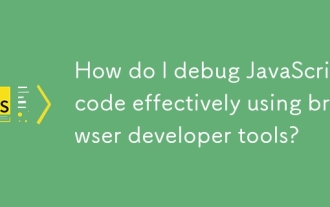
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
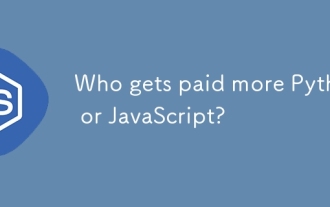
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
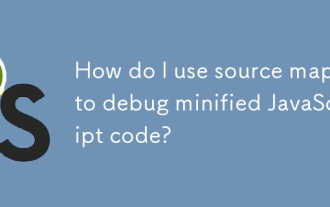
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
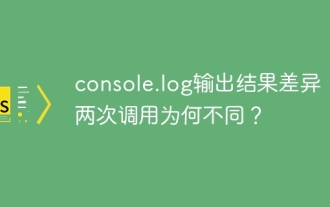
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
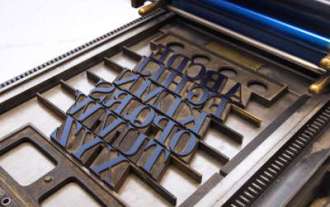
Once you have mastered the entry-level TypeScript tutorial, you should be able to write your own code in an IDE that supports TypeScript and compile it into JavaScript. This tutorial will dive into various data types in TypeScript. JavaScript has seven data types: Null, Undefined, Boolean, Number, String, Symbol (introduced by ES6) and Object. TypeScript defines more types on this basis, and this tutorial will cover all of them in detail. Null data type Like JavaScript, null in TypeScript
