PHP class code for generating thumbnails_PHP tutorial
/**
* Function: Generate thumbnails
* Author: phpox
* Date: Thu May 17 09:57:05 CST 2007
*/
class CreatMiniature
{
//Public variables
var $srcFile=""; //Original Picture
var $echoType; //Output picture type, link--not saved as a file; file--saved as a file
var $im=""; //Temporary variable
var $srcW=" "; //Original image width
var $srcH=""; //Original image height
//Set variables and initialization
function SetVar($srcFile,$echoType)
{
if (!file_exists($srcFile)){
echo 'The source image file does not exist!';
exit();
}
$this->srcFile=$srcFile;
$this->echoType=$echoType;
$info = "";
$data = GetImageSize($this->srcFile,$info);
switch ($ data[2])
{
case 1:
if(!function_exists("imagecreatefromgif")){
echo "Your GD library cannot use GIF format images, please use Jpeg or PNG Format! Return";
exit();
}
$this->im = ImageCreateFromGIF( $this->srcFile);
break;
case 2:
if(!function_exists("imagecreatefromjpeg")){
echo "Your GD library cannot use images in jpeg format, please Use images in other formats!返回";
exit();
}
$this->im = ImageCreateFromJpeg($this->srcFile);
break;
case 3:
$this->im = ImageCreateFromPNG($this->srcFile);
break;
}
$this->srcW=ImageSX($this->im);
$this->srcH=ImageSY($this->im);
}
//生成扭曲型缩图
function Distortion($toFile,$toW,$toH)
{
$cImg=$this->CreatImage($this->im,$toW,$toH,0,0,0,0,$this->srcW,$this->srcH);
return $this->EchoImage($cImg,$toFile);
ImageDestroy($cImg);
}
//生成按比例缩放的缩图
function Prorate($toFile,$toW,$toH)
{
$toWH=$toW/$toH;
$srcWH=$this->srcW/$this->srcH;
if($toWH<=$srcWH)
{
$ftoW=$toW;
$ftoH=$ftoW*($this->srcH/$this->srcW);
}
else
{
$ftoH=$toH;
$ftoW=$ftoH*($this->srcW/$this->srcH);
}
if($this->srcW>$toW||$this->srcH>$toH)
{
$cImg=$this->CreatImage($this->im,$ftoW,$ftoH,0,0,0,0,$this->srcW,$this->srcH);
return $this->EchoImage($cImg,$toFile);
ImageDestroy($cImg);
}
else
{
$cImg=$this->CreatImage($this->im,$this->srcW,$this->srcH,0,0,0,0,$this->srcW,$this->srcH);
return $this->EchoImage($cImg,$toFile);
ImageDestroy($cImg);
}
}
//生成最小裁剪后的缩图
function Cut($toFile,$toW,$toH)
{
$toWH=$toW/$toH;
$srcWH=$this->srcW/$this->srcH;
if($toWH<=$srcWH)
{
$ctoH=$toH;
$ctoW=$ctoH*($this->srcW/$this->srcH);
}
else
{
$ctoW=$toW;
$ctoH=$ctoW*($this->srcH/$this->srcW);
}
$allImg=$this->CreatImage($this->im,$ctoW,$ctoH,0,0,0,0,$this->srcW,$this->srcH);
$cImg=$this->CreatImage($allImg,$toW,$toH,0,0,($ctoW-$toW)/2,($ctoH-$toH)/2,$toW,$toH);
return $this->EchoImage($cImg,$toFile);
ImageDestroy($cImg);
ImageDestroy($allImg);
}
//生成背景填充的缩图
function BackFill($toFile,$toW,$toH,$bk1=255,$bk2=255,$bk3=255)
{
$toWH=$toW/$toH;
$srcWH=$this->srcW/$this->srcH;
if($toWH<=$srcWH)
{
$ftoW=$toW;
$ftoH=$ftoW*($this->srcH/$this->srcW);
}
else
{
$ftoH=$toH;
$ftoW=$ftoH*($this->srcW/$this->srcH);
}
if(function_exists("imagecreatetruecolor"))
{
@$cImg=ImageCreateTrueColor($toW,$toH);
if(!$cImg)
{
$cImg=ImageCreate($toW,$toH);
}
}
else
{
$cImg=ImageCreate($toW,$toH);
}
$backcolor = imagecolorallocate($cImg, $bk1, $bk2, $bk3); //填充的背景颜色
ImageFilledRectangle($cImg,0,0,$toW,$toH,$backcolor);
if($this->srcW>$toW||$this->srcH>$toH)
{
$proImg=$this->CreatImage($this->im,$ftoW,$ftoH,0,0,0,0,$this->srcW,$this->srcH);
if($ftoW<$toW)
{
ImageCopy($cImg,$proImg,($toW-$ftoW)/2,0,0,0,$ftoW,$ftoH);
}
else if($ftoH<$toH)
{
ImageCopy($cImg,$proImg,0,($toH-$ftoH)/2,0,0,$ftoW,$ftoH);
}
else
{
ImageCopy($cImg,$proImg,0,0,0,0,$ftoW,$ftoH);
}
}
else
{
ImageCopyMerge($cImg,$this->im,($toW-$ftoW)/2,($toH-$ftoH)/2,0,0,$ftoW,$ftoH,100);
}
return $this->EchoImage($cImg,$toFile);
ImageDestroy($cImg);
}
function CreatImage($img,$creatW,$creatH,$dstX,$dstY,$srcX,$srcY,$srcImgW,$srcImgH)
{
if(function_exists("imagecreatetruecolor"))
{
@$creatImg = ImageCreateTrueColor($creatW,$creatH);
if($creatImg)
ImageCopyResampled($creatImg,$img,$dstX,$dstY,$srcX,$srcY,$creatW,$creatH,$srcImgW,$srcImgH);
else
{
$creatImg=ImageCreate($creatW,$creatH);
ImageCopyResized($creatImg,$img,$dstX,$dstY,$srcX,$srcY,$creatW,$creatH,$srcImgW,$srcImgH);
}
}
else
{
$creatImg=ImageCreate($creatW,$creatH);
ImageCopyResized($creatImg,$img,$dstX,$dstY,$srcX,$srcY,$creatW,$creatH,$srcImgW,$srcImgH);
}
return $creatImg;
}
//输出图片,link---只输出,不保存文件。file--保存为文件
function EchoImage($img,$to_File)
{
switch($this->echoType)
{
case "link":
if(function_exists('imagejpeg')) return ImageJpeg($img);
else return ImagePNG($img);
break;
case "file":
if(function_exists('imagejpeg')) return ImageJpeg($img,$to_File);
else return ImagePNG($img,$to_File);
break;
}
}
}
?>

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


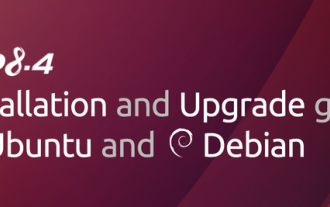
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
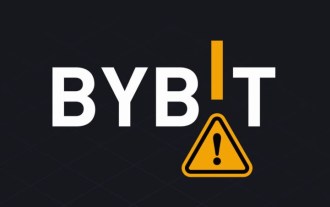
The way to update ByBit exchanges varies by platform and device: Mobile: Check for updates and install in the app store. Desktop Client: Check for updates in the Help menu and install automatically. Web page: You need to manually access the official website for updates. Failure to update the exchange can lead to security vulnerabilities, functional limitations, compatibility issues and reduced transaction execution efficiency.
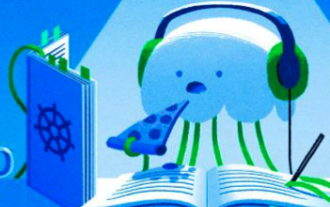
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
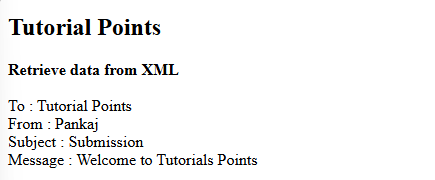
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
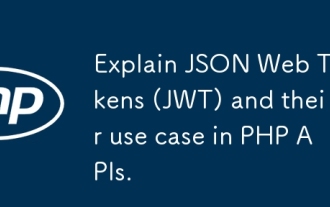
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
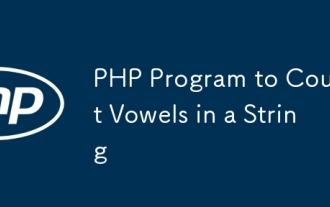
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
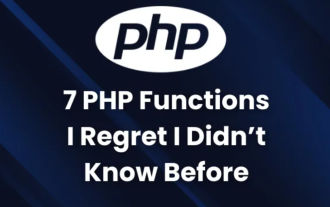
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
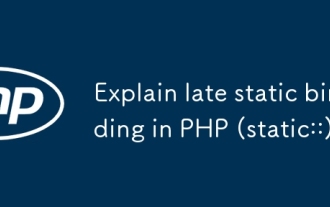
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
