Two powerful PHP image processing classes 1_PHP tutorial
/**
* Basic image processing, used to complete image indentation and watermark addition
* When the watermark image exceeds the target image size, the watermark image can automatically adapt to the target image and shrink
* The watermark image can be set to match the background Degree of Merger
*
* Copyright(c) 2005 by ustb99. All rights reserved
*
* To contact the author write to {@link mailto:ustb80@163.com}
*
* @author by chance
* @version $Id: thumb.class.php,v 1.9 2006/09/30 09:31:56 zengjian Exp $
* @package system
*/
/**
* ThumbHandler
* @access public
*/
/*
Usage:
Automatic cropping:
The program will crop the largest square from the middle according to the size of the image and shrink it according to the target size
$t->setSrcImg("img/test.jpg");
$t-> ;setCutType(1);//This sentence is OK
$t->setDstImg("tmp/new_test.jpg");
$t->createImg(60,60);
Manual cutting:
The program will take the image from the source image according to the specified position
$t->setSrcImg("img/test.jpg");
$t->setCutType(2) ;//Indicate manual cutting
$t->setSrcCutPosition(100, 100);//Coordinates of the starting point of the source image
$t->setRectangleCut(300, 200);//Cutting size
$t->setDstImg("tmp/new_test.jpg");
$t->createImg(300,200);
*/
class ThumbHandler
{
var $ dst_img; // Target file
var $h_src; // Image resource handle
var $h_dst; // New image handle
var $h_mask; // Watermark handle
var $img_create_quality = 100; // Image generation quality
var $img_display_quality = 80; // Image display quality, default is 75
var $img_scale = 0; // Image scaling ratio
var $src_w = 0; // Original image Width
var $src_h = 0;//Height of original image
var $dst_w = 0;//Total width of new image
var $dst_h = 0;//Total height of new image
var $ fill_w;// Fill graphic width
var $fill_h;// Fill graphic height
var $copy_w;// Copy graphic width
var $copy_h;// Copy graphic height
var $src_x = 0;//The starting abscissa of the original image drawing
var $src_y = 0;//The starting ordinate of the original image drawing
var $start_x;//The starting abscissa of the new image drawing
var $ start_y;// The starting ordinate of the new picture drawing
var $mask_word;// The watermark text
var $mask_img;// The watermark image
var $mask_pos_x = 0;//The abscissa of the watermark
var $mask_pos_y = 0; // Watermark vertical coordinate
var $mask_offset_x = 5; // Watermark horizontal offset
var $mask_offset_y = 5; // Watermark vertical offset
var $font_w;// Watermark font width
var $font_h;// Watermark font height
var $mask_w;// Watermark width
var $mask_h;// Watermark height
var $mask_font_color = "#ffffff";/ / Watermark text color
var $mask_font = 2;// Watermark font
var $font_size;// Size
var $mask_position = 0;// Watermark position
var $mask_img_pct = 50;/ / The degree of image merging, the larger the value, the lower the merging process
var $mask_txt_pct = 50; // The degree of text merging, the smaller the value, the lower the merging process
var $img_border_size = 0; // Image border size
var $img_border_color;// Image border color
var $_flip_x=0;//Number of horizontal flips
var $_flip_y=0;//Number of vertical flips
var $cut_type=0;/ / Cut type
var $img_type;// File type
// File type definition, and points out the function to output the image
var $all_type = array(
"jpg" => array ("output"=>"imagejpeg"),
"gif" => array("output"=>"imagegif"),
"png" => array("output"=> ;"imagepng"),
"wbmp" => array("output"=>"image2wbmp"),
"jpeg" => array("output"=>"imagejpeg")) ;
/**
* Constructor
*/
function ThumbHandler()
{
$this->mask_font_color = "#ffffff";
$this->font = 2;
$this->font_size = 12;
}
/**
* Get the width of the image
*/
function getImgWidth($src)
{
return imagesx($src);
}
/**
* Get the height of the image
*/
function getImgHeight($src)
{
return imagesy($src);
}
/**
* Set the image generation path
*
* @param string $src_img Image generation path
*/
function setSrcImg($src_img, $img_type=null)
{
if(!file_exists($src_img))
{
die("Picture does not exist");
}
if(!emptyempty($img_type))
{
$this->img_type = $img_type;
}
else
{
$this- >img_type = $this->_getImgType($src_img);
}
$this->_checkValid($this->img_type);
// The file_get_contents function requires PHP version>4.3 .0
$src = '';
if(function_exists("file_get_contents"))
{
$src = file_get_contents($src_img);
}
else
{
$handle = fopen ($src_img, "r");
while (!feof ($handle))
{
$src .= fgets($fd, 4096);
}
fclose ($handle);
}
if(emptyempty($src))
{
die("Image source is empty");
}
$this->h_src = @ImageCreateFromString($src);
$this->src_w = $this->getImgWidth($this->h_src);
$this->src_h = $ this->getImgHeight($this->h_src);
}
/**
* Set the image generation path
*
* @param string $dst_img Image generation path
*/
function setDstImg($dst_img)
{
$arr = explode('/',$dst_img);
$last = array_pop($arr);
$path = implode('/',$arr);
$this->_mkdirs($path);
$this->dst_img = $dst_img;
}
/**
* Set the display quality of the image
*
* @param string $n quality
*/
function setImgDisplayQuality($n)
{
$this->img_display_quality = (int)$n;
}
/**
* Set the image generation quality
*
* @param string $n quality
*/
function setImgCreateQuality($n)
{
$this->img_create_quality = (int)$n;
}
/**
* Set text watermark
*
* @param string $word watermark text
* @param integer $font watermark font
* @param string $color watermark font color
*/
function setMaskWord($word)
{
$this->mask_word .= $word;
}
/**
* Set font color
*
* @param string $color font color
*/
function setMaskFontColor($color="#ffffff")
{
$this->mask_font_color = $color;
}
/**
* Set watermark font
*
* @param string|integer $font font
*/
function setMaskFont($font=2)
{
if(!is_numeric($font) && !file_exists($font))
{
die("字体文件不存在");
}
$this->font = $font;
}
/**
* Set text font size, only valid for truetype fonts
*/
function setMaskFontSize($size = "12")
{
$this->font_size = $size;
}
/**
* Set image watermark
*
* @param string $img Watermark image source
*/
function setMaskImg($img)
{
$this->mask_img = $img;
}
/**
* Set the horizontal offset of the watermark
*
* @param integer $x horizontal offset
*/
function setMaskOffsetX($x)
{
$this->mask_offset_x = (int)$x;
}
/**
* Set the vertical offset of the watermark
*
* @param integer $y vertical offset
*/
function setMaskOffsetY($y)
{
$this->mask_offset_y = (int)$y;
}
/**
* Specify the watermark position
*
* @param integer $position position, 1: upper left, 2: lower left, 3: upper right, 0/4: lower right
*/
function setMaskPosition($position=0)
{
$this->mask_position = (int)$position;
}
/**
* Set the degree of image merging
*
* @param integer $n The degree of merging
*/
function setMaskImgPct($n)
{
$this->mask_img_pct = (int)$n;
}
/**
* Set the text merging degree
*
* @param integer $n Merging degree
*/
function setMaskTxtPct($n)
{
$this->mask_txt_pct = (int)$n;
}
/**
* Set thumbnail border
*
* @param (type) (parameter name) (description)
*/
function setDstImgBorder($size=1, $color="#000000")
{
$this->img_border_size = (int)$size;
$this->img_border_color = $color;
}
/**
* Flip horizontally
*/
function flipH()
{
$this->_flip_x++;
}
/**
* Flip vertically
*/
function flipV()
{
$this->_flip_y++;
}
/**
* Set cutting type
*
* @param (type) (parameter name) (description)
*/
function setCutType($type)
{
$this->cut_type = (int)$type;
}
/**
* Set image clipping
*
* @param integer $width rectangular clipping
*/
function setRectangleCut($width, $height)
{
$this->fill_w = (int)$width;
$this->fill_h = (int)$height;
}
/**
* Set the starting coordinate point for cutting the source image
*
* @param (type) (parameter name) (description)
*/
function setSrcCutPosition($x, $y)
{
$this->src_x = (int)$x;
$this->src_y = (int)$y;
}
/**
* Create image, main function
* @param integer $a When the second parameter is missing, this parameter will be used as a percentage,
* otherwise as the width value
* @param integer $b The height of the image after scaling
*/
function createImg($a, $b=null)
{
$num = func_num_args();
if(1 == $num)
{
$r = (int)$a;
if($r < 1)
{
die("图片缩放比例不得小于1");
}
$this->img_scale = $r;
$this->_setNewImgSize($r);
}
if(2 == $num)
{
$w = (int)$a;
$h = (int)$b;
if(0 == $w)
{
die("目标宽度不能为0");
}
if(0 == $h)
{
die("目标高度不能为0");
}
$this->_setNewImgSize($w, $h);
}
if($this->_flip_x%2!=0)
{
$this->_flipH($this->h_src);
}
if($this->_flip_y%2!=0)
{
$this->_flipV($this->h_src);
}
$this->_createMask();
$this->_output();
// 释放
if(imagedestroy($this->h_src) && imagedestroy($this->h_dst))
{
Return true;
}
else
{
Return false;
}
}
/**
* To generate watermark, two methods are called to generate watermark text and watermark image
*/
function _createMask()
{
if($this->mask_word)
{
// 获取字体信息
$this->_setFontInfo();
if($this->_isFull())
{
die("水印文字过大");
}
else
{
$this->h_dst = imagecreatetruecolor($this->dst_w, $this->dst_h);
$white = ImageColorAllocate($this->h_dst,255,255,255);
imagefilledrectangle($this->h_dst,0,0,$this->dst_w,$this->dst_h,$white);// 填充背景色
$this->_drawBorder();
imagecopyresampled( $this->h_dst, $this->h_src,
$this->start_x, $this->start_y,
$this->src_x, $this->src_y,
$this->fill_w, $this->fill_h,
$this->copy_w, $this->copy_h);
$this->_createMaskWord($this->h_dst);
}
}
if($this->mask_img)
{
$this->_loadMaskImg();//加载时,取得宽高
if($this->_isFull())
{
// 将水印生成在原图上再拷
$this->_createMaskImg($this->h_src);
$this->h_dst = imagecreatetruecolor($this->dst_w, $this->dst_h);
$white = ImageColorAllocate($this->h_dst,255,255,255);
imagefilledrectangle($this->h_dst,0,0,$this->dst_w,$this->dst_h,$white);// 填充背景色
$this->_drawBorder();
imagecopyresampled( $this->h_dst, $this->h_src,
$this->start_x, $this->start_y,
$this->src_x, $this->src_y,
$this->fill_w, $this->start_y,
$this->copy_w, $this->copy_h);
}
else
{
// 创建新图并拷贝
$this->h_dst = imagecreatetruecolor($this->dst_w, $this->dst_h);
$white = ImageColorAllocate($this->h_dst,255,255,255);
imagefilledrectangle($this->h_dst,0,0,$this->dst_w,$this->dst_h,$white);// 填充背景色
$this->_drawBorder();
imagecopyresampled( $this->h_dst, $this->h_src,
$this->start_x, $this->start_y,
$this->src_x, $this->src_y,
$this->fill_w, $this->fill_h,
$this->copy_w, $this->copy_h);
$this->_createMaskImg($this->h_dst);
}
}
if(emptyempty($this->mask_word) && emptyempty($this->mask_img))
{
$this->h_dst = imagecreatetruecolor($this->dst_w, $this->dst_h);
$white = ImageColorAllocate($this->h_dst,255,255,255);
imagefilledrectangle($this->h_dst,0,0,$this->dst_w,$this->dst_h,$white);// 填充背景色
$this->_drawBorder();
imagecopyresampled( $this->h_dst, $this->h_src,
$this->start_x, $this->start_y,
$this->src_x, $this->src_y,
$this->fill_w, $this->fill_h,
$this->copy_w, $this->copy_h);
}
}
/**
* Draw borders
*/
function _drawBorder()
{
if(!emptyempty($this->img_border_size))
{
$c = $this->_parseColor($this->img_border_color);
$color = ImageColorAllocate($this->h_src,$c[0], $c[1], $c[2]);
imagefilledrectangle($this->h_dst,0,0,$this->dst_w,$this->dst_h,$color);// 填充背景色
}
}
/**
* Generate watermark text
*/
function _createMaskWord($src)
{
$this->_countMaskPos();
$this->_checkMaskValid();
$c = $this->_parseColor($this->mask_font_color);
$color = imagecolorallocatealpha($src, $c[0], $c[1], $c[2], $this->mask_txt_pct);
if(is_numeric($this->font))
{
imagestring($src,
$this->font,
$this->mask_pos_x, $this->mask_pos_y,
$this->mask_word,
$color);
}
else
{
imagettftext($src,
$this->font_size, 0,
$this->mask_pos_x, $this->mask_pos_y,
$color,
$this->font,
$this->mask_word);
}
}
/**
* Generate watermark image
*/
function _createMaskImg($src)
{
$this->_countMaskPos();
$this->_checkMaskValid();
imagecopymerge($src,
$this->h_mask,
$this->mask_pos_x ,$this->mask_pos_y,
0, 0,
$this->mask_w, $this->mask_h,
$this->mask_img_pct);
imagedestroy($this->h_mask);
}
/**
* Load watermark image
*/
function _loadMaskImg()
{
$mask_type = $this->_getImgType($this->mask_img);
$this->_checkValid($mask_type);
// file_get_contents函数要求php版本>4.3.0
$src = '';
if(function_exists("file_get_contents"))
{
$src = file_get_contents($this->mask_img);
}
else
{
$handle = fopen ($this->mask_img, "r");
while (!feof ($handle))
{
$src .= fgets($fd, 4096);
}
fclose ($handle);
}
if(emptyempty($this->mask_img))
{
die("水印图片为空");
}
$this->h_mask = ImageCreateFromString($src);
$this->mask_w = $this->getImgWidth($this->h_mask);
$this->mask_h = $this->getImgHeight($this->h_mask);
}
/**
* Image output
*/
function _output()
{
$img_type = $this->img_type;
$func_name = $this->all_type[$img_type]['output'];
if(function_exists($func_name))
{
// 判断浏览器,若是IE就不发送头
if(isset($_SERVER['HTTP_USER_AGENT']))
{
$ua = strtoupper($_SERVER['HTTP_USER_AGENT']);
if(!preg_match('/^.*MSIE.*)$/i',$ua))
{
header("Content-type:$img_type");
}
}
$func_name($this->h_dst, $this->dst_img, $this->img_display_quality);
}
else
{
Return false;
}
}
/**
* Analyze color
*
* @param string $color Hexadecimal color
*/
function _parseColor($color)
{
$arr = array();
for($ii=1; $ii
$arr[] = hexdec(substr($color,$ii,2));
$ii++;
}
Return $arr;
}
/**
* Calculate the position coordinates
*/
function _countMaskPos()
{
if($this->_isFull())
{
switch($this->mask_position)
{
case 1:
// 左上
$this->mask_pos_x = $this->mask_offset_x + $this->img_border_size;
$this->mask_pos_y = $this->mask_offset_y + $this->img_border_size;
break;
case 2:
// 左下
$this->mask_pos_x = $this->mask_offset_x + $this->img_border_size;
$this->mask_pos_y = $this->src_h - $this->mask_h - $this->mask_offset_y;
break;
case 3:
// 右上
$this->mask_pos_x = $this->src_w - $this->mask_w - $this->mask_offset_x;
$this->mask_pos_y = $this->mask_offset_y + $this->img_border_size;
break;
case 4:
// 右下
$this->mask_pos_x = $this->src_w - $this->mask_w - $this->mask_offset_x;
$this->mask_pos_y = $this->src_h - $this->mask_h - $this->mask_offset_y;
break;
default:
// 默认将水印放到右下,偏移指定像素
$this->mask_pos_x = $this->src_w - $this->mask_w - $this->mask_offset_x;
$this->mask_pos_y = $this->src_h - $this->mask_h - $this->mask_offset_y;
break;
}
}
else
{
switch($this->mask_position)
{
case 1:
// 左上
$this->mask_pos_x = $this->mask_offset_x + $this->img_border_size;
$this->mask_pos_y = $this->mask_offset_y + $this->img_border_size;
break;
case 2:
// 左下
$this->mask_pos_x = $this->mask_offset_x + $this->img_border_size;
$this->mask_pos_y = $this->dst_h - $this->mask_h - $this->mask_offset_y - $this->img_border_size;
break;
case 3:
// 右上
$this->mask_pos_x = $this->dst_w - $this->mask_w - $this->mask_offset_x - $this->img_border_size;
$this->mask_pos_y = $this->mask_offset_y + $this->img_border_size;
break;
case 4:
// 右下
$this->mask_pos_x = $this->dst_w - $this->mask_w - $this->mask_offset_x - $this->img_border_size;
$this->mask_pos_y = $this->dst_h - $this->mask_h - $this->mask_offset_y - $this->img_border_size;
break;
default:
// 默认将水印放到右下,偏移指定像素
$this->mask_pos_x = $this->dst_w - $this->mask_w - $this->mask_offset_x - $this->img_border_size;
$this->mask_pos_y = $this->dst_h - $this->mask_h - $this->mask_offset_y - $this->img_border_size;
break;
}
}
}
/**
* Set font information
*/
function _setFontInfo()
{
if(is_numeric($this->font))
{
$this->font_w = imagefontwidth($this->font);
$this->font_h = imagefontheight($this->font);
// 计算水印字体所占宽高
$word_length = strlen($this->mask_word);
$this->mask_w = $this->font_w*$word_length;
$this->mask_h = $this->font_h;
}
else
{
$arr = imagettfbbox ($this->font_size,0, $this->font,$this->mask_word);
$this->mask_w = abs($arr[0] - $arr[2]);
$this->mask_h = abs($arr[7] - $arr[1]);
}
}
/**
* Set the new image size
*
* @param integer $img_w target width
* @param integer $img_h target height
*/
function _setNewImgSize($img_w, $img_h=null)
{
$num = func_num_args();
if(1 == $num)
{
$this->img_scale = $img_w;// 宽度作为比例
$this->fill_w = round($this->src_w * $this->img_scale / 100) - $this->img_border_size*2;
$this->fill_h = round($this->src_h * $this->img_scale / 100) - $this->img_border_size*2;
// 源文件起始坐标
$this->src_x = 0;
$this->src_y = 0;
$this->copy_w = $this->src_w;
$this->copy_h = $this->src_h;
// 目标尺寸
$this->dst_w = $this->fill_w + $this->img_border_size*2;
$this->dst_h = $this->fill_h + $this->img_border_size*2;
}
if(2 == $num)
{
$fill_w = (int)$img_w - $this->img_border_size*2;
$fill_h = (int)$img_h - $this->img_border_size*2;
if($fill_w < 0 || $fill_h < 0)
{
die("图片边框过大,已超过了图片的宽度");
}
$rate_w = $this->src_w/$fill_w;
$rate_h = $this->src_h/$fill_h;
switch($this->cut_type)
{
case 0:
// 如果原图大于缩略图,产生缩小,否则不缩小
if($rate_w < 1 && $rate_h < 1)
{
$this->fill_w = (int)$this->src_w;
$this->fill_h = (int)$this->src_h;
}
else
{
if($rate_w >= $rate_h)
{
$this->fill_w = (int)$fill_w;
$this->fill_h = round($this->src_h/$rate_w);
}
else
{
$this->fill_w = round($this->src_w/$rate_h);
$this->fill_h = (int)$fill_h;
}
}
$this->src_x = 0;
$this->src_y = 0;
$this->copy_w = $this->src_w;
$this->copy_h = $this->src_h;
// 目标尺寸
$this->dst_w = $this->fill_w + $this->img_border_size*2;
$this->dst_h = $this->fill_h + $this->img_border_size*2;
break;
// 自动裁切
case 1:
// 如果图片是缩小剪切才进行操作
if($rate_w >= 1 && $rate_h >=1)
{
if($this->src_w > $this->src_h)
{
$src_x = round($this->src_w-$this->src_h)/2;
$this->setSrcCutPosition($src_x, 0);
$this->setRectangleCut($fill_h, $fill_h);
$this->copy_w = $this->src_h;
$this->copy_h = $this->src_h;
}
elseif($this->src_w < $this->src_h)
{
$src_y = round($this->src_h-$this->src_w)/2;
$this->setSrcCutPosition(0, $src_y);
$this->setRectangleCut($fill_w, $fill_h);
$this->copy_w = $this->src_w;
$this->copy_h = $this->src_w;
}
else
{
$this->setSrcCutPosition(0, 0);
$this->copy_w = $this->src_w;
$this->copy_h = $this->src_w;
$this->setRectangleCut($fill_w, $fill_h);
}
}
else
{
$this->setSrcCutPosition(0, 0);
$this->setRectangleCut($this->src_w, $this->src_h);
$this->copy_w = $this->src_w;
$this->copy_h = $this->src_h;
}
// 目标尺寸
$this->dst_w = $this->fill_w + $this->img_border_size*2;
$this->dst_h = $this->fill_h + $this->img_border_size*2;
break;
// 手工裁切
case 2:
$this->copy_w = $this->fill_w;
$this->copy_h = $this->fill_h;
// 目标尺寸
$this->dst_w = $this->fill_w + $this->img_border_size*2;
$this->dst_h = $this->fill_h + $this->img_border_size*2;
break;
default:
break;
}
}
// 目标文件起始坐标
$this->start_x = $this->img_border_size;
$this->start_y = $this->img_border_size;
}
/**
* Check whether the watermark image is larger than the width and height of the generated image
*/
function _isFull()
{
Return ( $this->mask_w + $this->mask_offset_x > $this->fill_w
|| $this->mask_h + $this->mask_offset_y > $this->fill_h)
?true:false;
}
/**
* Check whether the watermark image exceeds the original image
*/
function _checkMaskValid()
{
if( $this->mask_w + $this->mask_offset_x > $this->src_w
|| $this->mask_h + $this->mask_offset_y > $this->src_h)
{
die("水印图片尺寸大于原图,请缩小水印图");
}
}
/**
* Get the image type
*
* @param string $file_path file path
*/
function _getImgType($file_path)
{
$type_list = array("1"=>"gif","2"=>"jpg","3"=>"png","4"=>"swf","5" => "psd","6"=>"bmp","15"=>"wbmp");
if(file_exists($file_path))
{
$img_info = @getimagesize ($file_path);
if(isset($type_list[$img_info[2]]))
{
Return $type_list[$img_info[2]];
}
}
else
{
die("文件不存在,不能取得文件类型!");
}
}
/**
* Check whether the image type is legal and call the array_key_exists function. This function requires
* PHP version is greater than 4.1.0
*
* @param string $img_type file type
*/
function _checkValid($img_type)
{
if(!array_key_exists($img_type, $this->all_type))
{
Return false;
}
}
/**
* Generate directory according to the specified path
*
* @param string $path path
*/
function _mkdirs($path)
{
$adir = explode('/',$path);
$dirlist = '';
$rootdir = array_shift($adir);
if(($rootdir!='.'||$rootdir!='..')&&!file_exists($rootdir))
{
@mkdir($rootdir);
}
foreach($adir as $key=>$val)
{
if($val!='.'&&$val!='..')
{
$dirlist .= "/".$val;
$dirpath = $rootdir.$dirlist;
if(!file_exists($dirpath))
{
@mkdir($dirpath);
@chmod($dirpath,0777);
}
}
}
}
/**
* Vertical flip
*
* @param string $src Image source
*/
function _flipV($src)
{
$src_x = $this->getImgWidth($src);
$src_y = $this->getImgHeight($src);
$new_im = imagecreatetruecolor($src_x, $src_y);
for ($y = 0; $y < $src_y; $y++)
{
imagecopy($new_im, $src, 0, $src_y - $y - 1, 0, $y, $src_x, 1);
}
$this->h_src = $new_im;
}
/**
* Flip horizontally
*
* @param string $src Image source
*/
function _flipH($src)
{
$src_x = $this->getImgWidth($src);
$src_y = $this->getImgHeight($src);
$new_im = imagecreatetruecolor($src_x, $src_y);
for ($x = 0; $x < $src_x; $x++)
{
imagecopy($new_im, $src, $src_x - $x - 1, 0, $x, 0, 1, $src_y);
}
$this->h_src = $new_im;
}
}
Usage example:
< ?php
require_once('lib/thumb.class.php');
$t = new ThumbHandler();
$t->setSrcImg( "img/test.jpg");
$t->setDstImg("tmp/new_test.jpg");
$t->setMaskImg("img/test.gif");
$t->setMaskPosition(1);
$t->setMaskImgPct(80);
$t->setDstImgBorder(4,"#dddddd");
//Specify zoom ratio
$t->createImg(300,200);
?>
require_once('lib/thumb.class.php');
$t = new ThumbHandler( );
// Basic usage
$t->setSrcImg("img/test.jpg");
$t->setMaskWord("test");
$t-> ;setDstImgBorder(10,"#dddddd");
//Specify the scaling ratio
$t->createImg(50);
?>
equire_once( 'lib/thumb.class.php');
$t = new ThumbHandler();
//Basic usage
$t->setSrcImg("img/test.jpg");
$t->setMaskWord("test");
//Specify fixed width and height
$t->createImg(200,200);
?>
require_once('lib/thumb.class.php');
$t = new ThumbHandler();
$t->setSrcImg("img/test.jpg");
$t- >setDstImg("tmp/new_test.jpg");
$t->setMaskWord("test");
// Specify fixed width and height
$t->createImg(200,200);
?>
require_once('lib/thumb.class.php');
$t = new ThumbHandler();
$t->setSrcImg( "img/test.jpg");
//Specify the font file address
$t->setMaskFont("c:/winnt/fonts/arial.ttf");
$t-> setMaskFontSize(20);
$t->setMaskFontColor("#ffff00");
$t->setMaskWord("test3333333");
$t->setDstImgBorder(99,"# dddddd");
$t->createImg(50);
?>
require_once('lib/thumb.class.php');
$ t = new ThumbHandler();
$t->setSrcImg("img/test.jpg");
$t->setMaskOffsetX(55);
$t->setMaskOffsetY(55 );
$t->setMaskPosition(1);
//$t->setMaskPosition(2);
//$t->setMaskPosition(3);
// $t->setMaskPosition(4);
$t->setMaskFontColor("#ffff00");
$t->setMaskWord("test");
// Specify fixed width and height
$t->createImg(50);
?>
require_once('lib/thumb.class.php');
$t = new ThumbHandler ();
$t->setSrcImg("img/test.jpg");
$t->setMaskFont("c:/winnt/fonts/simyou.ttf");
$ t->setMaskFontSize(20);
$t->setMaskFontColor("#ffffff");
$t->setMaskTxtPct(20);
$t->setDstImgBorder(10, "#dddddd");
$text = "Chinese";
$str = mb_convert_encoding($text, "UTF-8", "gb2312");
$t->setMaskWord($str );
$t->setMaskWord("test");
//Specify fixed width and height
$t->createImg(50);
?>

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
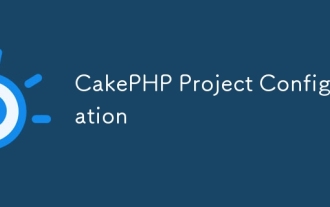
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
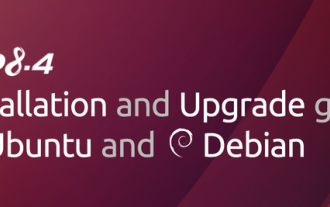
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
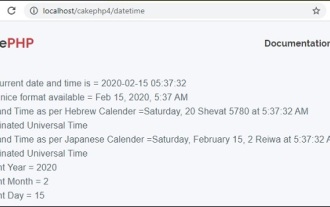
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
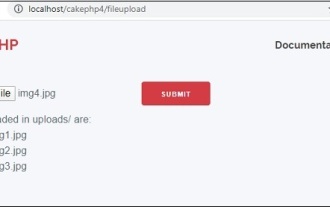
To work on file upload we are going to use the form helper. Here, is an example for file upload.
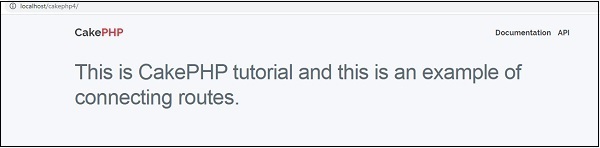
In this chapter, we are going to learn the following topics related to routing ?
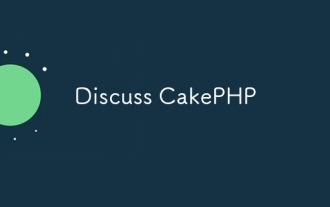
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
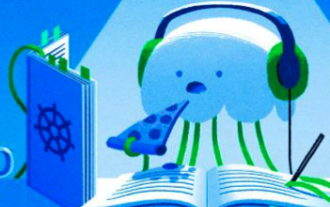
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
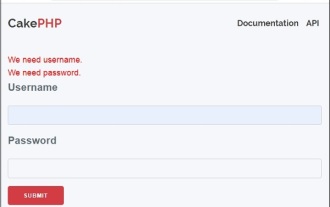
Validator can be created by adding the following two lines in the controller.
