


PHP graphics class for convenient watermarks and thumbnails_PHP tutorial
/*
*@author 27262681@qq.com
*copyright http://www.gowake.cn
*/
class img {
function __construct($arg = null) {
$args = func_get_args();
if($arg == null) {
return null;
}
$this->im = call_user_func_array(array($this,'create'),$args);
}
function __call($func,$arg) {
if(function_exists('image'.$func)) {
$funcstr = 'image'.$func;
}elseif (function_exists($func)){
$funcstr = $func;
} else {
error("No such class method or function"); /*
*Create image
*@param string/int Image file path or width
*@param int Height, can be omitted
*@param string 6-digit hexadecimal integer
*/
function create($arg = null) {
$args = func_get_args();
if(is_file($args[0])) {
$this->file = $args[0 ];
$size = getimagesize($this->file) or error('Picture type error'); : $size;
$type = image_type_to_extension($size[2],false);
$this->type = $this->type ? > $createfunc = 'imagecreatefrom'.$type;
$im = $createfunc($this->file); args[1]>0) {
$im = imagecreatetruecolor((int)$args[0],(int)$args[1]) or error("Sorry, the parameter is wrong! "); " else {
$color = hexdec(str_replace('#','',$args[2]));
}
$this->size = $this->size ? $this->size : array((int)$args[0] ,(int)$args[1]);
imagefill($im, 1, 1, $color);
, parameter error!");
}
//imagealphablending($im,false);//These two lines are used to record the transparent channel
imagesavealpha($im,true); );//Enable interlacing
return $im;
}
/*
*Generate thumbnails
*@param int $w Width of new image
* @param int $h The width of the new picture
*@param string/bool $color Optional, the background color of the new picture, false or empty to be transparent
*@param bool $lashen Optional, whether to stretch, The default is not to stretch
*/
function suolue($w = null,$h = null,$color = false,$lashen = false) {
$w_o = imagesx($this->im ); ;
} elseif ($w != null and $h == null){ ;
$im = $this->create($w,$h,$color);
$w_n = $w;
$h_n = $h;
if($w_o/ $h_o > $w/$h) {
$h_n = $w*$h_o/$w_o;
$y = ($h-$h_n)/2;
}elseif ($w_o /$h_o < $w/$h){
if($lashen) {
$w_n = $w; 🎜> imagecopyresampled($ im,$this->im,$x,$y,0,0,$w_n,$h_n,$w_o,$h_o);
//imagedestroy($this->im);
$this->im = $im;
return $im;
}
/*
*Write on the picture
*@param string $str The string to be written
*@param array $arg String related parameters, which is an associative array. left is the distance from the left, right is the distance from the right, left takes precedence, top is the distance from the top, bottom is the distance from the bottom, top takes precedence; angle is the angle, color is the 6-digit hexadecimal color, touming is the text transparency, font For the font file
*/
function write($str = '' , $arg = array()) {
$size = $arg['size'] ? $arg['size'] : 20;
$angle = $arg['angle'] ? $arg['angle'] : 0 ;
$color = $arg['color'] ? $arg['color'] : '000000 ';
$touming = $arg['touming'] ? $arg['touming'] : 100;
$touming = dechex((100-$touming)*127/100);
$ color = hexdec($touming.str_replace("#","",$color));
$font = $arg['font'] ? $arg['font'] : 'arial.ttf';
$boxarr = imagettfbbox($size,$angle,$font,$str);
$w = imagesx($this->im);
$h = imagesy($this->im );
$x_l = $x_r = $boxarr[0];
$y_t = $y_b = $boxarr[1];
for($i=0;$i<7; $i = $i+2) {
$x_r ? $boxarr[$i] : $x_r; $boxarr[$i+1] > $y_b ? $boxarr[$i+1] : $y_b;
}
$width = $x_r - $x_l; $y_t;
/*Get the exact offset*/
$im = $this->create($width*4,$height*4);
$tm = hexdec( '7ffffffff');
imagettftext($im,$size,$angle,$width*2,$height*2,$color,$font,$str);
for($i=0;$ i<$width*4;$i++) {
for($ii=0;$ii<$height*4;$ii++) {
if(imagecolorat($im,$i,$ii) ! = $tm) {
4;$i++) {
for($ii=$x_l;$ii<$width*4;$ii++) {
if(imagecolorat($im,$ii,$i) != $tm) {<> $ Y_t = $ i;
Break (2);
}
}
}
for($i=$width*4-1;$i>0;$i--) {
for($ii=$y_t;$ii<$height*4;$ii++) {
if(imagecolorat($im,$i,$ii) != $tm) {
$x_r = $i;
break(2);
}
}
}
for($i=$height*4-1;$i>0;$i--) {
for($ii=$x_l;$ii<=$x_r;$ii++) {
if(imagecolorat($im,$ii,$i) != $tm) {
$y_b = $i;
break(2);
}
}
}
$x_off = $x_l - $width*2;
$y_off = $y_b - $height*2;
$width = $x_r - $x_l; //精确宽度
$height = $y_b - $y_t; //精确高度
imagedestroy($im);
if(isset($arg['left'])) {
$x = (int)$arg['left'] - $x_off;
}elseif (isset($arg['right'])){
$x = $w - (int)$arg['right'] - $width - $x_off;
}else {
$x = ($w - $width)/2 - $x_off;
}
if(isset($arg['top'])) {
$y = (int)$arg['top'] - $y_off + $height;
}elseif (isset($arg['bottom'])){
$y = $h - (int)$arg['bottom'] - $y_off;
}else {
$y = ($h + $height)/2 - $y_off;
}
imagettftext($this->im,$size,$angle,$x,$y,$color,$font,$str);
return $this->im;
}
/*
*合并图片(如图片水影)
*@param string/resource $file 图片文件路径或这图片标识符
*@param array $arg 字符串相关的参数,为一个关联数组,left 为距左边距离,right为距右边距离,left优先,top为距顶部距离,bottom为距底部距离,top优先;touming为文字透明度
*/
function merge($file,$arg = array()) {
if(is_file($file)) {
$imc = $this->create($file);
}elseif(gettype($file)=='resource') {
$imc = $file;
}else {
error("没有图片");
}
$touming = $arg['touming'] ? (int)$arg['touming'] : 100 ;
$w = imagesx($this->im);
$h = imagesy($this->im);
$width = imagesx($imc);
$height = imagesy($imc);
if(isset($arg['left'])) {
$x = (int)$arg['left'];
}elseif (isset($arg['right'])){
$x = $w - (int)$arg['right'] - $width;
}else {
$x = ($w - $width)/2;
}
if(isset($arg['top'])) {
$y = (int)$arg['top'];
}elseif (isset($arg['bottom'])){
$y = $h - $height - $arg['bottom'];
}else {
$y = ($h - $height)/2;
}
imagecopymergegray($this->im,$imc,$x,$y,0,0,$width,$height,$touming);
}
/*
*输出图片
*@param string $type
*@param string $filename 要转存的文件路径
*@param int $zhiliang jpeg图片特有的,图像清晰度
*/
function display($type = null,$filename = null,$zhiliang = null) {
if($type == null) {
$type = $this->type ? $this->type : 'jpg';
}
if(($type == 'jpeg' or $type == 'jpg') and $zhiliang == null) {
$type = 'jpeg';
$zhiliang = 100;
}
if($filename == null) {
header('Content-type: image/'.$type);
}
$displayfunc = 'image'.$type;
$displayfunc($this->im,$filename,$zhiliang);
imagedestroy($this->im);
}
function randcolor($a,$b) {
$a = $a>255 ? 255 : (int)$a;
$a = $a<0 ? 0 : (int)$a;
$b = $b>255 ? 255 : (int)$b;
$b = $b<0 ? 0 : (int)$b;
for($i=0;$i<3;$i++) {
$color .= str_pad(dechex(mt_rand($a,$b)), 2, "0", STR_PAD_LEFT);
}
return $color;
}
}
/*
function error($msg,$debug = false) {
$err = new Exception($msg);
$str = "
n<span style="color:red" style="color:red">错误:</span>n".print_r($err->getTrace(),1)."n";
if($debug == true) {
file_put_contents(date('Y-m-d').".log",$str);
return $str;
}else{
die($str);
}
}
*/
?>
这是简单的用法实例
$img = new img('a.png');
$m = $img->im;
$im = $img->suolue(100);
$img->im = $m;
$img->suolue(300);
$img->merge($m,array('left'=>0,'top'=>0,'touming'=>60));
$img->merge($im,array('right'=>0,'top'=>0,'touming'=>60));
$img->merge($im,array('left'=>0,'bottom'=>0,'touming'=>60));
$img->merge($im,array('right'=>0,'bottom'=>0,'touming'=>60));
$img->write("春天来了",array('left'=>0,'top'=>0,'size'=>30,'color'=>$img->randcolor(0,180),'angle'=>-45,'font'=>'simfang.ttf','touming'=>80));
$img->write("春天来了",array('left'=>0,'bottom'=>0,'size'=>30,'color'=>$img->randcolor(0,180),'angle'=>45,'font'=>'simfang.ttf','touming'=>80));
$img->write("春天来了",array('right'=>0,'bottom'=>0,'size'=>30,'color'=>$img->randcolor(0,180),'angle'=>-45,'font'=>'simfang.ttf','touming'=>80));
$img->write("春天来了",array('right'=>0,'top'=>0,'size'=>30,'color'=>$img->randcolor(0,180),'angle'=>45,'font'=>'simfang.ttf','touming'=>80));
$img->display("gif");

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


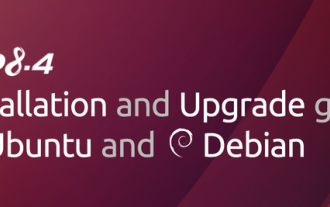
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
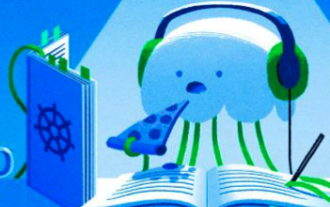
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
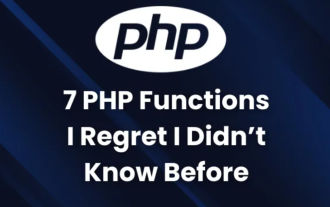
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
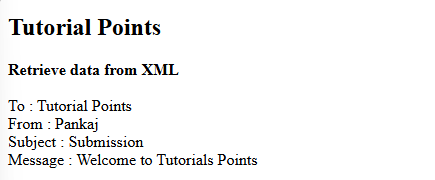
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
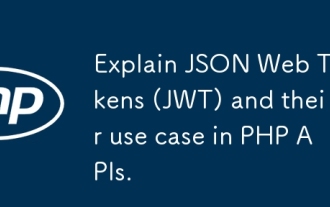
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
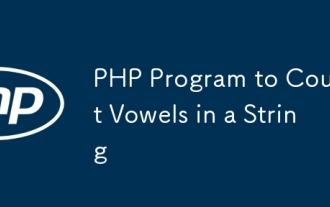
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
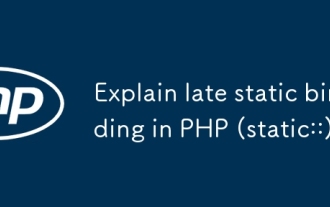
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
